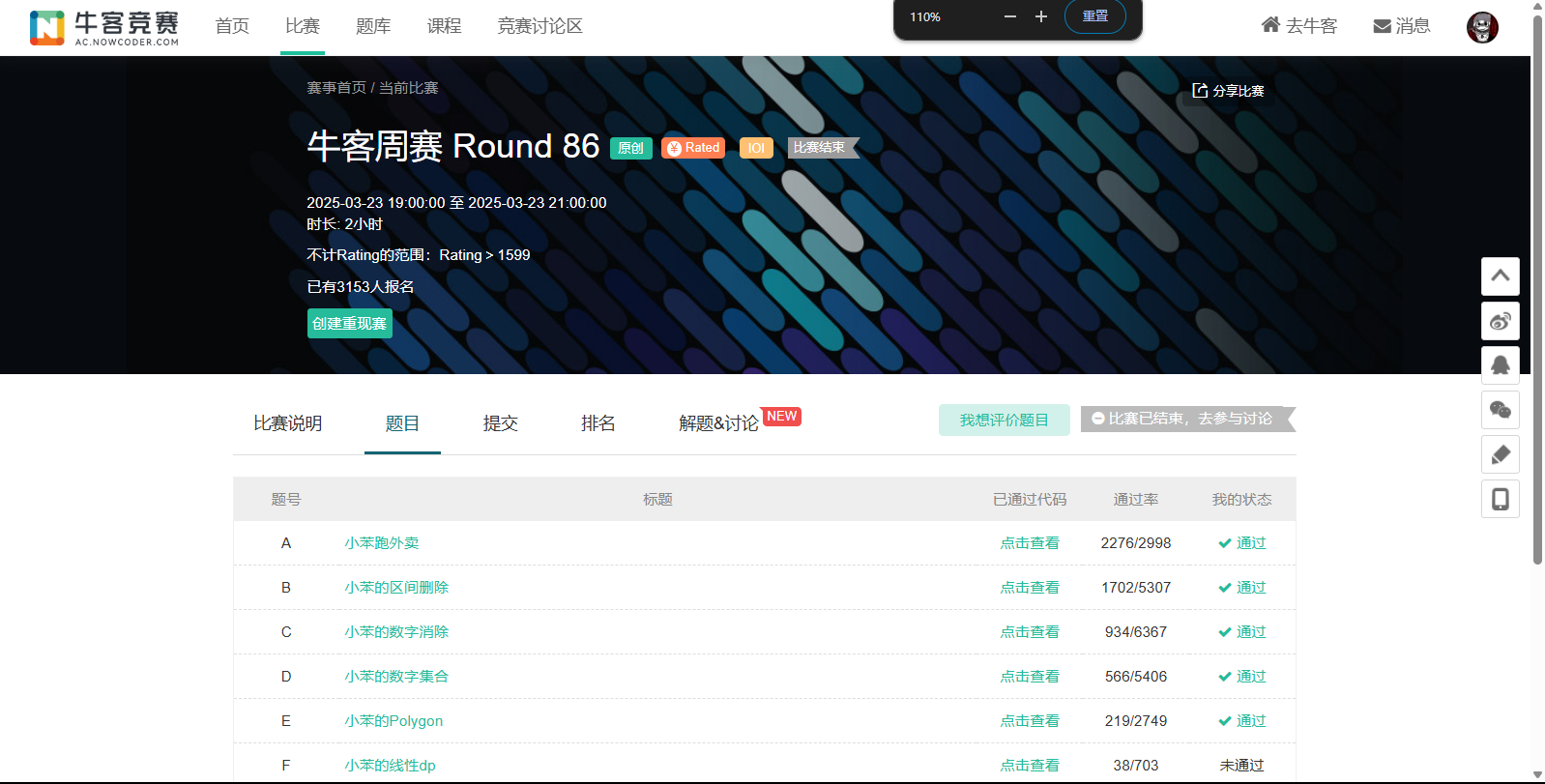
A 小苯跑外卖
除一下看有没有余数
有余数得多一天
没余数正好
// @github https://github.com/Dddddduo
// @github https://github.com/Dddddduo/acm-java-algorithm
// @github https://github.com/Dddddduo/Dduo-mini-data_structure
import java.util.*;
import java.io.*;
import java.math.*;
import java.lang.*;
import java.time.*;
/**
* 题目地址
* 牛客周赛 Round 86 A
*/
// xixi♡西
public class Main {
static IoScanner sc = new IoScanner();
static final int mod = (int) (1e9 + 7);
// static final int mod = (int) (1e9 + 7);
static int n;
static int arr[];
static boolean visited[];
static ArrayList<ArrayList<Integer>> adj = new ArrayList<>();
/**
* @throws IOException
*/
private static void solve() throws IOException {
// todo
long n=sc.nextLong();
long m=sc.nextInt();
if(m%n==0){
dduoln(m/n);
}else{
dduoln(m/n+1);
}
}
public static void main(String[] args) throws Exception {
int t = 1;
// t = sc.nextInt();
while (t-- > 0) {
solve();
}
}
static <T> void dduo(T t) {
System.out.print(t);
}
static <T> void dduoln() {
System.out.println("");
}
static <T> void dduoln(T t) {
System.out.println(t);
}
}
/**
* IoScanner类
*
* @author Dduo
* @version 1.0
* @description 通过IO流操作缓冲区减少了与底层输入输出设备的交互次数,旨在简化 Java 中的标准输入读取操作。
*/
class IoScanner {
BufferedReader bf;
StringTokenizer st;
BufferedWriter bw;
public IoScanner() {
bf = new BufferedReader(new InputStreamReader(System.in));
st = new StringTokenizer("");
bw = new BufferedWriter(new OutputStreamWriter(System.out));
}
public String nextLine() throws IOException {
return bf.readLine();
}
public String next() throws IOException {
while (!st.hasMoreTokens()) {
st = new StringTokenizer(bf.readLine());
}
return st.nextToken();
}
public char nextChar() throws IOException {
return next().charAt(0);
}
public int nextInt() throws IOException {
return Integer.parseInt(next());
}
public long nextLong() throws IOException {
return Long.parseLong(next());
}
public double nextDouble() throws IOException {
return Double.parseDouble(next());
}
public float nextFloat() throws IOException {
return Float.parseFloat(next());
}
public BigInteger nextBigInteger() throws IOException {
return new BigInteger(next());
}
public BigDecimal nextDecimal() throws IOException {
return new BigDecimal(next());
}
}
B 小苯的区间删除
一次删一个元素(选择一个长度为 1 的区间)
把负数全删掉
求和
// @github https://github.com/Dddddduo
// @github https://github.com/Dddddduo/acm-java-algorithm
// @github https://github.com/Dddddduo/Dduo-mini-data_structure
import java.util.*;
import java.io.*;
import java.math.*;
import java.lang.*;
import java.time.*;
/**
* 题目地址
* 牛客周赛 Round 86 B
*/
// xixi♡西
public class Main {
static IoScanner sc = new IoScanner();
static final int mod = (int) (1e9 + 7);
// static final int mod = (int) (1e9 + 7);
static int n;
static int arr[];
