一、动效设计
1、太阳系
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body{
margin: 0;
background-color: #000;
}
#sun{
width: 50px;
height: 50px;
background-color: white;
border-radius: 50%;
box-shadow: 0 0 20px 5px #fcd85d;
background-image: linear-gradient(#fcd85d 0%,#e1d300 50%,#d39b03 100%);
}
.center{
position: absolute;
top: 0;
left: 0;
bottom: 0;
right: 0;
margin: auto;
}
#planet1-box{
width: 200px;
height: 200px;
border-radius: 50%;
border:1px solid #666;
}
#planet1{
border-radius: 50%;
width: 20px;
height: 20px;
position: absolute;
background-color: #fff;
top: 90px;
left: -10px;
transform-origin: 110px 10px;
transform:rotate(45deg);
animation: circle 10s linear infinite;
}
#planet2-box{
width: 250px;
height: 250px;
border-radius: 50%;
border:1px solid #666;
}
#planet2{
border-radius: 50%;
width: 20px;
height: 20px;
position: absolute;
background-color: orange;
top: 115px;
left: -10px;
transform-origin: 135px 10px;
/* transform:rotate(45deg); */
animation: circle 10s 2s linear infinite;
}
#p2-starline{
position: absolute;
width: 30px;
height: 30px;
left: -6px;
top: -6px;
border:1px solid orange;
border-radius: 50%;
transform: skew(15deg);
}
@keyframes circle {
0%{
transform:rotate(0deg);
}
100%{
transform:rotate(360deg);
}
}
#sun,#planet1-box,#planet2-box{
transform: skewX(30deg);
}
</style>
</head>
<body>
<div id="sun" class="center"></div>
<div id="planet1-box" class="center">
<div id="planet1"></div>
</div>
<div id="planet2-box" class="center">
<div id="planet2">
<div id="p2-starline"></div>
</div>
</div>
</body>
</html>
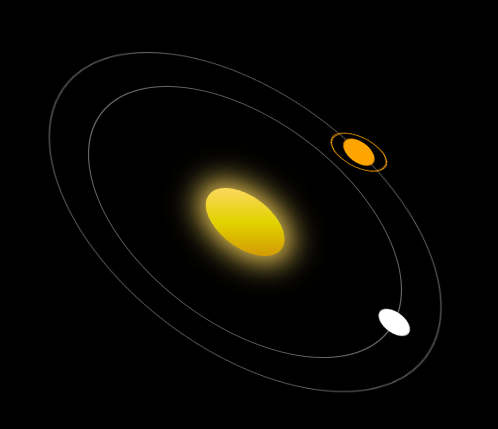
2、3d按钮
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
p{
width: 100px;
height: 50px;
text-align: center;
line-height: 50px;
color: white;
font-weight: bold;
background-image:linear-gradient(#549cc2,#397e9e);
box-shadow:0px 10px 0px 0px #333, 0 10px 10px 0px #000;
transform:rotateX(30deg);
margin: auto;
left: 0;
top: 0;
bottom: 0;
right: 0;
position: absolute;
border-radius: 2px;
transition: all .2s;
}
p:active{
transform:translateY(10px) rotateX(30deg);
box-shadow: 0 0 3px #000;
}
</style>
</head>
<body>
<p>click me</p>
</body>
</html>
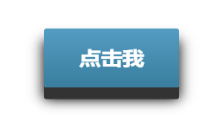
3、skew
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div{
margin: auto;
left: 0;
top: 0;
bottom: 0;
right: 0;
position: absolute;
width: 200px;
height: 200px;
background-color: red;
transform:skewX(0deg);
line-height: 200px;
text-align: center;
font-size: 30px;
color: white;
}
</style>
</head>
<body>
<div>skew</div>
</body>
</html>
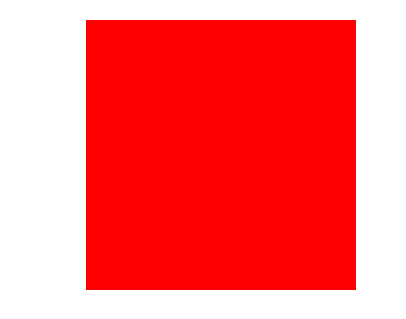
4、正方形
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#top,#center,#right{
width: 200px;
height: 200px;
margin: auto;
left: 0;
top: 0;
bottom: 0;
right: 0;
position: absolute;
}
#top{
top:-300px;
background-color: green;
transform:skewX(-45deg);
height: 100px;
left: 100px;
}
#right{
background-color: purple;
left: 400px;
transform:skewY(-45deg);
width: 100px;
transition: all 1s;
}
#center{
background-color: red;
}
#right:hover{
top: -100px;
left: 300px;
}
#line1,#line-rotate{
width: 200px;
margin: auto;
left: 0;
top: 0;
bottom: 0;
right: 0;
position: absolute;
}
#line1{
height: 200px;
border-left: 3px dashed white;
border-bottom: 3px dashed white;
transform:translate(100px,-100px);
}
#line-rotate{
height: 0px;
border-bottom:3px dashed white;
left: 155px;
transform-origin: 0 0;
transform:rotate(135deg);
width: 145px;
}
</style>
</head>
<body>
<div id="top"></div>
<div id="center"></div>
<div id="right"></div>
<div id="line1"></div>
<div id="line-rotate"></div>
</body>
</html>
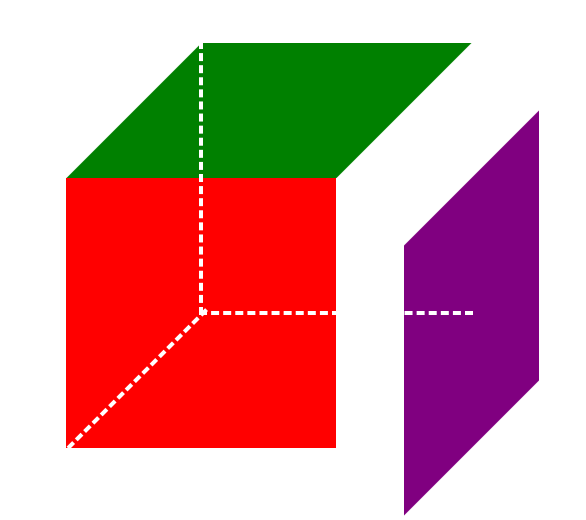
5、景深
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div{
width: 1000px;
height: 500px;
background-color: rgba(0,0,0,0.5);
position: relative;
margin: 200px auto;
/* 标签跟屏幕有500px的距离 */
perspective: 200px;
transform-style: preserve-3d;
}
#inner{
width: 200px;
height: 200px;
line-height: 200px;
text-align: center;
font-size: 30px;
color: white;
margin: auto;
left: 0;
top: 0;
bottom: 0;
right: 0;
position: absolute;
background-color: red;
animation: circle 20s infinite linear;
}
@keyframes circle {
0%{
transform: rotateY(0deg);
}
100%{
transform: rotateY(360deg);
}
}
</style>
</head>
<body>
<div>
<div id="inner">inner</div>
</div>
</body>
</html>
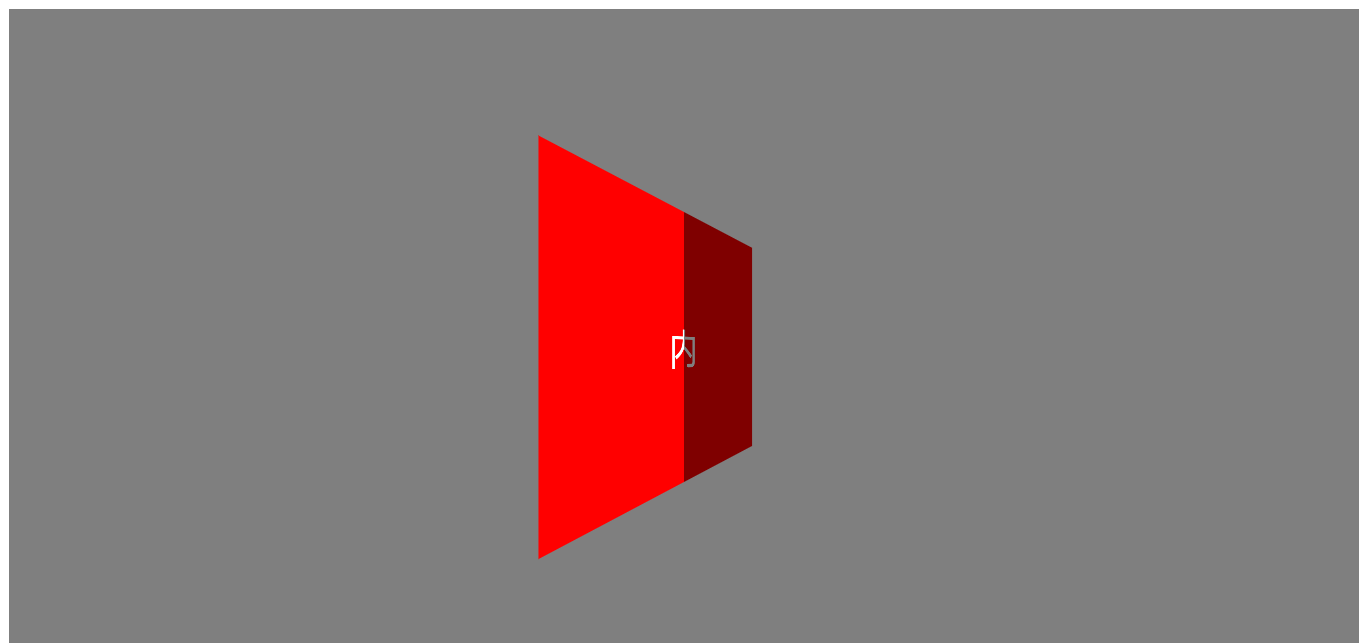
6、3d窗口
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#box{
width: 400px;
height: 150px;
line-height: 150px;
margin: 100px auto;
