文章目录
-
- 第一部分:概述与基础准备
-
- [1.1 游戏辅助工具的定义与用途](#1.1 游戏辅助工具的定义与用途)
- [1.2 开发环境准备](#1.2 开发环境准备)
- [1.3 项目创建与配置](#1.3 项目创建与配置)
- 第二部分:核心功能实现
-
- [2.1 屏幕点击功能实现](#2.1 屏幕点击功能实现)
-
- [2.1.1 基础点击功能](#2.1.1 基础点击功能)
- [2.1.2 多点触控实现](#2.1.2 多点触控实现)
- [2.2 滑动功能实现](#2.2 滑动功能实现)
-
- [2.2.1 基础滑动功能](#2.2.1 基础滑动功能)
- [2.2.2 曲线滑动实现](#2.2.2 曲线滑动实现)
- [2.3 屏幕颜色检测功能](#2.3 屏幕颜色检测功能)
-
- [2.3.1 基础颜色检测](#2.3.1 基础颜色检测)
- [2.3.2 区域颜色检测](#2.3.2 区域颜色检测)
- [2.4 图像识别功能实现](#2.4 图像识别功能实现)
-
- [2.4.1 基础图像识别](#2.4.1 基础图像识别)
- [2.4.2 多目标图像识别](#2.4.2 多目标图像识别)
- 第三部分:辅助服务与UI实现
-
- [3.1 无障碍服务实现](#3.1 无障碍服务实现)
- [3.2 悬浮窗控制界面](#3.2 悬浮窗控制界面)
- [3.3 主活动实现](#3.3 主活动实现)
- 第四部分:高级功能与优化
-
- [4.1 脚本录制与回放](#4.1 脚本录制与回放)
- [4.2 条件触发与自动化](#4.2 条件触发与自动化)
- [4.3 性能优化与多线程处理](#4.3 性能优化与多线程处理)
- 第五部分:测试与部署
-
- [5.1 功能测试方案](#5.1 功能测试方案)
- [5.2 性能测试与优化](#5.2 性能测试与优化)
- [5.3 应用打包与发布注意事项](#5.3 应用打包与发布注意事项)
- 第六部分:安全与伦理考虑
-
- [6.1 合法使用建议](#6.1 合法使用建议)
- [6.2 防滥用机制](#6.2 防滥用机制)
- [6.3 用户教育与责任](#6.3 用户教育与责任)
- 第七部分:扩展功能与未来方向
-
- [7.1 机器学习增强](#7.1 机器学习增强)
- [7.2 云同步与配置共享](#7.2 云同步与配置共享)
- [7.3 社区与用户自定义](#7.3 社区与用户自定义)
- 第八部分:总结与完整代码整合
-
- [8.1 项目结构总结](#8.1 项目结构总结)
- [8.2 关键类交互关系](#8.2 关键类交互关系)
- [8.3 完整代码整合与使用示例](#8.3 完整代码整合与使用示例)
- 第九部分:常见问题与解决方案
-
- [9.1 权限问题处理](#9.1 权限问题处理)
- [9.2 兼容性问题](#9.2 兼容性问题)
- [9.3 调试技巧](#9.3 调试技巧)
- 第十部分:未来发展与进阶学习
-
- [10.1 进阶功能方向](#10.1 进阶功能方向)
- [10.2 学习资源推荐](#10.2 学习资源推荐)
- [10.3 社区与贡献](#10.3 社区与贡献)
- 结语
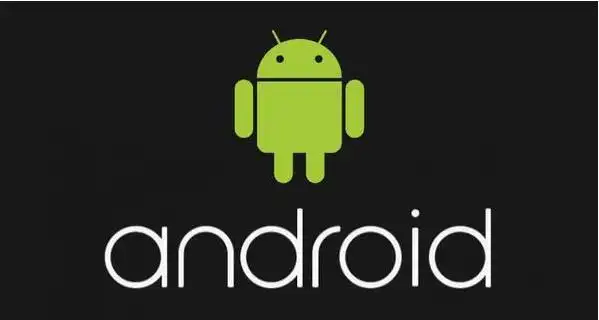
第一部分:概述与基础准备
1.1 游戏辅助工具的定义与用途
游戏辅助工具是一种能够帮助玩家自动化执行某些游戏操作或提供额外游戏信息的软件。这类工具通常可以实现以下功能:
- 自动点击屏幕特定位置
- 模拟滑动操作
- 检测屏幕特定位置的颜色
- 识别屏幕上的特定图像
- 自动执行重复性任务
1.2 开发环境准备
在开始开发前,我们需要准备以下环境:
- Android Studio:官方推荐的Android开发IDE
- Java Development Kit (JDK):建议使用JDK 8或更高版本
- Android设备或模拟器:用于测试辅助工具
- ADB工具:用于调试和连接设备
1.3 项目创建与配置
-
在Android Studio中创建新项目,选择"Empty Activity"模板
-
配置项目基本信息:
- 应用名称:GameAssistant
- 包名:com.example.gameassistant
- 最低API级别:建议API 21(Android 5.0)以上
-
在
AndroidManifest.xml
中添加必要权限:
xml
<uses-permission android:name="android.permission.ACCESSIBILITY_SERVICE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.FOREGROUND_SERVICE" />
<uses-permission android:name="android.permission.SYSTEM_ALERT_WINDOW" />
第二部分:核心功能实现
2.1 屏幕点击功能实现
2.1.1 基础点击功能
java
public class TouchUtils {
private static final String TAG = "TouchUtils";
/**
* 模拟点击屏幕指定位置
* @param x 点击的X坐标
* @param y 点击的Y坐标
* @return 是否执行成功
*/
public static boolean tap(int x, int y) {
try {
// 创建点击手势
GestureDescription.Builder builder = new GestureDescription.Builder();
Path path = new Path();
path.moveTo(x, y);
builder.addStroke(new GestureDescription.StrokeDescription(path, 0, 50));
// 执行手势
AccessibilityService service = GameAssistantService.getInstance();
if (service != null) {
return service.dispatchGesture(builder.build(), null, null);
}
} catch (Exception e) {
Log.e(TAG, "Tap error: " + e.getMessage());
}
return false;
}
/**
* 模拟长按屏幕指定位置
* @param x 点击的X坐标
* @param y 点击的Y坐标
* @param duration 长按持续时间(毫秒)
* @return 是否执行成功
*/
public static boolean longTap(int x, int y, int duration) {
try {
GestureDescription.Builder builder = new GestureDescription.Builder();
Path path = new Path();
path.moveTo(x, y);
builder.addStroke(new GestureDescription.StrokeDescription(path, 0, duration));
AccessibilityService service = GameAssistantService.getInstance();
if (service != null) {
return service.dispatchGesture(builder.build(), null, null);
}
} catch (Exception e) {
Log.e(TAG, "Long tap error: " + e.getMessage());
}
return false;
}
}
2.1.2 多点触控实现
java
public class MultiTouchUtils {
/**
* 模拟多点触控
* @param points 触摸点数组,每个元素包含x,y坐标
* @param duration 触摸持续时间(毫秒)
* @return 是否执行成功
*/
public static boolean multiTap(Point[] points, int duration) {
if (points == null || points.length == 0) {
return false;
}
try {
GestureDescription.Builder builder = new GestureDescription.Builder();
for (Point point : points) {
Path path = new Path();
path.moveTo(point.x, point.y);
builder.addStroke(new GestureDescription.StrokeDescription(path, 0, duration));
}
AccessibilityService service = GameAssistantService.getInstance();
if (service != null) {
return service.dispatchGesture(builder.build(), null, null);
}
} catch (Exception e) {
Log.e("MultiTouchUtils", "Multi tap error: " + e.getMessage());
}
return false;
}
}
2.2 滑动功能实现
2.2.1 基础滑动功能
java
public class SwipeUtils {
/**
* 模拟从起点滑动到终点
* @param startX 起始X坐标
* @param startY 起始Y坐标
* @param endX 结束X坐标
* @param endY 结束Y坐标
* @param duration 滑动持续时间(毫秒)
* @return 是否执行成功
*/
public static boolean swipe(int startX, int startY, int endX, int endY, int duration) {
try {
GestureDescription.Builder builder = new GestureDescription.Builder();
Path path = new Path();
path.moveTo(startX, startY);
path.lineTo(endX, endY);
builder.addStroke(new GestureDescription.StrokeDescription(path, 0, duration));
AccessibilityService service = GameAssistantService.getInstance();
if (service != null) {
return service.dispatchGesture(builder.build(), null, null);
}
} catch (Exception e) {
Log.e("SwipeUtils", "Swipe error: " + e.getMessage());
}
return false;
}
/**
* 模拟复杂滑动路径
* @param points 路径点数组
* @param duration 滑动持续时间(毫秒)
* @return 是否执行成功
*/
public static boolean swipePath(Point[] points, int duration) {
if (points == null || points.length < 2) {
return false;
}
try {
GestureDescription.Builder builder = new GestureDescription.Builder();
Path path = new Path();
path.moveTo(points[0].x, points[0].y);
for (int i = 1; i < points.length; i++) {
path.lineTo(points[i].x, points[i].y);
}
builder.addStroke(new GestureDescription.StrokeDescription(path, 0, duration));
AccessibilityService service = GameAssistantService.getInstance();
if (service != null) {
return service.dispatchGesture(builder.build(), null, null);
}
} catch (Exception e) {
Log.e("SwipeUtils", "Swipe path error: " + e.getMessage());
}
return false;
}
}
2.2.2 曲线滑动实现
java
public class CurveSwipeUtils {
/**
* 模拟贝塞尔曲线滑动
* @param startX 起始X坐标
* @param startY 起始Y坐标
