文章目录
同步编程&异步编程
在Java并发编程中,同步和异步是两种常见的处理方式。
同步是指多个任务按顺序执行,一个任务的执行需要等待上一个任务的完成。而异步则是指多个任务可以并行执行,不需要等待上一个任务完成。
在多线程编程中,同步是指多个线程按顺序执行,一个线程的执行需要等待上一个线程的完成;而异步是指多个线程可以并发执行,不需要等待其他线程的完成。
在实际应用中,同步通常适用于简单的线性任务,比如IO操作、数据库读写等;而异步通常适用于复杂的并发任务,比如并行计算、大规模数据处理等。
线程池
为什么要使用线程池?
当创建使用线程,需要进行new Thread操作,在JVM的堆内存中创建新的对象地址。
当使用完毕后,需要等待JVM的GC操作,检索Root根释放堆中的垃圾信息。
频繁的创建线程和销毁垃圾,极大的消耗了系统的资源,降低了处理其他业务的性能问题。
创建一个池,保存初始化的线程,用的时候取,不用的时候放入池中等待下次使用。
减少创建的线程数。
Spring自定义线程池
java
/**
* @author linghu
* 自定义线程池配置
* 需要使用线程池,则开启当前配置
*/
@Configuration
public class ThreadPoolConfig {
@Bean
public ThreadPoolExecutor myThreadPool(){
ThreadPoolExecutor threadPoolExecutor = new ThreadPoolExecutor(
2,//核心线程数,一直都能工作的数量
5,//请求处理大的时候,可以开放的最大工作数、最大线程数
3,//开启最大工作数后,当无请求时,还让其存活的时间、线程活跃时间
TimeUnit.SECONDS,//存活时间的单位
new LinkedBlockingDeque<>(10),//阻塞队列,保存操作请求线程
Executors.defaultThreadFactory(),//创建线程的工厂类
new ThreadPoolExecutor.AbortPolicy()//设置拒绝策略
);
return threadPoolExecutor;
}
}
具体案例:
java
@RestController
public class TestController {
@Autowired
private ThreadPoolExecutor threadPoolExecutor;
@Autowired
private MyService myService;
@RequestMapping("/getThreadPoolInfo")
public void getThreadPoolInfo(){
System.out.println("getActiveCount--"+threadPoolExecutor.getActiveCount());
System.out.println("getCorePoolSize--"+threadPoolExecutor.getCorePoolSize());
System.out.println("getPoolSize--"+threadPoolExecutor.getPoolSize());
}
@RequestMapping("/test1")
public String test1(){
System.out.println("进入test1 "+System.currentTimeMillis());
/** 这种调用其实比较复杂,还有更简单的调用法则 */
threadPoolExecutor.execute(()->{
try {
System.out.println("线程池处理数据 start "+System.currentTimeMillis());
TimeUnit.SECONDS.sleep(3);
System.out.println("线程池处理数据 end "+System.currentTimeMillis());
} catch (InterruptedException e) {
e.printStackTrace();
}
});
System.out.println("线程池调用结束 "+System.currentTimeMillis());
return "6666";
}
}
测试:


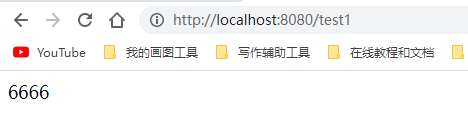
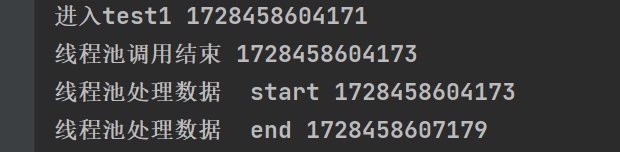
上面自定义的线程池能够实现异步调用执行方法。
SpringBoot轻松调用线程池
SpringBoot自定义线程池同上面的Spring,只不过多了几个注解简化了一些操作。SpringBoot能够通过这个注解更轻松的调用线程池。
我们将类上添加注解@EnableAsync
开启异步调用,让在需要使用的地方,直接使用@Async
就可以了。
java
@Configuration
@EnableAsync//注解开启异步调用Async
public class ThreadPoolConfig {
@Bean
public ThreadPoolExecutor myThreadPool(){
ThreadPoolExecutor threadPoolExecutor = new ThreadPoolExecutor(
2,//核心线程数,一直都能工作的数量
5,//请求处理大的时候,可以开放的最大工作数、最大线程数
3,//开启最大工作数后,当无请求时,还让其存活的时间、线程活跃时间
TimeUnit.SECONDS,//存活时间的单位
new LinkedBlockingDeque<>(10),//阻塞队列,保存操作请求线程
Executors.defaultThreadFactory(),//创建线程的工厂类
new ThreadPoolExecutor.AbortPolicy()//设置拒绝策略
);
return threadPoolExecutor;
}
}
然后编写一个测试类,同时也需要将具体的异步业务单独独立出来:
java
@RequestMapping("/test2")
public void test2() throws InterruptedException {
System.out.println("进入test2 "+System.currentTimeMillis());
myService.service1();
System.out.println("调用结束 "+System.currentTimeMillis());
}
服务类的代码如下所示:
java
// 具体使用哪个线程池
@Async("myThreadPoolExecutor")
public void service1() throws InterruptedException {
System.out.println("----- service1 start --------"+System.currentTimeMillis());
TimeUnit.SECONDS.sleep(3);
System.out.println("----- service1 end --------"+System.currentTimeMillis());
}
执行请求:
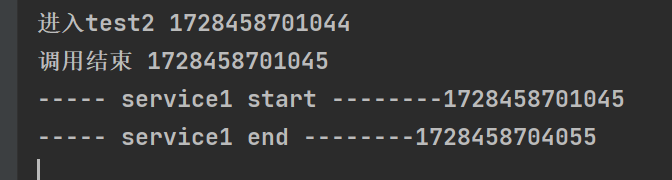
总结
我们其实用注解 @Async("myThreadPoolExecutor")
取代了
java
threadPoolExecutor.execute(()->{
try {
System.out.println("线程池处理数据 start "+System.currentTimeMillis());
TimeUnit.SECONDS.sleep(3);
System.out.println("线程池处理数据 end "+System.currentTimeMillis());
} catch (InterruptedException e) {
e.printStackTrace();
}
});