display: flex;
perspective: 200px;
transform-style: preserve-3d;
}
#left,#right{
width: 200px;
font-size: 30px;
font-weight: bold;
background-color: lightblue;
}
#left{
text-align:right ;
transform-origin: left center;
transform:rotateY(-30deg);
}
#right{
text-align: left;
transform-origin: right center;
transform:rotateY(30deg);
}
</style>
</head>
<body>
<div id="box">
<div id="left">HT</div>
<div id="right">ML</div>
</div>
</body>
</html>
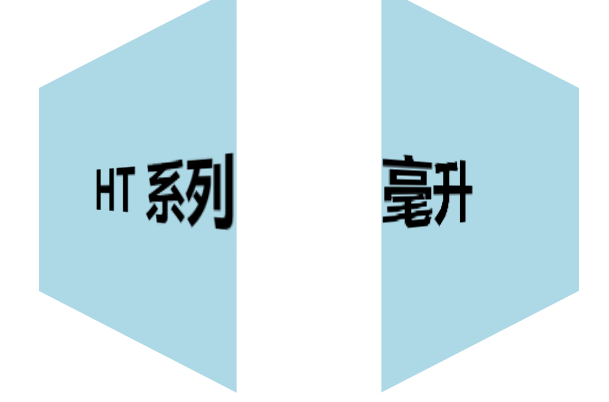
7、translate3d
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div{
width: 1000px;
height: 500px;
background-color: rgba(0,0,0,0.5);
position: relative;
margin: 200px auto;
/* 标签跟屏幕有500px的距离 */
perspective: 200px;
transform-style: preserve-3d;
}
#inner{
width: 200px;
height: 200px;
line-height: 200px;
text-align: center;
font-size: 30px;
color: white;
margin: auto;
left: 0;
top: 0;
bottom: 0;
right: 0;
position: absolute;
/* background-color: red; */
animation: circle 10s infinite linear;
background-image: url("images/line.png");
background-repeat: no-repeat;
background-size: cover;
}
@keyframes circle {
0%{
transform:rotate3d(1,1,1,0deg);
}
100%{
transform:rotate3d(1,1,1,360deg);
}
}
</style>
</head>
<body>
<div>
<div id="inner"></div>
</div>
</body>
</html>
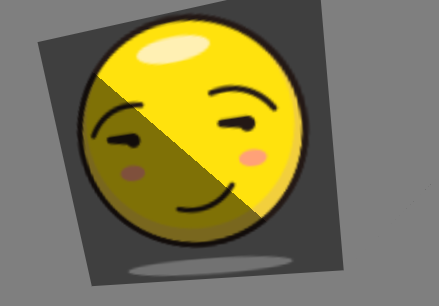
8、 rotate3d
9、transform-origin
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div{
width: 1000px;
height: 500px;
background-color: rgba(0,0,0,0.5);
position: relative;
margin: 200px auto;
/* 标签跟屏幕有500px的距离 */
perspective: 200px;
transform-style: preserve-3d;
}
#inner{
width: 200px;
height: 200px;
line-height: 200px;
text-align: center;
font-size: 30px;
color: white;
margin: auto;
left: 0;
top: 0;
bottom: 0;
right: 0;
position: absolute;
/* background-color: red; */
animation: circle 10s infinite linear;
background-image: url("images/line.png");
background-repeat: no-repeat;
background-size: cover;
transform-origin: 50% 50% -100px;
}
@keyframes circle {
0%{
transform:rotateY(0deg)
}
100%{
transform:rotateY(360deg)
}
}
</style>
</head>
<body>
<div>
<div id="inner"></div>
</div>
</body>
</html>
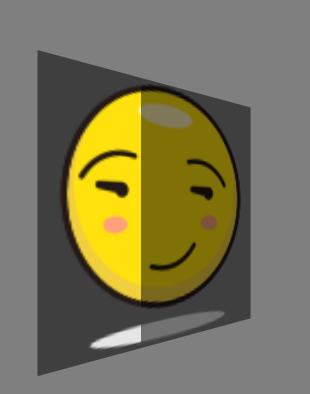
10、3d骰子
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body,html{
height: 100%;
}
body{
perspective: 500px;
}
#box{
width: 200px;
height: 200px;
margin: auto;
left: 0;
top: 0;
bottom: 0;
right: 0;
position: absolute;
transform-origin: 100px 100px -100px;
transform-style: preserve-3d;
transition: all 2s;
/* animation: circle 10s infinite linear; */
}
#box:hover{
transform: rotateX(90deg);
}
#box div{
width: 200px;
height: 200px;
background-color: rgba(15, 160, 201,0.5);
position: absolute;
top: 0;
left: 0;
text-align: center;
line-height: 200px;
font-size: 50px;
border-radius: 10px;
}
#box .item2{
transform-origin:left center;
transform:rotateY(90deg);
}
#box .item3{
transform-origin:right center;
transform:rotateY(-90deg);
}
#box .item4{
transform-origin:top center;
transform:rotateX(-90deg);
}
#box .item5{
transform-origin:bottom center;
transform:rotateX(90deg);
}
#box .item6{
/* transform-origin:bottom center; */
transform:translateZ(-200px);
}
@keyframes circle {
0%{
transform:rotate3d(0,1,0,0deg);
}
100%{
transform:rotate3d(0,1,0,360deg);
}
}
#box:hover{
animation-play-state: paused;
}
</style>
</head>
<body>
<div id="box">
<div class="item1">●</div>
<div class="item2">●●</div>
<div class="item3">●●●</div>
<div class="item4">●●●●</div>
<div class="item5">●●●●●</div>
<div class="item6">●●●●●●</div>
</div>
</body>
</html>
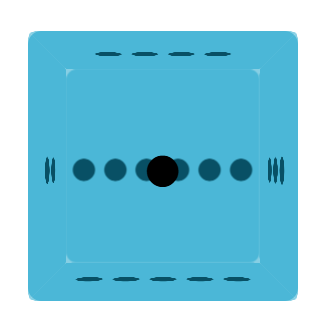
二、CSS3特效
1、电影特效
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#list{
width: 1000px;
display: flex;
justify-content: space-evenly;
flex-wrap: wrap;
}
.space{
perspective: 400px;
width: 300px;
height: 100px;
margin-top: 15px;
}
.box{
transform-style: preserve-3d;
transform-origin: 150px 50px -50px;
width: 300px;
height: 100px;
position: relative;
transition: all 2s;
}
.box:hover{
transform: rotateX(90deg);
}
.box img,.box div{
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
.box img{
/* opacity: 0.3; */
}
.box div{
background-color: #ddd;
transform-origin: top center;
transform:rotateX(-90deg) translateZ(100px);
}
.box div h3{
margin: 0;
}
.box div p{
word-wrap: break-word;
}
</style>
</head>
<body>
<div id="list">
<!-- 设置景深 -->
<div class="space">
<!-- 设置3d子元素 -->
<div class="box">
<img src="images/1.jpg" alt="">
<div>
<h3>阿巴阿巴</h3>
<p>abaabaabaabaabaabaabaabaabaabaabaabaabaabaaba</p>