* @param controlX 控制点X坐标
* @param controlY 控制点Y坐标
* @param endX 结束X坐标
* @param endY 结束Y坐标
* @param duration 滑动持续时间(毫秒)
* @return 是否执行成功
*/
public static boolean bezierSwipe(int startX, int startY,
int controlX, int controlY,
int endX, int endY,
int duration) {
try {
GestureDescription.Builder builder = new GestureDescription.Builder();
Path path = new Path();
path.moveTo(startX, startY);
path.quadTo(controlX, controlY, endX, endY);
builder.addStroke(new GestureDescription.StrokeDescription(path, 0, duration));
AccessibilityService service = GameAssistantService.getInstance();
if (service != null) {
return service.dispatchGesture(builder.build(), null, null);
}
} catch (Exception e) {
Log.e("CurveSwipeUtils", "Bezier swipe error: " + e.getMessage());
}
return false;
}
}
2.3 屏幕颜色检测功能
2.3.1 基础颜色检测
java
public class ColorDetector {
/**
* 获取屏幕指定位置的颜色值
* @param x X坐标
* @param y Y坐标
* @return 颜色值(ARGB格式)
*/
public static int getColorAtPoint(int x, int y) {
try {
AccessibilityService service = GameAssistantService.getInstance();
if (service == null) {
return 0;
}
// 获取屏幕截图
AccessibilityService.ScreenshotResult screenshot =
service.takeScreenshot(
service.getRootInActiveWindow(),
service.getConnectionId()
);
if (screenshot == null) {
return 0;
}
Bitmap bitmap = Bitmap.wrapHardwareBuffer(
screenshot.getHardwareBuffer(),
screenshot.getColorSpace()
);
if (bitmap == null) {
return 0;
}
// 检查坐标是否在有效范围内
if (x < 0 || y < 0 || x >= bitmap.getWidth() || y >= bitmap.getHeight()) {
return 0;
}
// 获取指定位置的颜色
int color = bitmap.getPixel(x, y);
bitmap.recycle();
return color;
} catch (Exception e) {
Log.e("ColorDetector", "Get color error: " + e.getMessage());
return 0;
}
}
/**
* 比较指定位置的颜色与目标颜色是否匹配
* @param x X坐标
* @param y Y坐标
* @param targetColor 目标颜色(ARGB格式)
* @param tolerance 容差值(0-255)
* @return 是否匹配
*/
public static boolean compareColor(int x, int y, int targetColor, int tolerance) {
int currentColor = getColorAtPoint(x, y);
if (currentColor == 0) {
return false;
}
int alphaDiff = Math.abs(Color.alpha(currentColor) - Color.alpha(targetColor));
int redDiff = Math.abs(Color.red(currentColor) - Color.red(targetColor));
int greenDiff = Math.abs(Color.green(currentColor) - Color.green(targetColor));
int blueDiff = Math.abs(Color.blue(currentColor) - Color.blue(targetColor));
return alphaDiff <= tolerance &&
redDiff <= tolerance &&
greenDiff <= tolerance &&
blueDiff <= tolerance;
}
}
2.3.2 区域颜色检测
java
public class AreaColorDetector {
/**
* 检测指定区域内是否存在目标颜色
* @param left 区域左边界
* @param top 区域上边界
* @param right 区域右边界
* @param bottom 区域下边界
* @param targetColor 目标颜色
* @param tolerance 容差值
* @return 是否找到目标颜色
*/
public static boolean detectColorInArea(int left, int top,
int right, int bottom,
int targetColor,
int tolerance) {
try {
AccessibilityService service = GameAssistantService.getInstance();
if (service == null) {
return false;
}
AccessibilityService.ScreenshotResult screenshot =
service.takeScreenshot(
service.getRootInActiveWindow(),
service.getConnectionId()
);
if (screenshot == null) {
return false;
}
Bitmap bitmap = Bitmap.wrapHardwareBuffer(
screenshot.getHardwareBuffer(),
screenshot.getColorSpace()
);
if (bitmap == null) {
return false;
}
// 调整边界确保在图片范围内
left = Math.max(0, left);
top = Math.max(0, top);
right = Math.min(bitmap.getWidth() - 1, right);
bottom = Math.min(bitmap.getHeight() - 1, bottom);
for (int y = top; y <= bottom; y++) {
for (int x = left; x <= right; x++) {
int color = bitmap.getPixel(x, y);
int alphaDiff = Math.abs(Color.alpha(color) - Color.alpha(targetColor));
int redDiff = Math.abs(Color.red(color) - Color.red(targetColor));
int greenDiff = Math.abs(Color.green(color) - Color.green(targetColor));
int blueDiff = Math.abs(Color.blue(color) - Color.blue(targetColor));
if (alphaDiff <= tolerance &&
redDiff <= tolerance &&
greenDiff <= tolerance &&
blueDiff <= tolerance) {
bitmap.recycle();
return true;
}
}
}
bitmap.recycle();
} catch (Exception e) {
Log.e("AreaColorDetector", "Detect color in area error: " + e.getMessage());
}
return false;
}
/**
* 在指定区域内查找所有匹配目标颜色的位置
* @param left 区域左边界
* @param top 区域上边界
* @param right 区域右边界
* @param bottom 区域下边界
* @param targetColor 目标颜色
* @param tolerance 容差值
* @return 匹配位置的列表
*/
public static List<Point> findAllColorPositions(int left, int top,
int right, int bottom,
int targetColor,
int tolerance) {
List<Point> positions = new ArrayList<>();
try {
AccessibilityService service = GameAssistantService.getInstance();
if (service == null) {
return positions;
}
AccessibilityService.ScreenshotResult screenshot =
service.takeScreenshot(
service.getRootInActiveWindow(),
service.getConnectionId()
);
if (screenshot == null) {
return positions;
}
Bitmap bitmap = Bitmap.wrapHardwareBuffer(
screenshot.getHardwareBuffer(),
screenshot.getColorSpace()
);
if (bitmap == null) {
return positions;
}
// 调整边界确保在图片范围内
left = Math.max(0, left);
top = Math.max(0, top);
right = Math.min(bitmap.getWidth() - 1, right);
bottom = Math.min(bitmap.getHeight() - 1, bottom);
for (int y = top; y <= bottom; y++) {
for (int x = left; x <= right; x++) {
int color = bitmap.getPixel(x, y);
int alphaDiff = Math.abs(Color.alpha(color) - Color.alpha(targetColor));
int redDiff = Math.abs(Color.red(color) - Color.red(targetColor));
int greenDiff = Math.abs(Color.green(color) - Color.green(targetColor));
int blueDiff = Math.abs(Color.blue(color) - Color.blue(targetColor));
if (alphaDiff <= tolerance &&
redDiff <= tolerance &&
greenDiff <= tolerance &&
blueDiff <= tolerance) {
positions.add(new Point(x, y));
}
}
}
bitmap.recycle();
} catch (Exception e) {
Log.e("AreaColorDetector", "Find all color positions error: " + e.getMessage());
}
return positions;
}
}
2.4 图像识别功能实现
2.4.1 基础图像识别
java
public class ImageRecognizer {
/**
* 在屏幕中查找目标图像
* @param template 目标图像(Bitmap)
* @param threshold 匹配阈值(0-1)
* @return 匹配位置,未找到返回null
*/
public static Point findImage(Bitmap template, float threshold) {
