2d刻度尺 : vtk 2D 刻度尺 2D 比例尺-CSDN博客
简介:
3D 刻度尺,也是常用功能,功能强大 3D 刻度尺 CubeAxesActor
vtkCubeAxes调整坐标轴的刻度、原点和显示效果,包括关闭小标尺、固定坐标轴原点,以及设置FlyMode模式。同时,展示了通过vtkOutlineFilter创建立体包围盒的方法,提供了一种只显示XYZ坐标轴和包围盒的解决方案。最后提到了vtkCamera的用法作为后续探讨的话题 ;
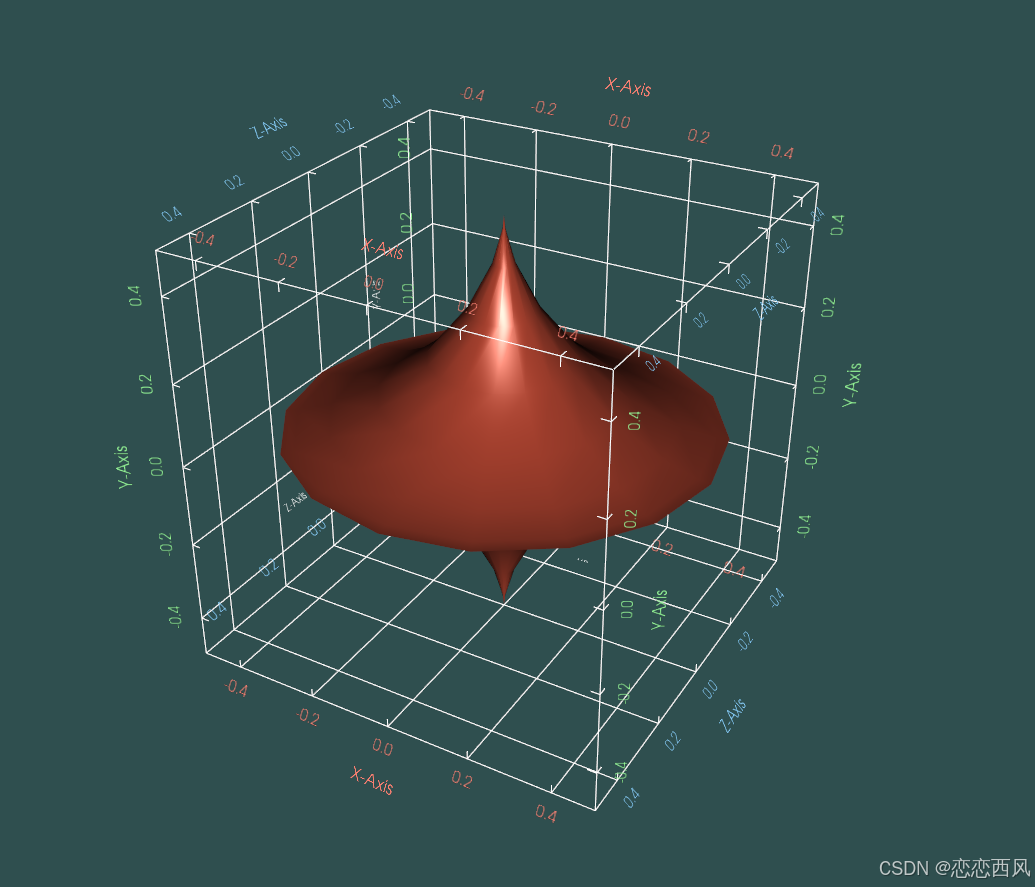
Part2:简单实现:
#!/usr/bin/env python
# noinspection PyUnresolvedReferences
import vtkmodules.vtkInteractionStyle
# noinspection PyUnresolvedReferences
import vtkmodules.vtkRenderingOpenGL2
from vtkmodules.vtkCommonColor import vtkNamedColors
from vtkmodules.vtkFiltersSources import vtkSuperquadricSource
from vtkmodules.vtkRenderingAnnotation import vtkCubeAxesActor
from vtkmodules.vtkRenderingCore import (
vtkActor,
vtkPolyDataMapper,
vtkRenderWindow,
vtkRenderWindowInteractor,
vtkRenderer
)
def main():
colors = vtkNamedColors()
backgroundColor = colors.GetColor3d("DarkSlateGray")
actorColor = colors.GetColor3d("Tomato")
axis1Color = colors.GetColor3d("Salmon")
axis2Color = colors.GetColor3d("PaleGreen")
axis3Color = colors.GetColor3d("LightSkyBlue")
# Create a superquadric
superquadricSource = vtkSuperquadricSource()
superquadricSource.SetPhiRoundness(3.1)
superquadricSource.SetThetaRoundness(1.0)
superquadricSource.Update() # needed to GetBounds later
renderer = vtkRenderer()
mapper = vtkPolyDataMapper()
mapper.SetInputConnection(superquadricSource.GetOutputPort())
superquadricActor = vtkActor()
superquadricActor.SetMapper(mapper)
superquadricActor.GetProperty().SetDiffuseColor(actorColor)
superquadricActor.GetProperty().SetDiffuse(.7)
superquadricActor.GetProperty().SetSpecular(.7)
superquadricActor.GetProperty().SetSpecularPower(50.0)
cubeAxesActor = vtkCubeAxesActor()
cubeAxesActor.SetUseTextActor3D(1)
cubeAxesActor.SetBounds(superquadricSource.GetOutput().GetBounds())
cubeAxesActor.SetCamera(renderer.GetActiveCamera())
cubeAxesActor.GetTitleTextProperty(0).SetColor(axis1Color)
cubeAxesActor.GetTitleTextProperty(0).SetFontSize(48)
