Author:MTingle
major:人工智能
Build your hopes like a tower!
目录
一.项目简介
本项目实现了一个完整OJ项目所应具有的大部分功能.基于 B 端和 C 端特性,实现功能与业务的合理切分。在用户端,实现了竞赛列表展示、历史竞赛回顾、竞赛报名参与、在线刷题以及竞赛排名查看等功能.管理端则具备发布竞赛、发布题目以及对违规用户执行拉黑操作等功能。对于游客,系统开放了浏览题目和竞赛信息的权限,同时限制其参赛与做题操作,在保证信息公开的同时维护了系统的公平性与安全性。
二.开发技术
SpringBoot、SpringCloud、MyBatisPlus、Redis、RabbitMQ、ES引擎、Lombok、JWT、Docker、雪花算法等
三.测试用例设计(持续更新中)
登录界面
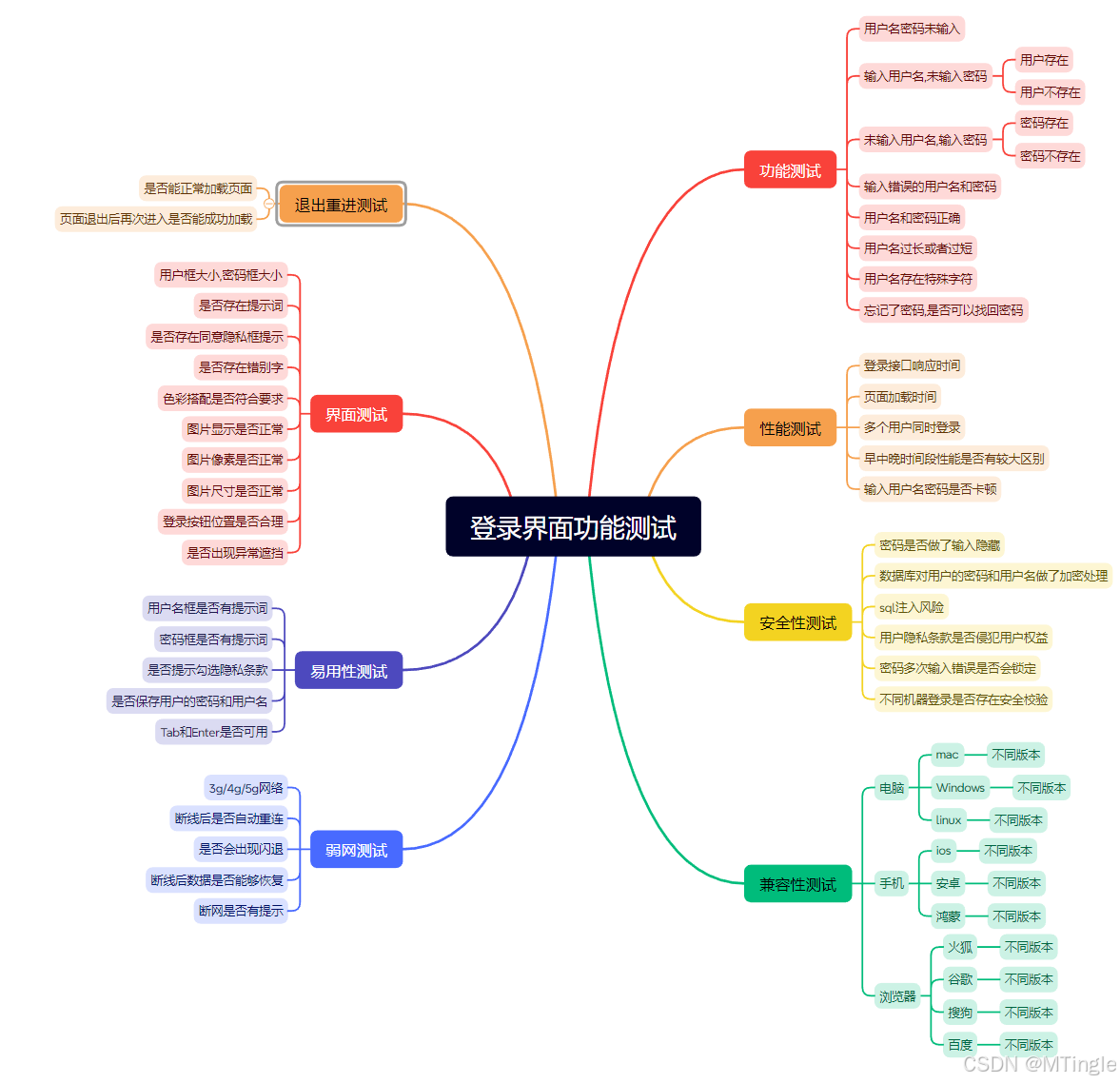
判题功能
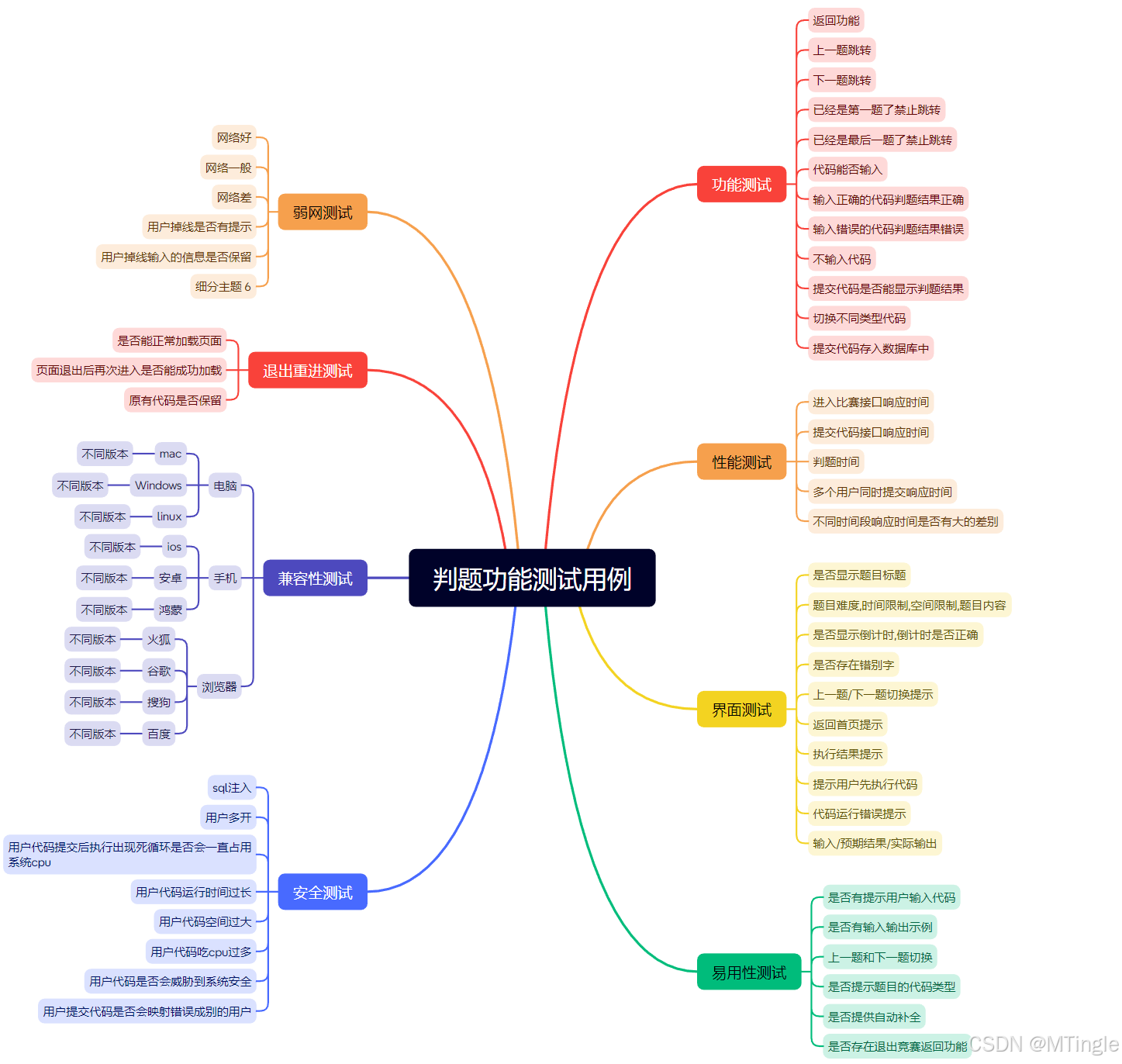
四.自动化测试代码(持续更新中)
管理端登录页面
java
package tests.sysuser;
import common.Utils;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import java.sql.Driver;
import java.time.Duration;
public class SysLoginPage extends Utils {
public static String url = "http://81.70.80.96:10030/oj/login";
public SysLoginPage() {
super(url);
}
public void loginPageRight(){
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.presenceOfElementLocated(By.cssSelector("#app > div > div.login-box > div.logo-box > div > div.sys-name")));
if (element!=null){