try {
AccessibilityService service = GameAssistantService.getInstance();
if (service == null || template == null) {
return null;
}
// 获取屏幕截图
AccessibilityService.ScreenshotResult screenshot =
service.takeScreenshot(
service.getRootInActiveWindow(),
service.getConnectionId()
);
if (screenshot == null) {
return null;
}
Bitmap screenBitmap = Bitmap.wrapHardwareBuffer(
screenshot.getHardwareBuffer(),
screenshot.getColorSpace()
);
if (screenBitmap == null) {
return null;
}
// 转换为灰度图像提高匹配效率
Mat screenMat = new Mat();
Utils.bitmapToMat(screenBitmap, screenMat);
Imgproc.cvtColor(screenMat, screenMat, Imgproc.COLOR_RGB2GRAY);
Mat templateMat = new Mat();
Utils.bitmapToMat(template, templateMat);
Imgproc.cvtColor(templateMat, templateMat, Imgproc.COLOR_RGB2GRAY);
// 模板匹配
int resultCols = screenMat.cols() - templateMat.cols() + 1;
int resultRows = screenMat.rows() - templateMat.rows() + 1;
Mat result = new Mat(resultRows, resultCols, CvType.CV_32FC1);
Imgproc.matchTemplate(screenMat, templateMat, result, Imgproc.TM_CCOEFF_NORMED);
Core.MinMaxLocResult mmr = Core.minMaxLoc(result);
Point matchLoc = mmr.maxLoc;
// 检查匹配度是否超过阈值
if (mmr.maxVal >= threshold) {
return new Point((int)matchLoc.x + templateMat.cols() / 2,
(int)matchLoc.y + templateMat.rows() / 2);
}
screenMat.release();
templateMat.release();
result.release();
screenBitmap.recycle();
} catch (Exception e) {
Log.e("ImageRecognizer", "Find image error: " + e.getMessage());
}
return null;
}
}
2.4.2 多目标图像识别
java
public class MultiImageRecognizer {
/**
* 在屏幕中查找所有匹配目标图像的位置
* @param template 目标图像(Bitmap)
* @param threshold 匹配阈值(0-1)
* @return 匹配位置列表
*/
public static List<Point> findAllImages(Bitmap template, float threshold) {
List<Point> positions = new ArrayList<>();
try {
AccessibilityService service = GameAssistantService.getInstance();
if (service == null || template == null) {
return positions;
}
// 获取屏幕截图
AccessibilityService.ScreenshotResult screenshot =
service.takeScreenshot(
service.getRootInActiveWindow(),
service.getConnectionId()
);
if (screenshot == null) {
return positions;
}
Bitmap screenBitmap = Bitmap.wrapHardwareBuffer(
screenshot.getHardwareBuffer(),
screenshot.getColorSpace()
);
if (screenBitmap == null) {
return positions;
}
// 转换为灰度图像
Mat screenMat = new Mat();
Utils.bitmapToMat(screenBitmap, screenMat);
Imgproc.cvtColor(screenMat, screenMat, Imgproc.COLOR_RGB2GRAY);
Mat templateMat = new Mat();
Utils.bitmapToMat(template, templateMat);
Imgproc.cvtColor(templateMat, templateMat, Imgproc.COLOR_RGB2GRAY);
// 模板匹配
int resultCols = screenMat.cols() - templateMat.cols() + 1;
int resultRows = screenMat.rows() - templateMat.rows() + 1;
Mat result = new Mat(resultRows, resultCols, CvType.CV_32FC1);
Imgproc.matchTemplate(screenMat, templateMat, result, Imgproc.TM_CCOEFF_NORMED);
// 查找所有超过阈值的匹配位置
Mat thresholdMat = new Mat();
Core.compare(result, new Scalar(threshold), thresholdMat, Core.CMP_GE);
MatOfPoint matches = new MatOfPoint();
Mat nonzero = new Mat();
Core.findNonZero(thresholdMat, nonzero);
if (nonzero.rows() > 0) {
matches = new MatOfPoint(nonzero);
for (int i = 0; i < matches.rows(); i++) {
double[] point = matches.get(i, 0);
int x = (int)point[0] + templateMat.cols() / 2;
int y = (int)point[1] + templateMat.rows() / 2;
positions.add(new Point(x, y));
}
}
// 释放资源
screenMat.release();
templateMat.release();
result.release();
thresholdMat.release();
matches.release();
nonzero.release();
screenBitmap.recycle();
} catch (Exception e) {
Log.e("MultiImageRecognizer", "Find all images error: " + e.getMessage());
}
return positions;
}
}
第三部分:辅助服务与UI实现
3.1 无障碍服务实现
java
public class GameAssistantService extends AccessibilityService {
private static GameAssistantService instance;
@Override
public void onCreate() {
super.onCreate();
instance = this;
}
@Override
public void onDestroy() {
super.onDestroy();
instance = null;
}
public static GameAssistantService getInstance() {
return instance;
}
@Override
public void onAccessibilityEvent(AccessibilityEvent event) {
// 处理无障碍事件
}
@Override
public void onInterrupt() {
// 服务中断处理
}
@Override
protected void onServiceConnected() {
super.onServiceConnected();
// 服务连接成功后的初始化
}
/**
* 获取屏幕截图
* @param window 目标窗口
* @param connectionId 连接ID
* @return 截图结果
*/
public ScreenshotResult takeScreenshot(AccessibilityWindowInfo window, int connectionId) {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.R) {
return takeScreenshot(window,
new Handler(getMainLooper()),
new ScreenshotCallback() {
@Override
public void onSuccess(ScreenshotResult screenshot) {
// 截图成功回调
}
@Override
public void onFailure(int errorCode) {
// 截图失败回调
}
});
}
return null;
}
}
3.2 悬浮窗控制界面
java
public class FloatingControlWindow extends FrameLayout {
private ImageView dragHandle;
private LinearLayout controlPanel;
private boolean isPanelExpanded = false;
public FloatingControlWindow(Context context) {
super(context);
initView(context);
}
private void initView(Context context) {
LayoutInflater.from(context).inflate(R.layout.floating_control, this);
dragHandle = findViewById(R.id.drag_handle);
controlPanel = findViewById(R.id.control_panel);
// 初始状态只显示拖动把手
controlPanel.setVisibility(GONE);
// 拖动把手点击事件
dragHandle.setOnClickListener(v -> {
isPanelExpanded = !isPanelExpanded;
controlPanel.setVisibility(isPanelExpanded ? VISIBLE : GONE);
});
// 拖动功能
dragHandle.setOnTouchListener(new OnTouchListener() {
private int initialX, initialY;
private float initialTouchX, initialTouchY;
@Override
public boolean onTouch(View v, MotionEvent event) {
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
initialX = ((ViewGroup.MarginLayoutParams)getLayoutParams()).leftMargin;
initialY = ((ViewGroup.MarginLayoutParams)getLayoutParams()).topMargin;
initialTouchX = event.getRawX();
initialTouchY = event.getRawY();
return true;
case MotionEvent.ACTION_MOVE:
int x = initialX + (int)(event.getRawX() - initialTouchX);
