目录

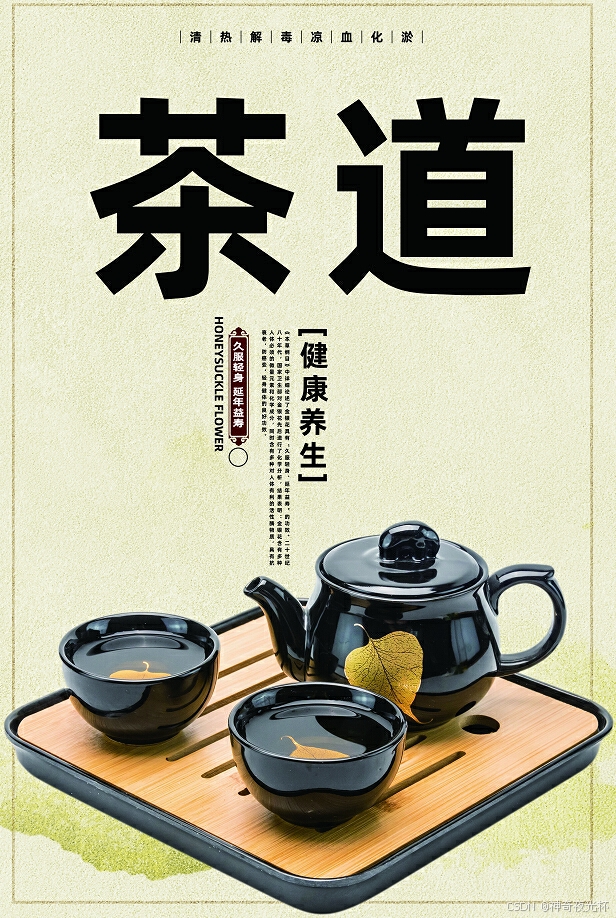

一、用法精讲
876、pandas.Index.duplicated方法
876-1、语法
python
# 876、pandas.Index.duplicated方法
pandas.Index.duplicated(keep='first')
Indicate duplicate index values.
Duplicated values are indicated as True values in the resulting array. Either all duplicates, all except the first, or all except the last occurrence of duplicates can be indicated.
Parameters:
keep
{'first', 'last', False}, default 'first'
The value or values in a set of duplicates to mark as missing.
'first' : Mark duplicates as True except for the first occurrence.
'last' : Mark duplicates as True except for the last occurrence.
False : Mark all duplicates as True.
Returns:
np.ndarray[bool]
876-2、参数
876-2-1、keep**(可选,默认值为'first')****:**字符串,该参数决定在检测重复项时保留哪个重复项,它有三个可选值:
- 'first': 保留第一个出现的重复项,标记其余的为重复。
- 'last': 保留最后一个出现的重复项,标记其余的为重复。
- False: 标记所有重复项为重复。
876-3、功能
用于识别索引中的重复值,它可以帮助你在数据处理中识别和处理重复数据的问题。
876-4、返回值
返回一个与索引长度相同的布尔数组,对于每个元素,如果该元素是重复的且不被保留,则返回True;否则返回False。
876-5、说明
无
876-6、用法
876-6-1、数据准备
python
无
876-6-2、代码示例
python
# 876、pandas.Index.duplicated方法
import pandas as pd
index = pd.Index(['a', 'b', 'c', 'b', 'a', 'd'])
# 保留第一个出现的重复项
print(index.duplicated(keep='first'))
# 保留最后一个出现的重复项
print(index.duplicated(keep='last'))
# 标记所有重复项
print(index.duplicated(keep=False))
876-6-3、结果输出
python
# 876、pandas.Index.duplicated方法
# [False False False True True False]
# [ True True False False False False]
# [ True True False True True False]
877、pandas.Index.equals方法
877-1、语法
python
# 877、pandas.Index.equals方法
pandas.Index.equals(other)
Determine if two Index object are equal.
The things that are being compared are:
The elements inside the Index object.
The order of the elements inside the Index object.
Parameters:
other
Any
The other object to compare against.
Returns:
bool
True if "other" is an Index and it has the same elements and order as the calling index; False otherwise.
877-2、参数
877-2-1、other**(必须)****:**另一个Index对象,用于与当前Index对象进行比较。
877-3、功能
比较当前Index对象与传入的other Index对象是否完全相等,比较的内容包括:
1、两个Index对象的长度是否相同
2、两个Index对象的数据类型是否相同
3、两个Index对象的所有元素是否完全相同(包括元素的顺序)
4、两个Index对象的名称(name属性)是否相同
877-4、返回值
返回一个布尔值:
- 如果两个Index对象完全相等,返回True
- 如果有任何不同,返回False
877-5、说明
无
877-6、用法
877-6-1、数据准备
python
无
877-6-2、代码示例
python
# 877、pandas.Index.equals方法
import pandas as pd
# 创建三个Index对象
index1 = pd.Index([1, 2, 3, 4], name='numbers')
index2 = pd.Index([1, 2, 3, 4], name='numbers')
index3 = pd.Index([1, 2, 3, 5], name='numbers')
# 比较Index对象
print(index1.equals(index2))
print(index1.equals(index3))
877-6-3、结果输出
python
# 877、pandas.Index.equals方法
# True
# False
878、pandas.Index.factorize方法
878-1、语法
python
# 878、pandas.Index.factorize方法
pandas.Index.factorize(sort=False, use_na_sentinel=True)
Encode the object as an enumerated type or categorical variable.
