第32集:使用 TensorFlow 构建神经网络
摘要
TensorFlow 是一个功能强大的深度学习框架,广泛应用于构建和训练神经网络模型。本集将带领您学习如何使用 TensorFlow 构建简单的神经网络,并深入理解其核心概念(如张量、计算图)以及神经网络的基本组件(如层、激活函数、损失函数)。通过实战案例,我们将使用 MNIST 数据集构建一个手写数字识别模型,帮助您掌握 TensorFlow 的基本用法。
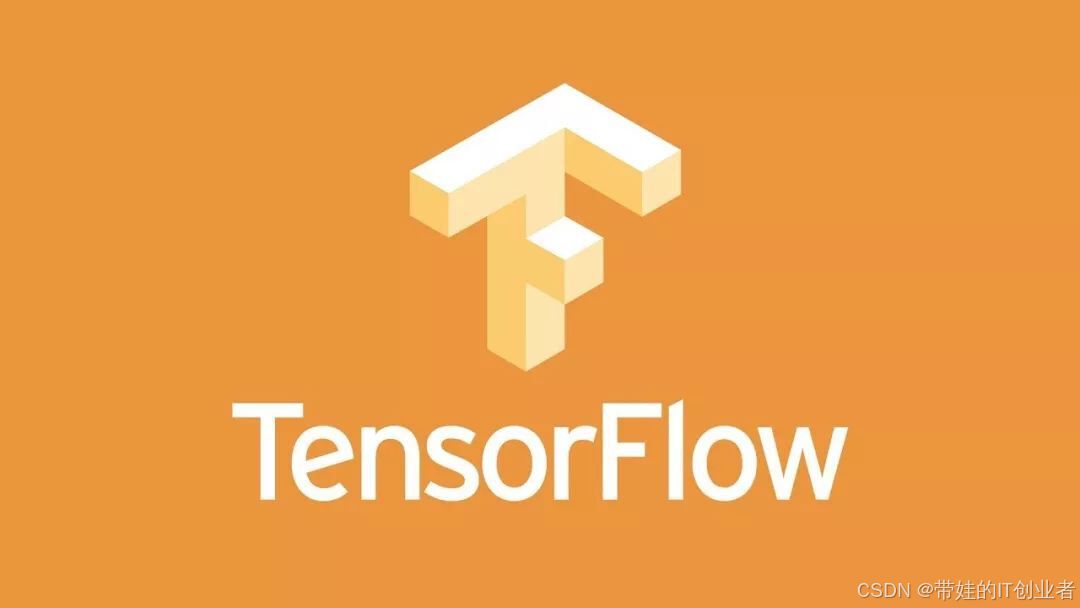
核心概念和知识点
1. TensorFlow 的基本架构
- 张量(Tensor):多维数组,是 TensorFlow 中的核心数据结构。
- 计算图(Computation Graph):TensorFlow 使用静态计算图机制,先定义计算流程,再执行计算。
- 会话(Session)与 Eager Execution:早期版本依赖会话运行计算图,而现代 TensorFlow 默认启用 Eager Execution 模式,支持动态计算。
2. 神经网络的基本组件
- 层(Layer):神经网络的基本单元,负责提取特征。
- 激活函数(Activation Function):如 ReLU、Sigmoid 和 Softmax,用于引入非线性。
- 损失函数(Loss Function):衡量模型预测值与真实值的差距,如交叉熵损失。
- 优化器(Optimizer):如梯度下降(SGD)、Adam,用于更新模型参数以最小化损失。
3. AI 大模型相关性分析
TensorFlow 是构建 AI 大模型的重要工具之一,尤其在分布式训练和大规模数据处理方面表现出色。例如:
- TensorFlow 提供了
tf.distribute
模块,支持多 GPU 和多节点的分布式训练。 - 通过
tf.keras
API,可以快速搭建复杂的深度学习模型(如 Transformer、BERT),并结合预训练权重实现迁移学习。
实战案例
案例:使用 TensorFlow 构建手写数字识别模型(MNIST 数据集)
背景
MNIST 数据集包含 70,000 张 28x28 像素的手写数字图像(0-9)。我们将使用 TensorFlow 构建一个多层感知机(MLP)模型来对这些图像进行分类。
代码实现
python
import tensorflow as tf
from tensorflow.keras import layers, models
from tensorflow.keras.datasets import mnist
from tensorflow.keras.utils import to_categorical
# 加载 MNIST 数据集
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# 数据预处理
x_train = x_train.reshape(-1, 28 * 28).astype('float32') / 255.0 # 展平并归一化
x_test = x_test.reshape(-1, 28 * 28).astype('float32') / 255.0
y_train = to_categorical(y_train, 10) # One-Hot 编码
y_test = to_categorical(y_test, 10)
# 构建神经网络模型
model = models.Sequential([
layers.Dense(128, activation='relu', input_shape=(28 * 28,)), # 隐藏层
layers.Dense(64, activation='relu'), # 隐藏层
layers.Dense(10, activation='softmax') # 输出层
])
# 编译模型
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
# 训练模型
history = model.fit(x_train, y_train, epochs=5, batch_size=128, validation_split=0.2)
# 评估模型
test_loss, test_acc = model.evaluate(x_test, y_test)
print(f"Test Accuracy: {test_acc:.2f}")
输出结果
Epoch 1/5
375/375 [==============================] - 3s 7ms/step - loss: 0.3465 - accuracy: 0.8998 - val_loss: 0.1715 - val_accuracy: 0.9490
...
Test Accuracy: 0.96
可视化
我们可以绘制训练过程中的损失和准确率变化曲线:
python
import matplotlib.pyplot as plt
# 绘制训练和验证的准确率曲线
plt.plot(history.history['accuracy'], label='Training Accuracy')
plt.plot(history.history['val_accuracy'], label='Validation Accuracy')
plt.title("Accuracy over Epochs")
plt.xlabel("Epoch")
plt.ylabel("Accuracy")
plt.legend()
plt.show()
总结
TensorFlow 提供了一个强大的工具链来构建和训练深度学习模型。通过本集的学习,我们掌握了如何使用 TensorFlow 构建一个多层感知机模型,并对其进行了训练和评估。此外,我们还了解了 TensorFlow 在分布式训练和大规模应用中的优势。
扩展思考
1. TensorFlow 和 PyTorch 的对比与选择
- TensorFlow:适合生产环境,特别是在分布式训练和部署方面表现优异。
- PyTorch:更灵活,适合研究和快速原型开发,其动态计算图机制易于调试。
两者的选择取决于具体需求:
- 如果需要快速实验和灵活性,PyTorch 更适合。
- 如果需要稳定性和可扩展性,TensorFlow 是更好的选择。
2. TensorFlow 在分布式训练中的优势
TensorFlow 提供了强大的分布式训练支持:
- 数据并行 :通过
tf.distribute.MirroredStrategy
实现多 GPU 并行训练。 - 模型并行 :通过
tf.distribute.Strategy
拆分模型到多个设备。 - 跨节点训练:支持多台机器的分布式训练,适合超大规模模型。
未来,TensorFlow 将继续在 AI 大模型领域发挥重要作用,特别是在工业级应用中。
专栏链接:Python实战进阶
下期预告:No33 : PyTorch 入门:动态计算图的优势