1. 节点信息查看
bash
#查看集群健康情况
curl -X GET 'localhost:9200/_cat/health?v&pretty'
#查看节点信息
curl -X GET 'localhost:9200/_cat/nodes?v&pretty'

2. 索引管理
在es中,索引就相当于是mysql中的库了。
bash
#查看索引列表
curl -X GET 'localhost:9200/_cat/indices?v&pretty'
# 创建index
curl -X PUT 'localhost:9200/customer?pretty'
curl -X PUT 'localhost:9200/users?pretty'
#删除索引
curl -X PUT 'localhost:9200/users?pretty'
curl -X GET 'localhost:9200/_cat/indices?v&pretty'
#删除索引
curl -X DELETE 'localhost:9200/users?pretty'
可以看到我们创建了users 这个索引,然后删除这个users 这个索引对象,
3. 文档管理
3.1 文档添加
文档添加的愈发是/index/type/id 其中,index是索引名称,type就相当于是mysql中的哪张表了。
bash
# 添加文档
#当customer这个文档不存在得情况,就会自动创建这个索引
#-d 数据类型
#-H 添加Head 头
#-XPUT put提交方式
#external/1
curl -X PUT 'localhost:9200/customer/external/1?pretty&pretty' -d '{"name": "John Doe"}' -H "Content-Type:application/json"
curl -X PUT 'localhost:9200/customer/external/2?pretty&pretty' -d '{"name": "yellowcong"}' -H "Content-Type:application/json"
#查询
#查询id为1得
curl -X GET 'localhost:9200/customer/external/1?pretty&pretty'
可以看到文档的类型是"_type" : "external"
3.2 文档更新
这个地方external ,有点像mysql中每条记录得id信息
bash
# 插入文档
curl -X PUT 'localhost:9200/customer/external/1?pretty&pretty' -d '{"name": "yellowcong2","age":12}' -H "Content-Type:application/json"
#获取列表
curl -X GET 'localhost:9200/customer/external/3?pretty&pretty'
#直接通过post /put 覆盖更新文档
curl -X PUT 'localhost:9200/customer/external/1/?pretty&pretty' -d '{"name": "yellowcong2","age":12}' -H "Content-Type:application/json"
#通过update 接口进行更新
curl -XPOST 'localhost:9200/customer/external/1/_update?pretty&pretty' -d'
{
"doc": { "name": "张三","age":12 }
}' -H "Content-Type:application/json"
#更新某个字段,比如age 这种 ,ctx._source 表示原文档
curl -XPOST 'localhost:9200/customer/external/1/_update?pretty&pretty' -d'
{
"script" : "ctx._source.age += 5"
}' -H "Content-Type:application/json"
#更新名称
curl -XPOST 'localhost:9200/customer/external/1/_update?pretty&pretty' -d'
{
"script" : "ctx._source.name = \"张2\""
}' -H "Content-Type:application/json"
3.3 文档删除
bash
#删除文档
curl -X DELETE 'localhost:9200/customer/external/1?pretty'
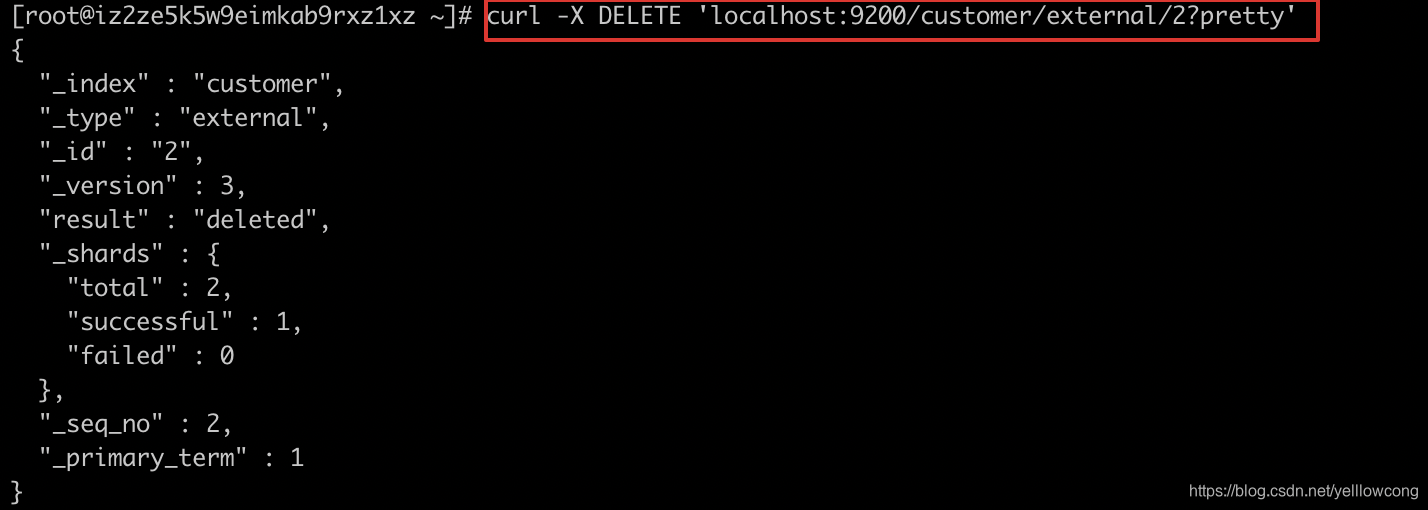
3.4 文档批处理
bash
#删除文档customer
curl -X DELETE 'localhost:9200/customer?pretty'
#批量插入数据,两条
