目录
一、文本文件---写文件
通过文件操作可以将数据持久化
对文件操作需要包含头文件**<fstream>**
文件类型分两类:
文本文件:文件以文本的ASCII码形式存储在计算机中
二进制文件:文件以文本的二进制形式存储在计算机中,一般不能直接读懂
操作文件的三大类:ofstream:写操作
ifstream:读操作
fstream:读写操作
|-------------|---------------|
| ios::in | 为读文件而打开文件 |
| ios::out | 为写文件而打开文件 |
| ios::ate | 初始位置:文件尾 |
| ios::app | 追加方式写文件 |
| ios::trunc | 如果文件存在先删除,再创建 |
| ios::binary | 二进制方式 |
[文件的打开方式]
文件打开方式可以配合使用,利用 | 操作符
例如:用二进制方式写文件:ios::binary | ios::out
代码示例
cpp
#include<iostream>
using namespace std;
#include<fstream> //包含头文件
void test(){
ofstream ofs; //创建流对象
ofs.open("text.txt",ios::out); // 指定打开方式
ofs<<"啦啦啦啦德玛西亚"<<endl; // 写内容
ofs.close(); // 关闭文件
}
int main(){
test();
return 0;
}
展示效果
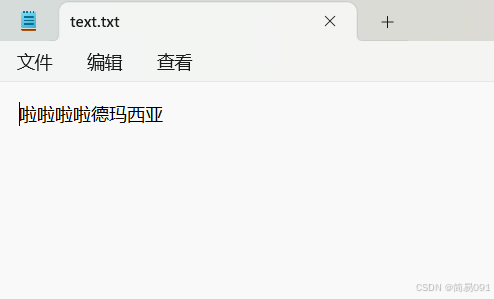
二、文本文件---读文件
代码示例
cpp
#include<iostream>
using namespace std;
#include<fstream> //包含头文件
void test(){
ifstream ifs; //创建流对象
ifs.open("text.txt",ios::in);
if(!ifs.is_open()){
cout<<"文件打开失败"<<endl;
return ;
}
//读文件
//方式一
// char r;
// while((r = ifs.get()) != EOF){
// cout<<r;
// }
//方式二
// string buf;
// while(getline(ifs,buf)){
// cout<<buf<<endl;
// }
//方式三
// char buf[1024] = { 0 };
// while(ifs>>buf){
// cout<<buf<<endl;
// }
//方式四
char buf[1024] = { 0 };
while(ifs.getline(buf,sizeof(buf))){
cout<<buf<<endl;
}
ifs.close(); // 关闭文件
}
int main(){
test();
return 0;
}
三、二进制文件---写文件
代码示例
cpp
#include<iostream>
using namespace std;
#include<fstream>
class person{
public:
char m_Name[64];
int m_age;
};
void test(){
ofstream ofs;
ofs.open("person.txt",ios::out | ios::binary);
person p = {"张三",18};
ofs.write((const char*)&p,sizeof(p));
ofs.close();
}
int main(){
test();
return 0;
}
四、二进制文件---读文件
代码示例
cpp
#include<iostream>
using namespace std;
#include<fstream>
class person{
public:
char m_Name[64];
int m_age;
};
void test(){
ifstream ifs;
ifs.open("person.txt",ios::in | ios::binary);
if(!ifs.is_open()){
cout<<"文件打开失败"<<endl;
return;
}
person p;
ifs.read((char*)&p,sizeof(person));
cout<<"姓名:"<<p.m_Name<<" 年龄:"<<p.m_age<<endl;
ifs.close();
}
int main(){
test();
return 0;
}