</div>
</div>
</div>
<!-- 设置景深 -->
<div class="space">
<!-- 设置3d子元素 -->
<div class="box">
<img src="images/1.jpg" alt="">
<div>
<h3>阿巴阿巴</h3>
<p>abaabaabaabaabaabaabaabaabaabaabaabaabaabaaba</p>
</div>
</div>
</div>
<!-- 设置景深 -->
<div class="space">
<!-- 设置3d子元素 -->
<div class="box">
<img src="images/1.jpg" alt="">
<div>
<h3>阿巴阿巴</h3>
<p>abaabaabaabaabaabaabaabaabaabaabaabaabaabaaba</p>
</div>
</div>
</div>
<!-- 设置景深 -->
<div class="space">
<!-- 设置3d子元素 -->
<div class="box">
<img src="images/1.jpg" alt="">
<div>
<h3>阿巴阿巴</h3>
<p>abaabaabaabaabaabaabaabaabaabaabaabaabaabaaba</p>
</div>
</div>
</div>
<!-- 设置景深 -->
<div class="space">
<!-- 设置3d子元素 -->
<div class="box">
<img src="images/1.jpg" alt="">
<div>
<h3>阿巴阿巴</h3>
<p>abaabaabaabaabaabaabaabaabaabaabaabaabaabaaba</p>
</div>
</div>
</div>
<!-- 设置景深 -->
<div class="space">
<!-- 设置3d子元素 -->
<div class="box">
<img src="images/1.jpg" alt="">
<div>
<h3>阿巴阿巴</h3>
<p>abaabaabaabaabaabaabaabaabaabaabaabaabaabaaba</p>
</div>
</div>
</div>
</div>
</body>
</html>
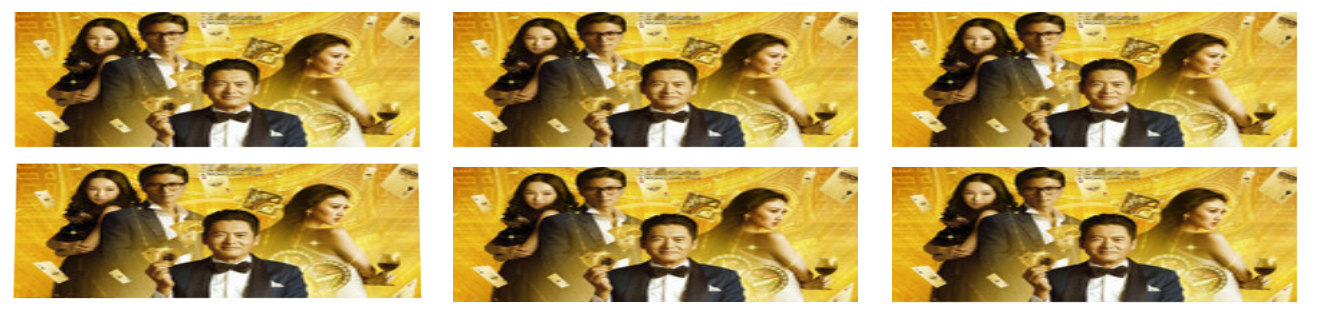
2、3d骰子-观察者
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body,html{
height: 100%;
}
body{
perspective: 500px;
perspective-origin: center top;
transition: all 1s;
}
body:hover{
perspective-origin: center;
}
#box{
width: 200px;
height: 200px;
margin: auto;
left: 0;
top: 0;
bottom: 0;
right: 0;
position: absolute;
transform-origin: 100px 100px -100px;
transform-style: preserve-3d;
transition: all 2s;
/* animation: circle 10s infinite linear; */
}
#box div{
width: 200px;
height: 200px;
background-color: rgba(15, 160, 201,0.5);
position: absolute;
top: 0;
left: 0;
text-align: center;
line-height: 200px;
font-size: 50px;
border-radius: 10px;
}
#box .item1{
transform: translateZ(0px);
}
#box .item2{
transform-origin:left center;
transform:rotateY(90deg) translateZ(00px);
}
#box .item3{
transform-origin:right center;
transform:rotateY(-90deg) translateZ(0px);
}
#box .item4{
transform-origin:top center;
transform:rotateX(-90deg) translateZ(0px);
}
#box .item5{
transform-origin:bottom center;
transform:rotateX(90deg) translateZ(0px);
}
#box .item6{
/* transform-origin:bottom center; */
transform:translateZ(-200px);
}
/* hover */
#box:hover .item1{
transform: translateZ(200px);
}
#box:hover .item2{
transform: rotateY(90deg) translateZ(-200px);
}
#box:hover .item3{
transform:rotateY(-90deg) translateZ(-200px);
}
#box:hover .item4{
transform:rotateX(-90deg) translateZ(-200px);
}
#box:hover .item5{
transform:rotateX(90deg) translateZ(-200px);
}
#box:hover .item6{
transform: translateZ(-400px);
}
@keyframes circle {
0%{
transform:rotate3d(0,1,0,0deg);
}
100%{
transform:rotate3d(0,1,0,360deg);
}
}
#box:hover{
/* animation-play-state: paused; */
animation: circle 10s 1s infinite linear;
}
#box div{
transition: all 1s;
}
</style>
</head>
<body>
<div id="box">
<div class="item1">●</div>
<div class="item2">●●</div>
<div class="item3">●●●</div>
<div class="item4">●●●●</div>
<div class="item5">●●●●●</div>
<div class="item6">●●●●●●</div>
</div>
</body>
</html>
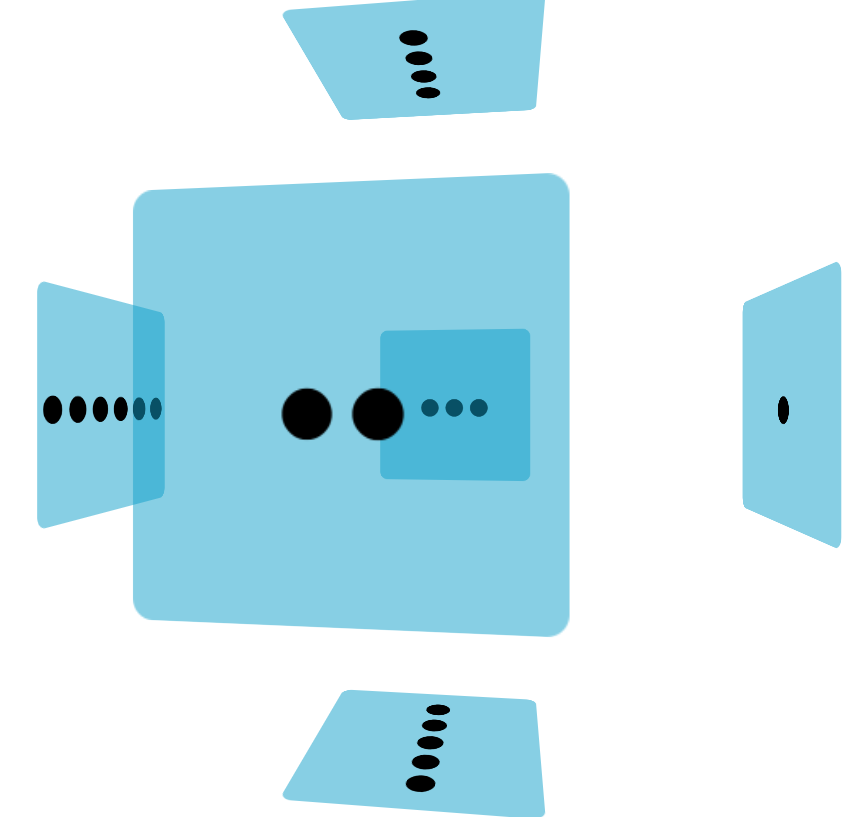
3、 3d骰子-走马灯
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body,html{
height: 100%;
}
body{
perspective: 500px;
perspective-origin: 50% center;
}
#box{
width: 200px;
height: 200px;
margin: auto;
left: 0;
top: 0;
bottom: 0;
right: 0;
position: absolute;
transform-origin: 100px 100px -100px;
transform-style: preserve-3d;
transition: all 2s;
/* animation: circle 10s infinite linear; */
}
#box:hover{
transform: rotateX(90deg);
}
#box div{
width: 200px;
height: 200px;
background-color: rgba(15, 160, 201,0.5);
position: absolute;
top: 0;
left: 0;
text-align: center;
line-height: 200px;
font-size: 50px;
border-radius: 10px;
}
#box .item2{
transform-origin:left center;
transform:rotateY(90deg);
}
#box .item3{
transform-origin:right center;
transform:rotateY(-90deg);
}
#box .item4{
transform-origin:top center;
transform:rotateX(-90deg);
}
#box .item5{
transform-origin:bottom center;
transform:rotateX(90deg);
}
#box .item6{
/* transform-origin:bottom center; */
transform:translateZ(-200px);
}
@keyframes circle {
0%{
transform:rotate3d(0,1,0,0deg);
}
100%{
transform:rotate3d(0,1,0,360deg);
}
}
#box:hover{
animation-play-state: paused;
}
</style>
</head>
<body>
<div id="box">
<div class="item1">●</div>
<div class="item2">●●</div>
<div class="item3">●●●</div>
<div class="item4">●●●●</div>
<div class="item5">●●●●●</div>
