一.el-empty空盒子组件的使用
直接复制下面的代码:
html
<el-empty description="description" />
展示效果:
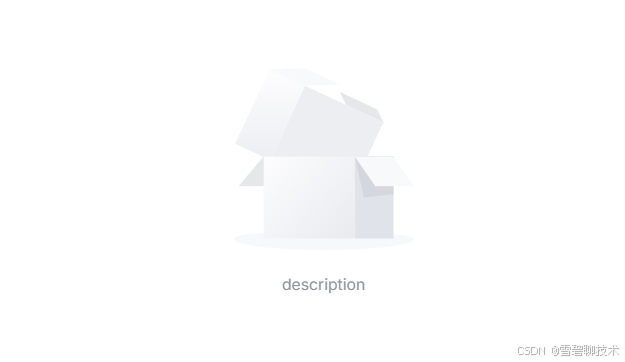
还可以自定义文字描述:
html
<el-empty description="暂未选择患者"/>
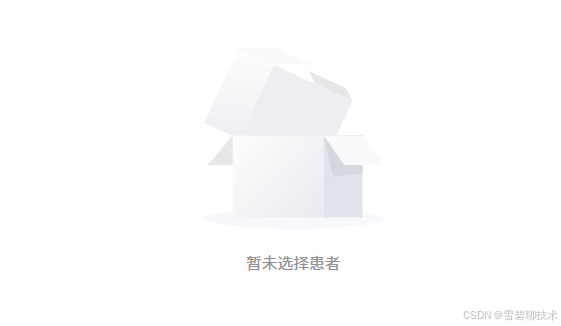
二.Collapse 折叠面板组件的使用
复制下面的代码:
html
<template>
<div>
<el-button @click="show = !show">Click Me</el-button>
<div style="margin-top: 20px">
<el-collapse-transition>
<div v-show="show" style="height: 400px">
<div class="transition-box">el-collapse-transition</div>
<div class="transition-box">el-collapse-transition</div>
</div>
</el-collapse-transition>
</div>
</div>
</template>
<script setup>
import { ref } from 'vue'
const show = ref(true)
</script>
<style>
.transition-box {
margin-bottom: 10px;
width: 200px;
height: 100px;
border-radius: 4px;
background-color: #409eff;
text-align: center;
color: #fff;
padding: 40px 20px;
box-sizing: border-box;
margin-right: 20px;
}
</style>
效果展示:
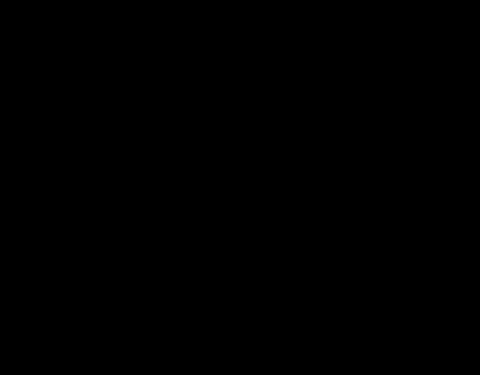
代码解读:
①自定义变量show
②v-show="show"这个属性,控制着折叠面板的显示。
③添加一个按钮,一点击就修改show的值,从而控制折叠面板是否显示。
三.综合练习
题目要求:
刚打开页面时,我们不显示患者病历表单,而是展示空盒子(表示门诊医生暂未选择看诊的患者)。等到医生选择了某个患者后,才会展示出患者病历表单。
代码大致思路:①先定义变量isChoose ,控制病历表单是否显示
javascriptconst isChoose = ref(false);
②定义一个卡片,里面分别存放空盒子组件和折叠面板,然后根据情况展示二者之一即可。
html<!-- 卡片(患者病历表单填写) --> <el-card style="width:976px;" shadow="hover" > <!-- 情况1:一开始显示空盒子 --> <el-empty description="暂未选择患者" v-show="!isChoose" /> <!-- 情况2:选择某个待诊患者后,显示出病例表单 --> <el-collapse-transition> <div v-show="isChoose" style="height:600px;width:100%;"> <el-form :model="medicalRecordDto" label-position="top"> <!-- 第一行 --> <el-row> <el-col :span="12"> <el-form-item label="主诉"> <el-input type="textarea" v-model="medicalRecordDto.chiefComplaint" placeholder="请输入主诉" style="width: 300px;margin-right: 10px" clearable></el-input> </el-form-item> </el-col> <el-col :span="12"> <el-form-item label="现病史"> <el-input type="textarea" v-model="medicalRecordDto.present" placeholder="请输入患者现病史" style="width: 300px;margin-right: 10px" clearable></el-input> </el-form-item> </el-col> </el-row> <!-- 第二行 --> <el-row> <el-col :span="12"> <el-form-item label="现病治疗情况"> <el-input type="textarea" v-model="medicalRecordDto.presentTreat" placeholder="请输入现病治疗情况" style="width: 300px;margin-right: 10px" clearable></el-input> </el-form-item> </el-col> <el-col :span="12"> <el-form-item label="既往史"> <el-input type="textarea" v-model="medicalRecordDto.history" placeholder="请输入既往史" style="width: 300px;margin-right: 10px" clearable></el-input> </el-form-item> </el-col> </el-row> <!