目录
[1. 方法语法概述](#1. 方法语法概述)
[2. 方法与函数的区别](#2. 方法与函数的区别)
[2.1 示例:定义方法](#2.1 示例:定义方法)
[3. 方法的参数](#3. 方法的参数)
[3.1 示例:带参数的方法](#3.1 示例:带参数的方法)
[4. 关联函数](#4. 关联函数)
[4.1 示例:关联函数](#4.1 示例:关联函数)
[5. 多个impl块](#5. 多个impl块)
[5.1 示例](#5.1 示例)
思维导图
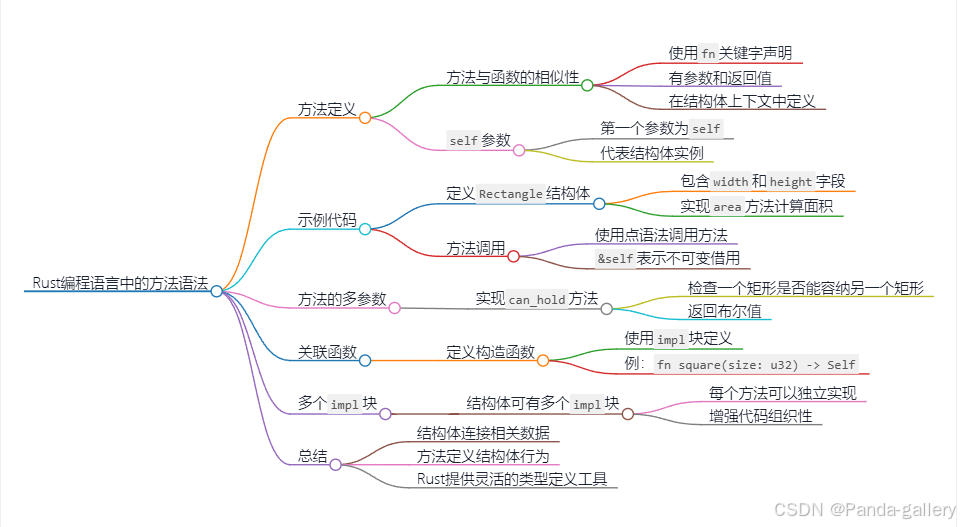
1. 方法语法概述
-
方法是定义在结构体、枚举或特征对象(trait object)中的函数,与特定类型实例相关联。
-
方法的第一个参数总是self,表示方法被调用的实例。self可以是以下三种形式之一:
-
&self:不可变借用,表示方法不会修改实例。
-
&mut self:可变借用,表示方法可能会修改实例。
-
self:获取所有权,表示方法会消耗实例。
- 方法通过impl(实现)块定义,所有在该块内的内容都与指定的类型相关联。
2. 方法与函数的区别
-
方法 总是与某个类型实例相关联,调用语法为实例.方法名(参数)。
-
函数 是独立的,调用语法为函数名(参数)。
2.1 示例:定义方法
以下示例展示了如何在Rectangle
结构体上定义area
方法,计算矩形的面积。
rust
#[derive(Debug)]
struct Rectangle {
width: u32,
height: u32,
}
impl Rectangle {
// 定义方法:计算面积
fn area(&self) -> u32 {
self.width * self.height
}
}
fn main() {
let rect = Rectangle {
width: 30,
height: 50,
};
println!("The area of the rectangle is {} square pixels.", rect.area());
}
输出结果:
rust
The area of the rectangle is 1500 square pixels.
3. 方法的参数
-
方法可以有多个参数,除了第一个self参数外,其他参数与函数的参数定义方式相同。
-
以下示例定义了can_hold方法,用于判断一个矩形是否可以容纳另一个矩形。
3.1 示例:带参数的方法
rust
#[derive(Debug)]
struct Rectangle {
width: u32,
height: u32,
}
impl Rectangle {
// 定义方法:判断当前矩形是否可以容纳另一个矩形
fn can_hold(&self, other: &Rectangle) -> bool {
self.width > other.width && self.height > other.height
}
}
fn main() {
let rect1 = Rectangle {
width: 30,
height: 50,
};
let rect2 = Rectangle {
width: 20,
height: 40,
};
println!("Can rect1 hold rect2? {}", rect1.can_hold(&rect2));
}
输出结果:
rust
Can rect1 hold rect2? true
4. 关联函数
-
关联函数 是定义在impl块内但不需要self参数的函数,通常用于构造函数。
-
关联函数通过类型名::函数名的方式调用。
4.1 示例:关联函数
rust
#[derive(Debug)]
struct Rectangle {
width: u32,
height: u32,
}
impl Rectangle {
// 定义关联函数:创建一个正方形矩形
fn square(size: u32) -> Self {
Self {
width: size,
height: size,
}
}
}
fn main() {
let square = Rectangle::square(10);
println!("Square: {:?}", square);
}
输出结果:
rust
Square: Rectangle { width: 10, height: 10 }
5. 多个impl块
- Rust允许为同一结构体定义多个impl块,这在某些情况下是有用的,例如为不同的功能组织代码。
5.1 示例:多个impl块
rust
#[derive(Debug)]
struct Rectangle {
width: u32,
height: u32,
}
impl Rectangle {
// 定义方法:计算面积
fn area(&self) -> u32 {
self.width * self.height
}
}
impl Rectangle {
// 定义方法:判断当前矩形是否可以容纳另一个矩形
fn can_hold(&self, other: &Rectangle) -> bool {
self.width > other.width && self.height > other.height
}
}
fn main() {
let rect1 = Rectangle {
width: 30,
height: 50,
};
let rect2 = Rectangle {
width: 20,
height: 40,
};
println!("Area of rect1: {}", rect1.area());
println!("Can rect1 hold rect2? {}", rect1.can_hold(&rect2));
}
输出结果:
rust
Area of rect1: 1500
Can rect1 hold rect2? true
tips:
- 方法第一个参数总是 self ,可选 &self 、&mut self 、self;
- 方法通过 impl 块定义,调用语法为 实例.方法名(参数);
- 关联函数不含 self 参数,调用语法为 类型名::函数名;
- Rust允许为同一结构体定义多个 impl
块。