- 字符串
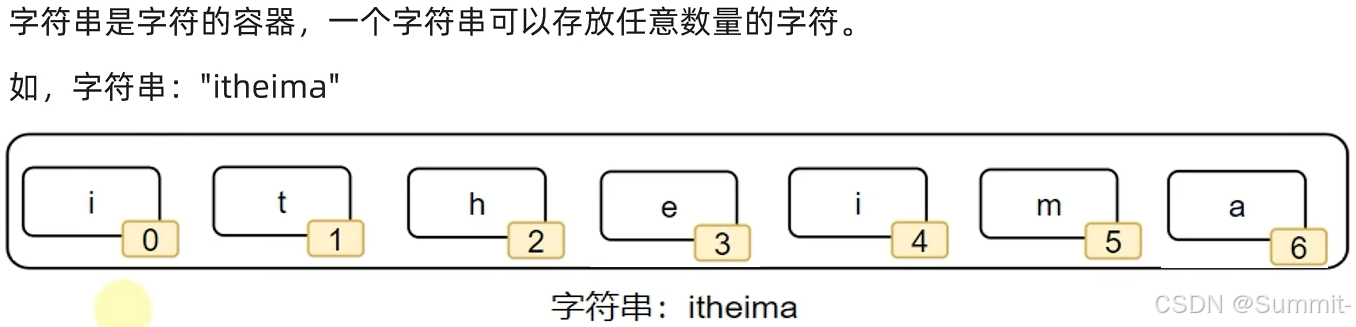
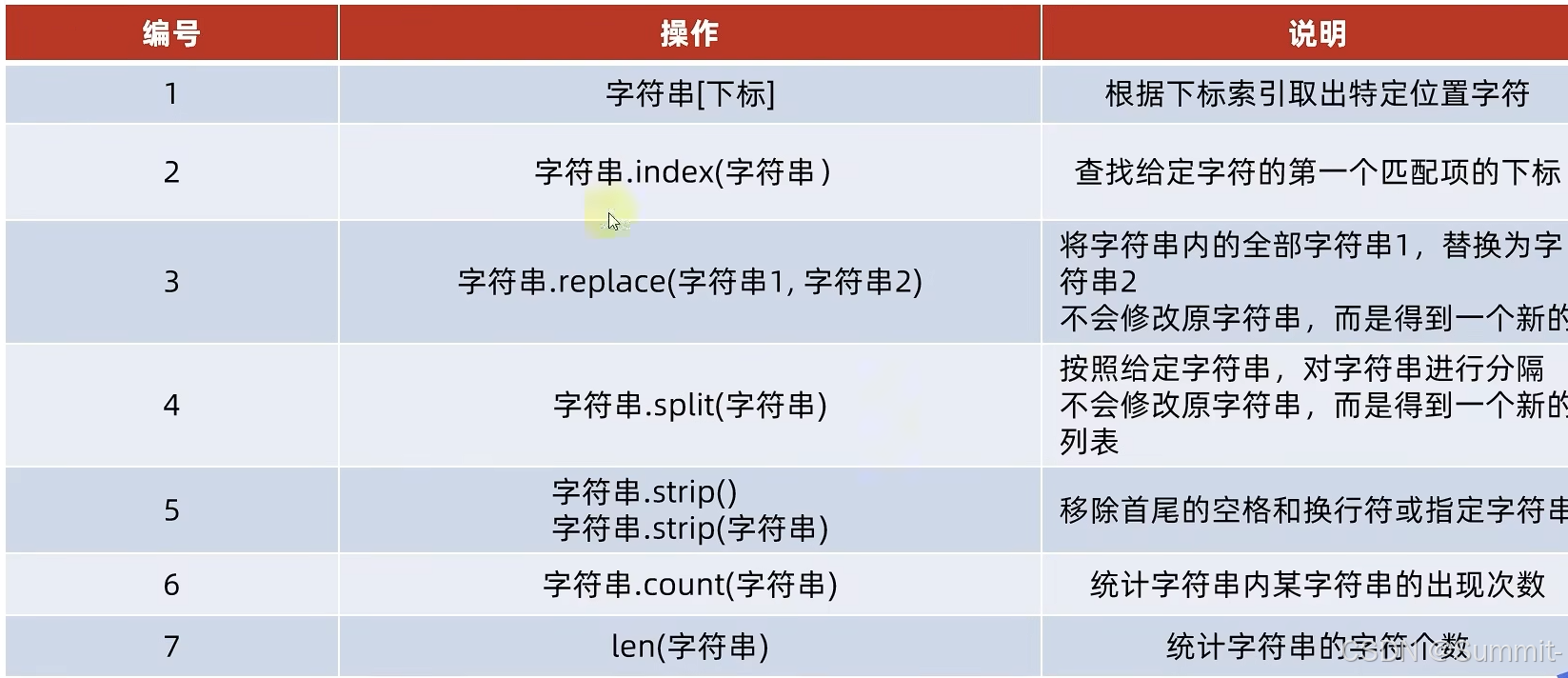
(1) 字符串能通过下标索引来获取其中的元素
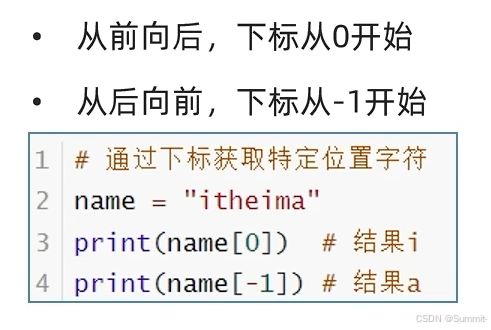
(2) 旧字符串无法修改特定下标的元素
(3) index------查找字符串中任意元素在整个字符串中的起始位置(单个字符或字符串都可以)
python
tmp_str = "supercarrydoinb"
tmp_position1 = tmp_str.index("s")
tmp_position2 = tmp_str.index("doinb")
print(f"{tmp_position1}, {tmp_position2}")
(4) replace------将原字符串中部分字符串1改为字符串2,生成一个新的字符串,不修改原字符串
python
tmp_str = "supercarrydoinb"
tmp_str1 = tmp_str.replace("doinb", "666")
print(f"{tmp_str1}")
(5) split------将字符串通过字符串中的子分割字符串分割成多个字符串存储到列表中,返回列表名
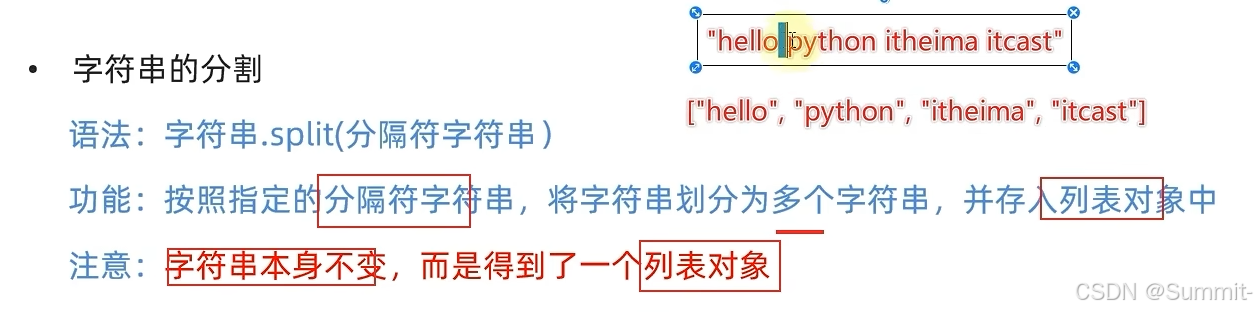