int y = initialY + (int)(event.getRawY() - initialTouchY);
WindowManager.LayoutParams params = (WindowManager.LayoutParams)getLayoutParams();
params.x = x;
params.y = y;
WindowManager wm = (WindowManager)getContext().getSystemService(WINDOW_SERVICE);
wm.updateViewLayout(FloatingControlWindow.this, params);
return true;
}
return false;
}
});
// 控制按钮初始化
Button btnTap = findViewById(R.id.btn_tap);
Button btnSwipe = findViewById(R.id.btn_swipe);
Button btnColor = findViewById(R.id.btn_color);
Button btnImage = findViewById(R.id.btn_image);
btnTap.setOnClickListener(v -> showTapDialog());
btnSwipe.setOnClickListener(v -> showSwipeDialog());
btnColor.setOnClickListener(v -> showColorDialog());
btnImage.setOnClickListener(v -> showImageDialog());
}
private void showTapDialog() {
// 显示点击设置对话框
AlertDialog.Builder builder = new AlertDialog.Builder(getContext());
builder.setTitle("设置点击操作");
View view = LayoutInflater.from(getContext()).inflate(R.layout.dialog_tap, null);
EditText etX = view.findViewById(R.id.et_x);
EditText etY = view.findViewById(R.id.et_y);
EditText etDuration = view.findViewById(R.id.et_duration);
builder.setView(view);
builder.setPositiveButton("确定", (dialog, which) -> {
try {
int x = Integer.parseInt(etX.getText().toString());
int y = Integer.parseInt(etY.getText().toString());
int duration = Integer.parseInt(etDuration.getText().toString());
TouchUtils.tap(x, y);
if (duration > 0) {
TouchUtils.longTap(x, y, duration);
}
} catch (NumberFormatException e) {
Toast.makeText(getContext(), "请输入有效的数字", Toast.LENGTH_SHORT).show();
}
});
builder.setNegativeButton("取消", null);
builder.show();
}
private void showSwipeDialog() {
// 显示滑动设置对话框
AlertDialog.Builder builder = new AlertDialog.Builder(getContext());
builder.setTitle("设置滑动操作");
View view = LayoutInflater.from(getContext()).inflate(R.layout.dialog_swipe, null);
EditText etStartX = view.findViewById(R.id.et_start_x);
EditText etStartY = view.findViewById(R.id.et_start_y);
EditText etEndX = view.findViewById(R.id.et_end_x);
EditText etEndY = view.findViewById(R.id.et_end_y);
EditText etDuration = view.findViewById(R.id.et_duration);
builder.setView(view);
builder.setPositiveButton("确定", (dialog, which) -> {
try {
int startX = Integer.parseInt(etStartX.getText().toString());
int startY = Integer.parseInt(etStartY.getText().toString());
int endX = Integer.parseInt(etEndX.getText().toString());
int endY = Integer.parseInt(etEndY.getText().toString());
int duration = Integer.parseInt(etDuration.getText().toString());
SwipeUtils.swipe(startX, startY, endX, endY, duration);
} catch (NumberFormatException e) {
Toast.makeText(getContext(), "请输入有效的数字", Toast.LENGTH_SHORT).show();
}
});
builder.setNegativeButton("取消", null);
builder.show();
}
private void showColorDialog() {
// 显示颜色检测对话框
AlertDialog.Builder builder = new AlertDialog.Builder(getContext());
builder.setTitle("设置颜色检测");
View view = LayoutInflater.from(getContext()).inflate(R.layout.dialog_color, null);
EditText etX = view.findViewById(R.id.et_x);
EditText etY = view.findViewById(R.id.et_y);
Button btnPickColor = view.findViewById(R.id.btn_pick_color);
View colorPreview = view.findViewById(R.id.color_preview);
EditText etTolerance = view.findViewById(R.id.et_tolerance);
builder.setView(view);
builder.setPositiveButton("检测", (dialog, which) -> {
try {
int x = Integer.parseInt(etX.getText().toString());
int y = Integer.parseInt(etY.getText().toString());
int tolerance = Integer.parseInt(etTolerance.getText().toString());
int color = ColorDetector.getColorAtPoint(x, y);
if (color != 0) {
String hexColor = String.format("#%08X", color);
Toast.makeText(getContext(), "检测到颜色: " + hexColor, Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(getContext(), "无法获取颜色", Toast.LENGTH_SHORT).show();
}
} catch (NumberFormatException e) {
Toast.makeText(getContext(), "请输入有效的数字", Toast.LENGTH_SHORT).show();
}
});
btnPickColor.setOnClickListener(v -> {
try {
int x = Integer.parseInt(etX.getText().toString());
int y = Integer.parseInt(etY.getText().toString());
int color = ColorDetector.getColorAtPoint(x, y);
if (color != 0) {
colorPreview.setBackgroundColor(color);
} else {
Toast.makeText(getContext(), "无法获取颜色", Toast.LENGTH_SHORT).show();
}
} catch (NumberFormatException e) {
Toast.makeText(getContext(), "请输入有效的坐标", Toast.LENGTH_SHORT).show();
}
});
builder.setNegativeButton("取消", null);
builder.show();
}
private void showImageDialog() {
// 显示图像识别对话框
AlertDialog.Builder builder = new AlertDialog.Builder(getContext());
builder.setTitle("设置图像识别");
View view = LayoutInflater.from(getContext()).inflate(R.layout.dialog_image, null);
ImageView ivTemplate = view.findViewById(R.id.iv_template);
Button btnSelectImage = view.findViewById(R.id.btn_select_image);
EditText etThreshold = view.findViewById(R.id.et_threshold);
builder.setView(view);
builder.setPositiveButton("识别", (dialog, which) -> {
try {
float threshold = Float.parseFloat(etThreshold.getText().toString());
if (threshold < 0 || threshold > 1) {
Toast.makeText(getContext(), "阈值必须在0-1之间", Toast.LENGTH_SHORT).show();
return;
}
if (ivTemplate.getDrawable() == null) {
Toast.makeText(getContext(), "请先选择模板图像", Toast.LENGTH_SHORT).show();
return;
}
Bitmap template = ((BitmapDrawable)ivTemplate.getDrawable()).getBitmap();
Point position = ImageRecognizer.findImage(template, threshold);
if (position != null) {
Toast.makeText(getContext(),
String.format("找到图像在(%d, %d)", position.x, position.y),
Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(getContext(), "未找到匹配图像", Toast.LENGTH_SHORT).show();
}
} catch (NumberFormatException e) {
Toast.makeText(getContext(), "请输入有效的阈值", Toast.LENGTH_SHORT).show();
}
});
btnSelectImage.setOnClickListener(v -> {
// 打开文件选择器选择模板图像
Intent intent = new Intent(Intent.ACTION_GET_CONTENT);
intent.setType("image/*");
((Activity)getContext()).startActivityForResult(intent, REQUEST_PICK_IMAGE);
});
