游戏规则
玩家点击棋盘下棋(X)。
AI 自动下棋(O)。
当有一方获胜或平局时,高亮显示获胜连线,并显示 2 秒的获胜信息。
2 秒后自动进入下一局游戏。
界面
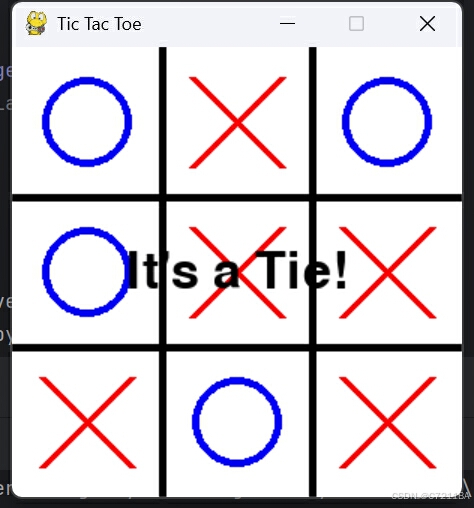
代码
python
import pygame
import sys
import random
import time
# Initialize pygame
pygame.init()
# Define colors
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
BLUE = (0, 0, 255)
# Define screen dimensions
WIDTH = 300
HEIGHT = 300
LINE_WIDTH = 5
CELL_SIZE = WIDTH // 3
# Create game window
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Tic Tac Toe")
# Initialize board
board = [" " for _ in range(9)]
# Define font
font = pygame.font.SysFont(None, 50)
# Draw the board
def draw_board():
screen.fill(WHITE)
for i in range(1, 3):
pygame.draw.line(screen, BLACK, (i * CELL_SIZE, 0), (i * CELL_SIZE, HEIGHT), LINE_WIDTH)
pygame.draw.line(screen, BLACK, (0, i * CELL_SIZE), (WIDTH, i * CELL_SIZE), LINE_WIDTH)
# Draw the pieces
def draw_pieces():
for i in range(9):
row = i // 3
col = i % 3
if board[i] == "X":
pygame.draw.line(screen, RED, (col * CELL_SIZE + 20, row * CELL_SIZE + 20),
((col + 1) * CELL_SIZE - 20, (row + 1) * CELL_SIZE - 20), LINE_WIDTH)
pygame.draw.line(screen, RED, ((col + 1) * CELL_SIZE - 20, row * CELL_SIZE + 20),
(col * CELL_SIZE + 20, (row + 1) * CELL_SIZE - 20), LINE_WIDTH)
elif board[i] == "O":
pygame.draw.circle(screen, BLUE, (col * CELL_SIZE + CELL_SIZE // 2, row * CELL_SIZE + CELL_SIZE // 2),
CELL_SIZE // 2 - 20, LINE_WIDTH)
# Check if a player has won
def check_winner(board, player):
win_conditions = [
[0, 1, 2], [3, 4, 5], [6, 7, 8], # Rows
[0, 3, 6], [1, 4, 7], [2, 5, 8], # Columns
[0, 4, 8], [2, 4, 6] # Diagonals
]
for condition in win_conditions:
if all(board[i] == player for i in condition):
return condition # Return the winning positions
return None
# Check if the board is full
def is_board_full(board):
return " " not in board
# AI move
def ai_move(board):
# Check if AI can win
for i in range(9):
if board[i] == " ":
board[i] = "O"
if check_winner(board, "O"):
return i
board[i] = " "
# Check if player can win and block
for i in range(9):
if board[i] == " ":
board[i] = "X"
if check_winner(board, "X"):
board[i] = "O"
return i
board[i] = " "
# Prefer the center position
if board[4] == " ":
return 4
# Choose a random available move
available_moves = [i for i, cell in enumerate(board) if cell == " "]
return random.choice(available_moves)
# Highlight the winning line
def highlight_winning_line(winning_positions):
for pos in winning_positions:
row = pos // 3
col = pos % 3
center_x = col * CELL_SIZE + CELL_SIZE // 2
center_y = row * CELL_SIZE + CELL_SIZE // 2
pygame.draw.circle(screen, (0, 255, 0), (center_x, center_y), 10)
# Show game over message
def show_game_over_message(winner):
if winner == "X":
message = "You Win!"
elif winner == "O":
message = "AI Wins!"
else:
message = "It's a Tie!"
text = font.render(message, True, BLACK)
screen.blit(text, (WIDTH // 2 - text.get_width() // 2, HEIGHT // 2 - text.get_height() // 2))
pygame.display.update()
time.sleep(2) # Display the message for 2 seconds
# Main game loop
def main():
global board
board = [" " for _ in range(9)]
current_player = "X" # Player starts first
game_over = False
winner = None
winning_positions = None
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
if not game_over and event.type == pygame.MOUSEBUTTONDOWN and current_player == "X":
x, y = pygame.mouse.get_pos()
row = y // CELL_SIZE
col = x // CELL_SIZE
index = row * 3 + col
if board[index] == " ":
board[index] = "X"
winning_positions = check_winner(board, "X")
if winning_positions:
winner = "X"
game_over = True
elif is_board_full(board):
winner = ""
game_over = True
else:
current_player = "O"
if not game_over and current_player == "O":
move = ai_move(board)
board[move] = "O"
winning_positions = check_winner(board, "O")
if winning_positions:
winner = "O"
game_over = True
elif is_board_full(board):
winner = ""
game_over = True
else:
current_player = "X"
draw_board()
draw_pieces()
if winning_positions:
highlight_winning_line(winning_positions)
pygame.display.update()
if game_over:
show_game_over_message(winner)
main() # Automatically start a new game
# Start the game
if __name__ == "__main__":
main()