**1.**栈
1.1****栈的概念及结构
栈:一种特殊的线性表,其只允许在固定的一端进行插入和删除元素操作。**进行数据插入和删除操作的一端****称为栈顶,另一端称为栈底。**栈中的数据元素遵守后进先出LIFO(Last In First Out)的原则。
压栈:栈的插入操作叫做进栈 / 压栈 / 入栈, 入数据在栈顶 。
出栈:栈的删除操作叫做出栈。 出数据也在栈顶 。
注意:栈后进先出
2.栈的实现
栈的实现一般可以使用 数组或者链表实现 ,相对而言数组的结构实现更优一些。因为数组在尾上插入数据的 代价比较小。
1.栈的初始化
定义栈顶有两种情况:1.栈顶指向元素 2.栈顶指向元素下一个一个位置
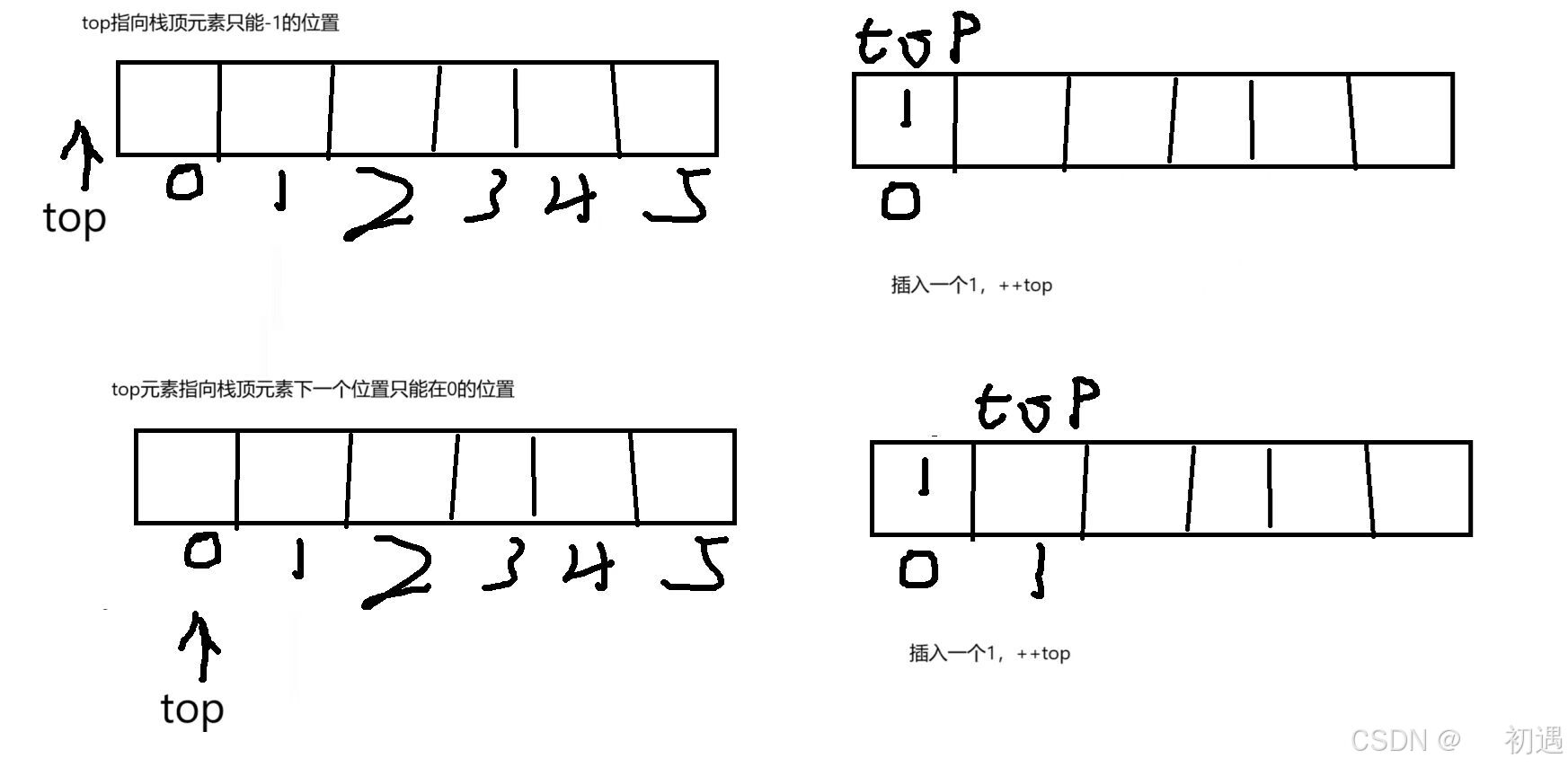
//初始化
void STInit(ST* ps)
{
assert(ps);
//top指向栈顶等于栈顶下一个元素
ps->top = 0;
ps->a = NULL;
ps->capcity = 0;
//top指向栈顶的元素
//ps->top=-1
}
2.栈的销毁
void StDestroy(ST* ps)//销毁
{
assert(ps);
ps->capcity = ps->top = 0;
free(ps->a);
ps->a = NULL;
}
3.入栈
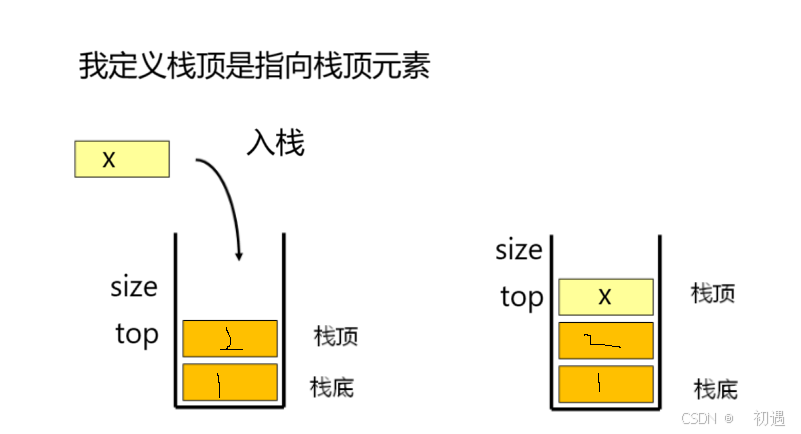
void STPush(ST* ps, STDataType x)//入栈
{
assert(ps);
if (ps->capcity == ps->top)
{
int newcapcity = ps->capcity = 0 ? 4 : ps->capcity * 2;
STDataType* temp = (STDataType*)realloc(ps->a, newcapcity * sizeof(STDataType));
if (temp == NULL)
{
perror("realloc fail");
exit(1);
}
ps->a = temp;
ps->capcity = newcapcity;
}
ps->a[ps->top++] = x;
}
4.出栈
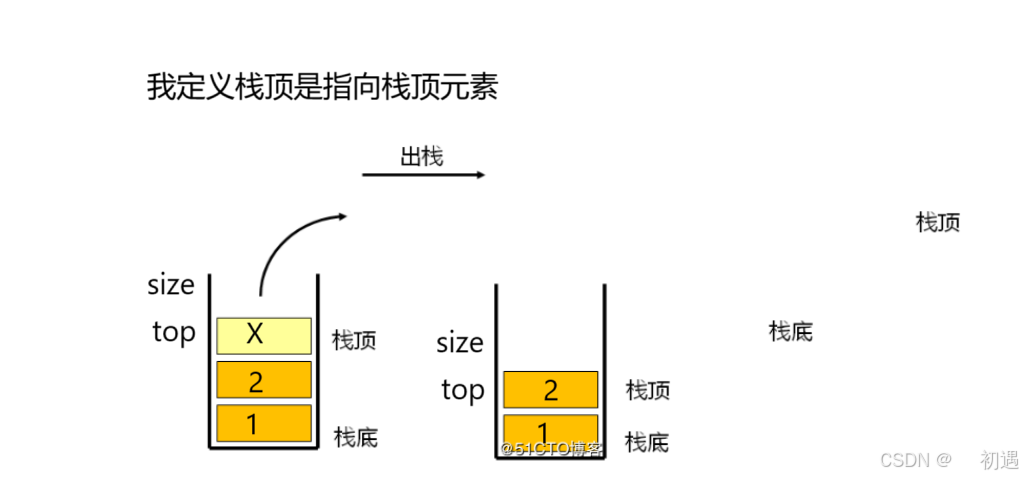
void STPop(ST* ps)//出栈
{
assert(ps);
assert(ps->top > 0);
//return ps->a[ps->top--];
ps->top--;
}
5.获取元素个数
//获取元素个数
int STSize(ST* ps)
{
assert(ps);
return ps->top;
}
6.获取栈顶数据
//获取栈顶数据
STDataType STTop(ST* ps)
{
assert(ps);
assert(ps->top > 0);
return ps->a[ps->top - 1];
}
7.判空
//判空
bool STEmpty(ST* ps)
{
assert(ps);
return ps->top == 0;
}
3.完整代码
#pragma once
#include<stdio.h>
#include<stdlib.h>
#include<assert.h>