System.out.println("页面加载成功");
}else{
System.out.println("页面加载失败");
}
}
public void login() throws InterruptedException {
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
// 账号密码为空
wait.until(ExpectedConditions.elementToBeClickable(By.cssSelector("#app > div > div.login-box > div.form-box > div.submit-box"))).click();
// 用户名: #app > div > div.login-box > div.form-box > div:nth-child(1) > div > div > input
// 密码: #app > div > div.login-box > div.form-box > div:nth-child(2) > div > div > input
// 密码未输入
wait.until(ExpectedConditions.presenceOfElementLocated(By.cssSelector("#app > div > div.login-box > div.form-box > div:nth-child(1) > div > div > input"))).sendKeys("abc");
wait.until(ExpectedConditions.elementToBeClickable(By.cssSelector("#app > div > div.login-box > div.form-box > div.submit-box"))).click();
// 输入密码,但用户不存在
wait.until(ExpectedConditions.presenceOfElementLocated(By.cssSelector("#app > div > div.login-box > div.form-box > div:nth-child(2) > div > div > input"))).sendKeys("123");
wait.until(ExpectedConditions.elementToBeClickable(By.cssSelector("#app > div > div.login-box > div.form-box > div.submit-box"))).click();
// 输入存在用户,但是密码错误
wait.until(ExpectedConditions.presenceOfElementLocated(By.cssSelector("#app > div > div.login-box > div.form-box > div:nth-child(1) > div > div > input"))).clear();
wait.until(ExpectedConditions.presenceOfElementLocated(By.cssSelector("#app > div > div.login-box > div.form-box > div:nth-child(1) > div > div > input"))).sendKeys("admin");
wait.until(ExpectedConditions.elementToBeClickable(By.cssSelector("#app > div > div.login-box > div.form-box > div.submit-box"))).click();
// 输入sql注入
wait.until(ExpectedConditions.presenceOfElementLocated(By.cssSelector("#app > div > div.login-box > div.form-box > div:nth-child(1) > div > div > input"))).clear();
wait.until(ExpectedConditions.presenceOfElementLocated(By.cssSelector("#app > div > div.login-box > div.form-box > div:nth-child(1) > div > div > input"))).sendKeys("'OR '1'=''1");