builder.setNegativeButton("取消", null);
builder.show();
}
}
3.3 主活动实现
java
public class MainActivity extends AppCompatActivity {
private static final int REQUEST_OVERLAY_PERMISSION = 1001;
private static final int REQUEST_ACCESSIBILITY_PERMISSION = 1002;
private static final int REQUEST_PICK_IMAGE = 1003;
private Button btnStartService;
private Button btnStopService;
private Switch switchAutoStart;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btnStartService = findViewById(R.id.btn_start_service);
btnStopService = findViewById(R.id.btn_stop_service);
switchAutoStart = findViewById(R.id.switch_auto_start);
btnStartService.setOnClickListener(v -> startAssistantService());
btnStopService.setOnClickListener(v -> stopAssistantService());
// 检查权限
checkPermissions();
}
private void checkPermissions() {
// 检查悬浮窗权限
if (!Settings.canDrawOverlays(this)) {
Intent intent = new Intent(Settings.ACTION_MANAGE_OVERLAY_PERMISSION,
Uri.parse("package:" + getPackageName()));
startActivityForResult(intent, REQUEST_OVERLAY_PERMISSION);
}
// 检查无障碍服务权限
if (!isAccessibilityServiceEnabled()) {
Intent intent = new Intent(Settings.ACTION_ACCESSIBILITY_SETTINGS);
startActivityForResult(intent, REQUEST_ACCESSIBILITY_PERMISSION);
}
}
private boolean isAccessibilityServiceEnabled() {
String serviceName = getPackageName() + "/" + GameAssistantService.class.getName();
AccessibilityManager am = (AccessibilityManager)getSystemService(ACCESSIBILITY_SERVICE);
List<AccessibilityServiceInfo> enabledServices = am.getEnabledAccessibilityServiceList(
AccessibilityServiceInfo.FEEDBACK_ALL_MASK);
for (AccessibilityServiceInfo service : enabledServices) {
if (serviceName.equals(service.getId())) {
return true;
}
}
return false;
}
private void startAssistantService() {
// 启动无障碍服务
Intent serviceIntent = new Intent(this, GameAssistantService.class);
startService(serviceIntent);
// 显示悬浮窗
showFloatingWindow();
Toast.makeText(this, "辅助服务已启动", Toast.LENGTH_SHORT).show();
}
private void stopAssistantService() {
// 停止无障碍服务
Intent serviceIntent = new Intent(this, GameAssistantService.class);
stopService(serviceIntent);
// 隐藏悬浮窗
hideFloatingWindow();
Toast.makeText(this, "辅助服务已停止", Toast.LENGTH_SHORT).show();
}
private void showFloatingWindow() {
WindowManager wm = (WindowManager)getSystemService(WINDOW_SERVICE);
FloatingControlWindow floatingWindow = new FloatingControlWindow(this);
WindowManager.LayoutParams params = new WindowManager.LayoutParams(
WindowManager.LayoutParams.WRAP_CONTENT,
WindowManager.LayoutParams.WRAP_CONTENT,
Build.VERSION.SDK_INT >= Build.VERSION_CODES.O ?
WindowManager.LayoutParams.TYPE_APPLICATION_OVERLAY :
WindowManager.LayoutParams.TYPE_PHONE,
WindowManager.LayoutParams.FLAG_NOT_FOCUSABLE,
PixelFormat.TRANSLUCENT);
params.gravity = Gravity.START | Gravity.TOP;
params.x = 100;
params.y = 100;
wm.addView(floatingWindow, params);
}
private void hideFloatingWindow() {
// 实现隐藏悬浮窗的逻辑
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == REQUEST_OVERLAY_PERMISSION) {
if (!Settings.canDrawOverlays(this)) {
Toast.makeText(this, "需要悬浮窗权限才能显示控制面板", Toast.LENGTH_SHORT).show();
}
} else if (requestCode == REQUEST_ACCESSIBILITY_PERMISSION) {
if (!isAccessibilityServiceEnabled()) {
Toast.makeText(this, "需要无障碍服务权限才能使用辅助功能", Toast.LENGTH_SHORT).show();
}
} else if (requestCode == REQUEST_PICK_IMAGE && resultCode == RESULT_OK && data != null) {
// 处理选择的模板图像
try {
InputStream inputStream = getContentResolver().openInputStream(data.getData());
Bitmap bitmap = BitmapFactory.decodeStream(inputStream);
// 更新对话框中的图像视图
// 这里需要根据实际实现调整
} catch (Exception e) {
Toast.makeText(this, "无法加载图像", Toast.LENGTH_SHORT).show();
}
}
}
}
第四部分:高级功能与优化
4.1 脚本录制与回放
java
public class ScriptRecorder {
private List<ScriptAction> actions = new ArrayList<>();
private boolean isRecording = false;
private long startTime;
public void startRecording() {
actions.clear();
isRecording = true;
startTime = System.currentTimeMillis();
}
public void stopRecording() {
isRecording = false;
}
public void recordTap(int x, int y, long duration) {
if (!isRecording) return;
long timestamp = System.currentTimeMillis() - startTime;
actions.add(new TapAction(x, y, duration, timestamp));
}
public void recordSwipe(int startX, int startY, int endX, int endY, long duration) {
if (!isRecording) return;
long timestamp = System.currentTimeMillis() - startTime;
actions.add(new SwipeAction(startX, startY, endX, endY, duration, timestamp));
}
public void playScript() {
if (actions.isEmpty()) return;
new Thread(() -> {
long startPlayTime = System.currentTimeMillis();
for (ScriptAction action : actions) {
long elapsedTime = System.currentTimeMillis() - startPlayTime;
long delay = action.getTimestamp() - elapsedTime;
if (delay > 0) {
try {
Thread.sleep(delay);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
if (action instanceof TapAction) {
TapAction tap = (TapAction)action;
if (tap.getDuration() > 0) {
TouchUtils.longTap(tap.getX(), tap.getY(), (int)tap.getDuration());
} else {
TouchUtils.tap(tap.getX(), tap.getY());
}
} else if (action instanceof SwipeAction) {
SwipeAction swipe = (SwipeAction)action;
SwipeUtils.swipe(swipe.getStartX(), swipe.getStartY(),
swipe.getEndX(), swipe.getEndY(),
(int)swipe.getDuration());
}
}
}).start();
}
public void saveScript(String fileName) {
try (ObjectOutputStream oos = new ObjectOutputStream(
new FileOutputStream(new File(getContext().getFilesDir(), fileName)))) {
oos.writeObject(actions);
} catch (IOException e) {
e.printStackTrace();
}
}
@SuppressWarnings("unchecked")
public void loadScript(String fileName) {
try (ObjectInputStream ois = new ObjectInputStream(
new FileInputStream(new File(getContext().getFilesDir(), fileName)))) {
actions = (List<ScriptAction>)ois.readObject();
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
public abstract class ScriptAction implements Serializable {
private long timestamp;
