1. 实现效果
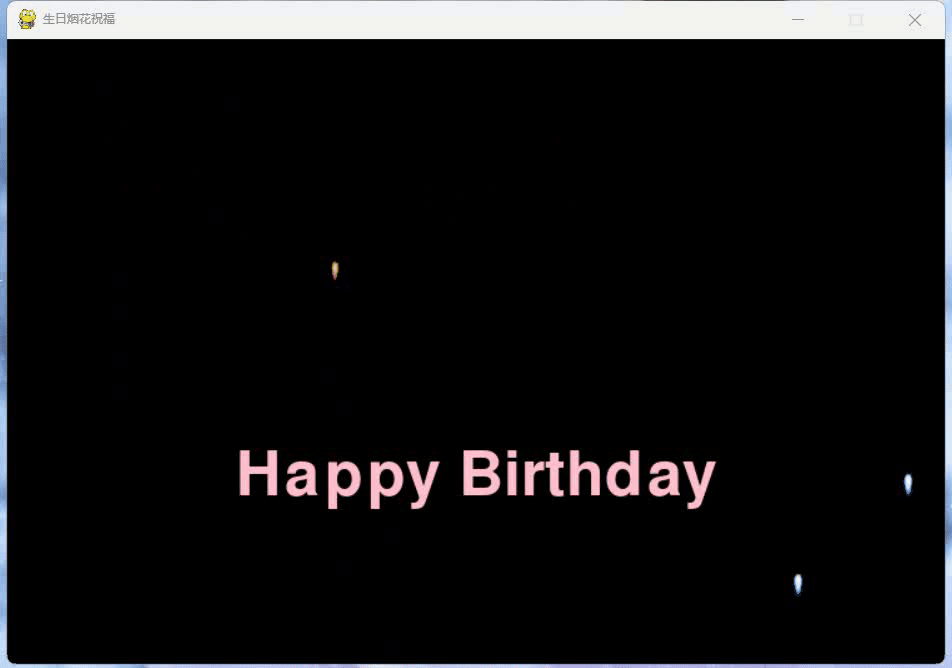
2. 素材加载
2个图片和3个音频

python
shoot_image = pygame.image.load('shoot(已去底).jpg') # 加载拼接的发射图像
flower_image = pygame.image.load('flower.jpg') # 加载拼接的烟花图 烟花不好去底
# 调整图像的像素为原图的1/2 因为图像相对于界面来说有些大
shoot_image = pygame.transform.scale(shoot_image, (shoot_image.get_size()[0]/2 ,shoot_image.get_size()[1]/2))
flower_image = pygame.transform.scale(flower_image, (flower_image.get_size()[0]/2 ,flower_image.get_size()[1]/2))
# 音频、音效
shoot_sound = pygame.mixer.Sound('shoot.mp3')
bomb_sound = pygame.mixer.Sound('bomb1.mp3')
bg_music = pygame.mixer.Sound('bg.mp3')
图像分块显示
python
# 每个部分的宽度
num_parts = 10 # 有10个shoot图
num_parts1 = 13 # 13个烟花
shoot_part_width = shoot_width // num_parts
flower_part_width = flower_width // num_parts1
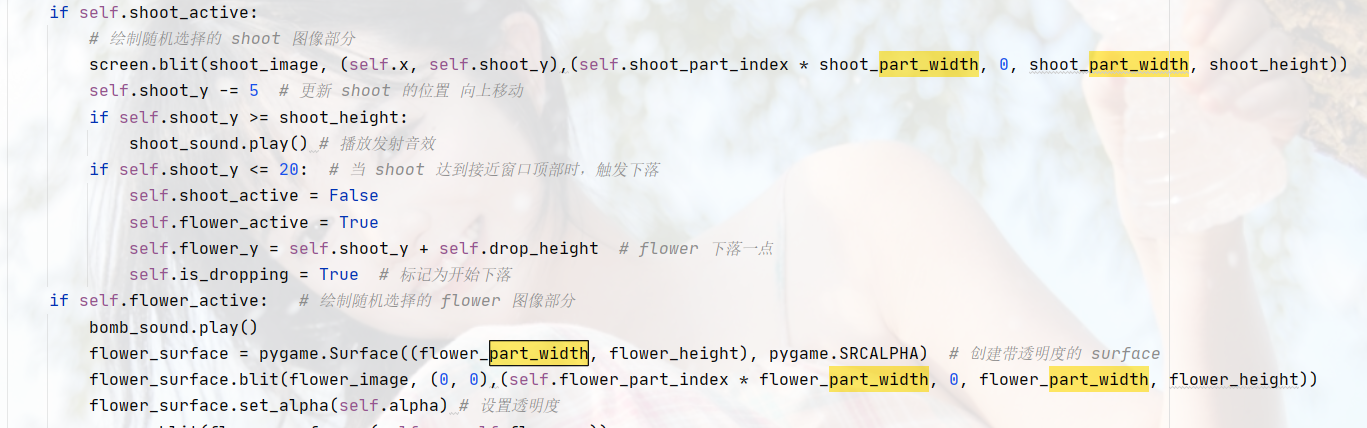
3. 烟花发射
发射shoot图从底部往上,快到顶部变烟花flower,然后下落一段距离,最后消失。
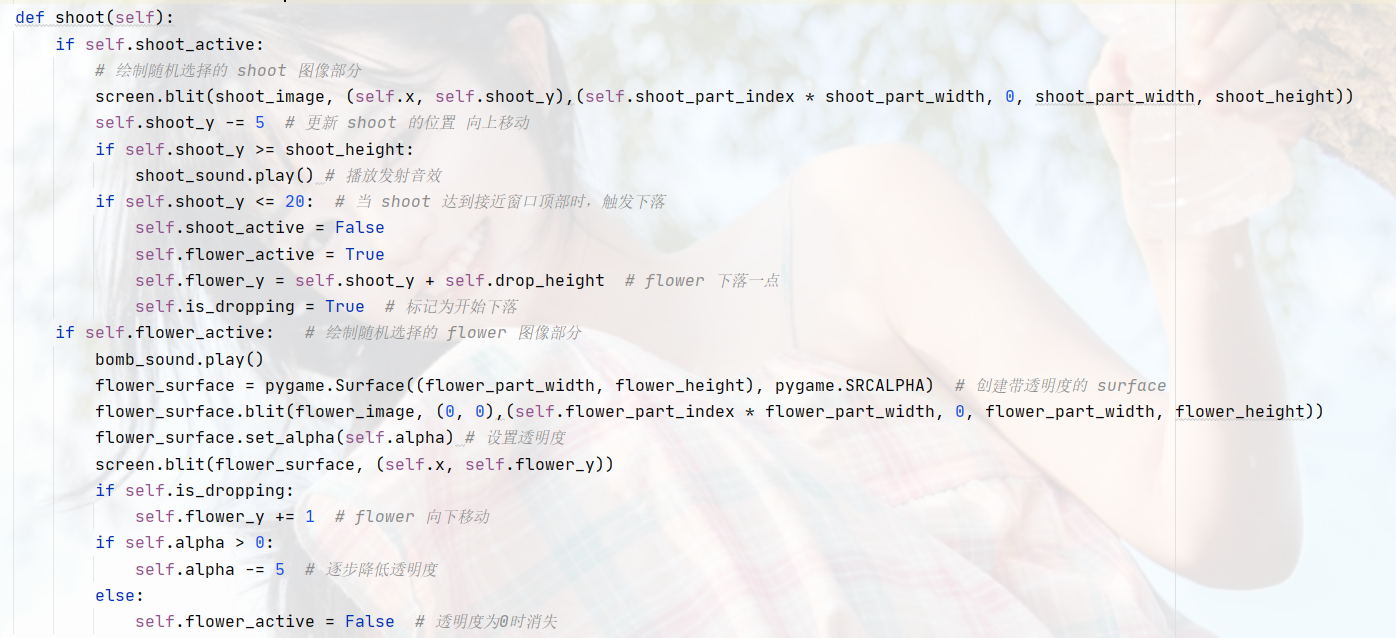
4. 画面定格
处理鼠标点击事件以暂停和恢复动画
5. 完整代码
python
# 2024-12-01 Python简单的生日祝福烟花
import pygame # pip install pygame
import random
pygame.init() # 初始化 pygame
WIDTH, HEIGHT = 750, 500 # 设置窗口尺寸
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("生日烟花祝福") # 窗口标题
#bg_image = pygame.image.load('bg.jpg')
shoot_image = pygame.image.load('shoot(已去底).jpg') # 加载拼接的发射图像
flower_image = pygame.image.load('flower.jpg') # 加载拼接的烟花图 烟花不好去底
# 调整图像的像素为原图的1/2 因为图像相对于界面来说有些大
shoot_image = pygame.transform.scale(shoot_image, (shoot_image.get_size()[0]/2 ,shoot_image.get_size()[1]/2))
