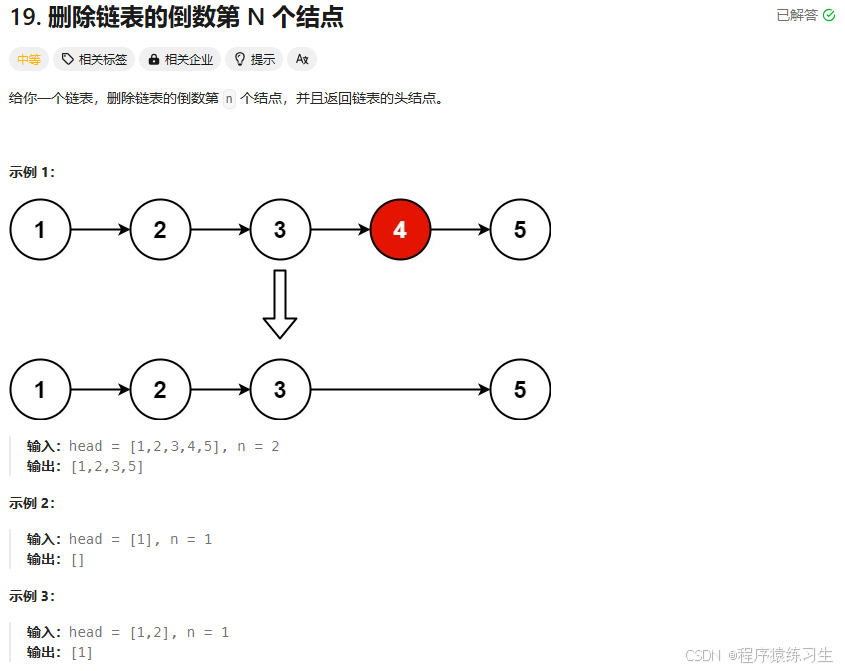
绝妙!快慢指针法,快指针先走n步(复杂度O(n),O(1)):
cpp
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
ListNode* removeNthFromEnd(ListNode* head, int n) {
//设定快慢指针,速度都是1,但是快指针先走n步,慢指针再同时出发,这样一来快指针到结尾时,慢指针到达指定位置
ListNode* fast = head;
ListNode* slow = head;
for(int i = 0; i < n; i++) fast = fast->next;
if(!fast) return head->next;//n=节点数时,不用移动了,特殊情况直接返回头节点的下一个
while(fast && fast->next)
{
fast = fast->next;
slow = slow->next;
}//循环结束的slow就是答案要求的起始位置
if(!slow->next) return nullptr;
slow->next = slow->next->next;
return head;
}
};