单体架构
在这里插入图片描述
微服务架构
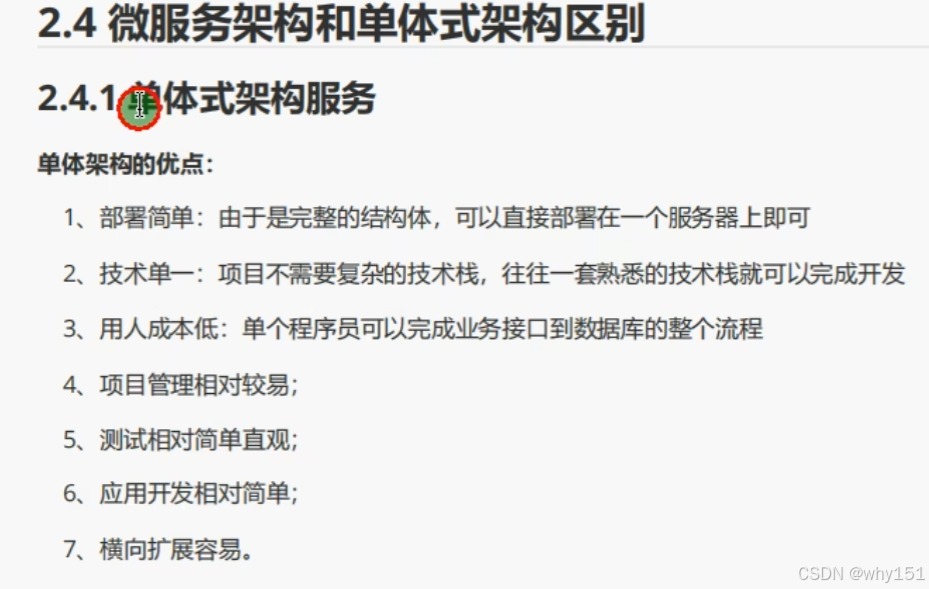
RPC架构(远程过程调用)
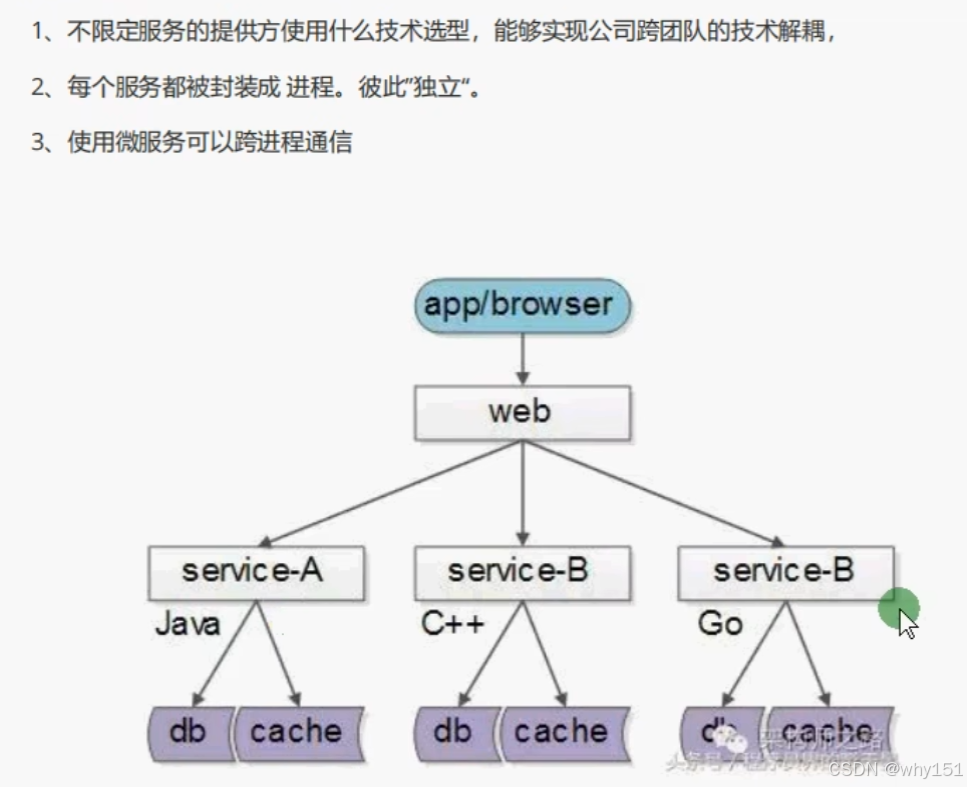
服务端实例代码:
go
package main
import (
"fmt"
"net"
"net/rpc"
"time"
)
type Hello struct {
}
func (h Hello) SayHello(req string, res *string) error {
*res = "你好," + req
fmt.Println(*res)
time.Sleep(10*time.Second)
return nil
}
func main() {
// 1. 注册RPC服务
err := rpc.RegisterName("hello", new(Hello))
if err!=nil{
fmt.Println(err)
}
// 2. 监听端口
listener, err := net.Listen("tcp", "127.0.0.1:8000")
if err!=nil{
fmt.Println(err)
}
// 3. 退出程序是关闭监听
defer listener.Close()
for{
// 4. 建立连接
conn, err := listener.Accept()