#include<stdbool.h>
typedef int STDataType;
typedef struct Stack
{
STDataType* a;
int capcity;
int top;
}ST;
//初始化和销毁
void STInit(ST* ps);
void StDestroy(ST* ps);
//入栈和出栈
void STPush(ST* ps, STDataType x);
void STPop(ST* ps);
//获取个数
int STSize(ST* ps);
//获取栈顶数据
STDataType STTop(ST* ps);
//判空
bool STEmpty(ST* ps);
#include"Stcak.h"
//初始化
void STInit(ST* ps)
{
assert(ps);
//top指向栈顶等于栈顶下一个元素
ps->top = 0;
ps->a = NULL;
ps->capcity = 0;
//top指向栈顶的元素
//ps->top=-1
}
void StDestroy(ST* ps)//销毁
{
assert(ps);
ps->capcity = ps->top = 0;
free(ps->a);
ps->a = NULL;
}
void STPush(ST* ps, STDataType x)//入栈
{
assert(ps);
if (ps->capcity == ps->top)
{
int newcapcity = ps->capcity = 0 ? 4 : ps->capcity * 2;
STDataType* temp = (STDataType*)realloc(ps->a, newcapcity * sizeof(STDataType));
if (temp == NULL)
{
perror("realloc fail");
exit(1);
}
ps->a = temp;
ps->capcity = newcapcity;
}
ps->a[ps->top++] = x;
}
void STPop(ST* ps)//出栈
{
assert(ps);
assert(ps->top > 0);
//return ps->a[ps->top--];
ps->top--;
}
//获取元素个数
int STSize(ST* ps)
{
assert(ps);
return ps->top;
}
//获取栈顶数据
STDataType STTop(ST* ps)
{
assert(ps);
assert(ps->top > 0);
return ps->a[ps->top - 1];
}
//判空
bool STEmpty(ST* ps)
{
assert(ps);
return ps->top == 0;
}
#include"Stcak.h"
void test()
{
ST sl;
STInit(&sl);
STPush(&sl, 1);
STPush(&sl, 2);
STPush(&sl, 3);
while (!STEmpty(&sl))
{
printf("%d ", STTop(&sl));
STPop(&sl);
}
}
int main()
{
test();
return 0;
}