练习1:
cpp
#include <iostream>
using namespace std;
//定义一个复数类
class Complex
{
private:
double real;
double imag;
public:
//无参构造
Complex():real(0),imag(0){}
//有参构造
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 重载 + 运算符
Complex operator+(const Complex &other) const;
// 重载 - 运算符
Complex operator-(const Complex &other) const;
// 重载 * 运算符
Complex operator*(const Complex &other) const;
// 重载 / 运算符
Complex operator/(const Complex &other) const;
// 重载 < 运算符
bool operator<(const Complex &other) const;
// 重载 > 运算符
bool operator>(const Complex &other) const;
// 重载 == 运算符
bool operator==(const Complex &other) const;
bool operator!=(const Complex &other) const
{
return !(*this == other);
}
// 重载 && 运算符
bool operator&&(const Complex &other)const;
// 重载 || 运算符
bool operator||(const Complex &other)const;
// 重载逻辑非运算符
bool operator!() const;
// 重载 前++ 运算符
Complex &operator++();
// 重载 前-- 运算符
Complex &operator--();
// 重载 后++ 运算符
Complex operator++(int);
// 重载 后-- 运算符
Complex operator--(int);
// 重载 << 运算符(友元函数)
friend ostream &operator<<(ostream& out, const Complex& c)
{
out << c.real << " + " << c.imag << "i";
return out;
}
};
// 重载 + 运算符
Complex Complex::operator+(const Complex &other) const
{
return Complex(real + other.real, imag + other.imag);
}
// 重载 - 运算符
Complex Complex::operator-(const Complex &other) const
{
return Complex(real - other.real,imag - other.imag);
}
// 重载 * 运算符
Complex Complex::operator*(const Complex &other) const
{
return Complex((real*other.real-imag*other.imag),(real*other.imag+imag*other.real));
}
// 重载 / 运算符
Complex Complex::operator/(const Complex &other) const
{
return Complex((real*other.real+imag*other.imag)/(other.imag*other.imag+other.real*other.real),(imag*other.real-real*other.imag)/(other.imag*other.imag+other.real*other.real));
}
// 重载 < 运算符
bool Complex::operator<(const Complex &other) const
{
if((real*real+imag*imag)<(other.real*other.real+other.imag*other.imag))
{
return 1;
}
return 0;
}
// 重载 > 运算符
bool Complex::operator>(const Complex &other) const
{
if((real*real+imag*imag)>(other.real*other.real+other.imag*other.imag))
{
return 1;
}
return 0;
}
// 重载 == 运算符
bool Complex::operator==(const Complex &other) const
{
if((real*real+imag*imag)==(other.real*other.real+other.imag*other.imag))
{
return 1;
}
return 0;
}
// 重载 && 运算符
bool Complex::operator&&(const Complex &other)const
{
return (real != 0 || imag != 0) && (other.real != 0 || other.imag != 0);
}
// 重载 || 运算符
bool Complex::operator||(const Complex &other)const
{
return (real != 0 || imag != 0) || (other.real != 0 || other.imag != 0);
}
// 重载逻辑非运算符
bool Complex::operator!() const
{
return (real == 0 && imag == 0); // 当复数为0时返回true
}
// 重载 前++ 运算符
Complex &Complex::operator++()
{
this->real++;
this->imag++;
return *this;
}
// 重载 前++ 运算符
Complex &Complex::operator--()
{
this->real--;
this->imag--;
return *this;
}
// 重载 后++ 运算符
Complex Complex::operator++(int)
{
Complex temp = *this;
this->real++;
this->imag++;
return temp;
}
// 重载 后++ 运算符
Complex Complex::operator--(int)
{
Complex temp = *this;
this->real--;
this->imag--;
return temp;
}
int main()
{
Complex a(1, 2);
Complex b(3, 4);