<div class="item6">●●●●●●</div>
</div>
</body>
</html>
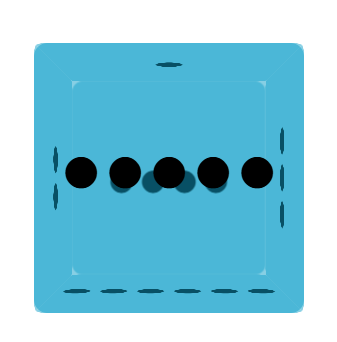
4、 css3选择器
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
table{
width: 600px;
margin: 0 auto;
border-spacing: 0;
text-align: center;
}
tr{
height: 50px;
}
td,th{
border:1px solid #ccc;
}
td:first-child{
color:red;
}
p:last-child{
color: blue;
}
.zs:first-child{
color: green;
}
td:nth-child(3){
color: yellowgreen;
}
tr:nth-child(2n-1){
background-color: #ddd;
}
tr:nth-child(2n){
background-color: #f3f3f3;
}
p:nth-last-child(2){
color:red;
}
/* p:nth-of-type(3){
background-color:red;
}
a:nth-of-type(2){
background-color:red;
} */
.item:nth-of-type(1){
background-color: red;
}
/* body :not(p,div,a){
background-color: rgb(126, 11, 233);
} */
/* tr的子标签,并且class不为item */
body tr :not(.item){
background-color: rgb(126, 11, 233);
}
</style>
</head>
<body>
<table>
<thead>
<tr>
<th>学号</th>
<th>姓名</th>
<th>年龄</th>
<th >性别</th>
</tr>
</thead>
<tbody>
<tr>
<td class="demo">001</td>
<td class="demo">张三</td>
<td >123456</td>
<td class="demo">34</td>
</tr>
<tr>
<td class="zs ">002</td>
<td class="item">小明</td>
<td>23</td>
<td class="">男</td>
</tr>
<tr>
<td >003</td>
<td class="item">周星星</td>
<td>20</td>
<td>女</td>
</tr>
<tr>
<td class="item">004</td>
<td>9527</td>
<td>30</td>
<td>男</td>
</tr>
<tr>
<td>005</td>
<td>李健仁</td>
<td>34</td>
<td>男</td>
</tr>
<tr>
<td>006</td>
<td>吴如花</td>
<td>40</td>
<td>女</td>
</tr>
</tbody>
</table>
<div>
<a href="#">第一个a标签</a>
<p >第一个p标签</p>
<a href="#" >第二个a标签</a>
<p >第二个p标签</p>
<a href="#" class="item">第三个a标签</a>
<p class="item">第三个p标签</p>
</div>
</body>
</html>
5、球体
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body{
background-color: #000;
}
#space,#box,#box div{
width: 200px;
height: 200px;
}
#space{
perspective: 500px;
margin: auto;
left: 0;
right: 0;
bottom: 0;
top: 0;
position: absolute;
transform:rotateZ(-30deg);
}
#box{
transform-style: preserve-3d;
position: relative;
transform-origin: center center;
animation: circle 15s infinite linear;
}
#box div{
position: absolute;
top: 0;
left: 0;
background-color: rgba(120,120,120,0.15);
border-radius: 50%;
}
#box div:first-child{
transform:rotateY(0deg);
}
#box div:nth-child(2){
transform:rotateY(30deg);
}
#box div:nth-child(3){
transform:rotateY(60deg);
}
#box div:nth-child(4){
transform:rotateY(90deg);
}
#box div:nth-child(5){
transform:rotateY(120deg);
}
#box div:nth-child(6){
transform:rotateY(150deg);
}
@keyframes circle {
0%{
transform:rotateY(0deg) ;
}
100%{
transform:rotateY(360deg) ;
}
}
</style>
</head>
<body>
<!-- 景深 -->
<div id="space">
<!-- 设置3d子元素 -->
<div id="box">
<!-- 子元素 -->
<div></div>
<div></div>
<div></div>
<div></div>
<div></div>
<div></div>
</div>
</div>
</body>
</html>
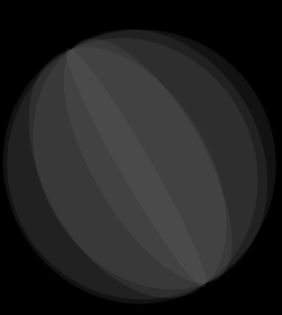
6、 css3-兄弟选择器
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
/* div p{
background-color: red;
} */
#box > p{
background-color: red;
}
</style>
</head>
<body>
<div id="box">
<a href="#">第一个a标签</a>
<p >第一个p标签</p>
<a href="#" >第二个a标签</a>
<p >第二个p标签</p>
<a href="#" class="item">第三个a标签</a>
<p class="item">第三个p标签</p>
<p>第四个p标签</p>
<p class="item">第五个p标签</p>
<div>
<p>子div里的p标签</p>
</div>
</div>
</body>
</html>
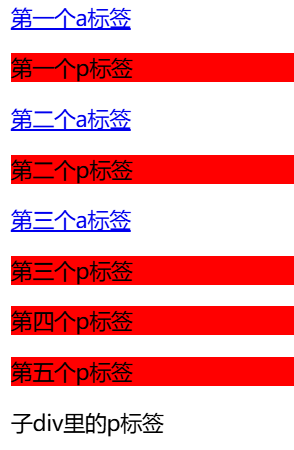
7、 css3-后代选择器 copy
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
/* div + p{
background-color: yellow;
}
.item + p{
background-color: yellow;
} */
div ~ p{
background-color: yellow;
}
</style>
</head>
<body>
<p>div 的上一个兄弟标签</p>
<div>
<a href="#">第一个a标签</a>
<p >第一个p标签</p>
<a href="#" >第二个a标签</a>
<p >第二个p标签</p>
<a href="#" class="item">第三个a标签</a>
<p class="item">第三个p标签</p>
<p>第四个p标签</p>
<p class="item">第五个p标签</p>
</div>
<p>div的真正的下一个兄弟标签</p>
<h1>插入一个兄弟标签</h1>
<p>这是div的 下一个兄弟p标签</p>
</body>
</html>
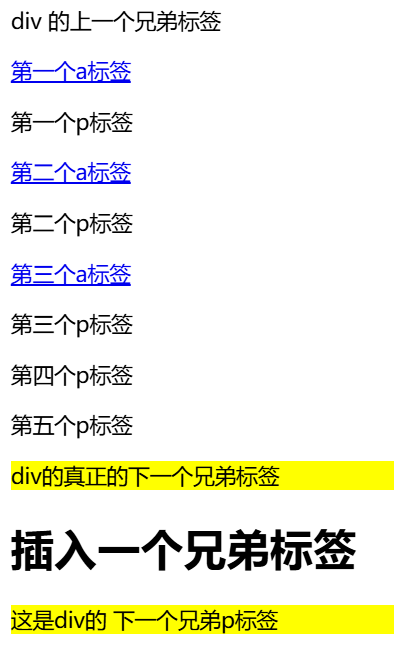
8、 css3-属性选择器
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
/* a[href]{
color: red;
} */
/* a[href="#"]{
color: red;
} */
/* a[href="http://www.baidu.com"]{
color: red;
} */
/* a[href*=abc]{
color:red;
} */
a[href~=abc]{
color:red;
}
*{
margin: 0;
padding: 0;
}
</style>
</head>
<body>
<div>
<a href="#">href=#的超链接</a> <br>
<a href="http://www.baidu.com">href=百度地址的超链接</a> <br>
<a >无href的超链接</a> <br>
<a href="">href为空的超链接</a> <br>
<a href="abc.html">href包含abc的超链接</a> <br>
</div>
</body>
</html>
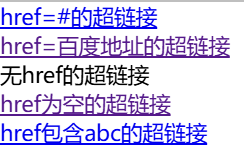
三、sass
1、球体
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body{
background-color: #000;
}
#space,#box,#box div{
width: 200px;