flower_image = pygame.transform.scale(flower_image, (flower_image.get_size()[0]/2 ,flower_image.get_size()[1]/2))
# 音频、音效
shoot_sound = pygame.mixer.Sound('shoot.mp3')
bomb_sound = pygame.mixer.Sound('bomb1.mp3')
bg_music = pygame.mixer.Sound('bg.mp3')
bg_music.play(-1) # 播放背景音乐
# 获取图像的宽度和高度
shoot_width, shoot_height = shoot_image.get_size()
flower_width, flower_height = flower_image.get_size()
# 每个部分的宽度
num_parts = 10 # 有10个shoot图
num_parts1 = 13 # 13个烟花
shoot_part_width = shoot_width // num_parts
flower_part_width = flower_width // num_parts1
class Firework: # 定义烟花类
def __init__(self, x):
self.x = x # 使用提供的x坐标
self.shoot_y = HEIGHT # shoot初始y坐标在底部
self.flower_y = 0 # flower初始位置上方
self.shoot_active = True
self.flower_active = False
self.shoot_part_index = random.randint(0, num_parts - 1) # 随机选择shoot的部分索引
self.flower_part_index = random.randint(0, num_parts - 1) # 随机选择flower的部分索引
self.drop_height = 10 # 下落的高度(在达到顶部后下落一点)
self.is_dropping = False # 是否开始下落
self.alpha = 255 # 透明度控制
def shoot(self):
if self.shoot_active:
# 绘制随机选择的 shoot 图像部分
screen.blit(shoot_image, (self.x, self.shoot_y),(self.shoot_part_index * shoot_part_width, 0, shoot_part_width, shoot_height))
self.shoot_y -= 5 # 更新 shoot 的位置 向上移动
if self.shoot_y >= shoot_height:
shoot_sound.play() # 播放发射音效
if self.shoot_y <= 20: # 当 shoot 达到接近窗口顶部时,触发下落
self.shoot_active = False
self.flower_active = True
self.flower_y = self.shoot_y + self.drop_height # flower 下落一点
self.is_dropping = True # 标记为开始下落
if self.flower_active: # 绘制随机选择的 flower 图像部分
bomb_sound.play()
