矩形的周长面积
- 定义一个矩形类Rec,包含私有属性length、width,包含公有成员方法:
- void set_length(int l); //设置长度
- void set_width(int w); //设置宽度
- int get_length(); //获取长度,将长度的值返回给调用处
- int get_width(); //获取宽度,将宽度的值返回给调用处
- void show(); //输出周长和面积
代码
cpp
#include <iostream>
using namespace std;
class Rec
{
const int length;
int width;
public:
void set_length(int l);
void set_width(int w);
int get_length();
int get_width();
void show();
Rec():length(3),width(4)
{
cout<<"无参:"<<endl;
cout <<"C:"<<2*(length+width)<<endl;
cout <<"S:"<<length*width<<endl;
}
Rec(int width):length(5)
{
cout <<"有参"<<endl;
cout <<"C:"<<2*(length+width)<<endl;
cout <<"S:"<<length*width<<endl;
}
};
void Rec::set_width(int width)
{
this->width=width;
}
int Rec::get_length()
{
return length;
}
int Rec::get_width()
{
return width;
}
void Rec::show()
{
cout <<"C:"<<2*(length+width)<<endl;
cout <<"S:"<<length*width<<endl;
}
int main()
{
Rec r1;
Rec r2(4);
r2.set_width(6);
r2.show();
return 0;
}
实现效果
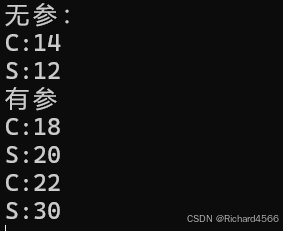
圆的参数
- 定义一个圆类,包含私有属性半径r,公有成员方法:
- void set_r(int r); //获取半径
- void show //输出周长和面积,show函数中需要一个提供圆周率的参数PI,该参数有默认值3.14
代码
cpp
#include <iostream>
using namespace std;
class yuan
{
int &r;
public:
void set_r(int r);
void show();
yuan(int &r):r(r){
cout <<"C="<<2*3.14*(float)r<<endl;
cout <<"S="<<3.14*(float)r*(float)r<<endl;
}
};
void yuan::set_r(int r)
{
this->r=r;
}
void yuan::show()
{
cout <<"C="<<2*3.14*(float)r<<endl;
cout <<"S="<<3.14*(float)r*(float)r<<endl;
}
int main()
{
int R=7;
yuan r1(R);
return 0;
}
实现效果
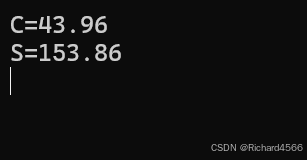
汽车的品类
- 定义一个Car类,包含私有属性,颜色color,品牌brand,速度speed,包含公有成员方法:
- void display(); //显示汽车的品牌,颜色和速度
- void acc(int a); //加速汽车
- set函数,设置类中的私有属性
代码
cpp
#include <iostream>
#include <iomanip>
using namespace std;
class Car
{
string cloor;
string ip;
int speed;
public:
void display();
void acc(int a);
void set_look(string c,string i,int s);
Car():cloor("black"),ip("别克"),speed(350)
{
cout <<"无参的构造函数:"<<endl;
cout <<"颜色:"<<setw(8)<<left<<cloor<<"品牌:"<<setw(8)<<left<<ip<<"速度:"<<setw(8)<<left<<speed<<endl;
}
Car(string cloor,string ip,int speed)
{
this->cloor=cloor;
this->ip=ip;
this->speed=speed;
cout <<"有参的构造函数"<<endl;
cout <<"颜色:"<<setw(8)<<left<<cloor<<"品牌:"<<setw(8)<<left<<ip<<"速度:"<<setw(8)<<left<<speed<<endl;
}
};
void Car::display()
{
cout <<"颜色:"<<setw(8)<<left<<cloor<<"品牌:"<<setw(8)<<left<<ip<<"速度:"<<setw(8)<<left<<speed<<endl;
}
void Car::acc(int a)
{
speed+=a;
}
void Car::set_look(string cloor,string ip,int speed)
{
this->cloor = cloor;
this->ip = ip;
this->speed=speed;
}
int main()
{
Car bieke;
Car penz("white","BWM",400);
penz.set_look("red","benz",360);
penz.acc(100);
penz.display();
return 0;
}
实现效果
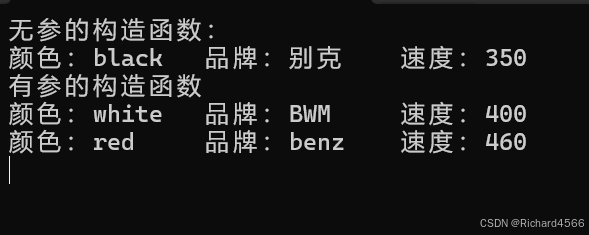
知识点思维导图
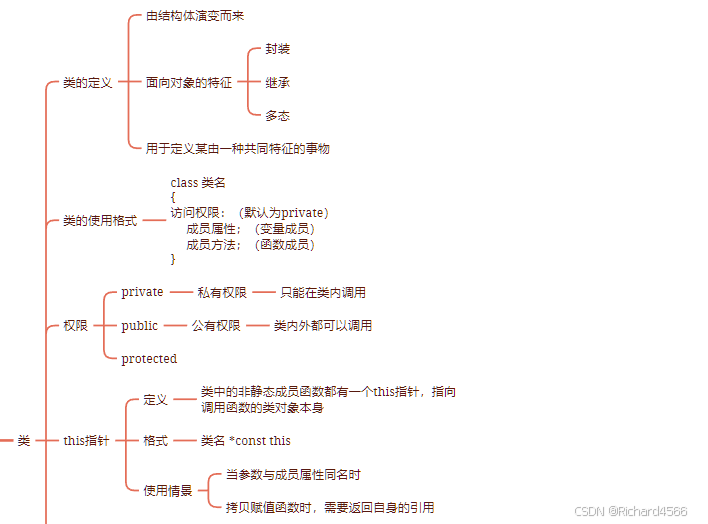
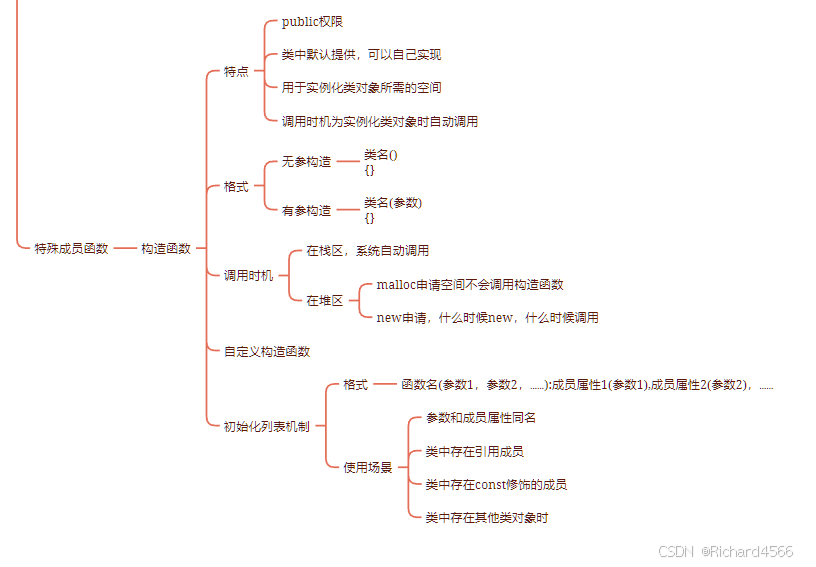