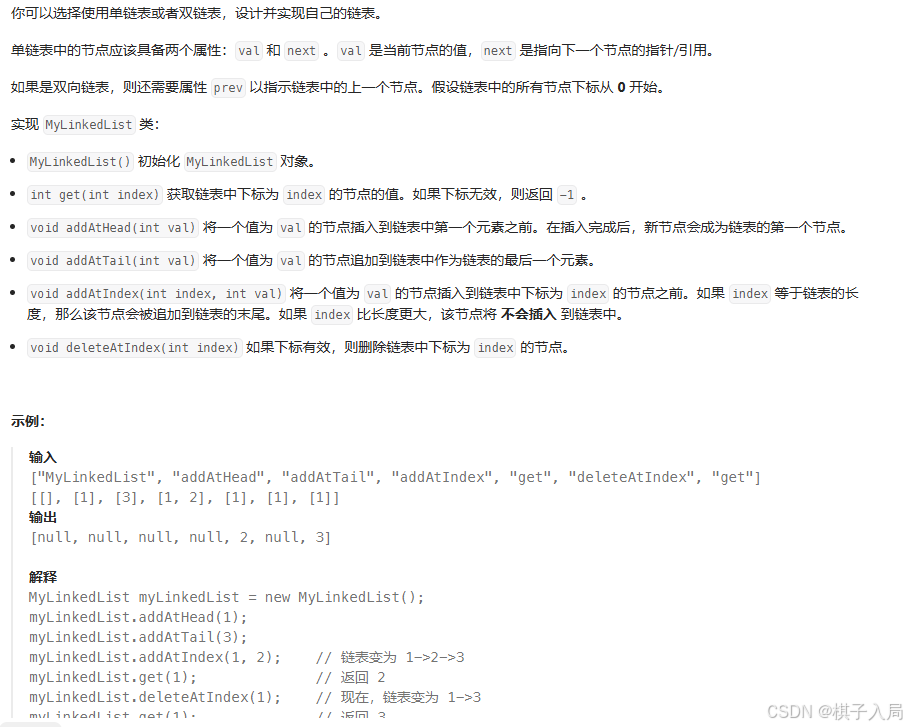
思路:
(1)获取第n个结点的值
明确链表结点下标从0开始,第n个结点
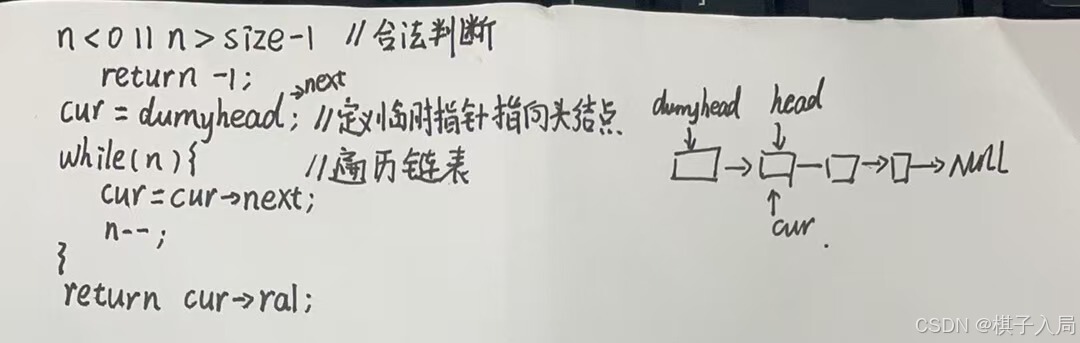
(2)头部插入结点
注意插入的顺序
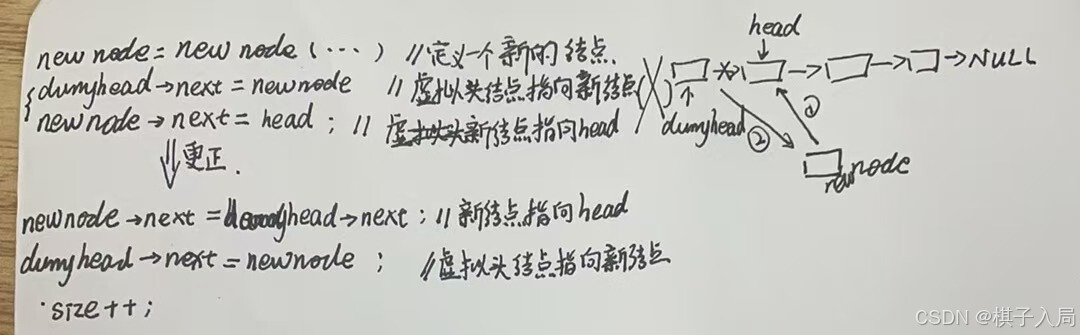
(3)尾部插入结点
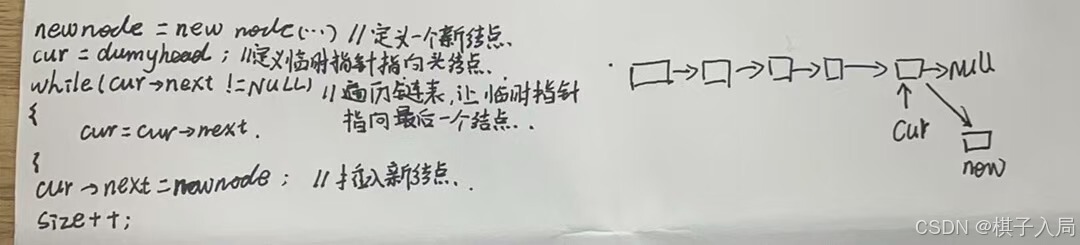
(4)第n个结点前插入结点
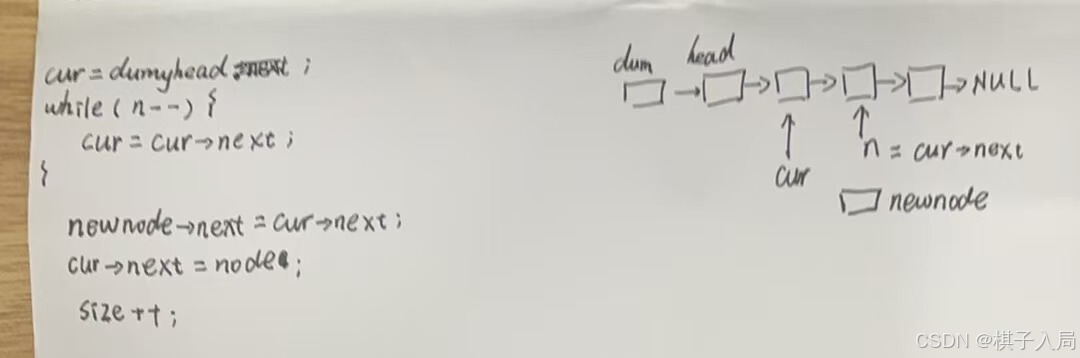
(5)删除第n个结点
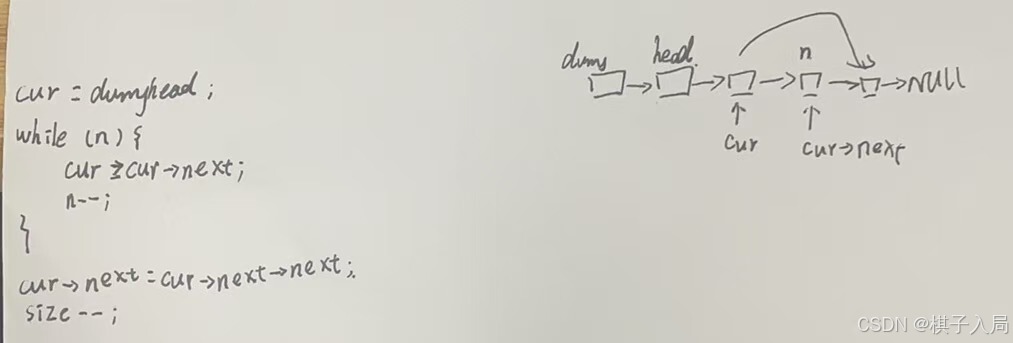
cpp
class MyLinkedList {
struct NodeList {
int val;
NodeList* next;
NodeList(int val):val(val), next(nullptr){}
};
private:
int size;
NodeList* dummyhead;
public:
MyLinkedList() {
dummyhead = new NodeList(0);
size = 0;
}
int get(int index) {
if (index < 0 || index > (size - 1)) {
return -1;
}
NodeList* current = dummyhead->next;
while(index--){
current = current->next;
}
return current->val;
}
void addAtHead(int val) {
NodeList* Newnode = new NodeList(val);
Newnode->next = dummyhead->next;
dummyhead->next = Newnode;
size++;
}
void addAtTail(int val) {
NodeList* Newnode = new NodeList(val);
NodeList* current = dummyhead;
while(current->next != NULL){
current = current->next;
}
current->next = Newnode;
size++;
}
void addAtIndex(int index, int val) {
if (index > size) {
return ;
}
NodeList* Newnode = new NodeList(val);
NodeList* current = dummyhead;
while(index--) {
current = current->next;
}
Newnode->next = current->next;
current->next = Newnode;
size++;
}
void deleteAtIndex(int index) {
if (index >= size || index < 0) {
return ;
}
NodeList* current = dummyhead;
while(index--) {
current = current ->next;
}
NodeList* tmp = current->next;
current->next = current->next->next;
delete tmp;
size--;
}
};