一、数据库表
sql
CREATE TABLE students (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100) NOT NULL,
age INT,
gender ENUM('Male', 'Female', 'Other'),
email VARCHAR(100) UNIQUE,
phone_number VARCHAR(20),
address VARCHAR(255),
date_of_birth DATE,
enrollment_date DATE,
course VARCHAR(100)
);
-- 插入10条学生数据
INSERT INTO students (name, age, gender, email, phone_number, address, date_of_birth, enrollment_date, course) VALUES
('John Doe', 20, 'Male', '[email protected]', '1234567890', '123 Main St, City', '2003-01-01', '2023-09-01', 'Computer Science'),
('Jane Smith', 21, 'Female', '[email protected]', '0987654321', '456 Elm Rd, Town', '2002-02-02', '2023-09-01', 'Business Administration'),
('Michael Johnson', 19, 'Male', '[email protected]', '1122334455', '789 Oak Ave, Village', '2004-03-03', '2023-09-01', 'Electrical Engineering'),
('Emily Davis', 22, 'Female', '[email protected]', '5544332211', '321 Pine Blvd, County', '2001-04-04', '2023-09-01', 'Mechanical Engineering'),
('William Brown', 20, 'Male', '[email protected]', '9988776655', '654 Cedar Ln, District', '2003-05-05', '2023-09-01', 'Civil Engineering'),
('Olivia Wilson', 21, 'Female', '[email protected]', '4433221100', '987 Walnut St, Borough', '2002-06-06', '2023-09-01', 'Chemistry'),
('Benjamin Taylor', 19, 'Male', '[email protected]', '7766554433', '246 Maple Dr, Neighborhood', '2004-07-07', '2023-09-01', 'Physics'),
('Grace Anderson', 22, 'Female', '[email protected]', '3322110099', '852 Birch Rd, Hamlet', '2001-08-08', '2023-09-01', 'Mathematics'),
('Henry Thompson', 20, 'Male', '[email protected]', '6655443322', '587 Aspen St, Province', '2003-09-09', '2023-09-01', 'Biology'),
('Chloe Robinson', 21, 'Female', '[email protected]', '9977665544', '101 Poplar Ave, Region', '2002-10-10', '2023-09-01', 'Psychology');
二、在pom文件中添加MyBatis相关依赖包,Mysql驱动依赖
XML
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- mybatis依赖 -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>3.0.3</version>
</dependency>
<!-- mysql依赖 -->
<dependency>
<groupId>com.mysql</groupId>
<artifactId>mysql-connector-j</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- mybatis测试依赖 -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter-test</artifactId>
<version>3.0.3</version>
<scope>test</scope>
</dependency>
当我们没有热部署的时候,我们必须在代码修改完后再重启程序,程序才会同步你修改的信息。那么我们可以手动启动热部署-》》》
XML
<!-- 热部署工具 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<optional>true</optional>
</dependency>
三、编写实体类
java
public class Student {
// 定义属性
private String name; // 姓名
private int age; // 年龄
private String gender; // 性别
private String email; // 邮箱
private String phoneNumber; // 电话号码
private String address; // 地址
private java.util.Date dateOfBirth; // 出生日期
private java.util.Date enrollmentDate; // 入学日期
private String course; // 课程
...setXXX and getXXX and constructor and toString...
四、 编写映射类StudentMapper
java
*/
@Mapper
public interface StudentMapper {
// @Select("select * from student where id = #{id}")
public List<Student> findById(int id);
// @Select("select * from student")
public List<Student> findAll();
}
注意:如果不写@Select注解,则就需要写Mapper映射文件
五、编写Mapper映射文件
XML
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.hlx.springbootdemo1.mapper.StudentMapper">
<select id="findById" parameterType="int" resultType="Student">
SELECT * FROM student WHERE id = #{id}
</select>
<select id="findAll" resultType="Student">
select * from student
</select>
</mapper>
六、编写配置文件
XML
#mysql数据源配置
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/school?useSSL=false&serverTimezone=UTC&allowPublicKeyRetrieval=true
spring.datasource.username=root
spring.datasource.password=123456
#mybatis
mybatis.mapper-locations=classpath*:mapper/*.xml
#实体类的别名
mybatis.type-aliases-package=com.hlx.springbootdemo1.entity
#日志
logging.pattern.console='%d{HH:mm:ss.SSS} %clr(%-5level) --- [%-15thread] %cyan(%-50logger{50}):%msg%n'
七、编写业务类
java
@Service
public class StudentService {
@Autowired
private StudentMapper studentMapper;
public List<Student> findAll(){
return (studentMapper.findAll());
}
public Student findById(int id){
return (studentMapper.findById(id));
}
}
八、编写控制器类
java
@RestController
public class StudentsController {
@Autowired
private StudentService studentService;
@GetMapping("/user/{id}")
public Student findById(@PathVariable int id) {
return studentService.findById(id);
}
@GetMapping("/user/all")
public List<Student> findAll() {
return studentService.findAll();
}
}
九、启动类
java
@SpringBootApplication
@MapperScan("com.hlx.springbootdemo1.mapper")
public class SpringbootDemo1Application {
public static void main(String[] args) {
SpringApplication.run(SpringbootDemo1Application.class, args);
}
}
十、项目结构
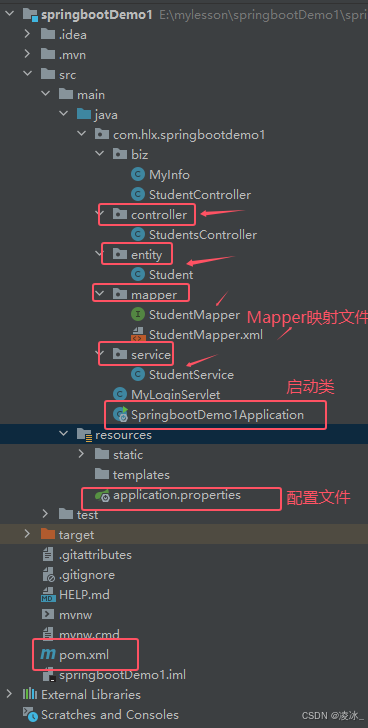