1. 面试题 17.14. 最小K个数
设计一个算法,找出数组中最小的k个数。以任意顺序返回这k个数均可。
示例:
输入: arr = [1,3,5,7,2,4,6,8], k = 4
输出: [1,2,3,4]
方法一:堆
c
class Solution {
public:
vector<int> smallestK(vector<int>& arr, int k) {
// topk问题------找最小的k个数建立大堆
vector<int> ret;
priority_queue<int> heap(arr.begin(), arr.begin() + k);
for (int i = k; i < arr.size(); i++){
if (!heap.empty()){
int top = heap.top();
if (arr[i] < top){
heap.pop();
heap.push(arr[i]);
}
}
}
for (int i = 0; i < k; i++){
ret.push_back(heap.top());
heap.pop();
}
reverse(ret.begin(), ret.end());
return ret;
}
};
方法二:排序
c
class Solution {
public:
vector<int> smallestK(vector<int>& arr, int k) {
// 使用排序
sort(arr.begin(), arr.end());
return vector<int>(arr.begin(), arr.begin() + k);
}
};
方法三:快排
c
class Solution {
public:
void Sort(vector<int> &arr, int left, int right){
// 三路划分+随机去基准值
if (left >= right) return;
int l = left - 1, r = right + 1;
int cur = left;
int key = arr[rand() % (right - left + 1) + left];
while (cur < r){
if (arr[cur] < key){
swap(arr[cur], arr[l + 1]);
l++;
cur++;
}else if (arr[cur] > key){
swap(arr[cur], arr[r - 1]);
// 这里不需要进行cur++,因为缓过来的数还没进行判断
r--;
}else{
cur++;
}
}
Sort(arr, left, l);
Sort(arr, r, right);
}
vector<int> smallestK(vector<int>& arr, int k) {
// 使用排序
Sort(arr, 0, arr.size() - 1);
return vector<int>(arr.begin(), arr.begin() + k);
}
};
2. LCR 154. 复杂链表的复制
请实现 copyRandomList 函数,复制一个复杂链表。在复杂链表中,每个节点除了有一个 next 指针指向下一个节点,还有一个 random 指针指向链表中的任意节点或者 null。
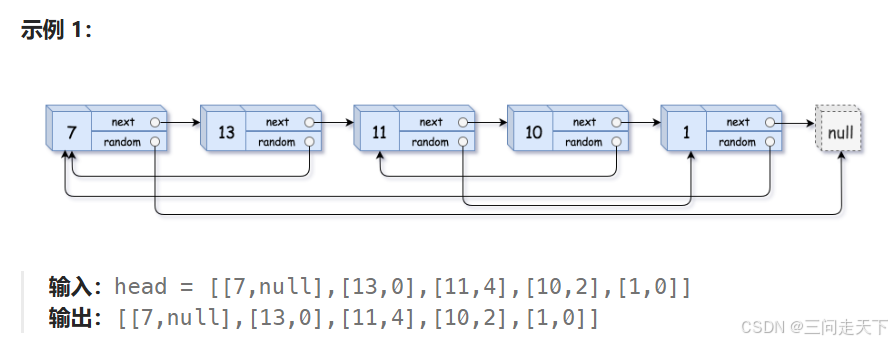
方法一:拼接+拆分
c
class Solution {
public:
Node* copyRandomList(Node* head) {
if (head == nullptr) return nullptr;
// 1. 将新赋值的节点都追加到原节点的next下
Node* cur = head;
while (cur != nullptr){
Node* next = cur->next;
Node* newNode = new Node(cur->val);
cur->next = newNode;
newNode->next = next;
cur = next;
}
// 新创建的randow就是原节点的random的next节点
cur = head;
while (cur != nullptr){
Node* newcur = cur->next;
if (cur->random == nullptr){
newcur->random = nullptr;
}else{
newcur->random = cur->random->next;
}
cur = cur->next->next;
}
// 将原节点与新节点断开
Node* ret = head->next;
cur = head;
while (cur){
Node* newcur = cur->next;
Node* next = newcur->next;
if (next != nullptr) newcur->next = next->next;
cur->next = next;
cur = next;
}
return ret;
}
};
方法二:哈希
c
class Solution {
public:
Node* copyRandomList(Node* head) {
if (head == nullptr) return nullptr;
unordered_map<Node*, Node*> hash;
Node* cur = head;
while (cur){
hash[cur] = new Node(cur->val);
cur = cur->next;
}
cur = head;
while (cur){
hash[cur]->next = hash[cur->next];
hash[cur]->random = hash[cur->random];
cur = cur->next;
}
return hash[head];
}
};