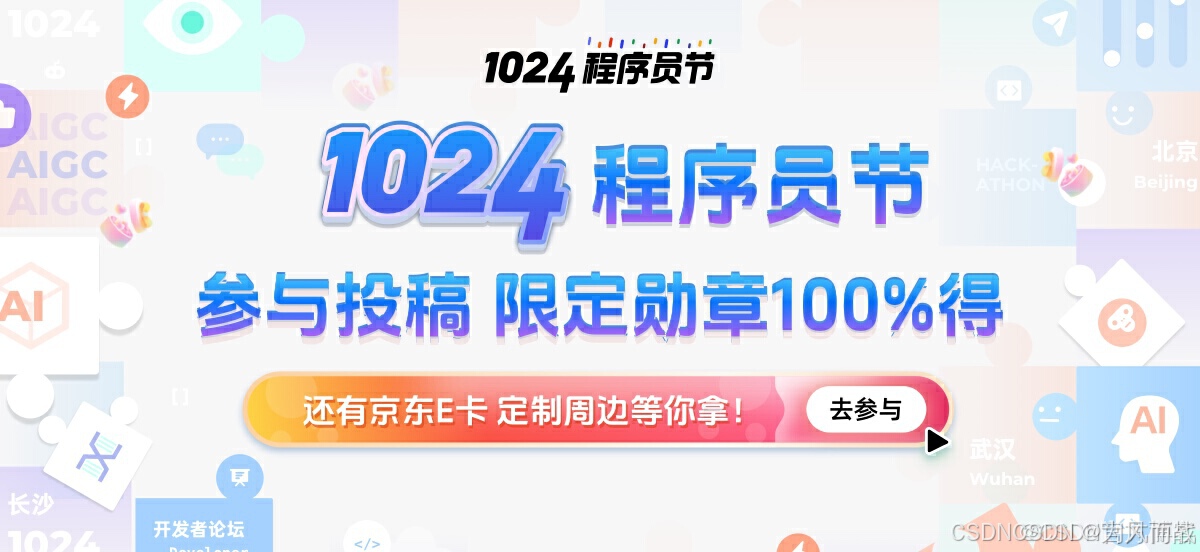
不废话,直接上代码一个简单的示例。
1、事件聚合
创建一个文件夹EventBLL,添加EventDemo.cs,代码如下。
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace WpfApp1.EventBLL
{
public class EventDemo :PubSubEvent<string>
{
}
}
MainWindowViewModel.cs代码如下。
cs
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using WpfApp1.EventBLL;
namespace WpfApp1.ViewModels
{
public class MainWindowViewModel : BindableBase
{
private string _mvvmString = "Hello Prism";
public string MVVMString
{
get { return _mvvmString; }
set { SetProperty(ref _mvvmString, value); }
}
private IEventAggregator _eventAggregator;
public MainWindowViewModel(IEventAggregator ea)
{
_eventAggregator = ea;
_eventAggregator.GetEvent<EventDemo>().Subscribe(GetEvent);
}
private void GetEvent(string str)
{
MVVMString = "接收到:" + str;
}
private DelegateCommand _EventPublishCommand;
public DelegateCommand EventPublishCommand
{
get { return _EventPublishCommand = new DelegateCommand(EventPublish); }
}
private void EventPublish()
{
_eventAggregator.GetEvent<EventDemo>().Publish("123");
}
}
}
MainWindow.xaml 修改代码如下。
XML
<Window x:Class="WpfApp1.Views.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp1"
xmlns:prism="http://prismlibrary.com/"
xmlns:i="http://schemas.microsoft.com/xaml/behaviors"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<StackPanel>
<TextBlock Text="{Binding MVVMString}" FontSize="16" HorizontalAlignment="Center" VerticalAlignment="Center"/>
<Button Content="发布" Command="{Binding EventPublishCommand}" Width="200" Height="30" HorizontalAlignment="Center" Margin="0,0,0,5"/>
</StackPanel>
</Window>
运行效果如下图。
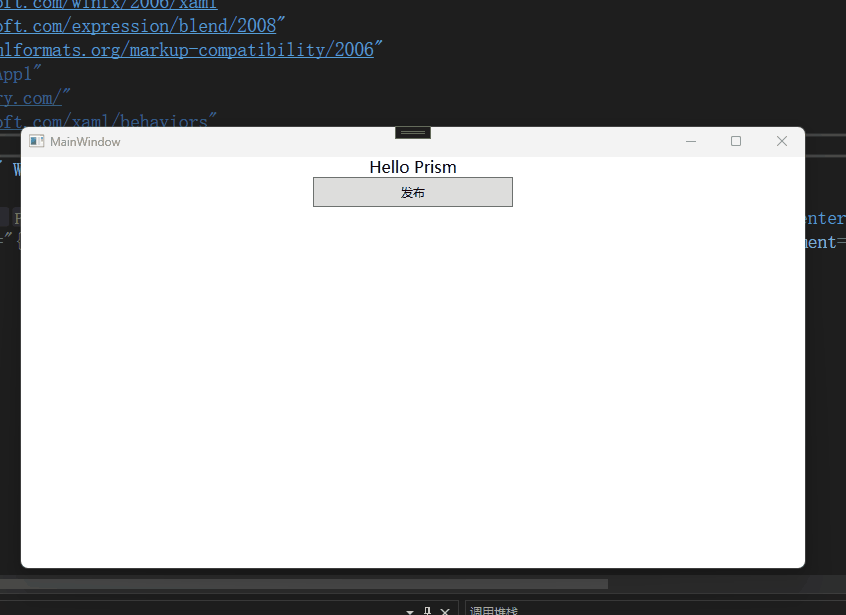
2、订阅过滤
ThreadOption:下列选择可供选择。
PublisherThread: 使用此设置接收发布者线程上的事件。这是默认设置。
BackgroundThread: 使用此设置异步接收A上的事件。网框架线池线。
UIThread: 使用此设置接收UI线程上的事件。
运行效果如图,通过下图可以看出当发布的是123是能够接收到。当发布的内容是23时没有接收到。这是因为订阅中过滤了不包含1的消息。