flower_surface = pygame.Surface((flower_part_width, flower_height), pygame.SRCALPHA) # 创建带透明度的 surface
flower_surface.blit(flower_image, (0, 0),(self.flower_part_index * flower_part_width, 0, flower_part_width, flower_height))
flower_surface.set_alpha(self.alpha) # 设置透明度
screen.blit(flower_surface, (self.x, self.flower_y))
if self.is_dropping:
self.flower_y += 1 # flower 向下移动
if self.alpha > 0:
self.alpha -= 5 # 逐步降低透明度
else:
self.flower_active = False # 透明度为0时消失
clock = pygame.time.Clock()
fireworks = []
running = True
paused = False # 初始化暂停状态为 False
font = pygame.font.Font(None, 74) # 设置字体, 使用默认字体, 大小为74
text_color = (255, 192, 203) # 文字颜色粉红色
birthday_text = font.render("Happy Birthday", True, text_color) # 文本"生日快乐"
text_rect = birthday_text.get_rect(center=(WIDTH // 2, HEIGHT - 150)) # 文本位置
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 处理鼠标点击事件以暂停和恢复动画
if event.type == pygame.MOUSEBUTTONDOWN:
paused = not paused # 切换暂停状态
if not paused:
# 每隔一段时间生成新烟花
if random.random() < 0.01: # 随机生成烟花 and len(fireworks) < 10
# 随机选择 X 坐标,避免与已有烟花重叠
new_firework_x = random.randint(0, WIDTH - shoot_part_width)
overlap = any(abs(new_firework_x - firework.x) < shoot_part_width for firework in fireworks)
if not overlap: # 如果没有重叠,添加新烟花
fireworks.append(Firework(new_firework_x))
screen.fill((0, 0, 0)) # 填充黑色背景 #screen.blit(bg_image, (0, 0))
for firework in fireworks:
firework.shoot()
screen.blit(birthday_text, text_rect) # 绘制生日文本
pygame.display.flip()
clock.tick(60) # 控制更新画面的速度
pygame.quit()