wait.until(ExpectedConditions.elementToBeClickable(By.cssSelector("#app > div > div.login-box > div.form-box > div.submit-box"))).click();
// 正常登录
wait.until(ExpectedConditions.presenceOfElementLocated(By.cssSelector("#app > div > div.login-box > div.form-box > div:nth-child(1) > div > div > input"))).clear();
wait.until(ExpectedConditions.presenceOfElementLocated(By.cssSelector("#app > div > div.login-box > div.form-box > div:nth-child(2) > div > div > input"))).clear();
wait.until(ExpectedConditions.presenceOfElementLocated(By.cssSelector("#app > div > div.login-box > div.form-box > div:nth-child(1) > div > div > input"))).sendKeys("admin");
wait.until(ExpectedConditions.presenceOfElementLocated(By.cssSelector("#app > div > div.login-box > div.form-box > div:nth-child(2) > div > div > input"))).sendKeys("admin");
wait.until(ExpectedConditions.elementToBeClickable(By.cssSelector("#app > div > div.login-box > div.form-box > div.submit-box"))).click();
// 等待登录成功后的页面跳转
wait.until(ExpectedConditions.urlContains("/oj/layout/question"));
}
}
管理端用户界面
java
package tests.sysuser;
import common.Utils;
import org.openqa.selenium.By;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import java.time.Duration;
public class SysuserListPage extends Utils {
public static String url = "http://81.70.80.96:10030/oj/layout/question";
public SysuserListPage() {
super(url);
}
public void findUser() throws InterruptedException {
System.out.println("开始搜索用户");
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(3));
wait.until(ExpectedConditions.presenceOfElementLocated(By.cssSelector("#app")));
wait.until(ExpectedConditions.elementToBeClickable(By.cssSelector("#app > section > main > div.left > aside > ul > li:nth-child(1) > span")));
driver.findElement(By.cssSelector("#app > section > main > div.left > aside > ul > li:nth-child(1) > span")).click();
wait.until(ExpectedConditions.presenceOfElementLocated(By.cssSelector("#app > section > main > div.right > form > div:nth-child(2) > div > div > div > input")));