This method is useful for obtaining a numeric representation of an array when all that matters is identifying distinct values. factorize is available as both a top-level function pandas.factorize(), and as a method Series.factorize() and Index.factorize().
Parameters:
sortbool, default False
Sort uniques and shuffle codes to maintain the relationship.
use_na_sentinelbool, default True
If True, the sentinel -1 will be used for NaN values. If False, NaN values will be encoded as non-negative integers and will not drop the NaN from the uniques of the values.
New in version 1.5.0.
Returns:
codesndarray
An integer ndarray that's an indexer into uniques. uniques.take(codes) will have the same values as values.
uniquesndarray, Index, or Categorical
The unique valid values. When values is Categorical, uniques is a Categorical. When values is some other pandas object, an Index is returned. Otherwise, a 1-D ndarray is returned.
Note
Even if there's a missing value in values, uniques will not contain an entry for it.
878-2、参数
878-2-1、sort**(可选,默认值为False)****:**布尔值,如果为True,则会对唯一值进行排序后再进行因子化;如果为False,则保持原有顺序。
878-2-2、use_na_sentinel**(可选,默认值为True)****:**布尔值,如果为True,缺失值(NaN)会被编码为-1;如果为False,缺失值会被编码为一个唯一的整数。
878-3、功能
将Index对象中的唯一值转换为整数索引,该转换过程称为因子化,通常用于将分类数据编码为整数,以便在数据分析和机器学习中更容易处理,通过因子化,可以有效地将字符串或其他非数值数据转换为数值形式,从而简化数据处理和分析过程。
878-4、返回值
返回一个元组(labels,uniques):
- labels:一个整数数组,表示每个元素对应的因子化标签。
- uniques:一个Index对象,包含唯一值。
878-5、说明
无
878-6、用法
878-6-1、数据准备
python
无
878-6-2、代码示例
python
# 878、pandas.Index.factorize方法
import pandas as pd
# 创建一个Index对象
index = pd.Index(['apple', 'banana', 'apple', 'orange', 'banana', 'orange', 'apple'])
# 因子化Index对象
labels, uniques = index.factorize()
print(labels)
print(uniques)
878-6-3、结果输出
python
# 878、pandas.Index.factorize方法
# [0 1 0 2 1 2 0]
# Index(['apple', 'banana', 'orange'], dtype='object')
879、pandas.Index.identical方法
879-1、语法
python
# 879、pandas.Index.identical方法
final pandas.Index.identical(other)
Similar to equals, but checks that object attributes and types are also equal.
Returns:
bool
If two Index objects have equal elements and same type True, otherwise False.
879-2、参数
879-2-1、other**(必须)****:**另一个Index对象,与当前Index对象进行比较。
879-3、功能
检查两个Index对象是否具有相同的值和数据类型。
879-4、返回值
返回一个布尔值,如果两个Index对象的值和数据类型完全相同,则返回True,否则返回False。
879-5、说明
无
879-6、用法
879-6-1、数据准备
python
无
879-6-2、代码示例
python
# 879、pandas.Index.identical方法
import pandas as pd
# 创建两个Index对象
index1 = pd.Index([1, 2, 3])
index2 = pd.Index([1, 2, 3])
# 比较两个Index对象
print(index1.identical(index2))
879-6-3、结果输出
python
# 879、pandas.Index.identical方法
# True
880、pandas.Index.insert方法
880-1、语法
python
# 880、pandas.Index.insert方法
pandas.Index.insert(loc, item)
Make new Index inserting new item at location.
Follows Python numpy.insert semantics for negative values.
Parameters:
loc
int
item
object
Returns:
Index
880-2、参数
880-2-1、loc**(必须)****:**整数,表示插入位置的索引,表示在当前Index中的插入位置。
880-2-2、item**(必须)****:**表示要插入的标签值,它可以是任何类型,但必须与Index中的其他标签兼容。
880-3、功能
在指定位置插入一个新的标签,从而扩展Index对象的长度,对于动态调整索引或在某些数据操作中插入新的标签非常有用。
880-4、返回值
返回一个新的Index对象,其中包含插入的标签,原始的Index对象不会被修改。
880-5、说明
无
880-6、用法
880-6-1、数据准备
python
无
880-6-2、代码示例
python
# 880、pandas.Index.insert方法
import pandas as pd
# 创建一个Index对象
index = pd.Index([1, 2, 3, 4])
# 在位置1插入新的标签
new_index = index.insert(1, 'new_label')
print(new_index)
880-6-3、结果输出
python
# 880、pandas.Index.insert方法
# Index([1, 'new_label', 2, 3, 4], dtype='object')