-- 第三行 --> <el-row> <el-col :span="12"> <el-form-item label="过敏史"> <el-input type="textarea" v-model="medicalRecordDto.allergy" placeholder="请输入过敏史" style="width: 300px;margin-right: 10px" clearable></el-input> </el-form-item> </el-col> <el-col :span="12"> <el-form-item label="体格检查"> <el-input type="textarea" v-model="medicalRecordDto.physique" placeholder="请输入体格检查" style="width: 300px;margin-right: 10px" clearable></el-input> </el-form-item> </el-col> </el-row> <!-- 第四行 --> <el-row> <el-col :span="12"> <el-form-item label="检查建议"> <el-input type="textarea" v-model="medicalRecordDto.proposal" placeholder="请输入检查建议" style="width: 300px;margin-right: 10px" clearable></el-input> </el-form-item> </el-col> <el-col :span="12"> <el-form-item label="注意事项"> <el-input type="textarea" v-model="medicalRecordDto.care" placeholder="请输入注意事项" style="width: 300px;margin-right: 10px" clearable></el-input> </el-form-item> </el-col> </el-row> <!-- 第五行 --> <el-row> <el-col :span="12"> <el-form-item label="检查结果"> <el-input type="textarea" v-model="medicalRecordDto.checkResult" placeholder="请输入检查结果" style="width: 300px;margin-right: 10px" clearable></el-input> </el-form-item> </el-col> <el-col :span="12"> <el-form-item label="诊断结果"> <el-input type="textarea" v-model="medicalRecordDto.diagnose" placeholder="请输入诊断结果" style="width: 300px;margin-right: 10px" clearable></el-input> </el-form-item> </el-col> </el-row> <!-- 第六行 --> <el-row> <el-col :span="12"> <el-form-item label="处理意见"> <el-input type="textarea" v-model="medicalRecordDto.handle" placeholder="请输入处理意见" style="width: 300px;margin-right: 10px" clearable></el-input> </el-form-item> </el-col> <el-col :span="12"> <el-button-group style="margin: 50px 25px" type="flex" justify="center"> <el-button type="primary" @click="saveRecord" :icon="Refresh" size="large">暂存</el-button> <el-button type="success" @click="commitRecord" :icon="Select" size="large">提交</el-button> <el-button type="warning" @click="resetRecord" :icon="Close" size="large">清屏</el-button> </el-button-group> </el-col> </el-row> </el-form> </div> </el-collapse-transition> </el-card>
③定义一个按钮,来改变isChoose的值,从而实现控制展示空盒子还是折叠面板。
html<el-button type="primary" text @click="isChoose = !isChoose">选择</el-button>
展示效果:
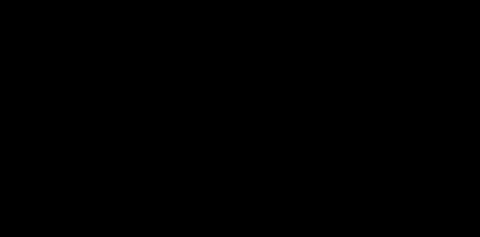
结语
以上就是el-empty空盒子组件和Collapse 折叠面板组件的使用,在项目中需要的话可以这么做。
喜欢本篇文章的话,可以留个免费的关注~