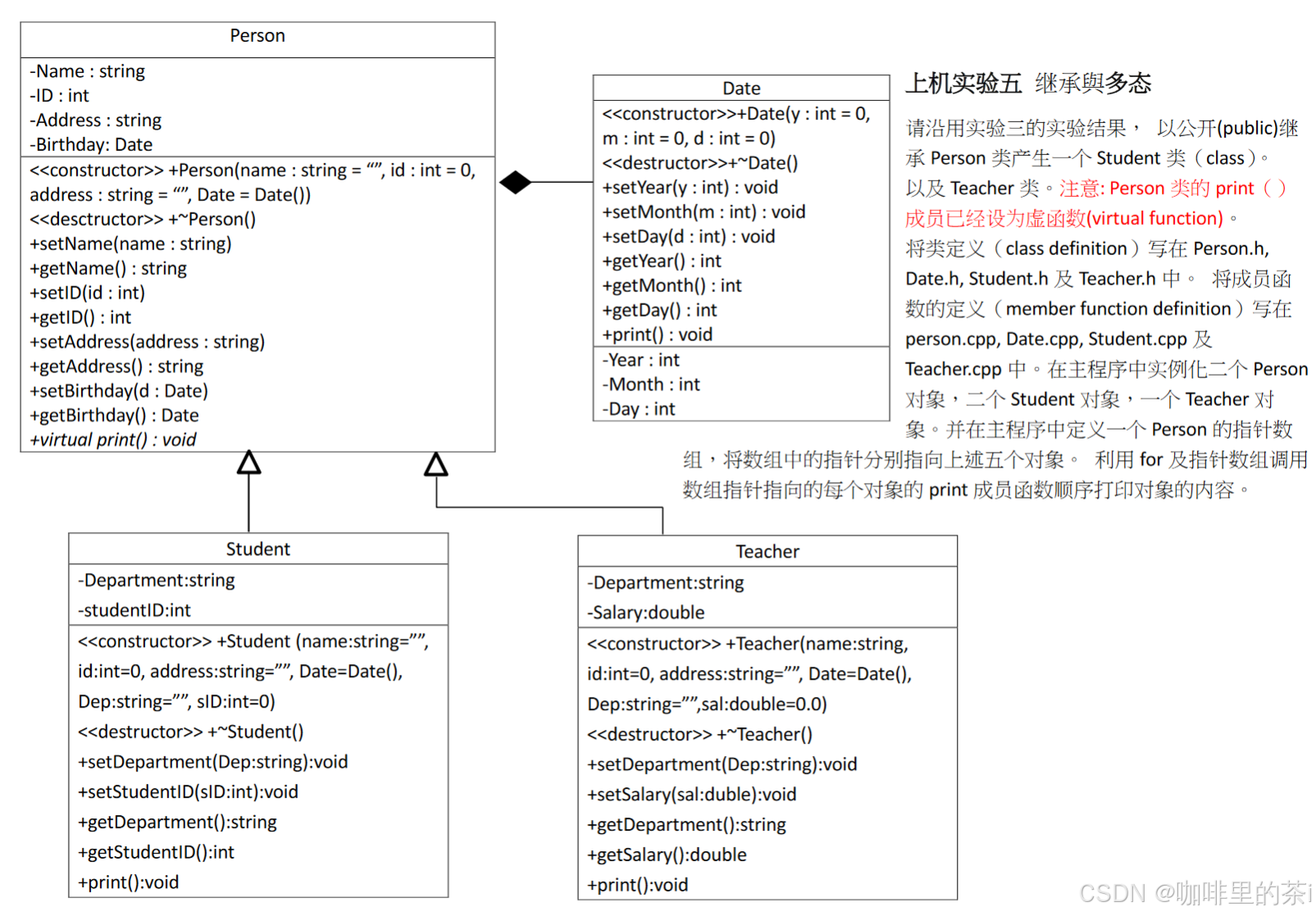
main.cpp
cpp
#include <iostream>
#include "Person.h"
#include "Student.h"
#include "Teacher.h"
using namespace std;
int main()
{
Person p1("Alice", 1, "123 Street");
Person p2("Bob", 2, "456 Avenue");
Student s1("Charlie", 3, "789 Boulevard", Date(2000, 3, 1), "Math", 1001);
Student s2("David", 4, "101 Lane", Date(2001, 7, 22), "Physics", 1002);
Teacher t1("Eve", 5, "202 Road", Date(1975, 1, 1), "Biology", 50000);
Person* people[] = {&p1, &p2, &s1, &s2, &t1}; // 容器
for (const auto& person : people) // output
{
person->print();
}
return 0;
}
Person.h
cpp
#ifndef PERSON_H
#define PERSON_H
#include <iostream>
#include "Date.h"
using namespace std;
class Person
{
public:
Person();
Person(string name, int id, string address);
~Person();
void setPerson(string name, int id, string address);
void setName(string name);
void setID(int id);
void setAddress(string address);
void setBirthday(Date d);
string getName();
int getID();
string getAddress();
Date getBirthday();
virtual void print(); // outPutResult
private:
string Name;
int ID;
string Address;
Date Birthday;
};
#endif // PERSON_H
Student.h
cpp
#ifndef STUDENT_H
#define STUDENT_H
#include <iostream>
#include "Person.h"
#include "Date.h"
using namespace std;
class Student :public Person
{
public:
Student();
Student(string name, int id, string address, Date date, string department, int studentID);
~Student();
void setDepartment(string department);
void setStudentID(int studentID);
string getDepartment();
int getStudentID();
virtual void print(); // outPutResult
private:
string Department;
int StudentID;
};
#endif // STUDENT_H
Teacher.h
cpp
#ifndef TEACHER_H
#define TEACHER_H
#include "Person.h"
#include "Date.h"
using namespace std;
class Teacher :public Person
{
public:
Teacher();