public ScriptAction(long timestamp) {
this.timestamp = timestamp;
}
public long getTimestamp() {
return timestamp;
}
public abstract void execute();
}
public class TapAction extends ScriptAction {
private int x, y;
private long duration;
public TapAction(int x, int y, long duration, long timestamp) {
super(timestamp);
this.x = x;
this.y = y;
this.duration = duration;
}
// getters and execute method
}
public class SwipeAction extends ScriptAction {
private int startX, startY, endX, endY;
private long duration;
public SwipeAction(int startX, int startY, int endX, int endY, long duration, long timestamp) {
super(timestamp);
this.startX = startX;
this.startY = startY;
this.endX = endX;
this.endY = endY;
this.duration = duration;
}
// getters and execute method
}
}
4.2 条件触发与自动化
java
public class ConditionTrigger {
private Handler handler = new Handler();
private Runnable checkRunnable;
private boolean isRunning = false;
/**
* 设置颜色条件触发
* @param x X坐标
* @param y Y坐标
* @param targetColor 目标颜色
* @param tolerance 容差
* @param action 满足条件时执行的动作
* @param interval 检查间隔(毫秒)
*/
public void setColorCondition(int x, int y, int targetColor, int tolerance,
Runnable action, long interval) {
stop();
checkRunnable = new Runnable() {
@Override
public void run() {
if (ColorDetector.compareColor(x, y, targetColor, tolerance)) {
action.run();
}
if (isRunning) {
handler.postDelayed(this, interval);
}
}
};
start();
}
/**
* 设置图像条件触发
* @param template 模板图像
* @param threshold 匹配阈值
* @param action 满足条件时执行的动作
* @param interval 检查间隔(毫秒)
*/
public void setImageCondition(Bitmap template, float threshold,
Consumer<Point> action, long interval) {
stop();
checkRunnable = new Runnable() {
@Override
public void run() {
Point position = ImageRecognizer.findImage(template, threshold);
if (position != null) {
action.accept(position);
}
if (isRunning) {
handler.postDelayed(this, interval);
}
}
};
start();
}
public void start() {
if (checkRunnable != null && !isRunning) {
isRunning = true;
handler.post(checkRunnable);
}
}
public void stop() {
isRunning = false;
handler.removeCallbacks(checkRunnable);
}
}
4.3 性能优化与多线程处理
java
public class TaskScheduler {
private static final int CORE_POOL_SIZE = 4;
private static final int MAX_POOL_SIZE = 8;
private static final long KEEP_ALIVE_TIME = 60L;
private ThreadPoolExecutor executor;
private Handler mainHandler;
public TaskScheduler() {
executor = new ThreadPoolExecutor(
CORE_POOL_SIZE,
MAX_POOL_SIZE,
KEEP_ALIVE_TIME,
TimeUnit.SECONDS,
new LinkedBlockingQueue<>());
mainHandler = new Handler(Looper.getMainLooper());
}
/**
* 在后台线程执行任务
* @param task 要执行的任务
*/
public void executeBackground(Runnable task) {
executor.execute(task);
}
/**
* 在后台线程执行任务,完成后在主线程回调
* @param backgroundTask 后台任务
* @param uiCallback 主线程回调
*/
public <T> void executeWithCallback(Callable<T> backgroundTask,
Consumer<T> uiCallback) {
executor.execute(() -> {
try {
final T result = backgroundTask.call();
mainHandler.post(() -> uiCallback.accept(result));
} catch (Exception e) {
e.printStackTrace();
}
});
}
/**
* 执行定时重复任务
* @param task 要执行的任务
* @param initialDelay 初始延迟(毫秒)
* @param period 执行间隔(毫秒)
* @return 可用于取消任务的Future
*/
public Future<?> scheduleAtFixedRate(Runnable task, long initialDelay, long period) {
ScheduledExecutorService scheduler = Executors.newSingleThreadScheduledExecutor();
return scheduler.scheduleAtFixedRate(task, initialDelay, period, TimeUnit.MILLISECONDS);
}
/**
* 停止所有任务
*/
public void shutdown() {
executor.shutdownNow();
}
}
第五部分:测试与部署
5.1 功能测试方案
java
public class FunctionTest {
private Context context;
private TaskScheduler scheduler;
public FunctionTest(Context context) {
this.context = context;
this.scheduler = new TaskScheduler();
}
public void testAllFunctions() {
testTap();
testSwipe();
testColorDetection();
testImageRecognition();
}
private void testTap() {
scheduler.executeWithCallback(() -> {
// 测试点击功能
boolean success = TouchUtils.tap(500, 500);
return "点击测试: " + (success ? "成功" : "失败");
}, result -> {
Toast.makeText(context, result, Toast.LENGTH_SHORT).show();
});
}
private void testSwipe() {
scheduler.executeWithCallback(() -> {
// 测试滑动功能
boolean success = SwipeUtils.swipe(300, 1000, 300, 500, 500);
return "滑动测试: " + (success ? "成功" : "失败");
}, result -> {
Toast.makeText(context, result, Toast.LENGTH_SHORT).show();
});
}
private void testColorDetection() {
scheduler.executeWithCallback(() -> {
// 测试颜色检测
int color = ColorDetector.getColorAtPoint(500, 500);
if (color == 0) {
return "颜色检测测试: 失败";
}
boolean match = ColorDetector.compareColor(500, 500, color, 10);
return "颜色检测测试: " + (match ? "成功" : "失败");
}, result -> {
Toast.makeText(context, result, Toast.LENGTH_SHORT).show();
});
}
private void testImageRecognition() {
// 从assets加载测试图像
scheduler.executeWithCallback(() -> {
try {
InputStream is = context.getAssets().open("test_template.png");
Bitmap template = BitmapFactory.decodeStream(is);
Point position = ImageRecognizer.findImage(template, 0.8f);
return "图像识别测试: " + (position != null ? "成功" : "失败");
} catch (IOException e) {
return "图像识别测试: 失败 - " + e.getMessage();
}
}, result -> {
Toast.makeText(context, result, Toast.LENGTH_SHORT).show();
});
}
}
5.2 性能测试与优化
java
public class PerformanceTest {
private static final int TEST_COUNT = 100;
private Context context;
public PerformanceTest(Context context) {
this.context = context;
}
public void runAllTests() {
testTapPerformance();
testSwipePerformance();
testColorDetectionPerformance();
testImageRecognitionPerformance();
}
private void testTapPerformance() {
long startTime = System.currentTimeMillis();
for (int i = 0; i < TEST_COUNT; i++) {
TouchUtils.tap(100, 100);
}
long duration = System.currentTimeMillis() - startTime;
double avgTime = (double)duration / TEST_COUNT;
String result = String.format("点击性能测试: %d次, 平均%.2fms/次",
TEST_COUNT, avgTime);
Toast.makeText(context, result, Toast.LENGTH_LONG).show();
}
// 其他性能测试方法类似...
