题目描述:
给你一个 m
行 n
列的矩阵 matrix
,请按照 顺时针螺旋顺序 ,返回矩阵中的所有元素。
示例 1:
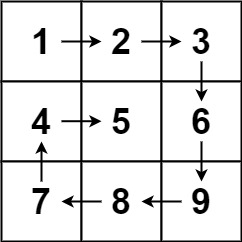
输入:matrix = [[1,2,3],[4,5,6],[7,8,9]]
输出:[1,2,3,6,9,8,7,4,5]
逻辑:
沿着某一边不断访问数组元素,每访问结束一边,就将代表该条边的变量往中心靠拢一次。当访问最底边或者最左边时,进行判断,是否发生触底事件。
cpp
class Solution {
public:
vector<int> spiralOrder(vector<vector<int>>& matrix) {
vector<int> result;
if (matrix.empty()) return result;
int top = 0, bottom = matrix.size() - 1;
int left = 0, right = matrix[0].size() - 1;
while (top <= bottom && left <= right) {
// Traverse from left to right along the top row
for (int i = left; i <= right; ++i) {
result.push_back(matrix[top][i]);
}
++top; // Move down to the next row
// Traverse from top to bottom along the right column
for (int i = top; i <= bottom; ++i) {
result.push_back(matrix[i][right]);
}
--right; // Move left to the next column
if (top <= bottom) {
// Traverse from right to left along the bottom row
for (int i = right; i >= left; --i) {
result.push_back(matrix[bottom][i]);
}
--bottom; // Move up to the previous row
}
if (left <= right) {
// Traverse from bottom to top along the left column
for (int i = bottom; i >= top; --i) {
result.push_back(matrix[i][left]);
}
++left; // Move right to the next column
}
}
return result;
}
};