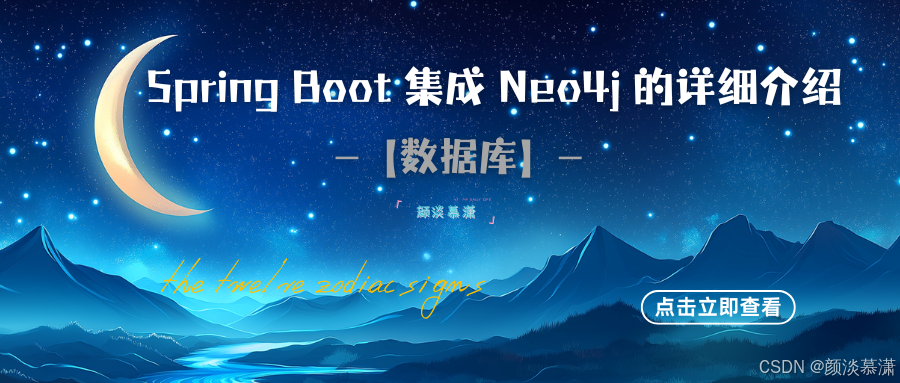
Spring Boot 提供了对 Neo4j 的良好支持,使得开发者可以更方便地使用图数据库。通过使用 Spring Data Neo4j,开发者可以轻松地进行数据访问、操作以及管理。本文将详细介绍如何在 Spring Boot 应用中集成 Neo4j,包括基本配置、实体定义、数据访问层的实现以及使用示例。
一、环境准备
1. 创建 Spring Boot 项目
可以使用 Spring Initializr 创建一个新的 Spring Boot 项目,选择以下依赖:
- Spring Web
- Spring Data Neo4j
2. 添加 Maven 依赖
在 pom.xml
中添加 Neo4j 的相关依赖:
xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-neo4j</artifactId>
</dependency>
<dependency>
<groupId>org.neo4j.driver</groupId>
<artifactId>neo4j-java-driver</artifactId>
<version>4.4.4</version> <!-- 根据最新版本调整 -->
</dependency>
二、配置 Neo4j
在 application.properties
或 application.yml
中配置 Neo4j 的连接信息:
properties
spring.data.neo4j.uri=bolt://localhost:7687
spring.data.neo4j.authentication.username=your_username
spring.data.neo4j.authentication.password=your_password
三、定义实体类
使用 @Node
注解定义 Neo4j 节点模型。以下是一个简单的 Person
实体类示例:
java
import org.springframework.data.annotation.Id;
import org.springframework.data.neo4j.core.schema.Node;
@Node
public class Person {
@Id
private Long id;
private String name;
private int age;
// 构造函数、getter 和 setter
public Person() {}
public Person(Long id, String name, int age) {
this.id = id;
this.name = name;
this.age = age;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
四、创建数据访问层
使用 Spring Data Neo4j 提供的 Neo4jRepository
接口来创建数据访问层。以下是 PersonRepository
的示例:
java
import org.springframework.data.neo4j.repository.Neo4jRepository;
public interface PersonRepository extends Neo4jRepository<Person, Long> {
Person findByName(String name);
}
五、服务层
在服务层中,你可以使用 @Service
注解来管理业务逻辑:
java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class PersonService {
private final PersonRepository personRepository;
@Autowired
public PersonService(PersonRepository personRepository) {
this.personRepository = personRepository;
}
public Person savePerson(Person person) {
return personRepository.save(person);
}
public List<Person> findAllPersons() {
return personRepository.findAll();
}
public Person findByName(String name) {
return personRepository.findByName(name);
}
}
六、控制层
创建控制器来处理 HTTP 请求:
java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api/persons")
public class PersonController {
private final PersonService personService;
@Autowired
public PersonController(PersonService personService) {
this.personService = personService;
}
@PostMapping
public Person createPerson(@RequestBody Person person) {
return personService.savePerson(person);
}
@GetMapping
public List<Person> getAllPersons() {
return personService.findAllPersons();
}
@GetMapping("/{name}")
public Person getPersonByName(@PathVariable String name) {
return personService.findByName(name);
}
}
七、运行应用
确保 Neo4j 数据库正在运行,然后启动你的 Spring Boot 应用。你可以使用 Postman 或其他 HTTP 客户端发送请求来测试 API。
示例请求
-
创建节点:
httpPOST /api/persons Content-Type: application/json { "id": 1, "name": "Alice", "age": 30 }
-
查询所有节点:
httpGET /api/persons
-
根据名称查询节点:
httpGET /api/persons/Alice
八、总结
通过上述步骤,你可以轻松地在 Spring Boot 应用中集成 Neo4j。使用 Spring Data Neo4j 不仅简化了数据访问层的实现,还提供了强大的查询能力和事务管理。希望这篇文章能帮助你快速上手并利用 Neo4j 的优势来构建你的应用程序。