height: 200px;
}
#space{
perspective: 500px;
margin: auto;
left: 0;
right: 0;
bottom: 0;
top: 0;
position: absolute;
transform:rotateZ(-30deg);
perspective-origin: top center;
}
#box{
transform-style: preserve-3d;
position: relative;
transform-origin: center center;
animation: circle 15s infinite linear;
}
#box div{
position: absolute;
top: 0;
left: 0;
background-color: rgba(120,120,120,0.15);
border-radius: 50%;
border:1px solid #ccc;
box-sizing: border-box;
}
#box div:first-child{
transform:rotateY(0deg);
}
#box div:nth-child(2){
transform:rotateY(30deg);
}
#box div:nth-child(3){
transform:rotateY(60deg);
}
#box div:nth-child(4){
transform:rotateY(90deg);
}
#box div:nth-child(5){
transform:rotateY(120deg);
}
#box div:nth-child(6){
transform:rotateY(150deg);
}
@keyframes circle {
0%{
transform:rotateY(0deg) ;
}
100%{
transform:rotateY(360deg) ;
}
}
/* x轴子元素 */
#box .x{
/* background-color: rgba(245, 1, 1,0.3); */
transform:rotateX(90deg);
/* border:1px solid white; */
}
#box :nth-child(8){
transform:rotateX(90deg) translateZ(30px) scale(0.95);
}
#box :nth-child(9){
transform:rotateX(90deg) translateZ(60px) scale(0.8);
}
#box :nth-child(10){
transform:rotateX(90deg) translateZ(-30px) scale(0.95);
}
#box :nth-child(11){
transform:rotateX(90deg) translateZ(-60px) scale(0.8);
}
</style>
</head>
<body>
<!-- 景深 -->
<div id="space">
<!-- 设置3d子元素 -->
<div id="box">
<!-- 子元素 -->
<div></div>
<div></div>
<div></div>
<div></div>
<div></div>
<div></div>
<!-- x轴 -->
<div class="x"></div>
<div class="x"></div>
<div class="x"></div>
<div class="x"></div>
<div class="x"></div>
</div>
</div>
</body>
</html>
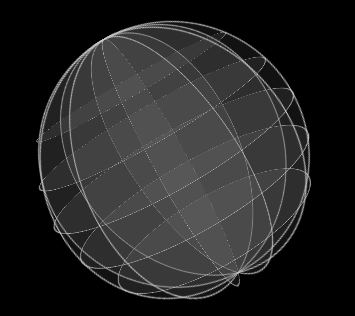
2、 轮播图
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div{
width: 1440px;
height: 500px;
margin: 0 auto;
overflow: hidden;
}
ul{
width: 500%;
height: 100%;
display: flex;
list-style: none;
padding: 0;
margin: 0;
transform:translateX(-0px);
animation: move 10s infinite;
}
li{
width: 1440px;
height: 100%;
}
li img{
width: 1440px;
height: 500px;
}
@keyframes move {
0%{
transform:translateX(-0px);
}
10%,20%{
transform:translateX(-1440px);
}
30%,40%{
transform:translateX(-2880px);
}
50%,60%{
transform:translateX(-4320px);
}
70%,80%{
transform:translateX(-5760px);
}
90%,100%{
transform: translateX(-7200px);
}
}
</style>
</head>
<body>
<div id="box">
<ul>
<li><img src="images/banner1.jpg" alt=""></li>
<li><img src="images/banner2.jpg" alt=""></li>
<li><img src="images/banner3.jpg" alt=""></li>
<li><img src="images/banner4.jpg" alt=""></li>
<li><img src="images/banner5.jpg" alt=""></li>
<li><img src="images/banner1.jpg" alt=""></li>
</ul>
</div>
</body>
</html>
3、轮播图-sass
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div{
width: 1440px;
height: 500px;
margin: 0 auto;
overflow: hidden;
}
ul{
width: 500%;
height: 100%;
display: flex;
list-style: none;
padding: 0;
margin: 0;
transform:translateX(-0px);
animation: move 10s infinite;
}
li{
width: 1440px;
height: 100%;
}
li img{
width: 1440px;
height: 500px;
}
@keyframes move {
0%{
transform:translateX(-0px);
}
10%,20%{
transform:translateX(-1440px);
}
30%,40%{
transform:translateX(-2880px);
}
50%,60%{
transform:translateX(-4320px);
}
70%,80%{
transform:translateX(-5760px);
}
100%{
transform: translateX(-7200px);
}
}
</style>
</head>
<body>
<div id="box">
<ul>
<li><img src="images/banner1.jpg" alt=""></li>
<li><img src="images/banner2.jpg" alt=""></li>
<li><img src="images/banner3.jpg" alt=""></li>
<li><img src="images/banner4.jpg" alt=""></li>
<li><img src="images/banner5.jpg" alt=""></li>
<li><img src="images/banner1.jpg" alt=""></li>
</ul>
</div>
</body>
</html>
3、 sass-嵌套
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div{
color: red;
}
p{
color: blue;
}
#box{
color: yellow;
}
#box:hover{
background-color: green;
}
#box:hover p{
color: white;
}
</style>
</head>
<body>
<div id="box">
<p>第一个p标签</p>
<p>第二个p标签</p>
</div>
</body>
</html>
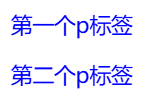
4、 sass-变量
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<header>header</header>
<main>main</main>
<footer>footer</footer>
</body>
</html>
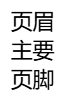
5、 scss-for循环
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div{
width: 200px;
height: 200px;
border:1px solid #ccc;
position: absolute;
margin: auto;
top: 0;
left: 0;
bottom: 0;
right: 0;
}
.item-1{
width: 200px;
height: 200px;
}
.item-2{
width: 250px;
height: 250px;
}
.item-3{
width: 300px;
height: 300px;
}
