javafx中实现发送qq邮箱验证码:
手动导入jar包方法:
第一步:开启QQ邮箱的 POP3/IMAP 或者 SMTP/IMAP 服务
打开qq邮箱(电脑端),找到设置里的账号与安全的安全设置,往下滑就可以找到 POP3/IMAP 或者 SMTP/IMAP 服务,并开启它,得到授权码。
第二步:网上下载java.mail.jar包,将它导到你项目里自己建的lib目录下,右击,添加为库。
第三步:在 pom.xml
中添加以下依赖配置,直接引用本地 JAR 文件
java
<dependency>
<groupId>javax.mail</groupId>
<artifactId>mail</artifactId>
<version>1.4.7</version> <!-- 根据实际版本修改 -->
<scope>system</scope>
<systemPath>${project.basedir}/lib/javax.mail.jar</systemPath>
</dependency>
并确保依赖已添加到模块的类路径中
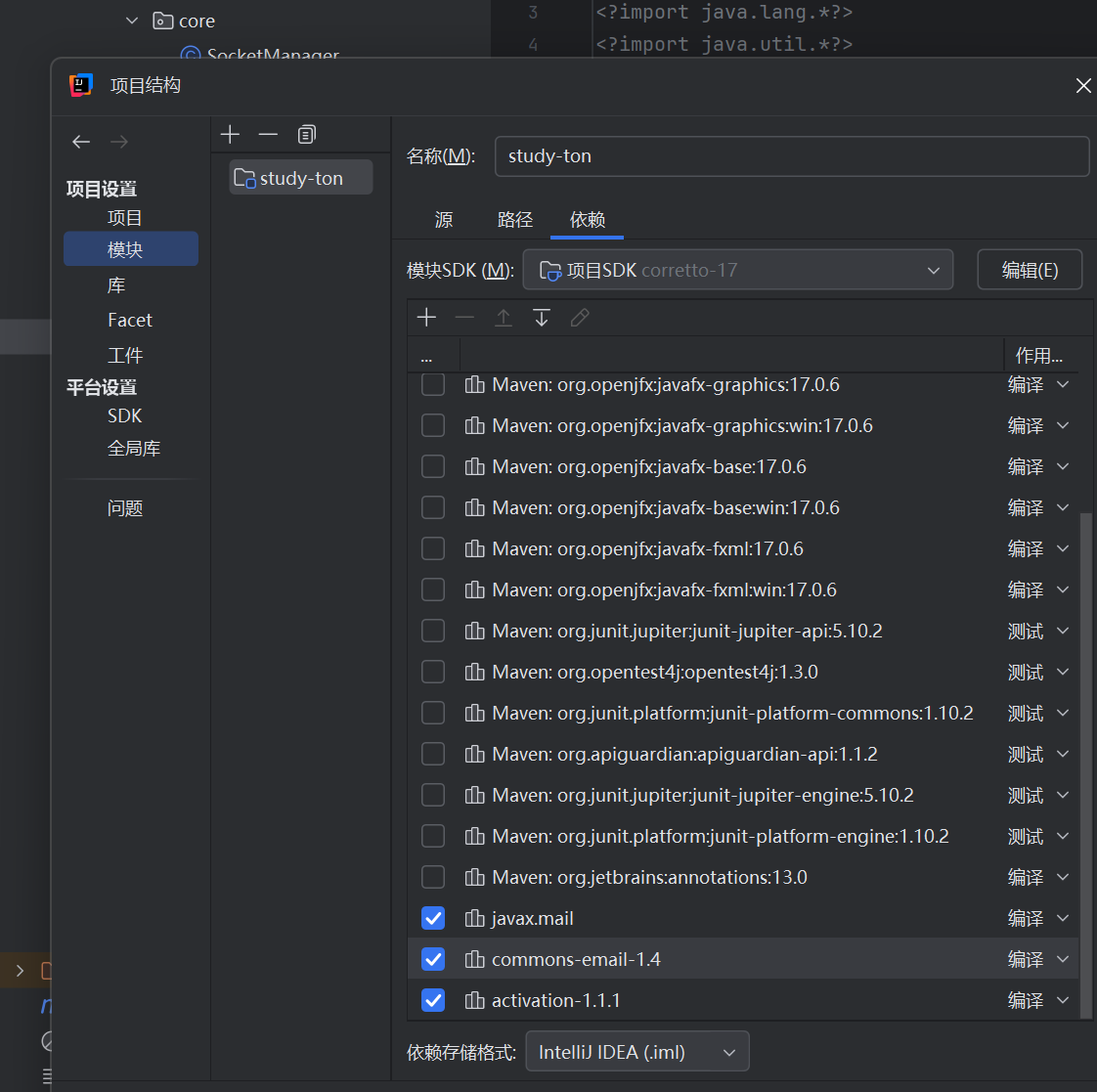
如果项目使用 module-info.java
,需添加对 java.mail
模块的依赖:
java
module your.module.name {
requires java.mail; // 添加此行
// 其他模块声明...
}
最后:
代码如下:
java
// QQ邮箱SMTP配置
private static final String SMTP_HOST = "smtp.qq.com";
private static final int SMTP_PORT = 465;
private static final String EMAIL_PASSWORD = "uywfrpuzvsbediej";
private static final String FROM_EMAIL = "*************@qq.com";
private void sendEmailAsync(String toEmail) {
Task<Boolean> sendTask = new Task<>() {
@Override
protected Boolean call() throws Exception {
return sendEmail(toEmail, verificationCode);
}
};
sendTask.setOnSucceeded(e -> {
if (sendTask.getValue()) {
startCountdown();
} else {
Platform.runLater(() -> {
new Alert(Alert.AlertType.ERROR, "验证码发送失败,请检查邮箱地址!").show();
sendCodeBtn.setDisable(false);
});
}
});
sendTask.setOnFailed(e -> {
Platform.runLater(() -> {
new Alert(Alert.AlertType.ERROR, "邮件发送失败:" + sendTask.getException().getMessage()).show();
sendCodeBtn.setDisable(false);
});
});
new Thread(sendTask).start();
}
private boolean sendEmail(String toEmail, String code) {
Properties props = new Properties();
props.put("mail.smtp.host", SMTP_HOST);
props.put("mail.smtp.port", SMTP_PORT);
props.put("mail.smtp.ssl.enable", "true");
props.put("mail.smtp.auth", "true");
Session session = Session.getInstance(props, new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(FROM_EMAIL, EMAIL_PASSWORD);
}
});
try {
MimeMessage message = new MimeMessage(session);
message.setFrom(new InternetAddress(FROM_EMAIL));
message.setRecipient(Message.RecipientType.TO, new InternetAddress(toEmail));
message.setSubject("【StudyXing】注册验证码");
message.setText("您的验证码是:" + code + ",有效期1分钟。");
Transport.send(message);
return true;
} catch (MessagingException e) {
e.printStackTrace();
return false;
}
}