List findIntersection & getUnion 求两个列表的交集和并集
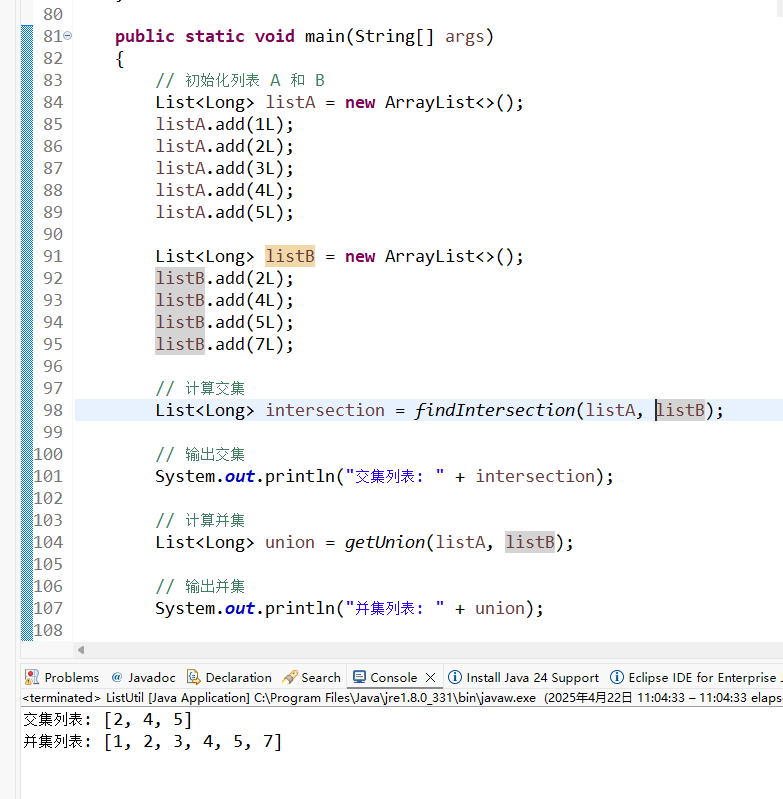
package zwf;
import java.util.ArrayList;
import java.util.LinkedHashSet;
import java.util.List;
/**
* 列表工具类
*
* @author ZengWenFeng
* @date 2025.04.22
* @mobile 13805029595
* @email [email protected]
*/
public class ListUtil
{
/**
* 计算两个列表的交集
*
* @param listA [1,2,3,4,5]
* @param listB [ 2, 4,5,7,8]
* @return listC [ 2, 4,5]
*/
public static List<Long> findIntersection(List<Long> listA, List<Long> listB)
{
if (listA == null || listA.size() <= 0 || listB == null || listB.size() <= 0)
{
return null;
}
List<Long> result = new ArrayList<Long>();
for (Long element : listA)
{
if (listB.contains(element))
{
result.add(element);
}
}
return result;
}
/**
* 计算两个列表的并集
*
* @param listA [1,2,3,4,5]
* @param listB [ 2, 4,5,7,8]
* @return listC [1,2,3,4,5,7,8]
*/
public static List<Long> getUnion(List<Long> listA, List<Long> listB)
{
if (listA == null && listB == null)
{
return new ArrayList<Long>();
}
if (listA == null)
{
return new ArrayList<Long>(new LinkedHashSet<Long>(listB));
}
if (listB == null)
{
return new ArrayList<Long>(new LinkedHashSet<Long>(listA));
}
LinkedHashSet<Long> unionSet = new LinkedHashSet<>(listA.size() + listB.size());
if (listA.size() > 0)
{
unionSet.addAll(listA);
}
if (listB.size() > 0)
{
unionSet.addAll(listB);
}
return new ArrayList<Long>(unionSet);
}
public static void main(String[] args)
{
// 初始化列表 A 和 B
List<Long> listA = new ArrayList<>();
listA.add(1L);
listA.add(2L);
listA.add(3L);
listA.add(4L);
listA.add(5L);
List<Long> listB = new ArrayList<>();
listB.add(2L);
listB.add(4L);
listB.add(5L);
listB.add(7L);
// 计算交集
List<Long> intersection = findIntersection(listA, listB);
// 输出交集
System.out.println("交集列表: " + intersection);
// 计算并集
List<Long> union = getUnion(listA, listB);
// 输出并集
System.out.println("并集列表: " + union);
}
}