static boolean visited[];
static ArrayList<ArrayList<Integer>> adj = new ArrayList<>();
/**
* @throws IOException
*/
private static void solve() throws IOException {
// todo
long n=sc.nextLong();
long k=sc.nextInt();
long arr[]=new long[(int) n];
for (long i = 0; i < n; i++) {
arr[(int) i]=sc.nextLong();
}
long sum=0;
for (long l : arr) {
if(l>0)sum+=l;
}
dduoln(sum);
}
public static void main(String[] args) throws Exception {
int t = 1;
t = sc.nextInt();
while (t-- > 0) {
solve();
}
}
static <T> void dduo(T t) {
System.out.print(t);
}
static <T> void dduoln() {
System.out.println("");
}
static <T> void dduoln(T t) {
System.out.println(t);
}
}
/**
* IoScanner类
*
* @author Dduo
* @version 1.0
* @description 通过IO流操作缓冲区减少了与底层输入输出设备的交互次数,旨在简化 Java 中的标准输入读取操作。
*/
class IoScanner {
BufferedReader bf;
StringTokenizer st;
BufferedWriter bw;
public IoScanner() {
bf = new BufferedReader(new InputStreamReader(System.in));
st = new StringTokenizer("");
bw = new BufferedWriter(new OutputStreamWriter(System.out));
}
public String nextLine() throws IOException {
return bf.readLine();
}
public String next() throws IOException {
while (!st.hasMoreTokens()) {
st = new StringTokenizer(bf.readLine());
}
return st.nextToken();
}
public char nextChar() throws IOException {
return next().charAt(0);
}
public int nextInt() throws IOException {
return Integer.parseInt(next());
}
public long nextLong() throws IOException {
return Long.parseLong(next());
}
public double nextDouble() throws IOException {
return Double.parseDouble(next());
}
public float nextFloat() throws IOException {
return Float.parseFloat(next());
}
public BigInteger nextBigInteger() throws IOException {
return new BigInteger(next());
}
public BigDecimal nextDecimal() throws IOException {
return new BigDecimal(next());
}
}
C 小苯的数字消除
这题如果要考虑对字符 串 处理 我感觉是很难的
我们不难想到可以用双端队列
如果队列尾部的元素跟要添加的元素一样
那么不用添加了 队尾的元素也出来吧
如果队列尾部的元素跟要添加的元素不一样
那么把这个元素到队列尾部
最后得到的队列肯定是 01010101 101010... 类似的
满足消除相同的
之后再对队列长度/2 即可得出答案
// @github https://github.com/Dddddduo
// @github https://github.com/Dddddduo/acm-java-algorithm
// @github https://github.com/Dddddduo/Dduo-mini-data_structure
import java.util.*;
import java.io.*;
import java.math.*;
import java.lang.*;
import java.time.*;
/**
* 题目地址
* 牛客周赛 Round 86 C
*/
// xixi♡西
public class Main {
static IoScanner sc = new IoScanner();
static final int mod = (int) (1e9 + 7);
// static final int mod = (int) (1e9 + 7);
static int n;
static int arr[];
static boolean visited[];
static ArrayList<ArrayList<Integer>> adj = new ArrayList<>();
static ArrayList<Integer>list=new ArrayList<>();
/**
* @throws IOException
*/
private static void solve() throws IOException {
// todo
int n=sc.nextInt();
String str=sc.next();
Deque<Character>queue= new LinkedList<>();