python
tmp_str = "super carry doinb"
tmp_str1 = tmp_str.split(" ")
print(f"{tmp_str1}")
(6) strip
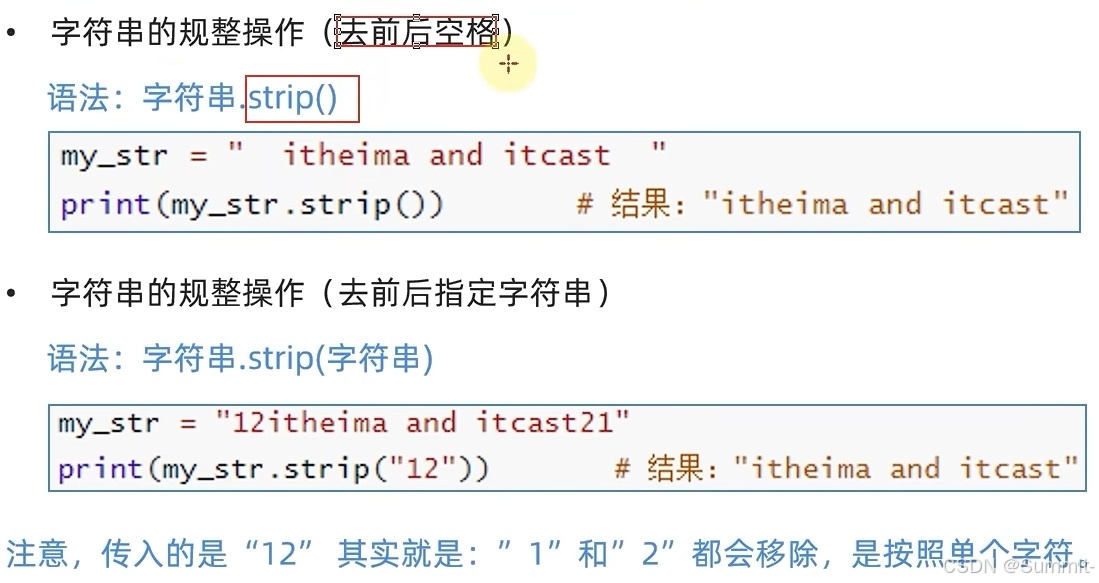
- 字符串.strip()
去除字符串前后的空格或回车符,将结果返回给新的字符串
python
tmp_str = (" super carry doinb ")
tmp_str1 = tmp_str.strip()
print(f"{tmp_str1}")
- 字符串.strip(字符串1)
去除字符串前后的字符串1,将结果返回给新的字符串
python
tmp_str = ("qwesuper carry doinbewq")
tmp_str1 = tmp_str.strip("qwe")
print(f"{tmp_str1}")
注意:对于strip中加参数,比如参数是ab,是分别去除a和b,而不是直接去除ab,非整体去除
(7) count------计算字符串中某个字符或部分字符串在整个字符串中的个数
python
tmp_str = (" super carry doinb ")
tmp_cnt = tmp_str.count("do")
print(f"{tmp_cnt}")
(8) len------计算字符串中字符的个数
