文章目录
- [1 nlohmann/json库说明](#1 nlohmann/json库说明)
- [2 nlohmann/json特点](#2 nlohmann/json特点)
- [3 nlohmann/json库的使用方法](#3 nlohmann/json库的使用方法)
-
- [3.1 引入头文件](#3.1 引入头文件)
- [3.2 解析JSON字符串](#3.2 解析JSON字符串)
- [3.3 访问JSON数据](#3.3 访问JSON数据)
- [3.4 生成JSON对象](#3.4 生成JSON对象)
- [3.5 修改JSON数据](#3.5 修改JSON数据)
- [3.6 将JSON写入文件](#3.6 将JSON写入文件)
- [3.7 遍历JSON对象](#3.7 遍历JSON对象)
- [4 代码示例](#4 代码示例)
-
- [4.1 定义JSON数值类型](#4.1 定义JSON数值类型)
- [4.2 从STL容器转换到json](#4.2 从STL容器转换到json)
- [4.3 string序列化和反序列化及JSON格式和C++对象之间转换](#4.3 string序列化和反序列化及JSON格式和C++对象之间转换)
- [4.4 stream的序列化和反序列化](#4.4 stream的序列化和反序列化)
- [4.5 任意类型转换](#4.5 任意类型转换)
- [5 总结](#5 总结)
1 nlohmann/json库说明
在现代软件开发中,JSON(JavaScript Object Notation)已经成为数据交换和存储的标配格式。C++作为一种高效且广泛使用的编程语言,在处理JSON数据时也需要一个强大且易用的库。nlohmann/json库正是这样一个选择,该库提供了丰富的API,使得在C++项目中处理JSON数据变得极为简单和高效。本文主要说明nlohmann/json库的特点、使用方法以及一些代码示例。
2 nlohmann/json特点
- 轻量级:nlohmann/json是一个单头文件库,只需将一个头文件引入项目即可使用。不需要链接额外的库文件,使用时非常方便;
- 简单易用:nlohmann/json库的接口设计非常直观,与C++ STL风格非常接近。提供了类似于STL容器的API,可以方便地处理JSON数据。同时,还支持多种数据类型,包括字符串、数字、布尔值、数组和对象等。能够轻松地进行序列化和反序列化操作;
- 功能强大:nlohmann/json库支持从JSON格式到C++标准容器(如std::vector、std::map)的自动转换。还支持将C++对象转换为JSON格式,或者将JSON数据解析为C++对象。该库还提供了对JSON数据的遍历、修改、删除等操作的支持;
- 高效性能:nlohmann/json库充分利用了C++11的特性,如移动语义和模板元编程等,以获得高效的性能。尽管在处理非常大的JSON文件时性能可能不是最优,但对于大多数应用场景来说,该库的性能已经足够出色。
- 兼容性强:nlohmann/json库支持多种JSON格式,包括RFC 8259、ECMA-404和JSON5等。这使得该库可以与不同的JSON数据源进行交互,提高了数据的兼容性;
- 开源免费:nlohmann/json是开源的,使用MIT许可证,可以免费使用和修改。提供了极大的便利和灵活性。
3 nlohmann/json库的使用方法
3.1 引入头文件
要使用nlohmann/json库,只需将头文件 single_include/nlohmann/json.hpp
引入C++项目中即可。例如:
cpp
#include <nlohmann/json.hpp>
using json = nlohmann::json;
3.2 解析JSON字符串
使用json::parse
函数将一个JSON格式的字符串解析为一个json
对象。例如:
cpp
std::string jsonString = R"({"title": "gad", "year": 1940})";
json jsonObj = json::parse(jsonString);
std::string title = jsonObj["title"];
int year = jsonObj["year"];
3.3 访问JSON数据
提供访问STL容器一样访问json
对象中的数据API。例如:
cpp
jsonObj["title"]; // 访问字符串类型的JSON数据(书名)
jsonObj["year"]; // 访问整数类型的JSON数据(出版年份)
jsonObj["is_available"];// 访问布尔类型的JSON数据(是否可借阅)
jsonObj["authors"]; // 访问数组类型的JSON数据(作者列表)
3.4 生成JSON对象
使用json
对象来创建一个JSON对象,并将其转换为字符串。例如:
cpp
json jsonObj;
jsonObj["title"] = "The Catcher in the Rye";
jsonObj["year"] = 1951;
jsonObj["is_available"] = true;
jsonObj["authors"] = { "J.D. Salinger", "Bob" };
std::string jsonStr = jsonObj.dump(4); // 参数4表示缩进4个空格
3.5 修改JSON数据
对json
对象中的数据直接进行修改。例如:
cpp
json jsonObj = {
{"title", "1984"},
{"year", 1949},
{"is_available", false},
{"authors", {"George Orwell"}}
};
jsonObj["year"] = 1950; // 修改出版年份
jsonObj["authors"].push_back("Erich Fromm"); // 添加新作者
3.6 将JSON写入文件
生成的json
对象保存到文件中,或者从文件中读取json
数据。例如:
cpp