queue.add(str.charAt(0));
for(int i=1;i<str.length();i++){
if(queue.isEmpty()){
queue.addLast(str.charAt(i));
continue;
}
if(queue.getLast().equals(str.charAt(i))){
// dduoln(i);
queue.removeLast();
}else{
queue.addLast(str.charAt(i));
}
}
// dduoln(queue.size());
dduoln(queue.size()/2);
}
// 计算两个数的最大公约数
public static long gcd(long a, long b) {
while (b != 0) {
long temp = b;
b = a % b;
a = temp;
}
return a;
}
// 计算两个数的最小公倍数
public static long lcm(long a, long b) {
return (a / gcd(a, b)) * b;
}
public static void main(String[] args) throws Exception {
int t = 1;
t = sc.nextInt();
while (t-- > 0) {
solve();
}
}
static <T> void dduo(T t) {
System.out.print(t);
}
static <T> void dduoln() {
System.out.println("");
}
static <T> void dduoln(T t) {
System.out.println(t);
}
}
/**
* IoScanner类
*
* @author Dduo
* @version 1.0
* @description 通过IO流操作缓冲区减少了与底层输入输出设备的交互次数,旨在简化 Java 中的标准输入读取操作。
*/
class IoScanner {
BufferedReader bf;
StringTokenizer st;
BufferedWriter bw;
public IoScanner() {
bf = new BufferedReader(new InputStreamReader(System.in));
st = new StringTokenizer("");
bw = new BufferedWriter(new OutputStreamWriter(System.out));
}
public String nextLine() throws IOException {
return bf.readLine();
}
public String next() throws IOException {
while (!st.hasMoreTokens()) {
st = new StringTokenizer(bf.readLine());
}
return st.nextToken();
}
public char nextChar() throws IOException {
return next().charAt(0);
}
public int nextInt() throws IOException {
return Integer.parseInt(next());
}
public long nextLong() throws IOException {
return Long.parseLong(next());
}
public double nextDouble() throws IOException {
return Double.parseDouble(next());
}
public float nextFloat() throws IOException {
return Float.parseFloat(next());
}
public BigInteger nextBigInteger() throws IOException {
return new BigInteger(next());
}
public BigDecimal nextDecimal() throws IOException {
return new BigDecimal(next());
}
}
D 小苯的数字集合
首先我们知道的是 两个数如果相同 异或运算得到的数为 0
那么如果现在集合里面有两个一样的数 那么只要再操作一次
之后就不难想到
最多操作 3 次就得出 0
因为前两次我们完全可以进行同样的两次运算
得到两个一样的数 然后第三次运算异或
所以最多经过 3 轮就能得出 0
之后我们完全可以枚举
如果要经过 1 轮得出答案 有 4 种组合 看一下这些组合有没有得出 0
如果要经过 2 轮得出答案 有 4*4=16 种组合 看一下这些组合有没有得出 0
// @github https://github.com/Dddddduo
// @github https://github.com/Dddddduo/acm-java-algorithm
// @github https://github.com/Dddddduo/Dduo-mini-data_structure
import java.util.*;
import java.io.*;
import java.math.*;
import java.lang.*;
import java.time.*;
/**
* 题目地址
* 牛客周赛 Round 86 D
*/
// xixi♡西
public class Main {
static IoScanner sc = new IoScanner();
static final int mod = (int) (1e9 + 7);
// static final int mod = (int) (1e9 + 7);
static int n;
static int arr[];
static boolean visited[];
static ArrayList<ArrayList<Integer>> adj = new ArrayList<>();
static ArrayList<Integer>list=new ArrayList<>();