if err!=nil{
fmt.Println(err)
}
// 绑定服务
go rpc.ServeConn(conn)
}
}
客户端示例代码:
go
package main
import (
"fmt"
"net/rpc"
)
func main() {
// 1. 与RPC微服务建立连接
conn, err := rpc.Dial("tcp", "127.0.0.1:8000")
if err!=nil{
fmt.Println(err)
}
// 2. 当程序退出时关闭连接
defer conn.Close()
// 3. 远程调用函数
var res string
err = conn.Call("hello.SayHello", "hahahha", &res)
fmt.Println(res)
}

Protobuf
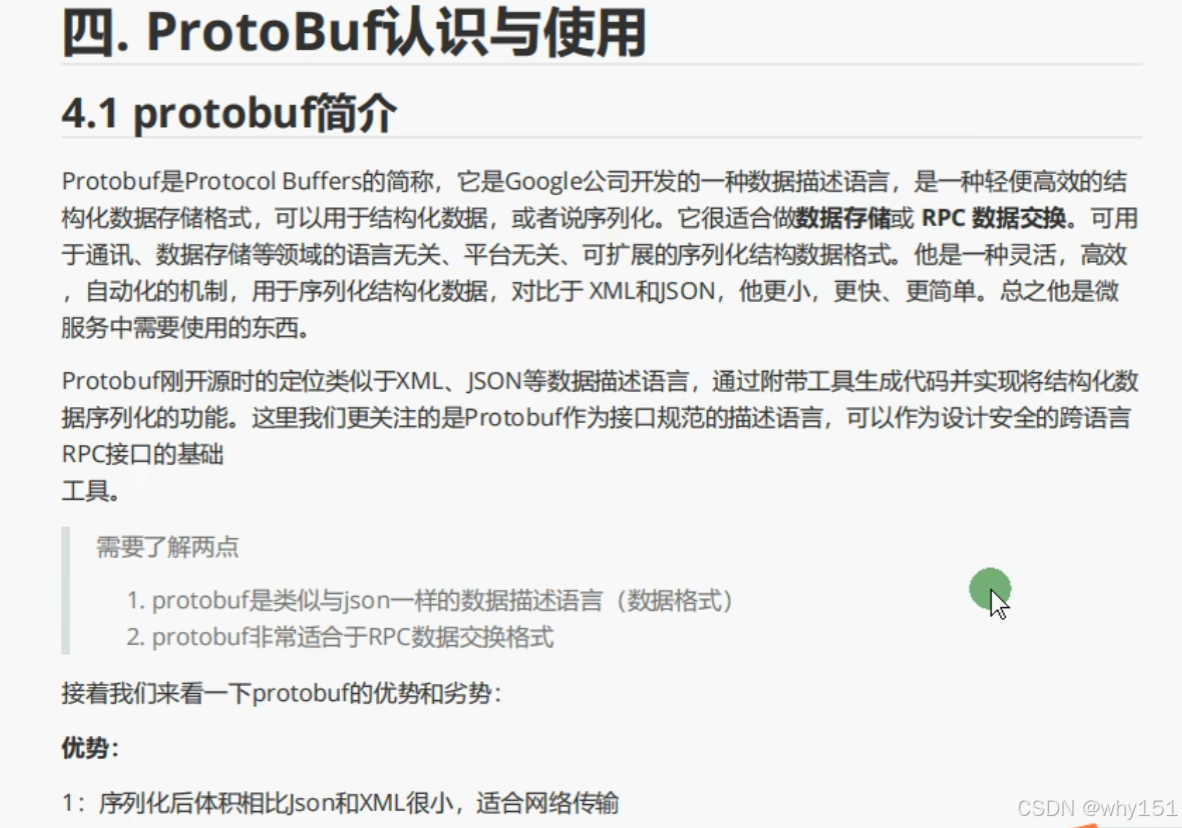
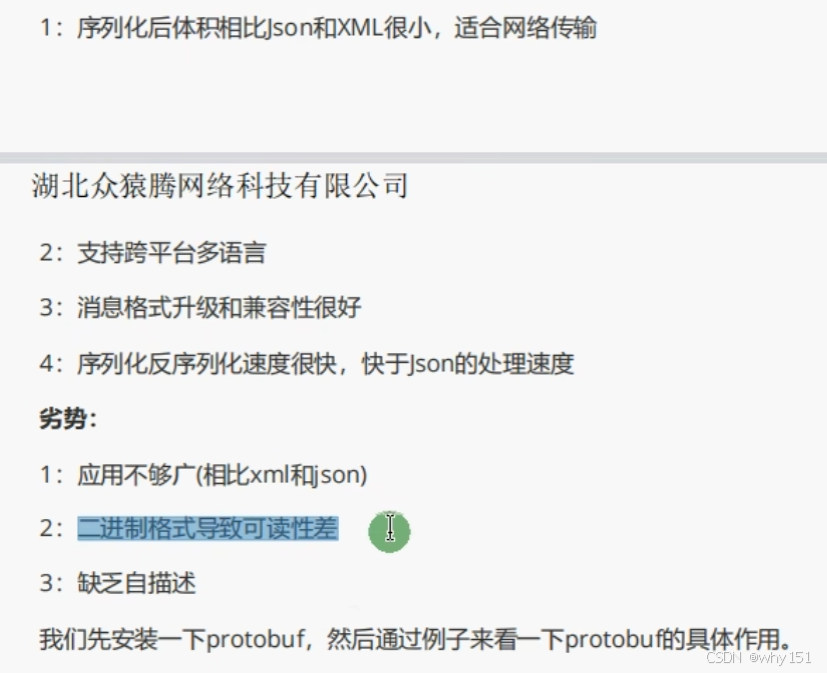
https://github.com/protocolbuffers/protobuf/releases/tag/v30.0
下载对应操作系统版本,然后解压缩,将bin文件夹的路径加入环境变量中
即为成功!
然后下载go对应的包
go get github.com/golang/protobuf/protoc-gen-go
使用样例
syntax = "proto3";
option go_package="./";
message User{
string name = 1;
bool male = 2;
repeated string hobby = 3;
}
// 执行:protoc --go_out=. *.proto
go
package main
import (
"fmt"
"google.golang.org/protobuf/proto"
)
func main() {
u := User{
Name: "张三",
Male: false,
}
data, _ := proto.Marshal(&u)
fmt.Println(data)
user := &User{}
proto.Unmarshal(data, user)
fmt.Println(user)
}
message嵌套
message SearchResponse {
message Result {
string url = 1;
string title = 2;
repeated string snippets = 3;
}
repeated Result results = 1;
}
定义服务类型
service SearchService {
rpc Search (SearchRequest) returns (SearchResponse);
}