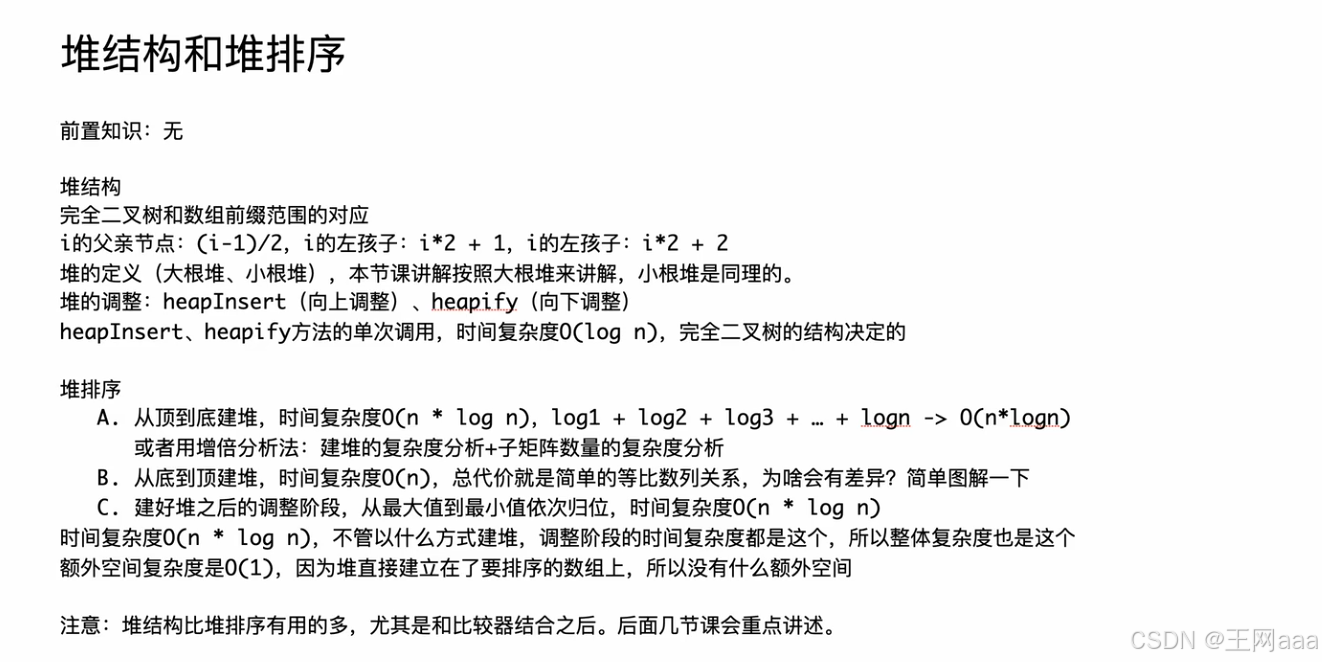
java
import java.io.*;
public class Main{
public static int MAXN = 100001;
public static int[] arr = new int[MAXN];
public static int n;
public static void main(String[] args) throws IOException{
BufferedReader br = new BufferedReader (new InputStreamReader(System.in));
StreamTokenizer in = new StreamTokenizer(br);
PrintWriter out = new PrintWriter(new OutputStreamWriter(System.out));
in.nextToken();
n = (int)in.nval;
for(int i = 0;i < n;i++){
in.nextToken();
arr[i] = (int)in.nval;
}
//堆排序
heapSort1(arr);
// heapSort2(arr);
//输出排序后的数组
for(int i = 0;i< n-1;i++){
out.print(arr[i] +" ");
}
out.print(arr[n-1]);
out.flush();
out.close();
br.close();
}
//heapInsert (i位置的数,向上调整大根堆)
public static void heapInsert(int i){
while(arr[i] > arr[(i-1)/2]){
swap(arr,i,(i-1)/2);
i = (i-1)/2;
}
}
//heapify (i位置的数,向下调整大根堆)
//当前堆的大小为size
public static void heapify(int i,int size){
int l = i*2 +1;
while(l<size){
//比较i的左孩子2i+1 和 右孩子2i+2,哪个大,选出更大的孩子下标
int best = (l + 1)<size && arr[l] < arr[l+1] ? (l+1) : l;
//再比较i和更大的孩子之间哪个大
best = arr[i] >arr[best] ? i : best;
if(best == i){
break;
}
swap(arr ,i , best);
i = best;
l = i*2 +1;
}
}
//heapSort1为从顶到底建立大根堆然后排序
//从顶到底建立大根堆 O(n*logn)
//依次弹出堆内最大值并排好序O(n*logn)
//整体时间复杂度O(n*logn)
public static void heapSort1(int arr[]){
for(int i=0;i<n;i++){
heapInsert(i);
}
int size = n;
while(size>1){
swap(arr,0,--size);
heapify(0,size);
}
}
//heapSort2为从底到顶建堆然后排序
//从底到顶建立大根堆O(n)
//依次弹出堆内最大值并排好序 O(n*logn)
//整体时间复杂度O(n*logn)
public static void heapSort2(int arr[]){
for(int i = n -1;i>=0;i--){
heapify(i,n);
}
int size = n;
while (size > 1) {
swap(arr,0, --size);
heapify(0, size);
}
}
//swap函数
public static void swap(int[] arr,int i,int j){
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}