string类
- 1.为什么要学string?
- 2.标准库类型的string类
-
- [2.1 string类的构造](#2.1 string类的构造)
- 2.2string类的析构
- 2.3读写string类
- 2.4string类的赋值重载
- 2.5string的遍历
1.为什么要学string?
在C语言中字符出串是以'/0'结尾的一些字符的结合,为了操作方便,C标准库中提供了一些str类库函数,但是这些库函数与字符串时分隔开的,不符合OOP(面向对象编程)的思想,并且底层空间需要用户自己管理,可能会越界访问。
2.标准库类型的string类
标准库类型string表示可变长的字符序列,使用string类型必须包含string头文件。作为标准库的一部分,string定义在命名空间std中。
cpp
#include<string>
using namespace std;
2.1 string类的构造
- string类的构造
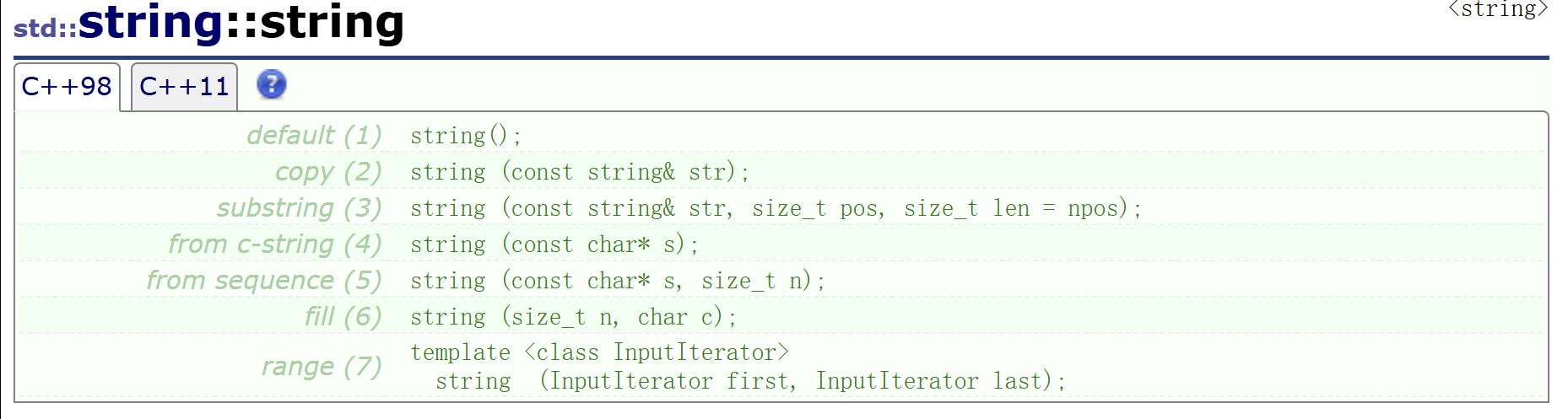
string常见的构造
cpp
#include<iostream>
#include<string>
using namespace std;
//class string
//{
// char* _str;
// size_t _size;
// size_t _capaicty;
//};//底层实现
int main()
{
string s1; //无参的构造
string s2("hello wrold"); //带参的构造
string s3 = "hello world"; //带参的构造
string s4(s3); //拷贝构造
string s5(10, '#'); //多个字符构造
return 0;
}
2.2string类的析构

string类的析构出了作用域自动调用,把string内的资源进行释放。
2.3读写string类
cpp
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s; //空字符串
cin >> s; //将string对象读入s
cout << s << endl; //输出s
return 0;
}
2.4string类的赋值重载

cpp
#include <iostream>
#include <string>
int main()
{
std::string str1, str2, str3;
str1 = "Test string: "; // c-string
str2 = 'x'; // single character
str3 = str1 + str2; // string
std::cout << str3 << '\n';
return 0;
}
2.5string的遍历

cpp
#include<iostream>
#include<string>
#include<vector>
#include<list>
using namespace std;
void test_string()
{
string s1("hello world");
s1[0]++;
cout << s1 << endl;
s1[0] = 'x';
cout << s1 << endl;
// 遍历1
// 下标+[]
for (size_t i = 0; i < s1.size(); i++)
{
s1[i]++;
}
cout << s1 << endl;
for (size_t i = 0; i < s1.size(); i++)
{
cout << s1[i] << " ";
}
cout << endl;
cout << s1.size() << endl;
//遍历2
//迭代器
string::iterator it = s1.begin();
while (it != s1.end())
{
(*it)--;
++it;
}
cout << endl;
it = s1.begin();
while (it != s1.end())
{
cout << *it << " ";
++it;
}
cout << endl;
}
void test_string2()
{
string s("hello world");
vector<int> v;
v.push_back(1);
v.push_back(2);
v.push_back(3);
v.push_back(4);
list<int> lt;
lt.push_back(10);
lt.push_back(20);
lt.push_back(30);
lt.push_back(40);
reverse(s.begin(), s.end());
reverse(v.begin(), v.end());
reverse(lt.begin(), lt.end());
for (auto& e : s)
{
e--;
}
//for (auto e : s)
for (char e : s)
{
cout << e << " ";
}
cout << endl;
char x = 'a';
// for (auto x : v)
for (int x : v)
{
cout << x << " ";
}
cout << endl;
for (auto e : lt)
{
cout << e << " ";
}
cout << endl;
}
int main()
{
return 0;
}