std::ofstream file("book.json");
file << jsonObj.dump(4); // 缩进4个空格
file.close();
// 从文件读取
std::ifstream inputFile("book.json");
json readJson;
inputFile >> readJson;
3.7 遍历JSON对象
使用迭代器来遍历json
对象中的键值对。例如:
cpp
for (auto it = jsonObj.begin(); it != jsonObj.end(); ++it) {
std::cout << it.key() << ": " << it.value() << std::endl;
}
4 代码示例
4.1 定义JSON数值类型
cpp
json jsonObj;
jsonObj["title"] = "The Catcher in the Rye";
jsonObj["year"] = 1951;
jsonObj["is_available"] = true;
jsonObj["authors"] = { "J.D. Salinger", "Bob" };
jsonObj["nothing"] = nullptr;
jsonObj["version"] = { 0, 1, 2 };
jsonObj["object"] = { { "state", "pub" }, { "next version", 42.99 } };
4.2 从STL容器转换到json
cpp
std::vector<int> intVector{ 0, 1, 2, 3 };
json jVec(intVector);
std::cout << "jVec = " << jVec << std::endl;
std::map<int, std::string> mapIntString{ {1, "one"},{2, "two"} };
json jMap(mapIntString);
std::cout << "jMap = " << jMap << std::endl;
std::deque<double> doubleDeque{ 1.2,2.2,3.3 };
json jDeque(doubleDeque);
std::cout << "jDeque = " << jDeque << std::endl;
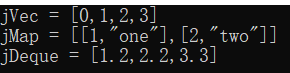
4.3 string序列化和反序列化及JSON格式和C++对象之间转换
cpp
#include <iostream>
#include <nlohmann/json.hpp>
using json = nlohmann::json;
struct Book {
std::string title;
int year;
bool isAvailable;
};
// 序列化:Book -> JSON
void to_json(json& j, const Book& book) {
j = json{
{"title", book.title},
{"year", book.year},
{"isAvailable", book.isAvailable} // JSON字段名可与C++成员不同
};
}
// 反序列化:JSON -> Book
void from_json(const json& j, Book& book) {
j.at("title").get_to(book.title); // 使用 .at() 确保字段存在
j.at("year").get_to(book.year);
j.at("isAvailable").get_to(book.isAvailable);
}
int main()
{
Book book1{ "gad", 30, true };
json jsonObj1 = book1;
std::cout << "序列化结果:\n" << jsonObj1.dump(2) << "\n\n";
// JSON转结构体
std::string jsonStr = R"({
"title": "The Great Gatsby",
"year": 1925,
"isAvailable": true
})";
auto j2 = json::parse(jsonStr);
Book book2 = j2.get<Book>(); // 自动调用 from_json
std::cout << "反序列化结果:\n"
<< "书名: " << book2.title << "\n"
<< "年份: " << book2.year << "\n"
<< "可借阅: " << std::boolalpha << book2.isAvailable << std::endl;
return 0;
}
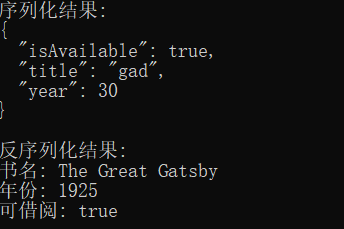
4.4 stream的序列化和反序列化
cpp
std::ifstream inputFile("input.json");
json inputJson;
inputFile >> inputJson;
std::ofstream outputFile("output.json");
outputFile << inputJson << std::endl;
4.5 任意类型转换
cpp
json j = { {"value", 42} };
int intValue = j["value"].get<int>(); // 显式类型转换
std::cout << "value = " << intValue << std::endl;
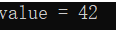
5 总结
nlohmann/json库是一个功能强大且简单易用的C++ JSON库。提供了丰富的API来解析、生成和操作JSON数据,并支持多种数据类型和高级功能。无论是处理简单的JSON数据还是复杂的JSON结构,nlohmann/json都能提供高效且方便的解决方案。