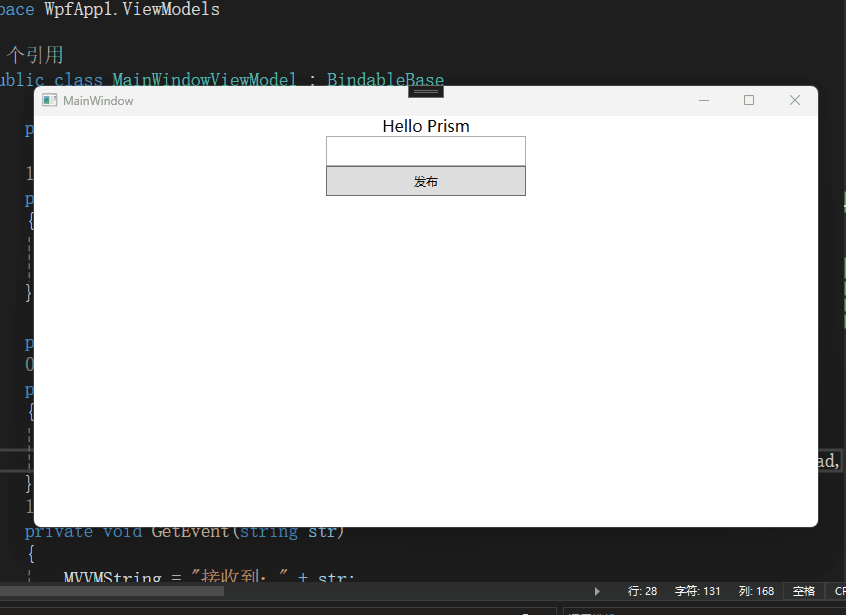
MainWindow.xaml代码如下。
XML
<Window x:Class="WpfApp1.Views.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp1"
xmlns:prism="http://prismlibrary.com/"
xmlns:i="http://schemas.microsoft.com/xaml/behaviors"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<StackPanel>
<TextBlock Text="{Binding MVVMString}" FontSize="16" HorizontalAlignment="Center" VerticalAlignment="Center"/>
<TextBox x:Name="tbMessage" HorizontalAlignment="Center" Width="200" Height="30"/>
<Button Content="发布" Command="{Binding EventPublishCommand}" CommandParameter="{Binding ElementName=tbMessage,Path=Text}" Width="200" Height="30" HorizontalAlignment="Center" Margin="0,0,0,5"/>
</StackPanel>
</Window>
MainWindowViewModel.cs代码如下。
cs
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using WpfApp1.EventBLL;
namespace WpfApp1.ViewModels
{
public class MainWindowViewModel : BindableBase
{
private string _mvvmString = "Hello Prism";
public string MVVMString
{
get { return _mvvmString; }
set { SetProperty(ref _mvvmString, value); }
}
private IEventAggregator _eventAggregator;
public MainWindowViewModel(IEventAggregator ea)
{
_eventAggregator = ea;
_eventAggregator.GetEvent<EventDemo>().Subscribe(GetEvent,ThreadOption.UIThread,false,(filter)=>filter.Contains("1"));
}
private void GetEvent(string str)
{
MVVMString = "接收到:" + str;
}
private DelegateCommand<string> _EventPublishCommand;
public DelegateCommand<string> EventPublishCommand
{
get { return _EventPublishCommand = new DelegateCommand<string>(EventPublish); }
}
private void EventPublish(string str)
{
_eventAggregator.GetEvent<EventDemo>().Publish(str);
}
}
}
可以看到(filter)=>filter.Contains("1"),在这里对订阅进行了过滤,只有包含1的内容才能被接收到。
keepSubscriberReferenceAlive 参数是类型bool :
true ,事件实例保持对订阅实例的强引用,从而不允许它获得垃圾收集。
false(当此参数省略时的默认值),事件对订阅服务器实例保持弱引用,从而允许垃圾收集器在没有其他引用时处理订阅服务器实例。当收集订阅者实例时,事件将自动取消订阅。