</style>
</head>
<body>
<div class="item-1"></div>
<div class="item-2"></div>
<div class="item-3"></div>
<div class="item-4"></div>
<div class="item-5"></div>
<div class="item-6"></div>
<div class="item-7"></div>
<div class="item-8"></div>
<div class="item-9"></div>
<div class="item-10"></div>
</body>
</html>
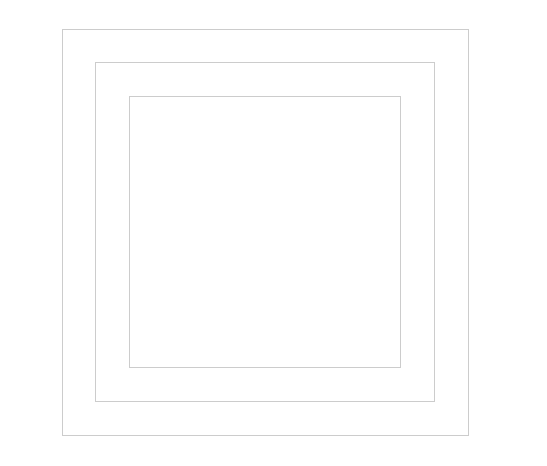
6、 sass-条件
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="css/变量.min.css">
</head>
<body>
<header>header</header>
<main>main</main>
<footer>footer</footer>
</body>
</html>
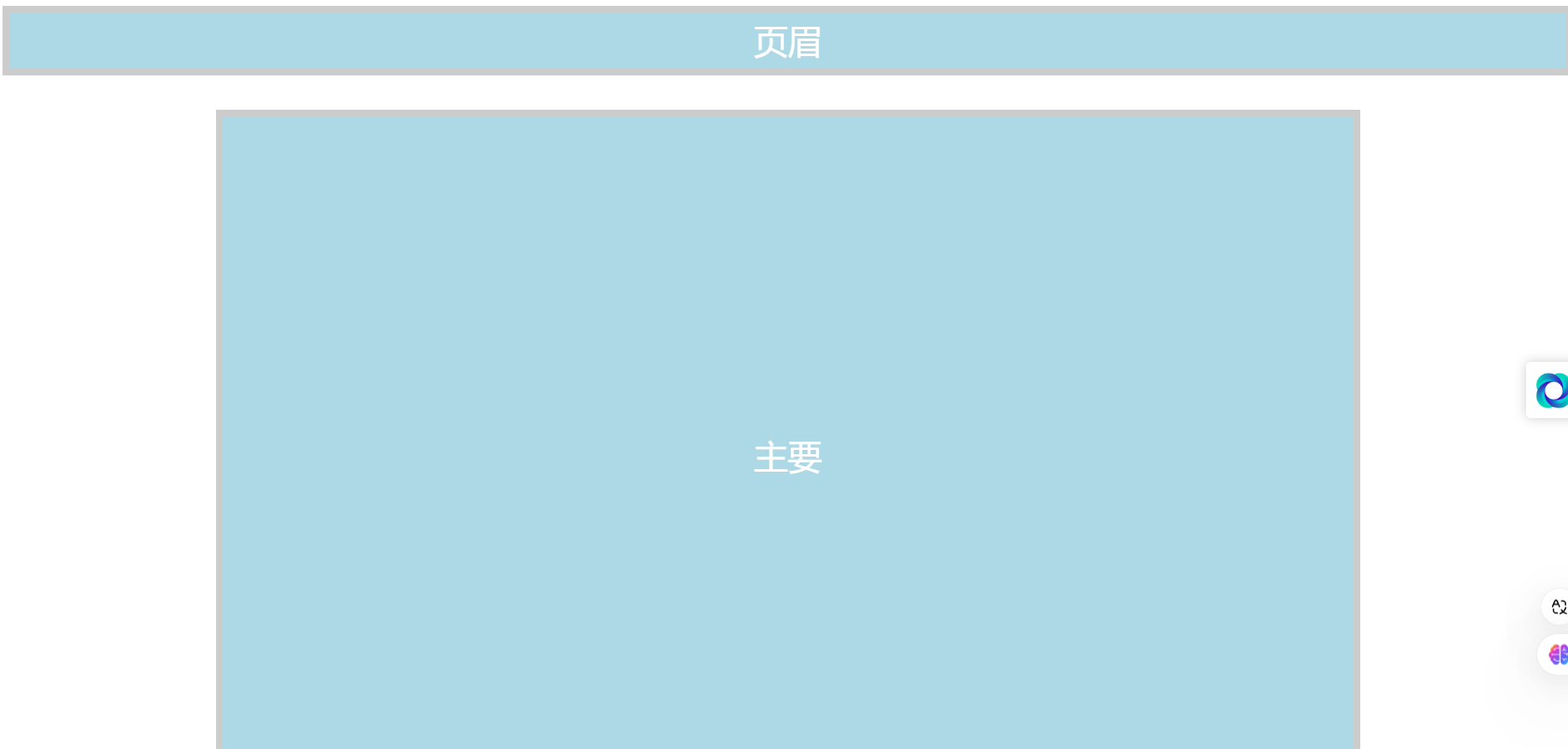
三、媒体设备类
1、媒体设备类型
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="媒体设备类型.css" media="only screen">
</head>
<body>
<div></div>
</body>
</html>
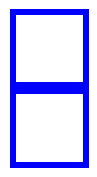
2、媒体特性
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="媒体特性.css">
</head>
<body>
<div></div>
</body>
</html>
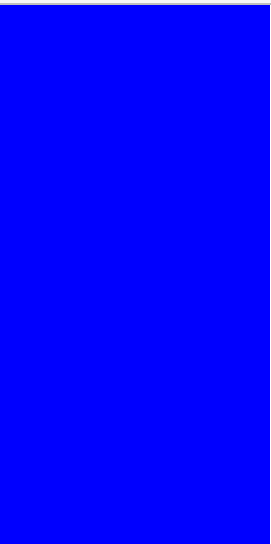
3、响应式布局练习
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<link rel="stylesheet" href="jq22.css">
</head>
<body>
<header>
<div id="nav">
<ul>
<li>第1项哈哈哈</li>
<li>第2项哈哈哈</li>
<li>第3项哈哈哈</li>
<li>第4项哈哈哈</li>
<li>第5项哈哈哈</li>
</ul>
</div>
<img src="images/logo.png" alt="" />
</header>
<main id="list">
<div class="item" >
<img src="images/id1.jpg" alt="" />
<p>[蜗牛]不锈钢装饰物品</p>
<p class="price">¥ 998</p>
</div>
<div class="item">
<img src="images/id1.jpg" alt="" />
<p>[蜗牛]不锈钢装饰物品</p>
<p class="price">¥ 998</p>
</div>
<div class="item">
<img src="images/id1.jpg" alt="" />
<p>[蜗牛]不锈钢装饰物品</p>
<p class="price">¥ 998</p>
</div>
<div class="item">
<img src="images/id1.jpg" alt="" />
<p>[蜗牛]不锈钢装饰物品</p>
<p class="price">¥ 998</p>
</div>
<div class="item">
<img src="images/id1.jpg" alt="" />
<p>[蜗牛]不锈钢装饰物品</p>
<p class="price">¥ 998</p>
</div>
<div class="item">
<img src="images/id1.jpg" alt="" />
<p>[蜗牛]不锈钢装饰物品</p>
<p class="price">¥ 998</p>
</div>
<div class="item">
<img src="images/id1.jpg" alt="" />
<p>[蜗牛]不锈钢装饰物品</p>
<p class="price">¥ 998</p>
</div>
<div class="item">
<img src="images/id1.jpg" alt="" />
<p>[蜗牛]不锈钢装饰物品</p>
<p class="price">¥ 998</p>
</div>
<div class="item">
<img src="images/id1.jpg" alt="" />
<p>[蜗牛]不锈钢装饰物品</p>
<p class="price">¥ 998</p>
</div>
<div class="item">
<img src="images/id1.jpg" alt="" />
<p>[蜗牛]不锈钢装饰物品</p>
<p class="price">¥ 998</p>
</div>
<div class="item">
<img src="images/id1.jpg" alt="" />
<p>[蜗牛]不锈钢装饰物品</p>
<p class="price">¥ 998</p>
</div>
<div class="item" >
<img src="images/id1.jpg" alt="" />
<p>[蜗牛]不锈钢装饰物品</p>
<p class="price">¥ 998</p>
</div>
</main>
</body>
</html>
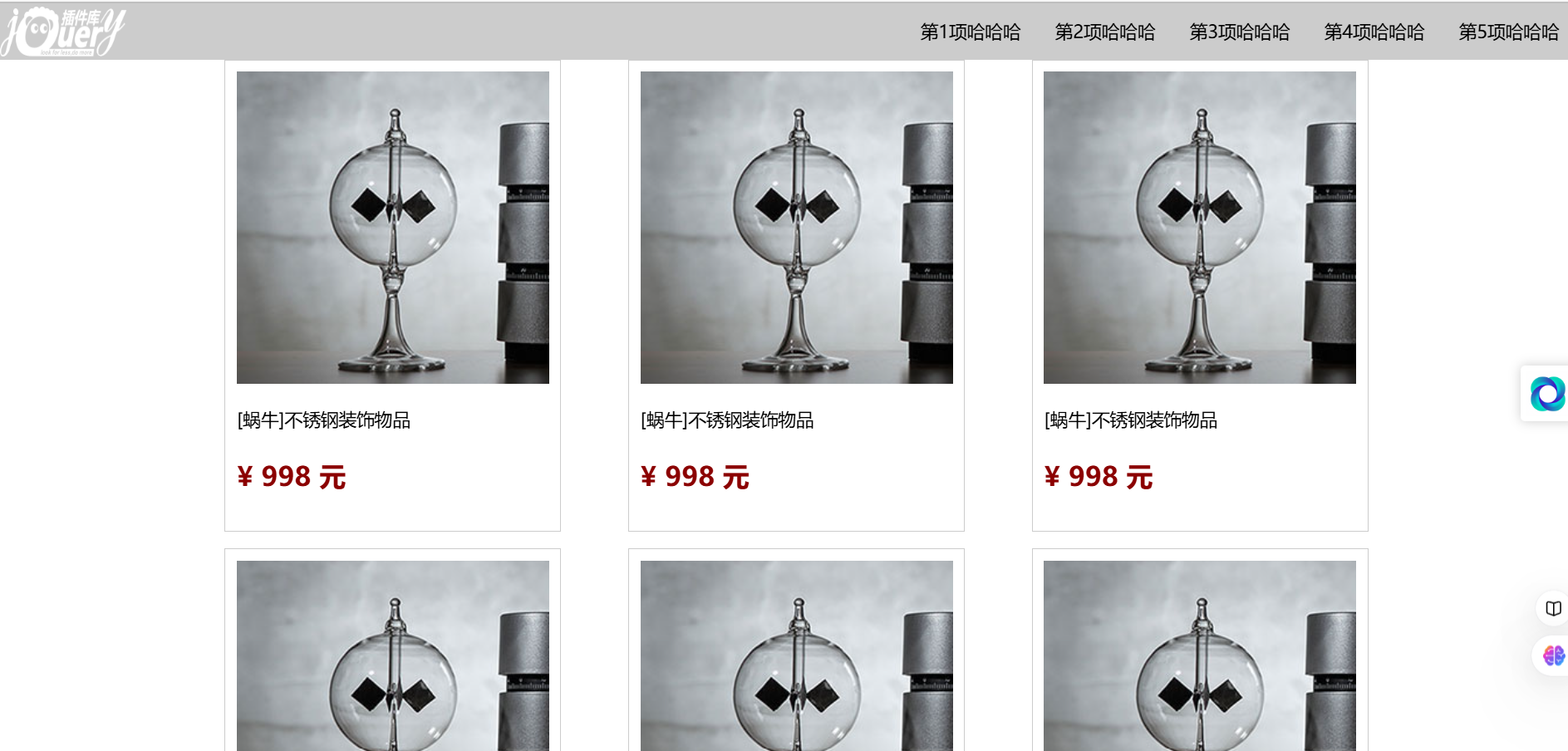
四、项目
1、宽度撑大
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body{
margin: 0;
}
header,footer{
height: 50px;
background-color:red;
line-height: 50px;
text-align: center;
}
footer{
margin-top: 30px;
background-color: blue;
}
main{
width: 90%;
margin: auto;
margin-top: 30px;
height: 600px;
background-color: purple;
}
#box{
width: 100%;
margin-left: 300px;
height: 500px;
}
</style>
</head>
<body>
<header>header</header>
<main>
<div id="box"></div>
</main>
<footer>footer</footer>
</body>
</html>
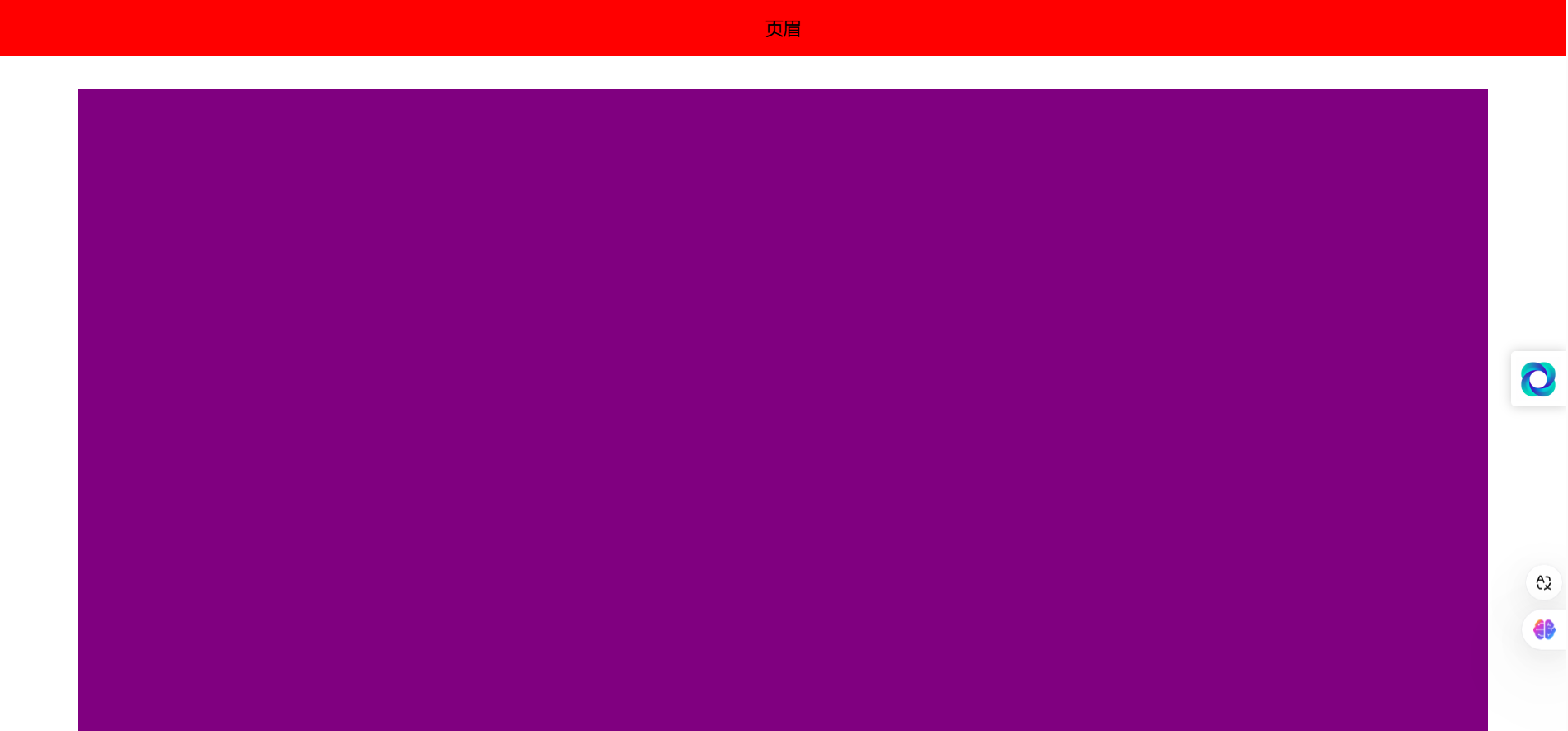
2、屏幕适配-2-pc端
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body{
font-size: 16px;
font-family: '微软雅黑';
}
header,footer{
height: 60px;
background-color: red;
color: white;
line-height: 60px;
}
header{
display: flex;
align-content: center;
justify-content: space-between;
}
header #logo{
width: 200px;
}
header nav{
width: 600px;
}
header nav ul{
list-style: none;
padding: 0;
margin: 0;
display: flex;
}
ul li{
flex-grow: 1;
}
main{
width: 100%;
height: 600px;
max-width: 1200px;
margin: 0 auto;
display: flex;
flex-wrap: nowrap;
min-width: 900px;
margin-top: 30px;
}
main #left{
width: 200px;
height: 100%;
background-color: darkred;
}
main #right{
flex-grow: 1;
margin-left: 15px;
background-color: purple;
}
.row{
height: 100px;
display: flex;
flex-wrap: nowrap;
}
.row >div{
margin-top: 15px;
}
.row :nth-child(1){
min-width: 200px;
background-color: green;
flex-grow: 1;
margin-left: 15px;
}
.row :nth-child(2){
min-width: 300px;
background-color: yellowgreen;
flex-grow: 1;
margin-left: 15px;
}
.row :nth-child(3){
min-width: 150px;
background-color: greenyellow;
flex-grow: 1;
margin-left: 15px;
margin-right: 15px;
}
</style>
</head>
<body>
<header>
<div class="logo">logo</div>
<nav>
<ul>
<li>第1项哈哈哈</li>
<li>第2项哈哈哈</li>
<li>第3项哈哈哈</li>
<li>第4项哈哈哈</li>
<li>第5项哈哈哈</li>
</ul>
</nav>
</header>