driver.findElement(By.cssSelector("#app > section > main > div.right > form > div:nth-child(2) > div > div > div > input"))
.sendKeys("乐");
System.out.println("输入用户完成,点击搜索");
wait.until(ExpectedConditions.elementToBeClickable(By.cssSelector("#app > section > main > div.right > form > div:nth-child(3) > div > button:nth-child(1) > span")));
driver.findElement(By.cssSelector("#app > section > main > div.right > form > div:nth-child(3) > div > button:nth-child(1) > span"))
.click();
System.out.println("用户搜索完成");
Thread.sleep(5);
}
public void blockAndRecover() throws InterruptedException {
System.out.println("开始执行用户拉黑");
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(10));
// 等待表格加载完成
wait.until(ExpectedConditions.presenceOfElementLocated(By.cssSelector("#app > section > main > div.right > div.el-table--fit.el-table--scrollable-x.el-table--enable-row-transition.el-table.el-table--layout-fixed.is-scrolling-left > div.el-table__inner-wrapper > div.el-table__body-wrapper > div > div.el-scrollbar__wrap.el-scrollbar__wrap--hidden-default > div > table > tbody > tr:nth-child(1) > td.el-table_2_column_16.el-table-fixed-column--right.is-first-column.el-table__cell > div")))
.click();
// // 等待按钮可点击
// Thread.sleep(5);
System.out.println("执行用户解禁");
Thread.sleep(5);
// 等待按钮再次可点击
driver.findElement(By.cssSelector("#app > section > main > div.right > div.el-table--fit.el-table--scrollable-x.el-table--enable-row-transition.el-table.el-table--layout-fixed.is-scrolling-left > div.el-table__inner-wrapper > div.el-table__body-wrapper > div > div.el-scrollbar__wrap.el-scrollbar__wrap--hidden-default > div > table > tbody > tr:nth-child(1) > td.el-table_2_column_16.el-table-fixed-column--right.is-first-column.el-table__cell > div > button > span"))
.click();
Thread.sleep(5);
}
}
main函数
java
package tests;
import tests.sysuser.SysLoginPage;
import tests.sysuser.SysuserListPage;
public class RunTests {