}
5.3 应用打包与发布注意事项
-
代码混淆配置 :
在
proguard-rules.pro
中添加以下规则:-keep class com.example.gameassistant.** { *; } -keep class org.opencv.** { *; }
-
权限声明 :
确保
AndroidManifest.xml
中已声明所有必要权限 -
无障碍服务配置 :
在
res/xml/service_config.xml
中配置无障碍服务:xml<accessibility-service xmlns:android="http://schemas.android.com/apk/res/android" android:description="@string/accessibility_service_description" android:accessibilityEventTypes="typeAllMask" android:accessibilityFlags="flagDefault|flagRetrieveInteractiveWindows" android:accessibilityFeedbackType="feedbackGeneric" android:notificationTimeout="100" android:canRetrieveWindowContent="true" android:settingsActivity="com.example.gameassistant.MainActivity"/>
-
应用签名 :
使用正式签名密钥对应用进行签名
-
隐私政策 :
准备隐私政策文档,说明应用收集的数据和使用方式
-
应用商店政策 :
确保应用符合Google Play等应用商店的政策要求
第六部分:安全与伦理考虑
6.1 合法使用建议
- 仅用于单机游戏:建议工具仅用于单机游戏,避免用于在线多人游戏
- 教育目的:明确说明工具主要用于学习和研究目的
- 免责声明:在应用中添加免责声明,说明开发者不对滥用行为负责
6.2 防滥用机制
-
使用限制:
javapublic class UsageLimiter { private static final long MAX_USAGE_TIME = 2 * 60 * 60 * 1000; // 2小时 private static final long DAILY_USAGE_LIMIT = 4 * 60 * 60 * 1000; // 每天4小时 private SharedPreferences prefs; private long startTime; private long todayUsageTime; public UsageLimiter(Context context) { prefs = context.getSharedPreferences("usage_stats", Context.MODE_PRIVATE); todayUsageTime = prefs.getLong("today_usage", 0); } public boolean canStart() { // 检查是否超过每日使用限制 if (todayUsageTime >= DAILY_USAGE_LIMIT) { return false; } startTime = System.currentTimeMillis(); return true; } public void stop() { long usageTime = System.currentTimeMillis() - startTime; todayUsageTime += usageTime; // 保存使用时间 prefs.edit().putLong("today_usage", todayUsageTime).apply(); } public void resetDailyLimit() { // 每天重置使用时间 prefs.edit().putLong("today_usage", 0).apply(); todayUsageTime = 0; } }
-
应用检测:
javapublic class GameChecker { public static boolean isAllowedGame(String packageName) { // 实现允许使用辅助工具的游戏包名检查 Set<String> allowedGames = new HashSet<>(); allowedGames.add("com.example.singleplayergame1"); allowedGames.add("com.example.singleplayergame2"); return allowedGames.contains(packageName); } public static boolean checkCurrentGame() { AccessibilityService service = GameAssistantService.getInstance(); if (service == null) { return false; } AccessibilityWindowInfo window = service.getRootInActiveWindow(); if (window == null) { return false; } String packageName = window.getRoot().getPackageName().toString(); return isAllowedGame(packageName); } }
6.3 用户教育与责任
- 在应用中添加"关于"页面,说明工具的合法用途
- 首次启动时显示使用条款和条件
- 定期提醒用户遵守游戏规则和服务条款
第七部分:扩展功能与未来方向
7.1 机器学习增强
java
public class MLEnhancer {
private Interpreter interpreter;
public MLEnhancer(Context context) {
try {
// 从assets加载TensorFlow Lite模型
AssetManager assetManager = context.getAssets();
InputStream inputStream = assetManager.open("game_model.tflite");
File modelFile = new File(context.getFilesDir(), "temp_model.tflite");
FileOutputStream outputStream = new FileOutputStream(modelFile);
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
inputStream.close();
outputStream.close();
interpreter = new Interpreter(modelFile);
} catch (IOException e) {
e.printStackTrace();
}
}
public float predictAction(Bitmap screen, float[] inputFeatures) {
if (interpreter == null) {
return -1;
}
// 将屏幕截图转换为模型输入格式
Bitmap resizedBitmap = Bitmap.createScaledBitmap(screen, 224, 224, true);
ByteBuffer inputBuffer = ByteBuffer.allocateDirect(224 * 224 * 3 * 4);
inputBuffer.order(ByteOrder.nativeOrder());
// 归一化像素值到[0,1]范围
for (int y = 0; y < 224; y++) {
for (int x = 0; x < 224; x++) {
int pixel = resizedBitmap.getPixel(x, y);
inputBuffer.putFloat(Color.red(pixel) / 255.0f);
inputBuffer.putFloat(Color.green(pixel) / 255.0f);
inputBuffer.putFloat(Color.blue(pixel) / 255.0f);
}
}
// 准备其他输入特征
ByteBuffer featureBuffer = ByteBuffer.allocateDirect(inputFeatures.length * 4);
featureBuffer.order(ByteOrder.nativeOrder());
for (float feature : inputFeatures) {
featureBuffer.putFloat(feature);
}
// 准备输出缓冲区
float[][] output = new float[1][1];
// 运行模型
Object[] inputs = {inputBuffer, featureBuffer};
Map<Integer, Object> outputs = new HashMap<>();
outputs.put(0, output);
interpreter.runForMultipleInputsOutputs(inputs, outputs);
return output[0][0];
}
}
7.2 云同步与配置共享
java
public class CloudSyncManager {
private static final String API_BASE_URL = "https://your-api-server.com/";
private Retrofit retrofit;
private CloudSyncService service;
public CloudSyncManager() {
retrofit = new Retrofit.Builder()
.baseUrl(API_BASE_URL)
.addConverterFactory(GsonConverterFactory.create())
.build();
service = retrofit.create(CloudSyncService.class);
}
public void uploadScript(ScriptRecorder script, String name, String description) {
// 将脚本转换为可上传的格式
ScriptData scriptData = convertScriptToData(script, name, description);
service.uploadScript(scriptData).enqueue(new Callback<ApiResponse>() {
@Override
public void onResponse(Call<ApiResponse> call, Response<ApiResponse> response) {
if (response.isSuccessful()) {
Log.d("CloudSync", "Script uploaded successfully");
} else {
Log.e("CloudSync", "Script upload failed");
}
}
@Override
public void onFailure(Call<ApiResponse> call, Throwable t) {
Log.e("CloudSync", "Script upload error: " + t.getMessage());
}
});
}
public void downloadScript(String scriptId, Consumer<ScriptRecorder> callback) {
service.downloadScript(scriptId).enqueue(new Callback<ScriptData>() {
@Override
public void onResponse(Call<ScriptData> call, Response<ScriptData> response) {
if (response.isSuccessful()) {
ScriptRecorder script = convertDataToScript(response.body());
callback.accept(script);
} else {
Log.e("CloudSync", "Script download failed");
}
}
@Override
public void onFailure(Call<ScriptData> call, Throwable t) {
Log.e("CloudSync", "Script download error: " + t.getMessage());
}
});
}
private interface CloudSyncService {
@POST("scripts/upload")
Call<ApiResponse> uploadScript(@Body ScriptData script);
@GET("scripts/download/{id}")
Call<ScriptData> downloadScript(@Path("id") String scriptId);
}
private static class ScriptData {
String name;
String description;
String gamePackage;
List<ActionData> actions;
}
private static class ActionData {
String type;
int[] coordinates;
long duration;
long timestamp;
}
}
7.3 社区与用户自定义
java
public class PluginManager {
private Context context;
private Map<String, PluginInfo> loadedPlugins = new HashMap<>();
public PluginManager(Context context) {
this.context = context;
}
public void loadPlugin(File pluginFile) {
try {
// 使用DexClassLoader加载插件
DexClassLoader classLoader = new DexClassLoader(
pluginFile.getAbsolutePath(),
context.getCodeCacheDir().getAbsolutePath(),
null,
context.getClassLoader());
// 加载插件入口类
Class<?> pluginClass = classLoader.loadClass("com.example.plugin.PluginEntry");
PluginEntry entry = (PluginEntry)pluginClass.newInstance();
// 注册插件
PluginInfo info = new PluginInfo();
info.name = entry.getName();
info.description = entry.getDescription();
info.icon = entry.getIcon();
info.entryClass = pluginClass.getName();
info.classLoader = classLoader;
loadedPlugins.put(info.name, info);
} catch (Exception e) {
Log.e("PluginManager", "Load plugin error: " + e.getMessage());
}
}
public void executePlugin(String pluginName) {
PluginInfo info = loadedPlugins.get(pluginName);
if (info == null) {
return;
}
try {
Class<?> pluginClass = info.classLoader.loadClass(info.entryClass);
PluginEntry entry = (PluginEntry)pluginClass.newInstance();
entry.execute(context);
} catch (Exception e) {
Log.e("PluginManager", "Execute plugin error: " + e.getMessage());
}
}
private static class PluginInfo {
String name;
String description;
Bitmap icon;
String entryClass;
ClassLoader classLoader;
}
public interface PluginEntry {
String getName();
String getDescription();
Bitmap getIcon();
void execute(Context context);
}
}
第八部分:总结与完整代码整合
8.1 项目结构总结
完整的项目结构如下:
GameAssistant/
├── app/
│ ├── src/
│ │ ├── main/
│ │ │ ├── java/com/example/gameassistant/
│ │ │ │ ├── core/
│ │ │ │ │ ├── TouchUtils.java
│ │ │ │ │ ├── SwipeUtils.java
│ │ │ │ │ ├── ColorDetector.java
│ │ │ │ │ ├── ImageRecognizer.java
│ │ │ │ │ └── MultiImageRecognizer.java
│ │ │ │ ├── service/
│ │ │ │ │ └── GameAssistantService.java
│ │ │ │ ├── ui/
│ │ │ │ │ ├── FloatingControlWindow.java
│ │ │ │ │ └── MainActivity.java
│ │ │ │ ├── utils/
│ │ │ │ │ ├── ScriptRecorder.java
│ │ │ │ │ ├── ConditionTrigger.java
│ │ │ │ │ └── TaskScheduler.java
│ │ │ │ ├── test/
│ │ │ │ │ ├── FunctionTest.java
│ │ │ │ │ └── PerformanceTest.java
│ │ │ │ └── extension/
│ │ │ │ ├── MLEnhancer.java
│ │ │ │ ├── CloudSyncManager.java
│ │ │ │ └── PluginManager.java
│ │ │ ├── res/
│ │ │ │ ├── layout/
│ │ │ │ ├── xml/
│ │ │ │ └── ...
