1. C++ 中 new 和delete 的基本用法
在 C++ 中,new 和 delete是用来动态分配和释放内存的关键字,它们是面向对象的替代方式,提供了比 C 语言更优雅的内存管理工具。
1.1 **new
**的使用
new 用于从堆中分配内存,并且自动 调用对象的构造函数来初始化分配的内存。
int* p = new int; // 分配一个整数 int* arr = new int[5]; // 分配一个整数数组 class MyClass { public: MyClass() { /* 构造函数 */ } }; MyClass* obj = new MyClass(); // 分配一个 MyClass 对象并调用构造函数
1.2 delete
的使用
delete 用于释放由 new分配的内存,并调用对象的析构函数来进行清理工作。
delete p; // 释放单个整数的内存 delete[] arr; // 释放数组内存 delete obj; // 释放 MyClass 对象的内存并调用析构函数
delete 和 delete[] 是不同的操作符,**delete[]**主要用于释放数组的内存
2. C 语言中的 malloc
和 **free
**的基本用法
2.1 malloc 的使用
malloc 函数用于从堆中分配一块连续的内存,返回指向这块内存的指针。
int* p = (int*)malloc(sizeof(int)); // 分配一个整数大小的内存 int* arr = (int*)malloc(5 * sizeof(int)); // 分配一个大小为5的整数数组
2.2 free 的使用
free 函数用于释放由 malloc分配的内存。
free(p); // 释放单个整数内存 free(arr); // 释放数组内存
需要注意的是,
malloc
只分配内存,不会初始化内存中的内容,free
也不会执行析构函数之类的清理工作。
3. new/delete 和 malloc/free 的区别
3.1 语法与功能上的区别
-
new/delete 是运算符,而 malloc/free 是函数。
-
new 会调用类的构造函数,delete会调用析构函数
-
malloc 和 free仅仅分配和释放内存,不涉及对象初始化和清理。
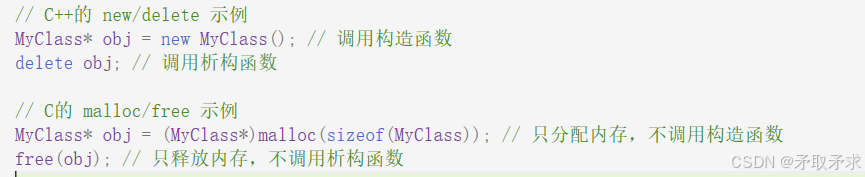
3.2 类型安全
new不需要显式类型转换,因为它返回正确的类型指针。
malloc 返回**
void*
**,因此需要进行显式类型转换。// C++ 使用 new,类型转换是隐式的 int* p = new int; // C 使用 malloc,需要显式转换 int* p = (int*)malloc(sizeof(int));
3.3⚠️⚠️⚠️内存分配失败的处理方式
-
new 在内存分配失败时会抛出异常,可以捕获进行错误处理。
-
malloc 在分配失败时返回 NULL,需要检查返回值来判断是否成功。
-
try { int* p = new int[10000000000]; // 分配内存失败会抛出异常 } catch (std::bad_alloc& e) { std::cerr << "Memory allocation failed: " << e.what() << std::endl; } int* p = (int*)malloc(10000000000 * sizeof(int)); if (p == NULL) { printf("Memory allocation failed\n"); }
4,两种代码对比直接下拉
🌟🌟🌟 整数🌟🌟🌟
使用
new/delete
:int* p = new int(42); // 分配一个整数并初始化为42 std::cout << *p << std::endl; // 输出:42 delete p; // 释放内存
使用
malloc/free
:int* p = (int*)malloc(sizeof(int)); *p = 42; // 初始化内存 printf("%d\n", *p); // 输出:42 free(p); // 释放内存
🌟🌟🌟数组 🌟🌟🌟
使用
new/delete[]
:int* arr = new int[5] {1, 2, 3, 4, 5}; for (int i = 0; i < 5; ++i) { std::cout << arr[i] << " "; // 输出:1 2 3 4 5 } delete[] arr; // 释放数组内存
使用
malloc/free
:int* arr = (int*)malloc(5 * sizeof(int)); for (int i = 0; i < 5; ++i) { arr[i] = i + 1; printf("%d ", arr[i]); // 输出:1 2 3 4 5 } free(arr); // 释放数组内存
代码对比
#include <iostream> #include <new> // std::bad_alloc #include <cstdio> // printf int main() { try { int* p = new int[100000000]; // 测试时使用较小值,如 100000000 std::cout << "Memory allocation successful using new!" << std::endl; delete[] p; // 记得释放内存 } catch (std::bad_alloc& e) { std::cerr << "Memory allocation failed: " << e.what() << std::endl; } int* q = (int*)malloc(100000000 * sizeof(int)); // 测试时使用较小值,如 100000000 if (q == NULL) { printf("Memory allocation failed using malloc\n"); } else { printf("Memory allocation successful using malloc!\n"); free(q); // 记得释放内存 } return 0; }
复盘🐂
-
new/delete
是 C++ 专有的动态内存管理工具,它们不仅分配和释放内存,还会调用构造函数和析构函数,适合用于面向对象编程。 -
malloc/free
是 C 语言中的动态内存管理函数,它们只负责分配和释放内存,不会进行对象的初始化和清理。 -
new
具有类型安全性 ,而malloc
需要显式类型转换。 -
在内存分配失败时 ,
new
抛出异常,而malloc
返回NULL
。
c++ 中空的理解
共勉 💪
同为未来的it人让我们在共同进步吧。
我很喜欢雷军的一段话:我们就悄悄的干,就算失败了咱也不丢人。