首先想到的就是用结构体来记录蚂蚁的状态,根据绝对位置来排序蚂蚁,遍历所有蚂蚁,如果相向则接着去判断他们是否感冒,如果感冒则都变成感冒状态,然后改变两只蚂蚁的方向
代码如下:
#include <iostream>
#include <vector>
#include <cmath> // 用于abs函数
#include <algorithm>
using namespace std;
struct Ant {
int position; // 蚂蚁的位置
bool hasCold; // 蚂蚁是否感冒
};
bool compare(const Ant &a, const Ant &b) {
return abs(a.position) < abs(b.position);
}
int main() {
int n;
cin >> n;
vector<Ant> ants(n);
// 初始化蚂蚁的位置和感冒状态
for (int i = 0; i < n; i++) {
cin >> ants[i].position;
ants[i].hasCold = (i == 0); // 第一只蚂蚁感冒了
}
sort(ants.begin(), ants.end(), compare);
// 遍历蚂蚁,使用双指针法
for (int i = 0; i < n - 1; ) {
// 检查当前蚂蚁和下一只蚂蚁是否相向而行
if (ants[i].position > 0 && ants[i + 1].position < 0) { // 如果符号相反,则相向而行
// 如果至少有一只蚂蚁感冒,则两只都感冒
if (ants[i].hasCold || ants[i + 1].hasCold) {
ants[i].hasCold = true;
ants[i + 1].hasCold = true;
}
// 改变两只蚂蚁的方向
ants[i].position = -ants[i].position;
ants[i + 1].position = -ants[i + 1].position;
if (i >= 2 ) i -= 2;
}
i++; // 移动到下一只蚂蚁
}
// 计算感冒蚂蚁的数量
int coldCount = 0;
for (int i = 0; i < n; i++) {
if (ants[i].hasCold) {
coldCount++;
}
}
cout << coldCount << endl;
return 0;
}
代码提交状态: Wrong Answer
代码运行状态: 错误数据如下所示 ×
输入:
5
-6 7 1 5 -8
输出
3
标准答案
4
转换思路
可以将蚂蚁碰面后调头视为穿过
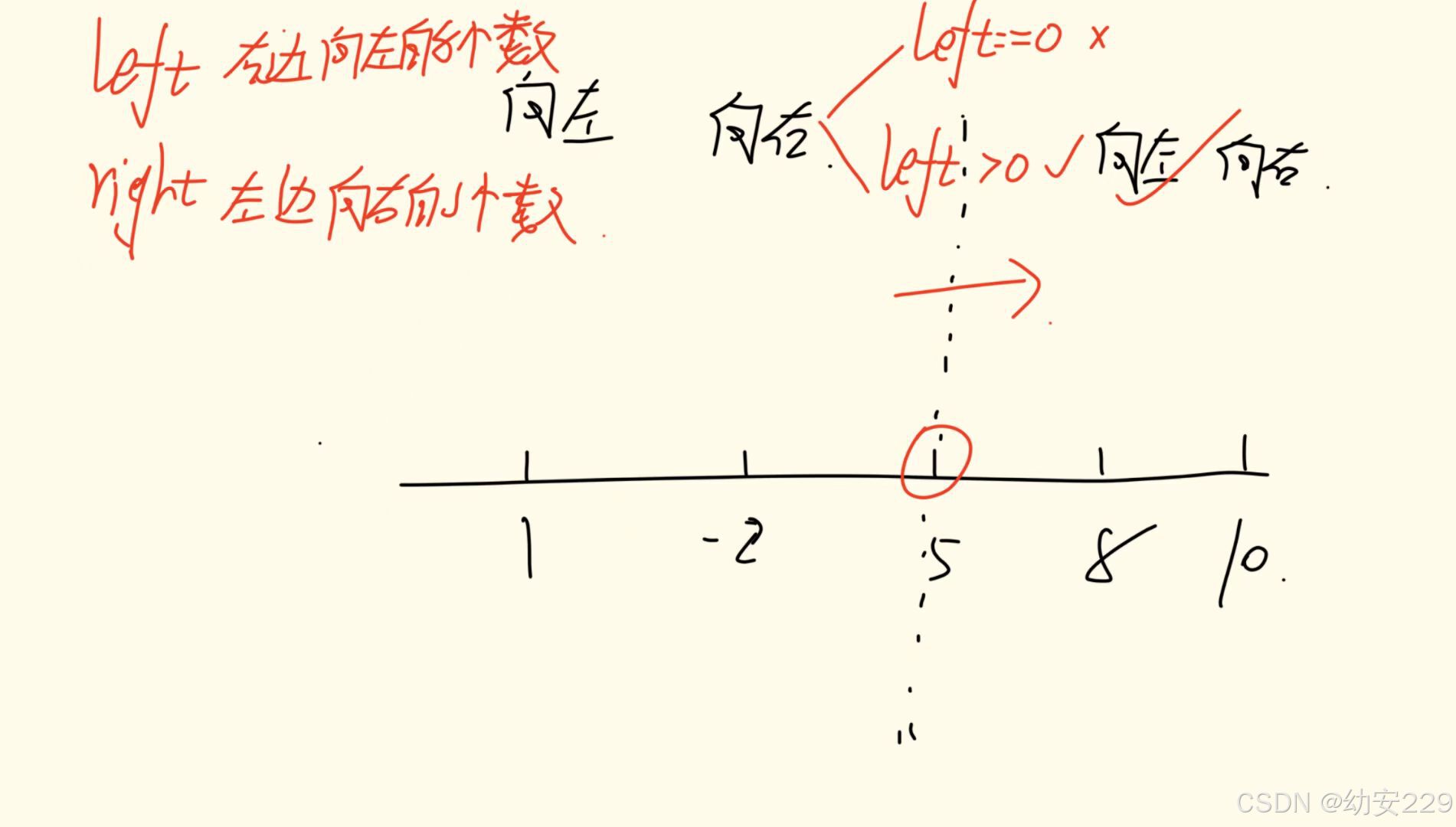
以第一只蚂蚁向右举例,在它右边的蚂蚁如果向左则会被感染,那么记录右边中向左蚂蚁的数量
对于左边的蚂蚁而言,如果有右边中有感染的蚂蚁方向向左,那么向右的蚂蚁就会感染,否则就不用担心
if (第一只蚂蚁向右,并且右边向左蚂蚁的数量为0)则感冒的蚂蚁数量为1
否则 感冒的蚂蚁数量为 left + right + 1
初始蚂蚁向左同理可推,
if (第一只蚂蚁向右,并且左边向右蚂蚁的数量为0)则感冒的蚂蚁数量为1
否则 感冒的蚂蚁数量为 left + right + 1
代码如下:
#include <iostream>
using namespace std;
const int N = 55;
int s[N];
int main() {
int n;
cin >> n;
for (int i = 0;i < n;i++) {
cin >> s[i];
}
int left = 0,right = 0;
for (int i = 1;i < n;i++) {
if (abs(s[i]) < abs(s[0]) && s[i] > 0) right++;
else if (abs(s[i]) > abs(s[0]) && s[i] < 0) left++;
}
if ((s[0] > 0 && left == 0) || (s[0] < 0 && right == 0)) cout << 1 << endl;
else cout << left + right + 1 << endl;
return 0;
}