1.h5端
<template>
<view class="container">
<view id="flipbook">
<view class="page page1">Page 1</view>
<view class="page page2">Page 2</view>
<view class="page page3">Page 3</view>
<view class="page page4">Page 4</view>
<view class="page fixed">Page 5</view>
</view>
</view>
</template>
<script>
//npm install jquery turn.js
import $ from 'jquery';
import 'turn.js';
export default {
data() {
return {
}
},
mounted() {
// 确保 DOM 加载完成
this.$nextTick(() => {
// 初始化翻书效果
$('#flipbook').turn({
width: 400, // 书本宽度
height: 300, // 书本高度
autoCenter: true, // 自动居中
display: 'single',
});
});
},
methods: {
}
}
</script>
<style>
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
#flipbook {
width: 400px;
height: 300px;
background-color: #f0f0f0;
}
.page {
width: 100%;
height: 100%;
display: flex;
justify-content: center;
align-items: center;
background-color: #fff;
border: 1px solid #ccc;
box-sizing: border-box;
font-size: 20px;
color: #333;
}
</style>
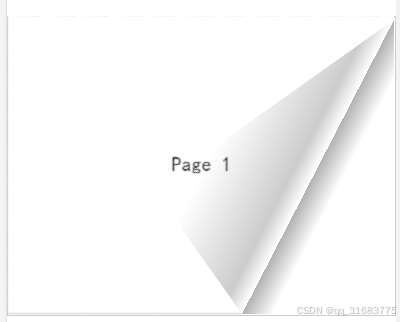
2.APP 端简单使用
<template>
<view class="container">
<!-- 使用 renderjs 的容器 -->
<view id="flipbook" :change:prop="renderjs.initTurnjs" :prop="{ pages: 4 }">
<view class="page page1">Page 16+66</view>
<view class="page page2">Page 277</view>
<view class="page page3">Page 388</view>
<view class="page page4">Page 4</view>
</view>
</view>
</template>
<script module="renderjs" lang="renderjs">
export default {
mounted() {
// 在 renderjs 中初始化 turn.js
//this.initTurnjs();
},
methods: {
initTurnjs() {
// 动态加载 jQuery 和 turn.js
const loadScript = (src, callback) => {
const script = document.createElement('script');
script.src = src;
script.onload = callback;
document.head.appendChild(script);
};
console.log(2222);
// 加载 jQuery
loadScript('http://192.168.1.95:9300/statics/jquery-3.5.1.min.js', () => {
// 加载 turn.js
console.log(333);
loadScript('http://192.168.1.95:9300/statics/turn.min.js', () => {
// 初始化 turn.js
console.log(444);
$('#flipbook').turn({
width: 300,
height: 300,
autoCenter: true,
display: 'single',
pages: 4,
when: {
turned: function(event, page, view) {
console.log('Turned to page', page);
// 在这里可以添加加载新页面的逻辑
}
}
});
});
});
}
}
}
</script>
<style>
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
#flipbook {
width: 300px;
height: 300px;
background-color: #f0f0f0;
}
.page {
width: 100%;
height: 100%;
display: flex;
justify-content: center;
align-items: center;
background-color: #fff;
border: 1px solid #ccc;
box-sizing: border-box;
font-size: 20px;
color: #333;
}
</style>
3.app端 添加页
<template>
<view >
<view id="onePage" v-show="false">
<view class="content">
<view class="title">
{{content.title}}
</view>
<view class="details">
{{content.details}}
</view>
</view>
</view>
<button @click="add">添加一页</button>
<view class="container">
<!-- 使用 renderjs 的容器 -->
<view id="flipbook" :change:listSize="renderjs.initTurnjs" :listSize="listSize" :addEventRandom="addEventRandom" :change:addEventRandom="renderjs.addEvent">
<view class="page">欢迎</view>
</view>
</view>
</view>
</template>
<script>
import Vue from 'vue'
export default {
components: {
},
data() {
return {
addEventRandom:null,
content:{
title:0,
details:'未初始化'
},
listSize:1,
currentSize:1
}
},
created() {
},
onLoad(option) {
},
onShow() {
},
methods: {
add(){
//本次添加几页(总页数要是偶数,否则翻到最后一页不能向前翻)
var addnum=0;
if(this.currentSize % 2 === 0){
//偶数
addnum = 2;
this.currentSize = this.currentSize + 2;
}else{
//奇数
addnum = 1;
this.currentSize = this.currentSize + 1;
}
let count = 0;
const intervalId = setInterval(() => {
++count;
//填充数据
this.content.title = '标题'+Math.floor(1000 + Math.random() * 9000);
this.content.details = '内容'+Math.floor(1000 + Math.random() * 9000);
//通知加一页
this.addEventRandom = Math.floor(1000 + Math.random() * 9000);
if (count === addnum) {
clearInterval(intervalId); // 停止定时器
}
}, 1000);
}
}
}
</script>
<script module="renderjs" lang="renderjs">
export default {
data() {
return {
turn:null,
pages:1
}
},
mounted() {
// 在 renderjs 中初始化 turn.js
//this.initTurnjs();
},
methods: {
initTurnjs(newValue, oldValue) {
var that=this;
this.pages = newValue;
// 动态加载 jQuery 和 turn.js
const loadScript = (src, callback) => {
const script = document.createElement('script');
script.src = src;
script.onload = callback;
document.head.appendChild(script);
};
// 加载 jQuery
loadScript('http://192.168.1.95:9300/statics/jquery-3.5.1.min.js', () => {
// 加载 turn.js
loadScript('http://192.168.1.95:9300/statics/turn.min.js', () => {
// 初始化 turn.js
that.turn = $('#flipbook').turn({
width: 300,
height: 300,
autoCenter: true,
display: 'single',
acceleration:true,
pages: this.pages,
when: {
turned: function(event, page, view) {
console.log('Turned to page', page);
},
first(){
//console.log('当前页面是第一页');
},
last(){
//console.log('当前页面是最后页');
}
}
});
//console.log(that.turn);
});
});
},
addEvent(newValue, oldValue){
//调试
//console.log(888);
//console.log($('#onePage').html());
const newPage = $('<div>').addClass('page').html($('#onePage').html());
this.turn.turn('addPage', newPage, this.pages + 1); // 添加到末尾
this.pages = this.pages + 1
$("#flipbook").turn("pages", this.pages);
//调试
//console.log($('#flipbook').html());
}
}
}
</script>
<style>
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
#flipbook {
width: 300px;
height: 300px;
background-color: #f0f0f0;
}
.page {
width: 100%;
height: 100%;
display: flex;
justify-content: center;
align-items: center;
background-color: #fff;
border: 1px solid #ccc;
box-sizing: border-box;
font-size: 20px;
color: #333;
}
</style>
两个js文件我也上传百度云盘作为备份
通过网盘分享的文件:uniapp使用turn.js
链接: https://pan.baidu.com/s/199ncUbcdcKaPNK9p-hnoRQ?pwd=hikp 提取码: hikp
jquery.js 是在网上找的
turn.js是在github下载的
Turn.js: The page flip effect in HTML5
GitHub - blasten/turn.js: The page flip effect for HTML5
app端的效果视频
通过网盘分享的文件:6542f4649d16ffe6b78bdf194547af58.mp4
链接: https://pan.baidu.com/s/1LGAGEm7KpibZ2QJTAYczZg?pwd=n37g 提取码: n37g