这个例程展示了如何使用 C# 代码和 XAML 绘制覆盖有网格的 3D 表面。
示例使用 WPF 和 C# 将纹理应用于三角形展示了如何将纹理应用于三角形。
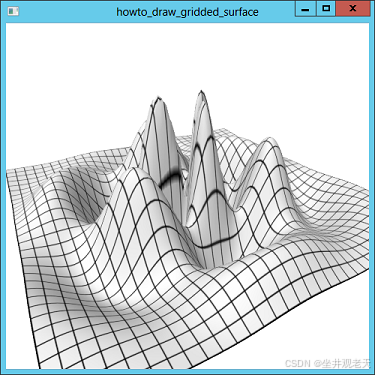
此示例只是使用该技术将包含大网格的位图应用于表面。
在类级别,程序使用以下代码来定义将点的 X 和 Z 坐标映射到 0.0 - 1.0 范围的比例因子
cs
private const double texture_xscale = (xmax - xmin);
private const double texture_zscale = (zmax - zmin);
下面的代码显示了程序如何向 3D 模型添加新点。
cs
// A dictionary to hold points for fast lookup.
private Dictionary<Point3D, int> PointDictionary =
new Dictionary<Point3D, int>();
// If the point already exists, return its index.
// Otherwise create the point and return its new index.
private int AddPoint(Point3DCollection points,
PointCollection texture_coords, Point3D point)
{
// If the point is in the point dictionary,
// return its saved index.
if (PointDictionary.ContainsKey(point))
return PointDictionary[point];
// We didn't find the point. Create it.
points.Add(point);
PointDictionary.Add(point, points.Count - 1);
// Set the point's texture coordinates.
texture_coords.Add(
new Point(
(point.X - xmin) * texture_xscale,
(point.Z - zmin) * texture_zscale));
// Return the new point's index.
return points.Count - 1;
}
与前面的示例一样,代码定义了一个字典来保存Point,以便可以快速查找它们。AddPoint方法查找一个点,如果该点尚不存在,则添加它。然后,它使用该点的 X 和 Z 坐标将该点映射到对象纹理使用的 UV 坐标的 0.0 - 1.0 范围。换句话说,具有最小 X/Z 坐标的点被映射到 (0, 0) 附近的 U/V 坐标,具有最大 X/Z 坐标的点被映射到 (1, 1) 附近的 U/V 坐标。
创建三角形后,程序使用以下代码来创建其材质。
cs
// Make the surface's material using an image brush.
ImageBrush grid_brush = new ImageBrush();
grid_brush.ImageSource =
new BitmapImage(new Uri("Grid.png", UriKind.Relative));
DiffuseMaterial grid_material = new DiffuseMaterial(grid_brush);
文件 Grid.png 仅包含一个 513×513 像素的网格。或者,您也可以在代码中创建网格。第三种方法是使用偏导数来确定应该在哪里绘制线条,然后使用细长的矩形或框将它们绘制在表面上。(后面的帖子将解释如何绘制细长的框。)然而,这会需要做更多的工作。
省略了大量细节,可以在其他例程里看到。
它们相互依存,所以您已经在之前的帖子中看到了关键点。
细节也相当长,所以为了节省空间,我不会在每篇文章中都包含它们。