curl -XPOST 'localhost:9200/customer/external/_bulk?pretty' -d'
{"index":{"_id":"5"}}
{"name": "John Doe" }
{"index":{"_id":"6"}}
{"name": "Jane Doe" }
' -H "Content-Type:application/json"
#查看列表
curl 'localhost:9200/customer/_search?pretty'
#批量更新和删除
curl -XPOST 'localhost:9200/customer/external/_bulk?pretty' -d'
{"update":{"_id":"5"}}
{"doc": { "name": "John Doe becomes Jane Doe" } }
{"delete":{"_id":"6"}}
' -H "Content-Type:application/json"
#查看索引
curl 'localhost:9200/customer/external/5
批量插入两条数据
查看插入的数据
4 搜索
4.1 查询所有数据
bash
#查看customer得所有数据
curl -XGET 'localhost:9200/customer/_search?pretty'
#这个q就相当于是查询条件
curl -XGET 'localhost:9200/customer/_search?q=*&pretty'
#第三种查询所有得写法
curl -X GET 'localhost:9200/customer/_search?pretty' -d'
{
"query": { "match_all": {} }
}'
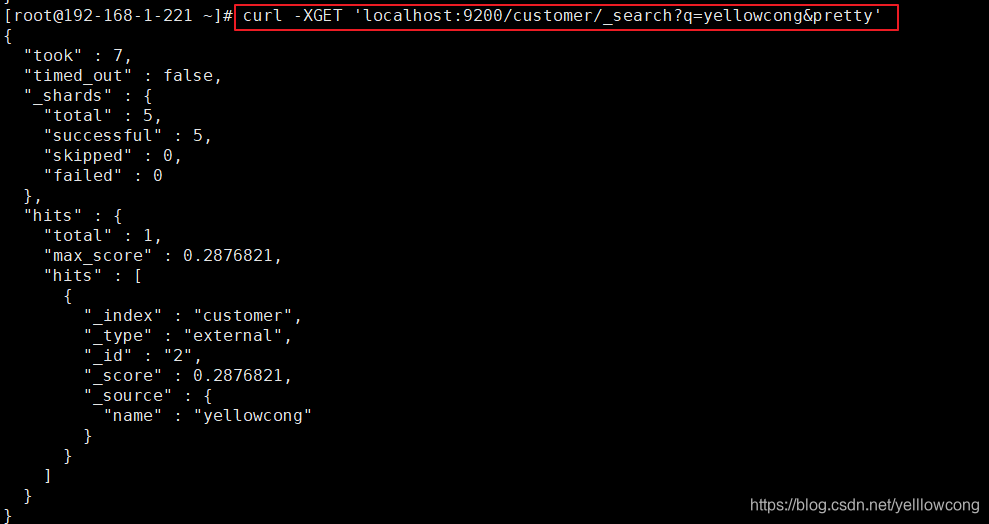
4.2 分页查询
bash
#删除之前的文档
curl -X DELETE 'localhost:9200/customer?pretty'
#批量插入数据,插入六条数据
curl -XPOST 'localhost:9200/customer/external/_bulk?pretty' -d'
{"index":{"_id":"1"}}
{"name": "yellowcong","age":12 }
{"index":{"_id":"2"}}
{"name": "李二" ,"age":65}
{"index":{"_id":"3"}}
{"name": "张三" ,"age":22}
{"index":{"_id":"4"}}
{"name": "赵四","age":18 }
{"index":{"_id":"5"}}
{"name": "王五","age":30 }
{"index":{"_id":"6"}}
{"name": "赵六","age":20 }
' -H "Content-Type:application/json"
#查看所有
curl -X GET 'localhost:9200/customer/_search?pretty'
#分页查询,排序方式通过_id ,页面大小为2
#query 查询条件
#sort 排序方式
#from 开始页面
#size 页面大小
curl -XGET 'localhost:9200/customer/_search?pretty' -d'
{
"query": { "match_all": {} },
"sort": { "_id": { "order": "asc" } },
"from": 0,
"size": 2
}' -H "Content-Type:application/json"
#返回指定的字段,通过_source 指定只需要返回的字段
curl -XGET 'localhost:9200/customer/_search?pretty' -d'
{
"query": { "match_all": {} },
"_source": [ "name"]
}' -H "Content-Type:application/json"
4.3 按条件查询
bash
#与查询
curl -XGET 'localhost:9200/customer/_search?pretty' -d'
{
"query": { "match_all": {"name":"yellowcong"} },
"sort": { "_id": { "order": "asc" } }
}' -H "Content-Type:application/json"
#或查询must,should,和 must_not
参考文章
https://www.cnblogs.com/fhen/p/7055798.html
http://cwiki.apachecn.org/pages/viewpage.action?pageId=4260713
http://cwiki.apachecn.org/pages/viewpage.action?pageId=4260761