│ │ │ └── AndroidManifest.xml
│ │ └── ...
├── build.gradle
└── ...
8.2 关键类交互关系
- MainActivity:应用入口,负责权限检查和启动服务
- GameAssistantService:核心无障碍服务,提供屏幕访问和操作能力
- FloatingControlWindow:悬浮控制界面,提供用户交互
- 功能工具类 :
- TouchUtils:点击操作
- SwipeUtils:滑动操作
- ColorDetector:颜色检测
- ImageRecognizer:图像识别
- 辅助类 :
- ScriptRecorder:脚本录制与回放
- ConditionTrigger:条件触发
- TaskScheduler:任务调度
8.3 完整代码整合与使用示例
以下是一个完整的使用示例,展示如何组合各个功能模块:
java
public class GameAutomationExample {
private Context context;
private ScriptRecorder scriptRecorder;
private ConditionTrigger conditionTrigger;
public GameAutomationExample(Context context) {
this.context = context;
this.scriptRecorder = new ScriptRecorder();
this.conditionTrigger = new ConditionTrigger();
}
public void startAutoPlay() {
// 1. 录制脚本
scriptRecorder.startRecording();
// 模拟用户操作
TouchUtils.tap(100, 200); // 点击开始按钮
SwipeUtils.swipe(300, 1000, 300, 500, 500); // 滑动屏幕
// 结束录制并保存
scriptRecorder.stopRecording();
scriptRecorder.saveScript("level1_script");
// 2. 设置条件触发
Bitmap targetImage = loadImageFromAssets("target.png");
conditionTrigger.setImageCondition(targetImage, 0.8f, position -> {
// 当检测到目标图像时点击它
TouchUtils.tap(position.x, position.y);
}, 1000);
// 3. 执行脚本
scriptRecorder.loadScript("level1_script");
scriptRecorder.playScript();
}
public void stopAutoPlay() {
conditionTrigger.stop();
}
private Bitmap loadImageFromAssets(String fileName) {
try {
InputStream is = context.getAssets().open(fileName);
return BitmapFactory.decodeStream(is);
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
}
第九部分:常见问题与解决方案
9.1 权限问题处理
java
public class PermissionHelper {
public static boolean checkAndRequestPermissions(Activity activity) {
List<String> missingPermissions = new ArrayList<>();
// 检查悬浮窗权限
if (!Settings.canDrawOverlays(activity)) {
missingPermissions.add("OVERLAY");
}
// 检查无障碍服务
if (!isAccessibilityServiceEnabled(activity)) {
missingPermissions.add("ACCESSIBILITY");
}
// 检查存储权限
if (ContextCompat.checkSelfPermission(activity, Manifest.permission.WRITE_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
missingPermissions.add(Manifest.permission.WRITE_EXTERNAL_STORAGE);
}
if (!missingPermissions.isEmpty()) {
if (missingPermissions.contains("OVERLAY")) {
Intent intent = new Intent(Settings.ACTION_MANAGE_OVERLAY_PERMISSION,
Uri.parse("package:" + activity.getPackageName()));
activity.startActivityForResult(intent, REQUEST_OVERLAY_PERMISSION);
}
if (missingPermissions.contains("ACCESSIBILITY")) {
Intent intent = new Intent(Settings.ACTION_ACCESSIBILITY_SETTINGS);
activity.startActivityForResult(intent, REQUEST_ACCESSIBILITY_PERMISSION);
}
List<String> runtimePermissions = missingPermissions.stream()
.filter(p -> !p.equals("OVERLAY") && !p.equals("ACCESSIBILITY"))
.collect(Collectors.toList());
if (!runtimePermissions.isEmpty()) {
ActivityCompat.requestPermissions(activity,
runtimePermissions.toArray(new String[0]),
REQUEST_RUNTIME_PERMISSIONS);
}
return false;
}
return true;
}
}
9.2 兼容性问题
java
public class CompatibilityUtils {
public static boolean isFeatureSupported(Context context, String feature) {
PackageManager pm = context.getPackageManager();
switch (feature) {
case "ACCESSIBILITY":
return Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN;
case "SCREENSHOT":
return Build.VERSION.SDK_INT >= Build.VERSION_CODES.R;
case "OVERLAY":
return Build.VERSION.SDK_INT >= Build.VERSION_CODES.M;
default:
return pm.hasSystemFeature(feature);
}
}
public static void showUnsupportedMessage(Activity activity, String feature) {
String message;
switch (feature) {
case "ACCESSIBILITY":
message = "您的设备不支持无障碍服务或版本过低";
break;
case "SCREENSHOT":
message = "截图功能需要Android 11或更高版本";
break;
case "OVERLAY":
message = "悬浮窗功能需要Android 6.0或更高版本";
break;
default:
message = "您的设备不支持此功能";
}
new AlertDialog.Builder(activity)
.setTitle("不支持的设备")
.setMessage(message)
.setPositiveButton("确定", null)
.show();
}
}
9.3 调试技巧
-
ADB调试命令:
# 查看无障碍服务状态 adb shell settings get secure enabled_accessibility_services # 模拟点击 adb shell input tap x y # 模拟滑动 adb shell input swipe x1 y1 x2 y2 duration
-
日志过滤:
javapublic class DebugLogger { private static final String TAG = "GameAssistant"; private static boolean isDebug = BuildConfig.DEBUG; public static void d(String message) { if (isDebug) { Log.d(TAG, message); } } public static void e(String message, Throwable e) { if (isDebug) { Log.e(TAG, message, e); } } }
-
性能监控:
javapublic class PerformanceMonitor { private static Map<String, Long> startTimes = new HashMap<>(); public static void startTrace(String tag) { startTimes.put(tag, System.currentTimeMillis()); } public static void endTrace(String tag) { Long startTime = startTimes.get(tag); if (startTime != null) { long duration = System.currentTimeMillis() - startTime; DebugLogger.d(tag + " took " + duration + "ms"); startTimes.remove(tag); } } }
第十部分:未来发展与进阶学习
10.1 进阶功能方向
- AI游戏策略:集成强化学习算法,让工具能够自主制定游戏策略
- 3D游戏支持:开发针对3D游戏的物体识别和操作功能
- 云游戏适配:支持云游戏平台的自动化操作
- 跨平台支持:将核心功能移植到其他平台如iOS、Windows
10.2 学习资源推荐
-
Android官方文档:
- Accessibility Service: https://developer.android.com/guide/topics/ui/accessibility/service
- Input Events: https://developer.android.com/training/gestures
-
OpenCV文档:
- Android OpenCV: https://opencv.org/android/
-
机器学习:
- TensorFlow Lite: https://www.tensorflow.org/lite
-
游戏逆向工程:
- Game Hacking: https://gamehacking.academy/
10.3 社区与贡献
- 开源项目:考虑将项目开源,接受社区贡献
- 插件市场:建立用户插件市场,分享自动化脚本
- 用户论坛:创建用户交流论坛,分享使用经验
- 教程与文档:编写详细的使用文档和教程视频
结语
本文详细介绍了如何使用Java开发一个功能完善的Android游戏辅助工具,涵盖了从基础点击、滑动操作到高级图像识别和自动化脚本的所有关键实现细节。通过无障碍服务、图像处理和智能算法等技术,我们构建了一个强大而灵活的工具框架。