Teacher(string name, int id, string address, Date date, string department, double salary);
~Teacher();
void setDepartment(string department);
void setSalary(double salary);
string getDepartment();
double getSalary();
virtual void print(); // outPutResult
private:
string Department;
double Salary;
};
#endif // TEACHER_H
Person.cpp
cpp
#include "Person.h"
#include <iostream>
using namespace std;
Person::Person()
{
Name = "S.M.Wang";
ID = 070145;
Address = "莲花路200号";
}
Person::Person(string name, int id, string address)
{
setPerson(name, id, address);
}
Person::~Person()
{
//cout << "object Destructor is called" << endl;
}
void Person::setPerson(string name, int id, string address)
{
Name = name;
ID = id;
Address = address;
}
void Person::setName(string name)
{
Name = name;
}
void Person::setID(int id)
{
ID = id;
}
void Person::setAddress(string address)
{
Address = address;
}
string Person::getName()
{
return Name;
}
int Person::getID()
{
return ID;
}
string Person::getAddress()
{
return Address;
}
void Person::setBirthday(Date d) // 调用的形参是类
{
Birthday = d;
}
Date Person::getBirthday() // 返回的是类
{
return Birthday;
}
void Person::print()
{
cout << "Name:" << getName() << endl;
cout << "ID:" << getID() << endl;
cout << "Address:" << getAddress() << endl;
cout << "Birthday:" << getBirthday().getYear(); // getBirthday()返回的是类,再调用类中的子函数满足cout的返回值。
cout << " " << getBirthday().getMonth();
cout << " " << getBirthday().getDay() << endl;
cout << endl;
}
Student.cpp
cpp
#include "Student.h"
#include <iostream>
using namespace std;
Student::Student()
{
}
Student::Student(string name, int id, string address, Date date, string department, int studentID)
{
setPerson(name, id, address);
setBirthday(date);
setDepartment(department);
setStudentID(studentID);
}
Student::~Student()
{