Complex c = a + b; // 使用重载的 + 运算符
cout << "************** 加减乘除 ***************" << endl;
cout << "a + b >> " << c << endl;; // 使用重载的 << 运算符
c = a - b;
cout << "a - b >> " << c << endl;;
c = a / b;
cout << "a / b >> " << c << endl;
c = a * b;
cout << "a * b >> "<< c << endl;;
cout << "************** 关系运算符 ***************" << endl;
cout << "(a < b) >> " <<(a < b) << endl;
cout << "(a > b) >> " << (a > b) << endl;
cout << "(a == b) >> " << (a == a) << endl;
cout << "(a == b) >> " <<(a == b) << endl;
cout << "************** 逻辑运算符 ***************" << endl;
cout << "a&&b >> " << (a&&b) << endl;
cout << "a||b >> " << (a||b) << endl;
cout << "!a >> " << !a << endl;
cout << "!(!a) >> " << !(!a) << endl;
cout << "************** 前自增自减 ***************" << endl;
cout << "c >> " << c << endl;
cout << "++c >> " << ++c << endl;
cout << "--c >> " << --c << endl;
cout << "************** 后自增自减 ***************" << endl;
cout << "c >> " << c << endl;
cout << "c++ >> " << c++ << endl;
cout << "c >> " << c << endl;
cout << "c-- >> " << c-- << endl;
cout << "c >> " << c << endl;
return 0;
}
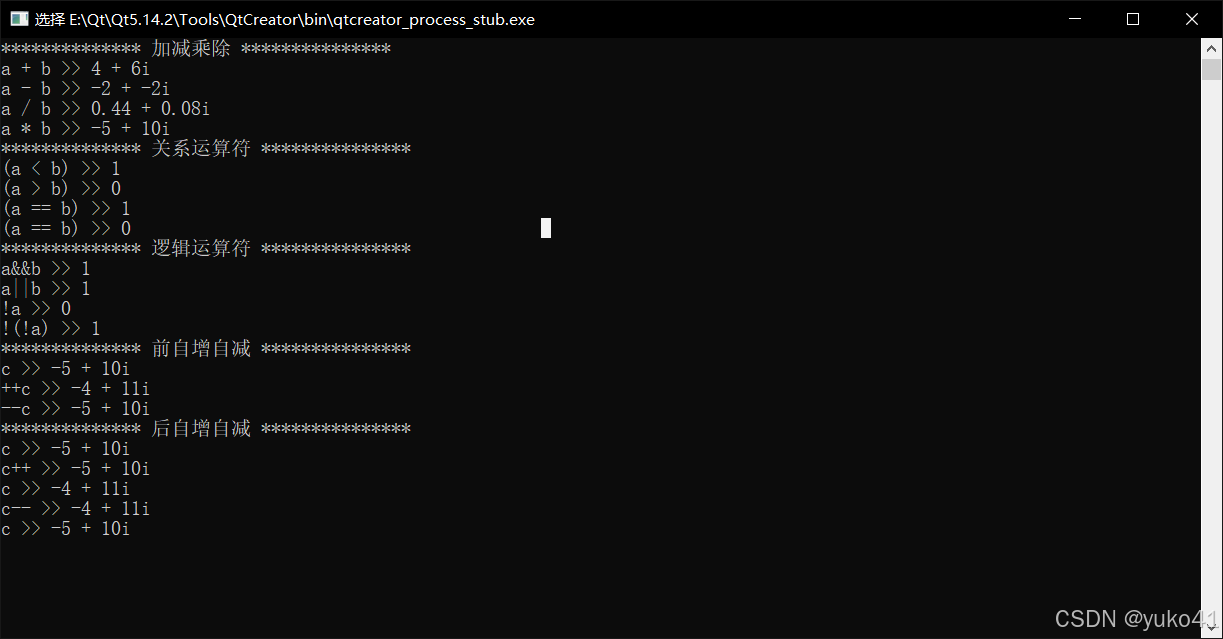
练习2:
#include <iostream>
#include <cstring>
using namespace std;
class Mystring
{
char *str;
int len;
public:
// 无参构造函数
Mystring() : str(new char[1]), len(0)
{
str[0] = '\0'; // 确保空字符串以null结尾
}
// 有参构造函数
Mystring(const char *str)
{
len = strlen(str);
this->str = new char[len + 1]; // +1 为结束符留空间
strcpy(this->str, str);
}
~Mystring()
{
delete [] str;
}
//拷贝构造函数
Mystring(const Mystring &other)
{
this->len = other.len;
this->str = new char[other.len+1];
strcpy(this->str,other.str);
}
// 拷贝赋值函数
Mystring &operator=(const Mystring &other)
{
if (this != &other)
{ // 添加自赋值检查
delete[] str; // 先删除原有内存
len = other.len;
str = new char[len + 1]; // +1 为结束符留空间
strcpy(str, other.str);
}
return *this;
}
// 加法运算符重载
Mystring operator+(const Mystring &other) const // 返回新对象,参数使用const
{
Mystring result;
result.len = this->len + other.len;
delete[] result.str; // 删除默认构造函数分配的内存
result.str = new char[result.len + 1]; // +1 为结束符留空间
strcpy(result.str, this->str);
strcat(result.str, other.str);
return result;
}
// 重载 == 运算符
bool operator==(const Mystring& other) const {
if (len != other.len) {
return false;
}
return strcmp(str, other.str) == 0;
}
// 重载 > 运算符
bool operator>(const Mystring& other) const
{
return strcmp(str, other.str) > 0;
}
// 重载 < 运算符
bool operator<(const Mystring& other) const
{
return strcmp(str, other.str) < 0;
}
char &my_at(int location)
{
if(location<0||location>len)
{
perror("越界访问");
exit(1);
}
return *(str+location);
}
void show()
{
printf(str);
}
};
int main()
{
char s[10] = "hello";
Mystring str1(s);
str1.show();
cout << endl;
Mystring str2("world");
str2.show();
cout << endl;
Mystring str3 = str2; // 复制构造
str3.show();
cout << endl;
Mystring str4;
str4 = str1 + str2; // 使用加法运算符
str4.show();
cout << endl;
//cout << str4.my_at(16) << endl;
cout << (str3 == str2) << endl; // 输出1(true)
cout << (str1 == str3) << endl; // 输出0(false)
cout << (str1 > str3) << endl; // 输出0(false,因为'h'<'w')
cout << (str3 > str1) << endl; // 输出1(true)
return 0;
}
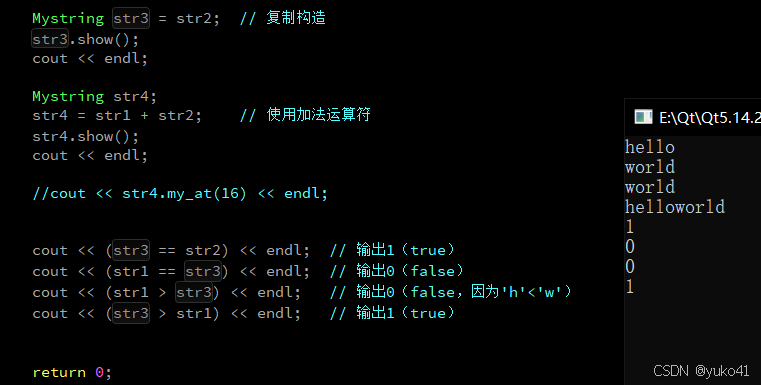