/**
* @throws IOException
*/
private static void solve() throws IOException {
// todo
long x = sc.nextLong();
long y = sc.nextLong();
if (x == 0 || y == 0) {
dduoln(0);
return;
} else if ( (x & y) == 0 || (x | y) == 0 || (x ^ y) == 0 || gcd(x,y)==0) {
dduoln(1);
return;
}
long z=0;
// &
z=x&y;
if(judge(x,z)||judge(x,y)||judge(z,y)){
dduoln("2");
return;
}
// |
z=x|y;
if(judge(x,z)||judge(x,y)||judge(z,y)){
dduoln("2");
return;
}
// ^
z=x^y;
if(judge(x,z)||judge(x,y)||judge(z,y)){
dduoln("2");
return;
}
// gcd
z=gcd(x,y);
if(judge(x,z)||judge(x,y)||judge(z,y)){
dduoln("2");
return;
}
dduoln(3);
}
public static boolean judge(long x,long y){
if((x&y)==0||(x|y)==0||(x^y)==0||gcd(x,y)==0){
return true;
}else return false;
}
// 计算两个数的最大公约数
public static long gcd(long a, long b) {
while (b != 0) {
long temp = b;
b = a % b;
a = temp;
}
return a;
}
// 计算两个数的最小公倍数
public static long lcm(long a, long b) {
return (a / gcd(a, b)) * b;
}
public static void main(String[] args) throws Exception {
int t = 1;
t = sc.nextInt();
while (t-- > 0) {
solve();
}
}
static <T> void dduo(T t) {
System.out.print(t);
}
static <T> void dduoln() {
System.out.println("");
}
static <T> void dduoln(T t) {
System.out.println(t);
}
}
/**
* IoScanner类
*
* @author Dduo
* @version 1.0
* @description 通过IO流操作缓冲区减少了与底层输入输出设备的交互次数,旨在简化 Java 中的标准输入读取操作。
*/
class IoScanner {
BufferedReader bf;
StringTokenizer st;
BufferedWriter bw;
public IoScanner() {
bf = new BufferedReader(new InputStreamReader(System.in));
st = new StringTokenizer("");
bw = new BufferedWriter(new OutputStreamWriter(System.out));
}
public String nextLine() throws IOException {
return bf.readLine();
}
public String next() throws IOException {
while (!st.hasMoreTokens()) {
st = new StringTokenizer(bf.readLine());
}
return st.nextToken();
}
public char nextChar() throws IOException {
return next().charAt(0);
}
public int nextInt() throws IOException {
return Integer.parseInt(next());
}
public long nextLong() throws IOException {
return Long.parseLong(next());
}
public double nextDouble() throws IOException {
return Double.parseDouble(next());
}
public float nextFloat() throws IOException {
return Float.parseFloat(next());
}
public BigInteger nextBigInteger() throws IOException {
return new BigInteger(next());
}
public BigDecimal nextDecimal() throws IOException {
return new BigDecimal(next());
}
}
E 小苯的Polygon
封闭凸多边形内角都小于 180 度
首先要知道 要构成封闭凸多边形
那么得最长那条边比其余的边加起来要小就行
即 max<sum
所以我们先对数组排序
因为数据量很小 我考虑的暴力了一点 直接枚举了每条边都是最长边的情况
然后 就是很经典 dp 模版 选一些边要求和大于 sum 而且这些和要最小
// @github https://github.com/Dddddduo
// @github https://github.com/Dddddduo/acm-java-algorithm
// @github https://github.com/Dddddduo/Dduo-mini-data_structure
import java.util.*;
import java.io.*;
import java.math.*;