//cout << "object Destructor is called" << endl;
}
void Student::setDepartment(string department)
{
Department = department;
}
string Student::getDepartment()
{
return Department;
}
void Student::setStudentID(int studentID)
{
StudentID = studentID;
}
int Student::getStudentID()
{
return StudentID;
}
void Student::print()
{
cout << "Name:" << getName() << endl;
cout << "ID:" << getID() << endl;
cout << "Address:" << getAddress() << endl;
cout << "Birthday:" << getBirthday().getYear(); // getBirthday()返回的是类,再调用类中的子函数满足cout的返回值。
cout << " " << getBirthday().getMonth();
cout << " " << getBirthday().getDay() << endl;
cout << "Department:" << getDepartment() << endl;
cout << "StudentID:" << getStudentID() << endl;
cout << endl;
}
Teacher.cpp
cpp
#include "Teacher.h"
#include <iostream>
using namespace std;
Teacher::Teacher()
{
}
Teacher::Teacher(string name, int id, string address, Date date, string department, double salary)
{
setPerson(name, id, address);
setBirthday(date);
setDepartment(department);
setSalary(salary);
}
Teacher::~Teacher()
{
//cout << "object Destructor is called" << endl;
}
void Teacher::setDepartment(string department)
{
Department = department;
}
string Teacher::getDepartment()
{
return Department;
}
void Teacher::setSalary(double salary)
{
Salary = salary;
}
double Teacher::getSalary()
{
return Salary;
}
void Teacher::print()
{
cout << "Name:" << getName() << endl;
cout << "ID:" << getID() << endl;
cout << "Address:" << getAddress() << endl;
cout << "Birthday:" << getBirthday().getYear(); // getBirthday()返回的是类,再调用类中的子函数满足cout的返回值。
cout << " " << getBirthday().getMonth();
cout << " " << getBirthday().getDay() << endl;
cout << "Department:" << getDepartment() << endl;
cout << "Salary:" << getSalary() << endl;
cout << endl;
}
Result:
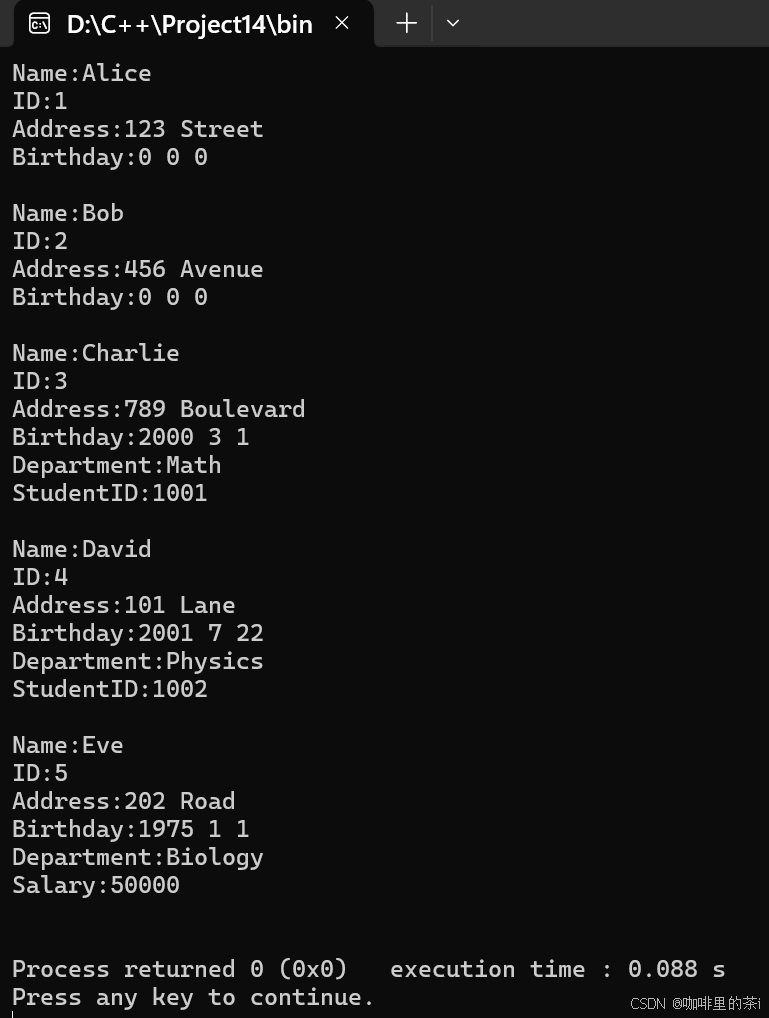