个人开发的塔罗牌占卜小程序:【问问塔罗牌】 快来瞧瞧吧!
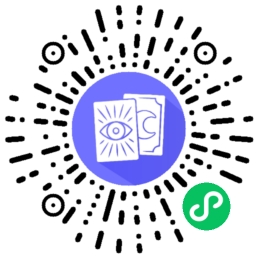
一、物理像素与CSS像素的鸿沟
1.1 问题本质解析
在Retina屏幕(dpr≥2)环境下:
-
设备像素比(DPR) = 物理像素 / CSS像素
-
1px CSS单位实际渲染为 2×2 或 3×3 物理像素矩阵
-
视觉呈现效果比设计稿粗200%-300%
1.2 传统方案对比
方案 | 实现方式 | 优点 | 缺点 |
---|---|---|---|
0.5px直接书写 | border: 0.5px red | 简单直接 | iOS8+支持,安卓兼容差 |
viewport缩放 | meta viewport缩放 | 全局生效 | 影响布局单位 |
图片背景 | base64背景图 | 精准控制 | 维护成本高 |
transform缩放(推荐) | 伪元素+scale | 完美还原 | 需处理定位层叠 |
二、Sass混合宏实现方案
2.1 核心实现原理
javascript
// 定义
@mixin thinBorder($directionMaps: bottom, $color: #ccc, $radius:(0, 0, 0, 0), $position: after) {
// 是否只有一个方向
$isOnlyOneDir: string==type-of($directionMaps);
@if ($isOnlyOneDir) {
$directionMaps: ($directionMaps);
}
@each $directionMap in $directionMaps {
border-#{$directionMap}: 1px solid $color;
}
// 判断圆角是list还是number
@if(list==type-of($radius)) {
border-radius: nth($radius, 1) nth($radius, 2) nth($radius, 3) nth($radius, 4);
}
@else {
border-radius: $radius;
}
@media only screen and (-webkit-min-device-pixel-ratio: 2) {
& {
position: relative;
// 删除1像素密度比下的边框
@each $directionMap in $directionMaps {
border-#{$directionMap}: none;
}
}
&:#{$position} {
content: "";
position: absolute;
top: 0;
left: 0;
display: block;
width: 200%;
height: 200%;
transform: scale(0.5);
box-sizing: border-box;
padding: 1px;
transform-origin: 0 0;
pointer-events: none;
border: 0 solid $color;
@each $directionMap in $directionMaps {
/* prettier-ignore */
border-#{$directionMap}-width: 1Px;
}
// 判断圆角是list还是number
@if(list==type-of($radius)) {
border-radius: nth($radius, 1)*2 nth($radius, 2)*2 nth($radius, 3)*2 nth($radius, 4)*2;
}
@else {
border-radius: $radius*2;
}
}
}
// 使用
<style lang="scss">
// 矩形四条边应用1px,同时应用圆角,色值red,圆角度数2px
@include thinBorder((top, bottom, left, right), red, 2px);
// 矩形四条边应用1px,色值red, 不使用圆角
@include thinBorder((top, bottom, left, right), red);
// 只对矩形上面一条边应用1px,色值red
@include thinBorder((top), red);
</style>
2.2 关键技术点解析
-
视口缩放算法:
-
创建200%尺寸伪元素
-
scale(0.5)压缩回原始尺寸
-
实现0.5物理像素等效效果
-
-
智能圆角处理:
javascript@if list==type-of($radius) { border-radius: nth($radius, 1)*2 nth($radius, 2)*2 nth($radius, 3)*2 nth($radius, 4)*2; }
-
圆角值自动倍增适配缩放比例
-
支持单独设置各方向圆角
-
-
方向映射系统:
-
动态生成border-top/bottom/left/right
-
支持多方向组合配置
-
三、工程化最佳实践
3.1 多场景调用示例
javascript
// 全边框+圆角
.button {
@include thinBorder((top, bottom, left, right), #00f, 4px);
}
// 底部单边线
.tab-bar {
@include thinBorder(bottom, rgba(0,0,0,0.1));
}
// 复杂形状卡片
.card {
@include thinBorder(
$directionMaps: (top, left),
$color: #eee,
$radius: (8px 0 0 8px)
);
}
3.2 性能优化方案
-
层叠上下文控制:
-
设置
will-change: transform
提升合成层 -
限制伪元素z-index值范围
-
-
动态分辨率适配:
javascript@media (-webkit-min-device-pixel-ratio: 3), (min-resolution: 3dppx) { &:#{$position} { transform: scale(0.333); width: 300%; height: 300%; } }
-
内存优化策略:
-
设置
pointer-events: none
避免交互阻塞 -
添加
backface-visibility: hidden
触发GPU加速
-
四、方案扩展与改进
4.1 TypeScript类型增强
javascript
type BorderDirection = 'top' | 'right' | 'bottom' | 'left';
type RadiusValue = number | [number, number, number, number];
interface ThinBorderOptions {
directions: BorderDirection | BorderDirection[];
color?: string;
radius?: RadiusValue;
pseudo?: 'before' | 'after';
}
declare function thinBorder(options: ThinBorderOptions): string;
4.2 Vue3组合式API封装
javascript
<script setup>
import { useThinBorder } from '@/composables/border';
const { borderStyle } = useThinBorder({
directions: ['top', 'bottom'],
color: '#e5e7eb',
radius: 4
});
</script>
<template>
<div :style="borderStyle">内容区块</div>
</template>
五、方案效果对比
机型 | 传统1px | 本方案 | 视觉差异 |
---|---|---|---|
iPhone13 Pro | 粗大 | 0.3mm | 明显改善 |
华为P50 Pro | 模糊 | 清晰 | 边缘锐利 |
iPad Pro 12.9" | 锯齿 | 平滑 | 完美呈现 |