cubeAxesActor.GetLabelTextProperty(0).SetColor(axis1Color)
cubeAxesActor.GetTitleTextProperty(1).SetColor(axis2Color)
cubeAxesActor.GetLabelTextProperty(1).SetColor(axis2Color)
cubeAxesActor.GetTitleTextProperty(2).SetColor(axis3Color)
cubeAxesActor.GetLabelTextProperty(2).SetColor(axis3Color)
cubeAxesActor.DrawXGridlinesOn()
cubeAxesActor.DrawYGridlinesOn()
cubeAxesActor.DrawZGridlinesOn()
cubeAxesActor.SetGridLineLocation(cubeAxesActor.VTK_GRID_LINES_FURTHEST)
cubeAxesActor.XAxisMinorTickVisibilityOff()
cubeAxesActor.YAxisMinorTickVisibilityOff()
cubeAxesActor.ZAxisMinorTickVisibilityOff()
cubeAxesActor.SetFlyModeToStaticEdges()
renderer.AddActor(cubeAxesActor)
renderer.AddActor(superquadricActor)
renderer.GetActiveCamera().Azimuth(30)
renderer.GetActiveCamera().Elevation(30)
renderer.ResetCamera()
renderer.SetBackground(backgroundColor)
renderWindow = vtkRenderWindow()
renderWindow.AddRenderer(renderer)
renderWindow.SetSize(640, 480)
renderWindow.SetWindowName('CubeAxesActor')
renderWindowInteractor = vtkRenderWindowInteractor()
renderWindowInteractor.SetRenderWindow(renderWindow)
renderWindow.Render()
renderer.GetActiveCamera().Zoom(0.8)
renderWindowInteractor.Start()
if __name__ == '__main__':
main()
Part3: 三维图表上相应的坐标,在VTK中提供了相应的类vtkCubeAxes和vtkCubeAxes2D
vtkCubeAxesActor2D在数据集的边界框上绘制轴,并用x-y-z坐标标记轴。
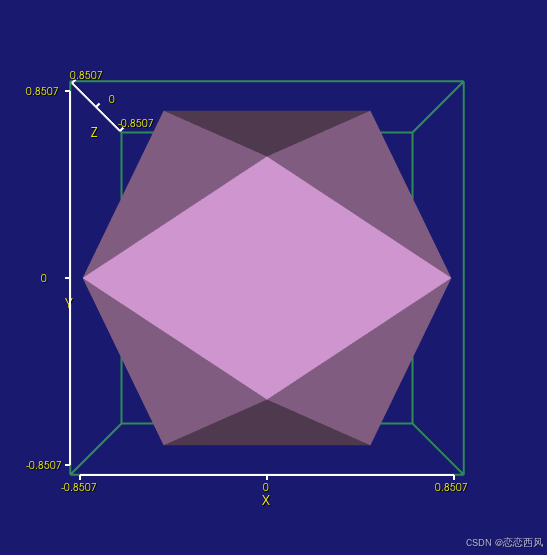
Part4 实现:
cpp
import vtk
colors = vtk.vtkNamedColors()
icosahedron = vtk.vtkPlatonicSolidSource()
icosahedron.SetSolidTypeToIcosahedron()
normals = vtk.vtkPolyDataNormals()
normals.SetInputConnection(icosahedron.GetOutputPort())
icosahedron_mapper = vtk.vtkPolyDataMapper()
icosahedron_mapper.SetInputConnection(normals.GetOutputPort())
icosahedron_mapper.ScalarVisibilityOff()
icosahedron_actor = vtk.vtkLODActor()
icosahedron_actor.SetMapper(icosahedron_mapper)
icosahedron_actor.GetProperty().SetColor(colors.GetColor3d("Plum"))
