1、下载安装
pip install imgkit
pip install pdfkit
2、wkhtmltopdf工具包,下载安装
下载地址:https://wkhtmltopdf.org/downloads.html
3、生成图片
import imgkit
path_wkimg = r'D:\app\wkhtmltopdf\bin\wkhtmltoimage.exe' # 工具路径,安装的路径
cfg = imgkit.config(wkhtmltoimage=path_wkimg)
# 1、将html文件转为图片
options = {'encoding': 'utf8'} # 解决乱码
imgkit.from_file(r'temp.html', 'helloworld.jpg', config=cfg, options=options)
# 2、从url获取html,再转为图片
# imgkit.from_url('https://httpbin.org/ip', 'ip.jpg', config=cfg, options=options)
# 3、将字符串转为图片
# imgkit.from_string('Hello!', 'hello.jpg', config=cfg)
4、转为pdf
import pdfkit
path_wkpdf = r'D:\app\wkhtmltopdf\bin\wkhtmltopdf.exe' # 工具路径
cfg = pdfkit.configuration(wkhtmltopdf=path_wkpdf)
# 1、将html文件转为pdf
pdfkit.from_file(r'./helloworld.html', 'helloworld.pdf', configuration=cfg)
# 传入列表
pdfkit.from_file([r'./helloworld.html', r'./111.html', r'./222.html'], 'helloworld.pdf', configuration=cfg)
# 2、从url获取html,再转为pdf
pdfkit.from_url('https://httpbin.org/ip', 'ip.pdf', configuration=cfg)
# 传入列表
pdfkit.from_url(['https://httpbin.org/ip','https://httpbin.org/ip'], 'ip.pdf', configuration=cfg)
# 3、将字符串转为pdf
pdfkit.from_string('Hello!','hello.pdf', configuration=cfg)
5、a4纸张大小
import imgkit
path_wkimg = r'D:\app\wkhtmltopdf\bin\wkhtmltoimage.exe' # 工具路径
cfg = imgkit.config(wkhtmltoimage=path_wkimg)
# # 1、将html文件转为图片
# A4纸张大小
# options = {
# 'encoding': 'utf8', # 解决乱码
# 'width': 599,
# 'height': 845
# }
options = {
'encoding': 'utf8',
'width': 599,
# 'height': 845
}
imgkit.from_file(r'temp.html', 'helloworld.jpg', config=cfg, options=options)
<!DOCTYPE html>
<html>
<head>
<title>打印</title>
</head>
<body style="margin: 5px">
<!--<div style="width: 599px;height: 845px">-->
<div style="width: 599px;height: 100%">
<div>大得</div>
<div>大得</div>
</div>
</body>
</html>
5、a4纸生成图片
import imgkit
path_wkimg = r'D:\app\wkhtmltopdf\bin\wkhtmltoimage.exe' # 工具路径
cfg = imgkit.config(wkhtmltoimage=path_wkimg)
# # 1、将html文件转为图片
# A4纸张大小
# options = {
# 'encoding': 'utf8', # 解决乱码
# 'width': 599,
# 'height': 845
# }
options = {
'encoding': 'utf8',
'width': 599,
'height': 845
}
imgkit.from_file(r'temp.html', 'helloworld.jpg', config=cfg, options=options)
<!DOCTYPE html>
<html>
<head>
<title>打印</title>
<style>
body {
margin: 5px;
}
.container {
width: 599px;
height: 100%;
}
.row {
height: 300px;
}
.row div {
/* 允许在单词内部换行,确保长字符串能正常换行 */
word-wrap: break-word;
word-break: break-all;
}
</style>
</head>
<body>
<div class="container">
<div class="row">
<div>大得6666666666669999999999999999999999999999999999999999999999999999999999999999999999977777777777777778888888888888</div>
<div>大得</div>
</div>
<div class="row">
<div>大得</div>
<div>大得</div>
</div>
</div>
</body>
</html>
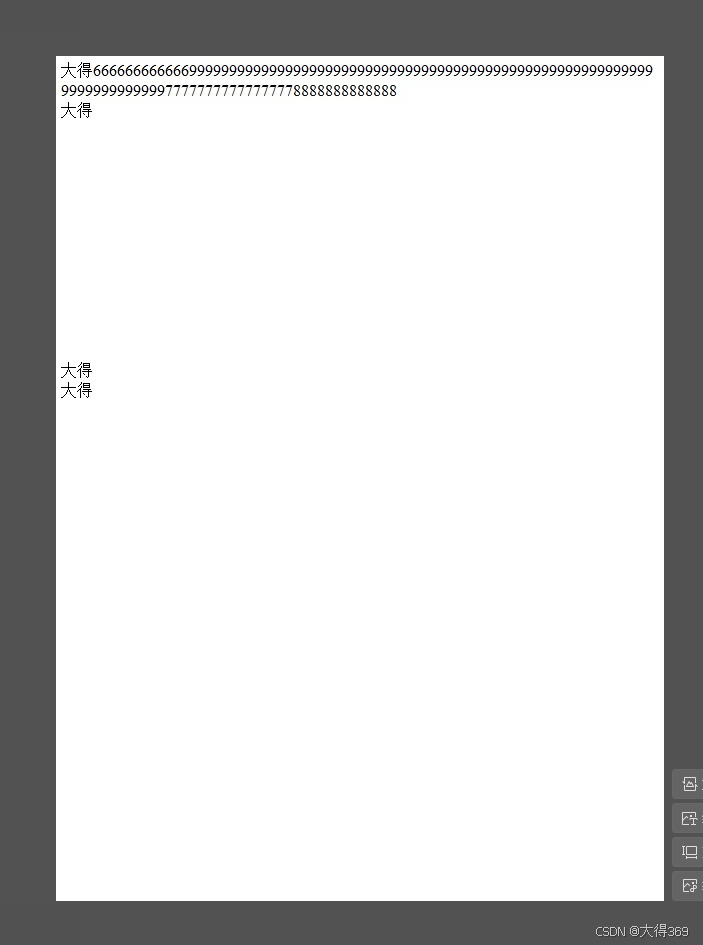
6、模糊问题,使用quality,zoom解决
import imgkit
path_wkimg = r'D:\app\wkhtmltopdf\bin\wkhtmltoimage.exe' # 工具路径
cfg = imgkit.config(wkhtmltoimage=path_wkimg)
# # 1、将html文件转为图片
# A4纸张大小
options = {
'encoding': 'utf8', # 乱码
'width': 599,
'enable-local-file-access': '', # 允许访问本地文件
'quality': 100, # 提高分辨率,100最高
'zoom': 4 # 增加缩放比例
}
imgkit.from_file(r'temp.html', 'helloworld.jpg', config=cfg, options=options)
<!DOCTYPE html>
<html>
<head>
<title>打印</title>
</head>
<body style="margin: 5px">
<div style="width: 599px;height: 100%">
<div style="height: 300px;">
<div>大得666666666666999999999999999999999</div>
<div>大得</div>
</div>
<div style="height: 300px;">
<div>大得</div>
<div>大得</div>
</div>
</div>
</body>
</html>