昨天工作耽搁了,没来得及打卡每日一题,今日补上:
标题:Length of Longest Fibonacci Subsequence
题目:
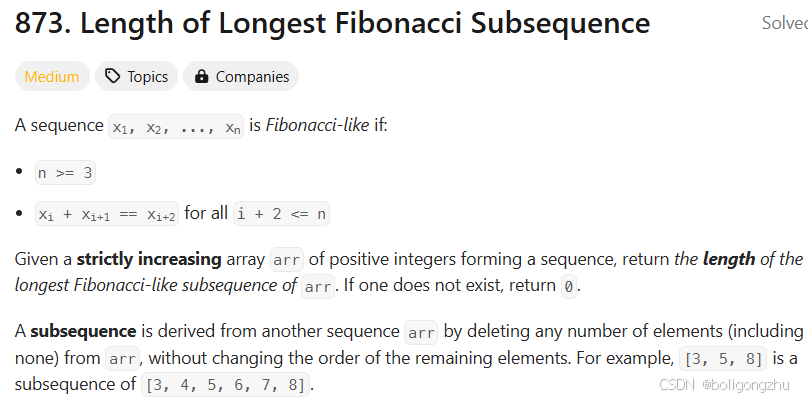
例子:
Example 1:
Input: arr = [1,2,3,4,5,6,7,8]
Output: 5
Explanation: The longest subsequence that is fibonacci-like: [1,2,3,5,8].
Example 2:
Input: arr = [1,3,7,11,12,14,18]
Output: 3
Explanation: The longest subsequence that is fibonacci-like: [1,11,12], [3,11,14] or [7,11,18].

解题思路:
- 先看这道题数量级是10的3次方,因此时间复杂度能接受O(n^2),但不能接受O(n^3)。
- 确定解题用到的算法及数据结构:要求最长子序列的长度,只要确定子序列最前两个数字,后面的数字都是确定的,因此两层循环确定最前面两个数字,查看整个序列有多少个数字包含在序列中即可。查看的过程可以用unordered_set,查询时间复杂度为O(1)。
代码:
cpp
class Solution {
public:
int lenLongestFibSubseq(vector<int>& arr) {
unordered_set<int> set(arr.begin(), arr.end());
int res = 0;
for(int i = 0; i < arr.size() - 2; i++){
for(int j = i+1; j < arr.size() - 1; j ++){
int len = 2, start = arr[i], second = arr[j], next = arr[i] + arr[j];
while(next <= arr.back() && set.count(next)) {
len ++;
start = second;
second = next;
next = start + second;
}
if (len > 2) res = max(res, len);
}
}
return res;
}
};
O(N^2)<时间复杂度<O(N^3)
空间复杂度为O(N)