outline = vtk.vtkOutlineFilter()
outline.SetInputConnection(normals.GetOutputPort())
map_outline = vtk.vtkPolyDataMapper()
map_outline.SetInputConnection(outline.GetOutputPort())
outline_actor = vtk.vtkActor()
outline_actor.SetMapper(map_outline)
outline_actor.GetProperty().SetColor(colors.GetColor3d("SeaGreen"))
outline_actor.GetProperty().SetLineWidth(2)
ren1 = vtk.vtkRenderer()
ren1.SetViewport(0, 0, 0.5, 1.0)
ren2 = vtk.vtkRenderer()
ren2.SetViewport(0.5, 0, 1.0, 1.0)
ren2.SetActiveCamera(ren1.GetActiveCamera())
renWin = vtk.vtkRenderWindow()
renWin.AddRenderer(ren1)
renWin.AddRenderer(ren2)
renWin.SetWindowName("CubeAxesActor2D")
renWin.SetSize(1200, 600)
iren = vtk.vtkRenderWindowInteractor()
iren.SetRenderWindow(renWin)
style = vtk.vtkInteractorStyleTrackballCamera()
iren.SetInteractorStyle(style)
ren1.AddViewProp(icosahedron_actor)
ren1.AddViewProp(outline_actor)
ren2.AddViewProp(icosahedron_actor)
ren2.AddViewProp(outline_actor)
ren1.SetBackground(colors.GetColor3d("MidnightBlue"))
ren2.SetBackground(colors.GetColor3d("MidnightBlue"))
tprop = vtk.vtkTextProperty()
tprop.SetColor(colors.GetColor3d("Yellow"))
tprop.ShadowOn()
tprop.SetFontSize(20)
axes1 = vtk.vtkCubeAxesActor2D()
axes1.SetInputConnection(normals.GetOutputPort())
axes1.SetCamera(ren1.GetActiveCamera())
axes1.SetLabelFormat("%6.4g")
axes1.SetFlyModeToOuterEdges()
axes1.SetAxisTitleTextProperty(tprop)
axes1.SetAxisLabelTextProperty(tprop)
axes1.GetProperty().SetLineWidth(2)
ren1.AddViewProp(axes1)
axes2 = vtk.vtkCubeAxesActor2D()
axes2.SetViewProp(icosahedron_actor)
axes2.SetCamera(ren2.GetActiveCamera())
axes2.SetLabelFormat("%6.4g")
axes2.SetFlyModeToClosestTriad()
axes2.ScalingOff()
axes2.SetAxisTitleTextProperty(tprop)
axes2.SetAxisLabelTextProperty(tprop)
axes2.GetProperty().SetLineWidth(2)
ren2.AddViewProp(axes2)
ren1.ResetCamera()
iren.Initialize()
renWin.Render()
iren.Start()