1.顺序存储结构
cs
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
#define OK 1
typedef struct {
int key; // 关键字域
} ElemType;
typedef struct {
ElemType *R;
int length;
} SSTable;
int InitList_SSTable(SSTable *L) {
L->R = (ElemType *)malloc(MAXSIZE * sizeof(ElemType));
if (!L->R) {
printf("初始化错误\n");
return 0;
}
L->length = 0;
return OK;
}
int Insert_SSTable(SSTable *L) {
int j = 0;
for (int i = 0; i < MAXSIZE; i++) {
L->R[i].key = j;
L->length++;
j++;
}
return 1;
}
int Search_Seq(SSTable ST, int key) {
// 在顺序表ST中顺序查找其关键字等于key的数据元素。若找到,则函数值为
// 该元素在表中的位置,否则为0
for (int i = ST.length - 1; i >= 0; --i) {
if (ST.R[i].key == key) return i + 1; // C语言中数组从0开始,但通常位置从1开始计数,这里返回i+1
}
return 0;
}
void Show_End(int result, int testkey) {
if (result == 0)
printf("未找到%d\n", testkey);
else
printf("找到%d位置为%d\n", testkey, result);
}
int main() {
SSTable ST;
InitList_SSTable(&ST);
Insert_SSTable(&ST);
int testkey1 = 7, testkey2 = 200;
int result;
result = Search_Seq(ST, testkey1);
Show_End(result, testkey1);
result = Search_Seq(ST, testkey2);
Show_End(result, testkey2);
// 释放动态分配的内存
free(ST.R);
return 0;
}
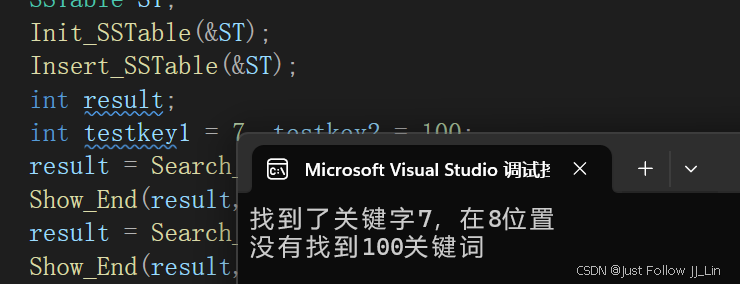
2.链式存储结构
cs
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
typedef struct Node
{
int data;
struct Node* next;
}Node;
Node* Create(int data)
{
Node* newnode = (Node*)malloc(sizeof(Node));
if (newnode == NULL)
{
return;
}
newnode->data = data;
newnode->next = NULL;
return newnode;
}
//尾插
void appendNode(Node** headRef, int data)
{
Node* newnode = Create(data);
if (*headRef == NULL)
{
*headRef = newnode;
return;
}
Node* temp = *headRef;
while (temp->next != NULL)
{
temp = temp->next;
}
temp->next = newnode;
}
//顺序查找
Node* Find_Node(Node* head, int key)
{
Node* current = head;
while (current)
{
if (current->data == key)
{
return current;
}
current = current->next;
}
return NULL;
}
void printNode(Node* head)
{
Node* temp = head;
while (temp)
{
printf("%d ", temp->data);
temp = temp->next;
}
}
void Free_Node(Node* head)
{
Node* temp = head;
while (temp)
{
Node* pcur = temp->next;
free(temp);
temp = pcur;
}
}
int main()
{
Node* temp = NULL;
appendNode(&temp, 10);
appendNode(&temp, 20);
appendNode(&temp, 30);
appendNode(&temp, 40);
printf("链表内容如下:\n");
printNode(temp);
printf("\n");
int key = 30;
Node* result = Find_Node(temp, key);
if (result != NULL)
{
printf("链表中存在key的值为%d", key);
}
else {
printf("链表中不存在key的值为%d", key);
}
Free_Node(temp);
return 0;
}
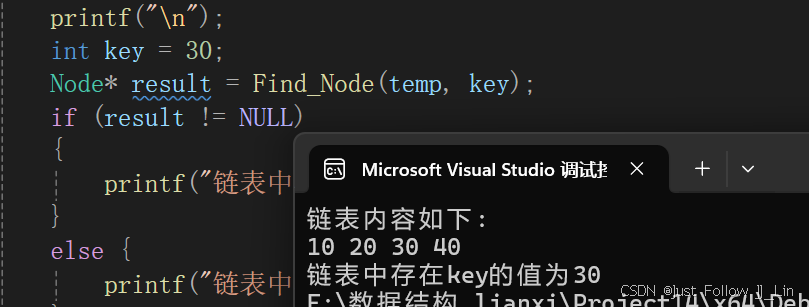