python
tmp_str = ("supercarrydoinb")
tmp_len = len(tmp_str)
print(f"{tmp_len}")
(9) while循环
python
tmp_str = ("supercarrydoinb")
tmp_cnt = 0
while tmp_cnt < len(tmp_str):
print(f"{tmp_str[tmp_cnt]}")
tmp_cnt += 1
(10) for循环
python
tmp_str = ("supercarrydoinb")
for i in tmp_str:
print(f"{i}")
(11) 字符串特点
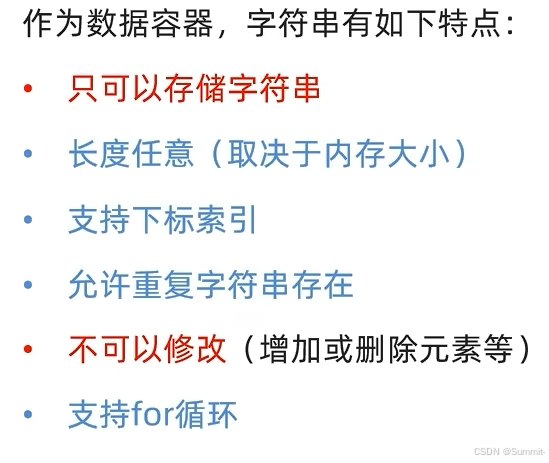
字符串只能存储字符类型,且不可被修改
(12) 练习
python
tmp_str = ("super carry doinb")
tmp_count = tmp_str.count("super")
print(f"{tmp_count}")
tmp_str1 = tmp_str.replace(" ", "|")
print(f"{tmp_str1}")
tmp_str2 = tmp_str1.split("|")
print(f"{tmp_str2}")
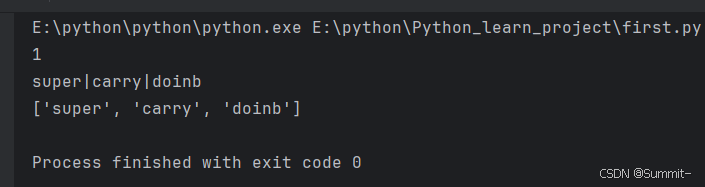