<main>
<div id="left"></div>
<div id="right">
<div class="row">
<div></div>
<div></div>
<div></div>
</div>
</div>
</main>
</body>
</html>
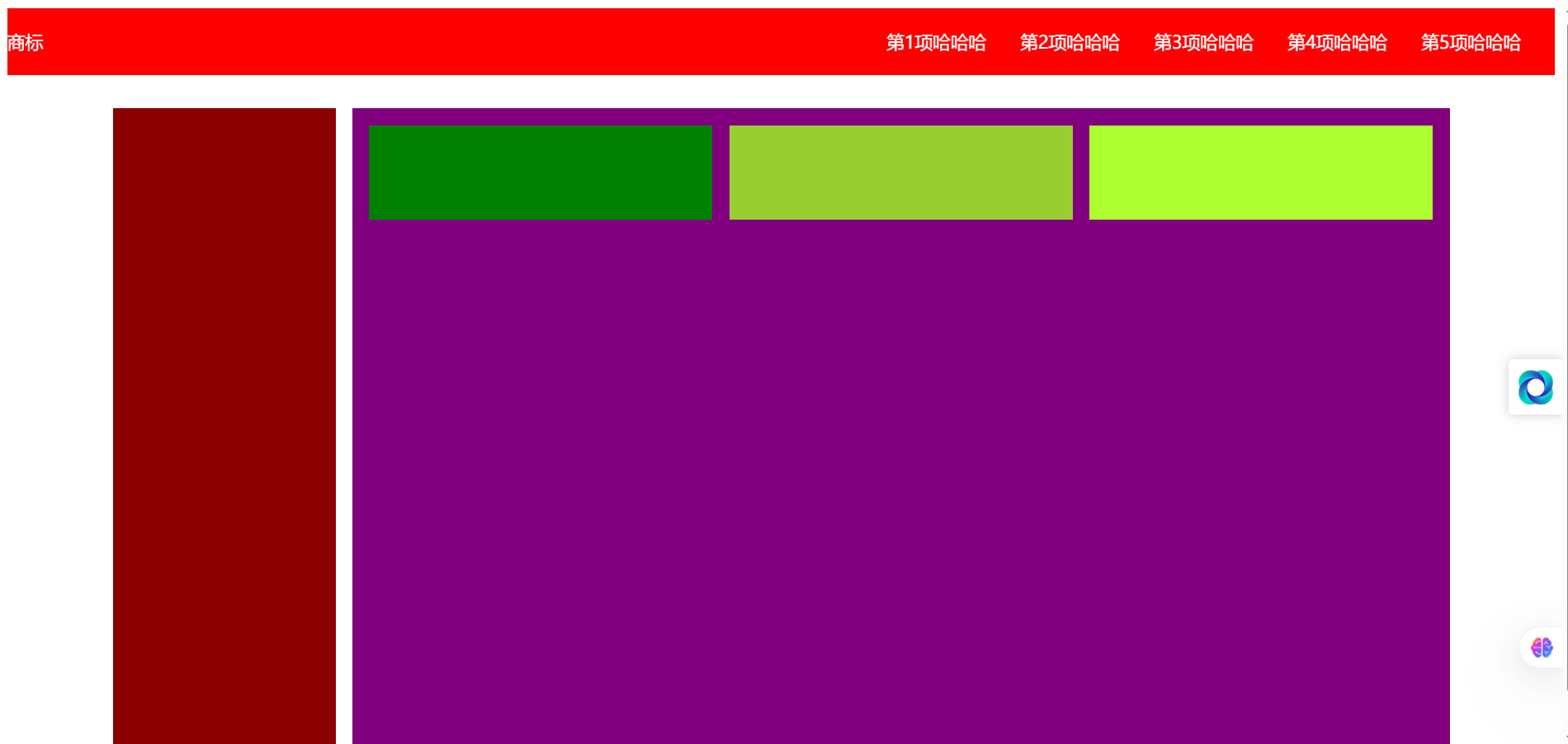
3、屏幕适配
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/font/bootstrap-icons.css">
<style>
header,footer{
min-width: 600px;
height: 100px;
width: 100%;
max-width: 1920px;
margin: 0 auto;
text-align: center;
}
header{
background-color: red;
margin-bottom: 100px;
}
footer{
background-color: blue;
}
</style>
</head>
<body>
<i class="bi-alarm" style="font-size: 2rem; color: cornflowerblue;"></i>
<header>header</header>
<footer>footer</footer>
</body>
</html>
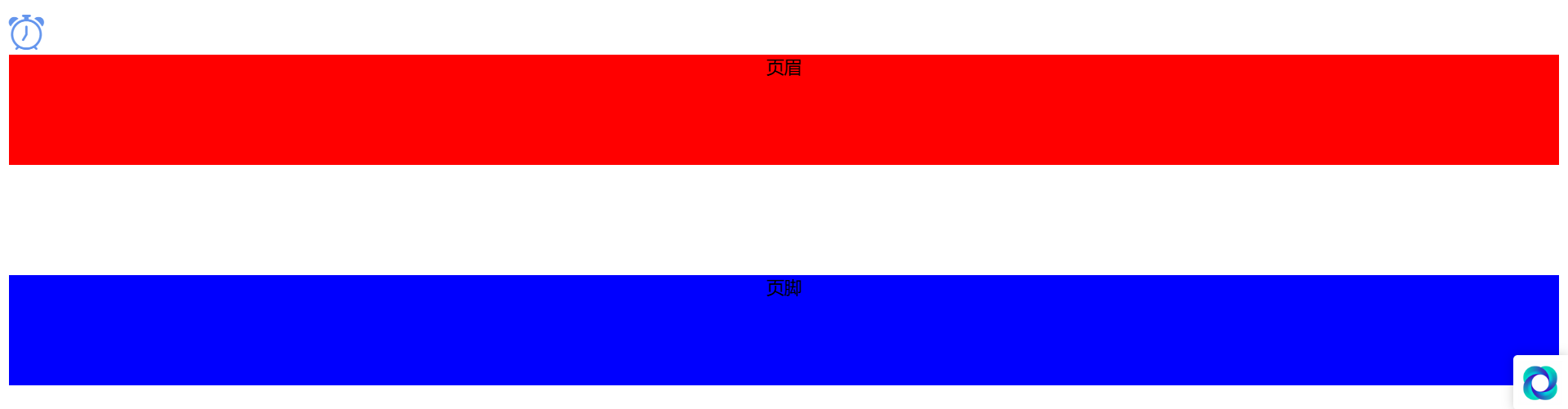
4、图标使用
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/font/bootstrap-icons.css">
</head>
<body>
<i class="bi bi-zoom-out"></i>
</body>
</html>
五、bootstrap
1、引入
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="bootstrap-4.6.0-dist/css/bootstrap.min.css">
</head>
<body>
<!-- 所有内容 -->
<button class="btn btn-info">登录</button>
<!--所有内容结束 -->
<!-- 保证使用bootstrap的组件能够正常工作 -->
<script src="jquery-3.0.0/jquery-3.0.0.min.js"></script>
<script src="bootstrap-4.6.0-dist/js/bootstrap.min.js"></script>
<script src="bootstrap-4.6.0-dist/js/bootstrap.bundle.min.js"></script>
<script>
</script>
</body>
</html>

2、 栅格系统
3、 jq22实战
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="bootstrap-4.6.0-dist/css/bootstrap.min.css">
<style>
header,footer{
height: 50px;
background-color: red;
}
footer{
margin-top: 30px;
}
main{
margin-top: 30px;
}
main .item img{
width: 100%;
padding: 5px;
border:1px solid #ccc;
}
main .item:hover{
box-shadow: 0 0 10px #000;
}
</style>
</head>
<body>
<header></header>
<main class="container" id="main">
<div class="row">
<!-- .item 会分配每行的 12列 -->
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid" >
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid">
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid">
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid">
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid" >
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid">
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid">
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid">
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid" >
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid">
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid">
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid">
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid" >
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid">
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid">
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
<div class="item col-xl-3 col-lg-4 col-md-6 col-sm-6 col-6">
<img src="image/id1.jpg" alt="" class="img-fluid">
<p>[蜗牛家具超市]陶瓷艺术花瓶</p>
<p>¥ 999</p>
</div>
</div>
</main>
<footer></footer>
</body>
</html>
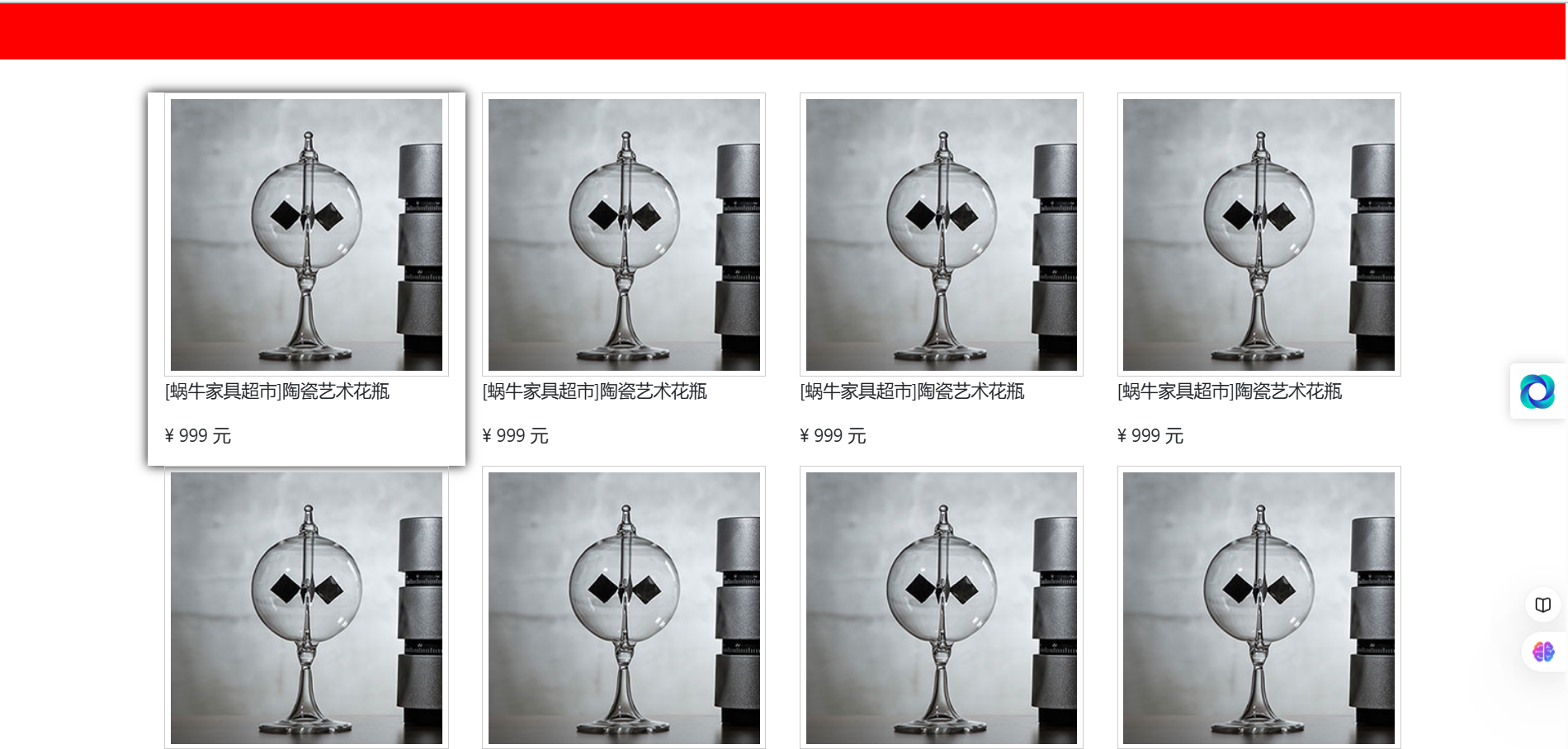
4、 常用无js组件
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="bootstrap-4.6.0-dist/css/bootstrap.min.css">
<style>
body{
padding-left: 40px;
box-sizing: border-box;
}
.login{
width: 400px;
height: 400px;
}
</style>
</head>
<body>
<h2>按钮</h2>
<button type="button" class="btn btn-primary">Primary</button>
<button type="button" class="btn btn-info">Primary</button>
<button type="button" class="btn btn-danger">Primary</button>
<button type="button" class="btn btn-light">Primary</button>
<h2>表单</h2>
<div class="login">
<form>
<div class="form-group">
<label for="exampleInputEmail1">Email address</label>
<input type="email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp">
<small id="emailHelp" class="form-text text-muted">We'll never share your email with anyone else.</small>
</div>
<div class="form-group">
<label for="exampleInputPassword1">Password</label>
<input type="password" class="form-control" id="exampleInputPassword1">
</div>
<div class="form-group form-check">
<input type="checkbox" class="form-check-input" id="exampleCheck1">
<label class="form-check-label" for="exampleCheck1">Check me out</label>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
</div>
<h2>表格</h2>
<table class="table table-striped">
<tr>
<td>007</td>
<td>詹姆斯</td>
<td>男</td>
</tr>
<tr>
<td>007</td>
<td>詹姆斯</td>
<td>男</td>
</tr>
<tr>
<td>007</td>
<td>詹姆斯</td>
<td>男</td>
</tr>
</table>
<h2>卡片</h2>
<div class="card" style="width: 18rem;">
<img src="image/id1.jpg" class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">花瓶1</h5>
