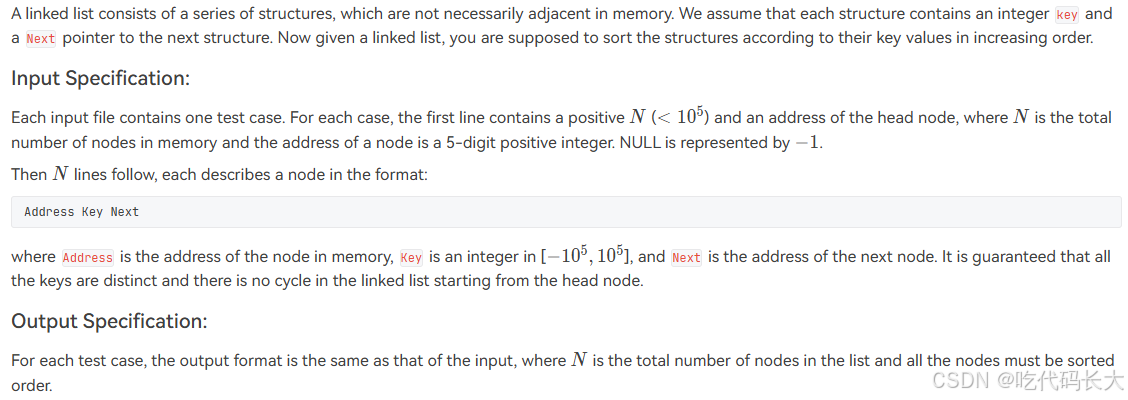
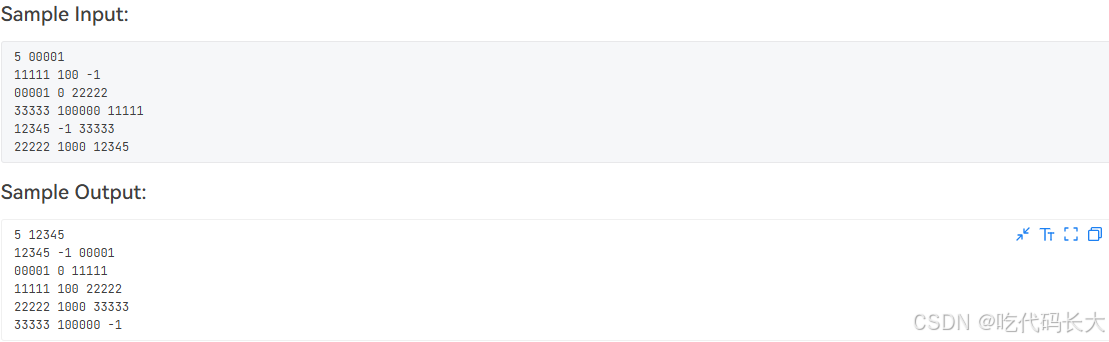
cpp
#include <cstdio>
#include <algorithm>
using namespace std;
const int maxn = 100005;
struct Node{
int address,data,next,flag;
}nodes[maxn];
bool cmp(Node a, Node b){
if(a.flag != b.flag) return a.flag > b.flag;
else return a.data < b.data;
}
int main() {
int n,head;
scanf("%d%d", &n, &head);
int tmp;
for(int i = 0; i < n; i++){
scanf("%d", &tmp);
scanf("%d%d", &nodes[tmp].data, &nodes[tmp].next);
nodes[tmp].address = tmp;
nodes[tmp].flag = 0;
}
int valid = 0;
int cur;
for(cur = head; cur != -1; cur=nodes[cur].next){
valid++;
nodes[cur].flag = 1;
}
if(valid == 0){
printf("0 -1\n");
return 0;
}
sort(nodes, nodes+maxn, cmp);
printf("%d %05d\n", valid, nodes[0].address);
for(int i = 0; i < valid; i++){
if(i != valid - 1){
printf("%05d %d %05d\n", nodes[i].address, nodes[i].data, nodes[i+1].address);
}else{
printf("%05d %d -1\n", nodes[i].address, nodes[i].data);
}
}
return 0;
}