public static void main(String[] args) throws InterruptedException {
SysLoginPage sysLoginPage = new SysLoginPage();
sysLoginPage.loginPageRight();
sysLoginPage.login();
SysuserListPage userListPage = new SysuserListPage();
userListPage.findUser();
userListPage.blockAndRecover();
sysLoginPage.quit();
}
}
五.性能测试报告
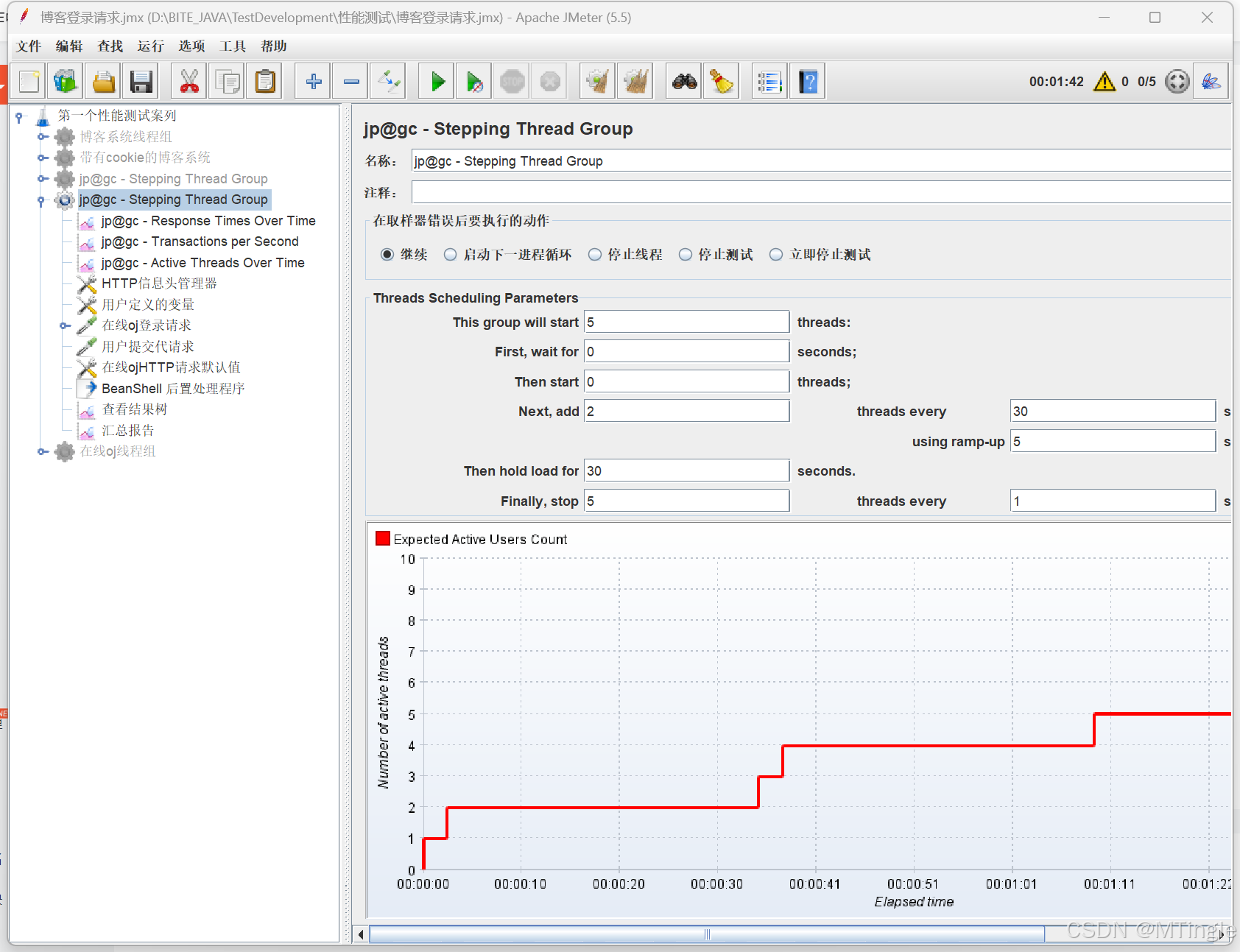
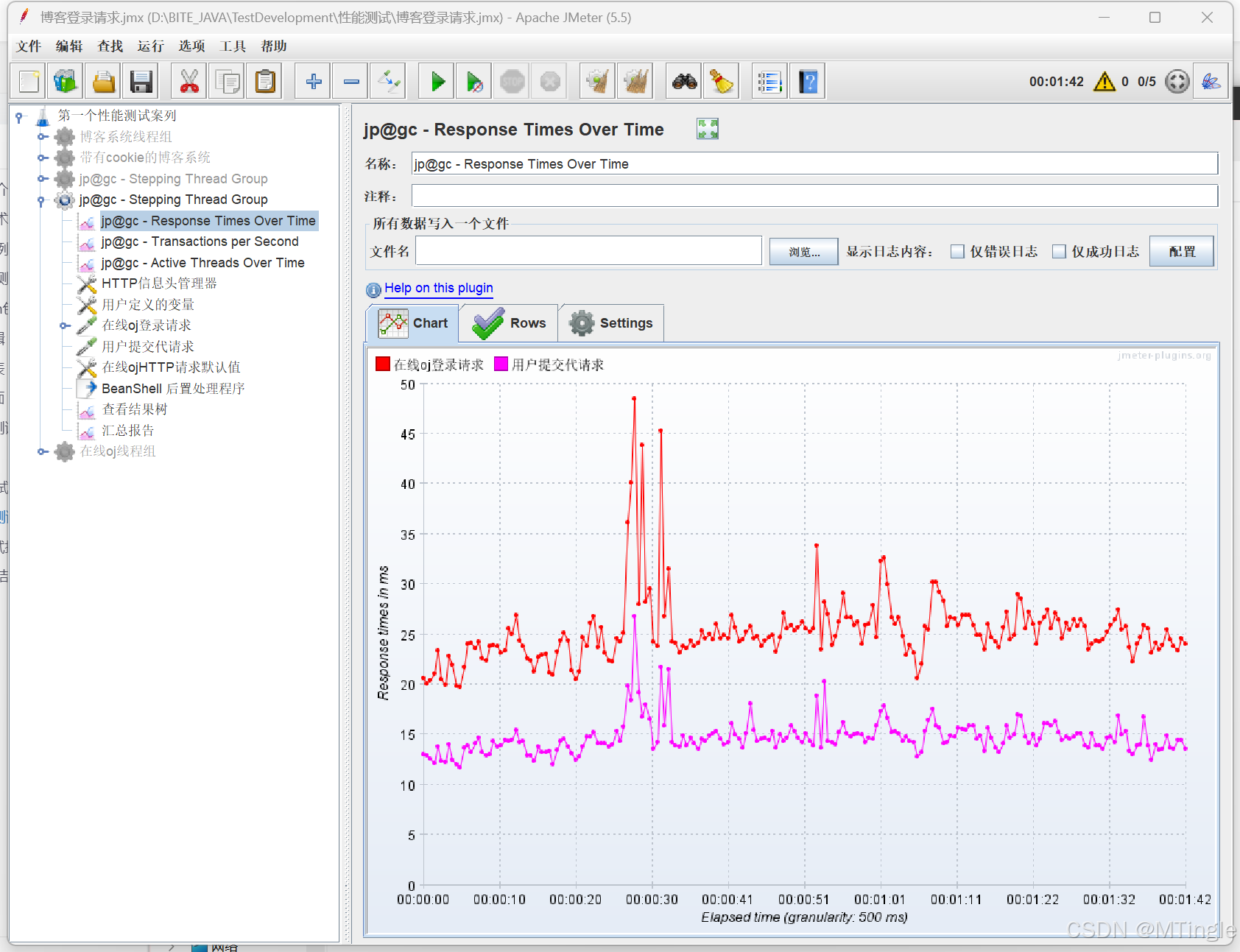
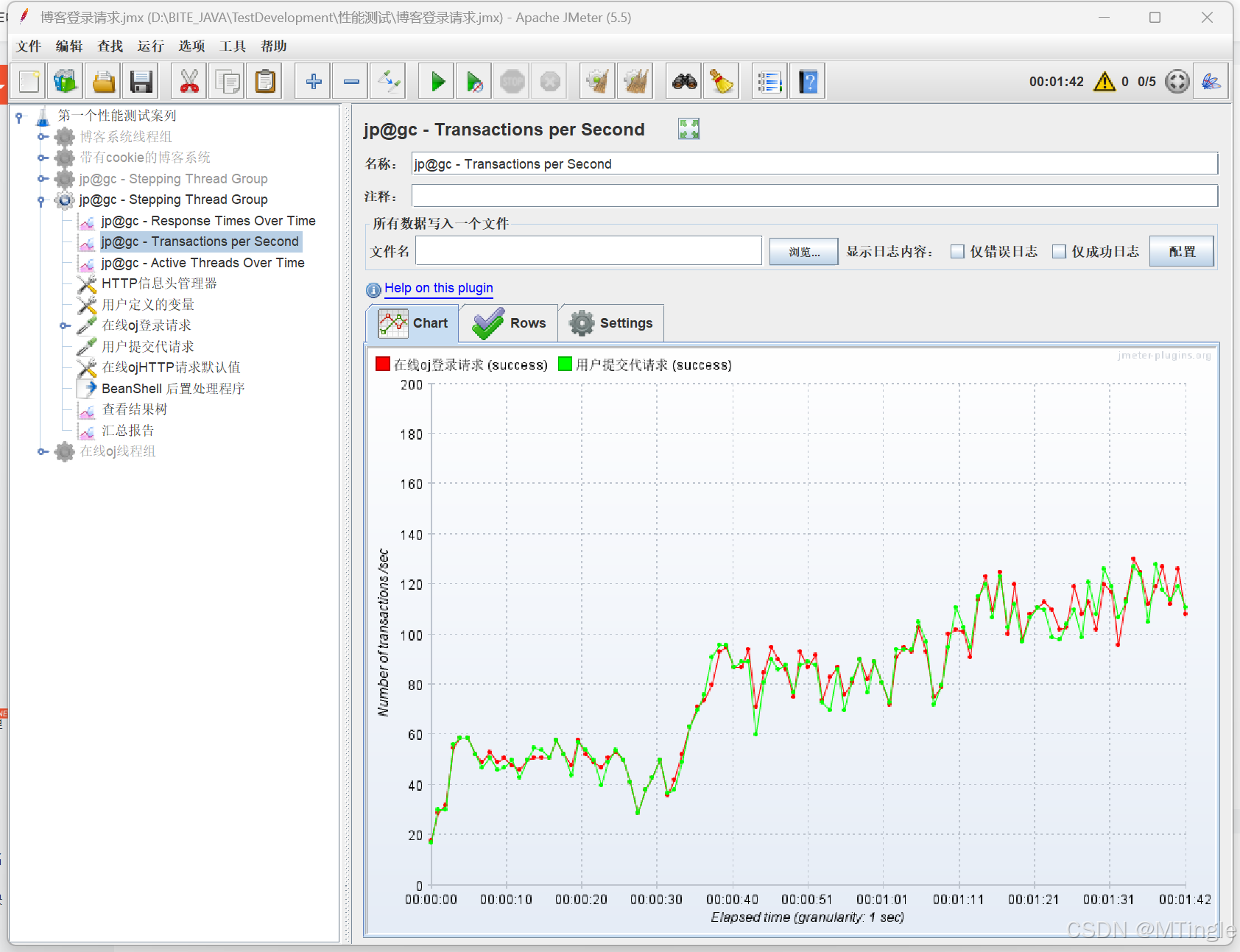
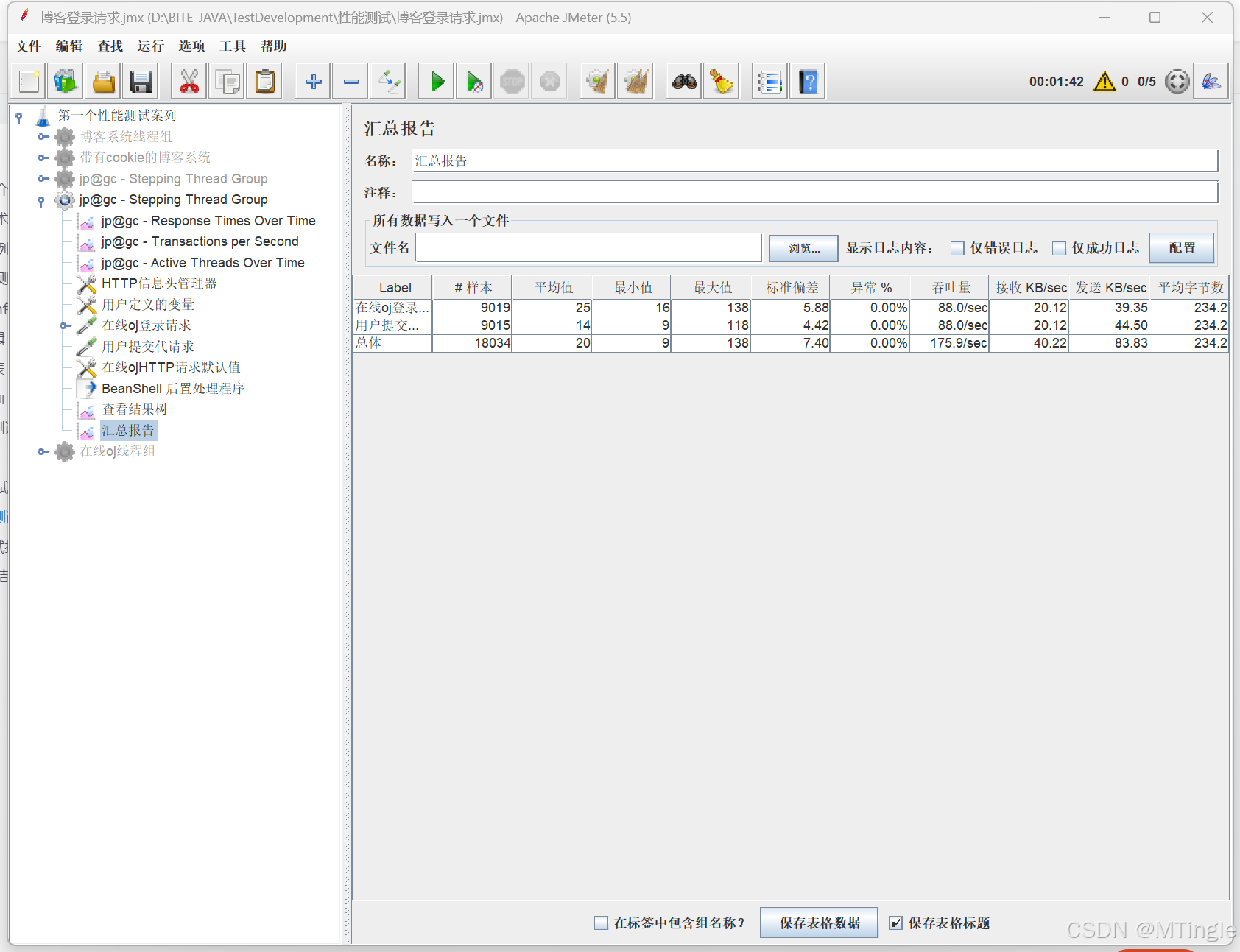
总体情况
报告展示了使用 Apache JMeter 进行性能测试的结果。测试涉及 "在线用户登录" 和 "用户提交代码" 等操作,整体样本数总计 18034 个,无异常情况(异常 % 为 0.00%) ,吞吐量总体为 175.9/sec,说明系统在测试期间平均每秒能处理 175.9 个请求。
具体指标分析
- 响应时间
- 在线用户登录:平均值 25ms,最小值 16ms,最大值 138ms ,标准偏差 5.88 。表明多数登录请求响应较快,但存在个别稍长时间的响应。
- 用户提交代码:平均值 14ms,最小值 9ms,最大值 118ms ,标准偏差 4.42 。整体响应时间比登录稍短,也有一定响应时间波动。
- 数据传输
- 接收数据:"在线用户登录" 和 "用户提交" 接收速率均为 20.12KB/sec ,总体 40.22KB/sec ,说明接收数据速度稳定。
- 发送数据:"在线用户登录" 39.35KB/sec,"用户提交" 44.50KB/sec ,总体 83.83KB/sec ,发送数据量上 "用户提交" 稍高。
- 平均字节数:两者均为 234.2 ,说明每个请求或响应数据包大小相对固定。