import java.lang.*;
import java.time.*;
/**
* 题目地址
* 牛客周赛 Round 86 E
*/
// xixi♡西
public class Main {
static IoScanner sc = new IoScanner();
static final int mod = (int) (1e9 + 7);
// static final int mod = (int) (1e9 + 7);
static int n;
static int arr[];
static boolean visited[];
static ArrayList<ArrayList<Integer>> adj = new ArrayList<>();
/**
* @throws IOException
*/
private static void solve() throws IOException {
// todo
int n = sc.nextInt();
int[] a = new int[n];
for (int i = 0; i < n; i++) {
a[i] = sc.nextInt();
}
Arrays.sort(a);
int min = Integer.MAX_VALUE;
for (int i = 0; i < n; i++) {
int max = a[i];
if (max >= min) {
break;
}
List<Integer> list = new ArrayList<>();
for (int j = 0; j < i; j++) {
list.add(a[j]);
}
if (list.isEmpty()) {
continue;
}
int sum = 0;
for (int x : list) {
sum += x;
}
if (sum <= max) {
continue;
}
boolean[] dp = new boolean[sum + 5];
dp[0] = true;
// 当前最大值
int c = 0;
for (int x : list) {
for (int j = c; j >= 0; j--) {
if (dp[j] == true) {
int newSum = j + x;
if (newSum > sum) {
continue;
}
if (dp[newSum] == false) {
dp[newSum] = true;
if (newSum > c) {
c = newSum;
}
}
}
}
}
for (int sumOther = max + 1; sumOther <= c; sumOther++) {
if (dp[sumOther]) {
int perimeter = sumOther + max;
if (perimeter < min) {
min = perimeter;
}
break;
}
}
}
dduoln(min == 2147483647 ? "-1" : min);
}
public static void main(String[] args) throws Exception {
int t = 1;
t = sc.nextInt();
while (t-- > 0) {
solve();
}
}
static <T> void dduo(T t) {
System.out.print(t);
}
static <T> void dduoln() {
System.out.println("");
}
static <T> void dduoln(T t) {
System.out.println(t);
}
}
/**
* IoScanner类
*
* @author Dduo
* @version 1.0
* @description 通过IO流操作缓冲区减少了与底层输入输出设备的交互次数,旨在简化 Java 中的标准输入读取操作。
*/
class IoScanner {
BufferedReader bf;
StringTokenizer st;
BufferedWriter bw;
public IoScanner() {
bf = new BufferedReader(new InputStreamReader(System.in));
st = new StringTokenizer("");
bw = new BufferedWriter(new OutputStreamWriter(System.out));
}
public String nextLine() throws IOException {
return bf.readLine();
}
public String next() throws IOException {
while (!st.hasMoreTokens()) {
st = new StringTokenizer(bf.readLine());
}
return st.nextToken();
}
public char nextChar() throws IOException {
return next().charAt(0);
}
public int nextInt() throws IOException {
return Integer.parseInt(next());
}
public long nextLong() throws IOException {
return Long.parseLong(next());
}
public double nextDouble() throws IOException {
return Double.parseDouble(next());
}
public float nextFloat() throws IOException {
return Float.parseFloat(next());
}
public BigInteger nextBigInteger() throws IOException {
return new BigInteger(next());
}
public BigDecimal nextDecimal() throws IOException {
return new BigDecimal(next());
}
}
F 小苯的线性dp
不会