<p class="card-text">好看又不贵</p>
<a href="#" class="btn btn-primary">立即购买</a>
</div>
</div>
<h2>警告框</h2>
<div class="alert alert-warning alert-dismissible fade show" role="alert">
<strong>Holy guacamole!</strong> You should check in on some of those fields below.
<button type="button" class="close" data-dismiss="alert" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
</body>
</html>
5、常用js组件
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<link rel="stylesheet" href="bootstrap-4.6.0-dist/css/bootstrap.min.css" />
<style>
#nav{
height: 40px;
line-height: 40px;
}
</style>
</head>
<body>
<h2>标签页</h2>
<ul id="nav" class="nav nav-tabs">
<li class="active">
<a href="#home" data-toggle="tab">
菜鸟教程
</a>
</li>
<li><a href="#ios" data-toggle="tab">iOS</a></li>
</ul>
<div id="myTabContent" class="tab-content">
<div class="tab-pane fade in active show" id="home">
<p>菜鸟教程是一个提供最新的web技术站点,本站免费提供了建站相关的技术文档,帮助广大web技术爱好者快速入门并建立自己的网站。菜鸟先飞早入行------学的不仅是技术,更是梦想。</p>
</div>
<div class="tab-pane fade" id="ios">
<p>iOS 是一个由苹果公司开发和发布的手机操作系统。最初是于 2007 年首次发布 iPhone、iPod Touch 和 Apple
TV。iOS 派生自 OS X,它们共享 Darwin 基础。OS X 操作系统是用在苹果电脑上,iOS 是苹果的移动版本。</p>
</div>
</div>
<h2>模态框</h2>
<!-- Button trigger modal -->
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#demo1">
Launch demo modal
</button>
<h2>下拉菜单</h2>
<div class="dropdown">
<button class="btn btn-secondary dropdown-toggle" type="button" id="dropdownMenuButton" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false">
Dropdown button
</button>
<div class="dropdown-menu" aria-labelledby="dropdownMenuButton">
<a class="dropdown-item" href="#">Action</a>
<a class="dropdown-item" href="#">Another action</a>
<a class="dropdown-item" href="#">Something else here</a>
</div>
</div>
<h2>警告框</h2>
<div class="alert alert-warning alert-dismissible fade show" role="alert">
<strong>Holy guacamole!</strong> You should check in on some of those
fields below.
<button
type="button"
class="close"
data-dismiss="alert"
aria-label="Close"
>
<span aria-hidden="true">×</span>
</button>
</div>
<div class="alert alert-warning alert-dismissible fade show" role="alert">
<strong>Holy guacamole!</strong> You should check in on some of those
fields below.
<button
type="button"
class="close"
data-dismiss="alert"
aria-label="Close"
>
<span aria-hidden="true">×</span>
</button>
</div>
<h2>轮播图</h2>
<div id="carouselExampleIndicators" class="carousel slide" data-ride="carousel">
<ol class="carousel-indicators">
<li data-target="#carouselExampleIndicators" data-slide-to="0" class="active"></li>
<li data-target="#carouselExampleIndicators" data-slide-to="1"></li>
<li data-target="#carouselExampleIndicators" data-slide-to="2"></li>
<li data-target="#carouselExampleIndicators" data-slide-to="3"></li>
<li data-target="#carouselExampleIndicators" data-slide-to="4"></li>
</ol>
<div class="carousel-inner">
<div class="carousel-item active">
<img src="image/banner1.jpg" class="d-block w-100" alt="...">
</div>
<div class="carousel-item">
<img src="image/banner2.jpg" class="d-block w-100" alt="...">
</div>
<div class="carousel-item">
<img src="image/banner3.jpg" class="d-block w-100" alt="...">
</div>
<div class="carousel-item">
<img src="image/banner4.jpg" class="d-block w-100" alt="...">
</div>
<div class="carousel-item">
<img src="image/banner5.jpg" class="d-block w-100" alt="...">
</div>
</div>
<a class="carousel-control-prev" href="#carouselExampleIndicators" role="button" data-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
<span class="sr-only">Previous</span>
</a>
<a class="carousel-control-next" href="#carouselExampleIndicators" role="button" data-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
<span class="sr-only">Next</span>
</a>
</div>
<!-- 模态框 -->
<div class="modal fade" id="demo1" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">登录</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<!-- 表单 -->
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-dismiss="modal">重置</button>
<button type="button" class="btn btn-primary">登录</button>
</div>
</div>
</div>
</div>
<!--所有内容结束 -->
<!-- 保证使用bootstrap的组件能够正常工作 -->
<script src="jquery-3.0.0/jquery-3.0.0.min.js"></script>
<script src="bootstrap-4.6.0-dist/js/bootstrap.bundle.min.js"></script>
<script src="bootstrap-4.6.0-dist/js/bootstrap.min.js"></script>
</body>
</html>
今天的分享就到这里了
🌈本篇博客的内容【网页核心页面设计】已经结束。
🌈若对你有些许帮助,可以点赞、关注、评论支持下博主,你的支持将是我前进路上最大的动力。