Java 性能优化:如何在资源受限的环境下实现高效运行?
在计算机系统中,性能优化是一项至关重要的任务,尤其是在资源受限的环境下,如何让 Java 程序高效运行是许多开发者面临的挑战。本文将深入探讨 Java 性能优化的策略和技巧,并通过详细代码实例进行说明。
一、内存管理优化
(一)对象创建优化
- 减少不必要的对象创建
- 在 Java 中,频繁的对象创建会消耗大量的内存和 CPU 资源。尽量重用对象,而不是频繁创建新的对象。
- 示例代码:
- 演示不优化和优化后的代码对比,未优化代码频繁创建新的字符串对象,优化代码则利用字符串缓冲区进行拼接。
java
// 不优化的代码
String result = "";
for (int i = 0; i < 1000; i++) {
result += "数字:" + i;
}
// 优化后的代码
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append("数字:").append(i);
}
String result = sb.toString();
- 对象池技术
- 对于一些创建成本较高的对象,可以使用对象池来管理对象的创建和回收。当需要使用对象时,从对象池中获取,使用完毕后归还对象池,而不是直接销毁对象。
- 示例代码:
- 创建一个简单的对象池,管理数据库连接对象。通过定义一个连接池类,使用数组或列表存储可用的连接对象,提供获取和归还连接的方法。
java
public class ConnectionPool {
private final Connection[] connections;
private int currentIndex = 0;
public ConnectionPool(int size) {
connections = new Connection[size];
for (int i = 0; i < size; i++) {
connections[i] = new Connection();
}
}
public synchronized Connection getConnection() {
if (currentIndex >= connections.length) {
return null; // 池已满
}
return connections[currentIndex++];
}
public synchronized void releaseConnection(Connection connection) {
for (int i = 0; i < connections.length; i++) {
if (connections[i] == connection) {
currentIndex = i;
break;
}
}
}
}
(二)内存泄漏防范
- 及时释放资源
- 在使用完资源后,要及时释放资源,如文件句柄、数据库连接、网络连接等。避免这些资源一直被占用,导致内存泄漏。
- 示例代码:
- 在使用 FileInputStream 读取文件后,及时关闭流。
java
FileInputStream fis = null;
try {
fis = new FileInputStream("example.txt");
// 读取文件内容
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fis != null) {
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
- 避免静态字段持有大对象
- 静态字段的生命周期与类的生命周期相同,如果静态字段持有大对象,会导致这些对象无法被垃圾回收。尽量避免使用静态字段存储大对象,或者在不再需要时手动设置静态字段为 null。
- 示例代码:
- 将一个大对象数组设置为静态字段,演示可能导致内存泄漏的情况,以及如何在适当的时候释放它。
java
public class MemoryLeakExample {
private static Object[] bigObjects;
public static void loadBigObjects() {
bigObjects = new Object[10000];
// 初始化大对象数组
}
public static void releaseBigObjects() {
bigObjects = null;
}
}
二、算法优化
(一)时间复杂度优化
- 选择高效的算法
- 不同的算法在处理相同问题时,时间复杂度可能相差很大。尽量选择时间复杂度较低的算法,以提高程序的运行效率。
- 示例代码:
- 比较冒泡排序(时间复杂度 O(n²))和快速排序(时间复杂度 O(n log n))的性能差异。
java
// 冒泡排序
public static void bubbleSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - 1 - i; j++) {
if (arr[j] > arr[j + 1]) {
// 交换元素
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
// 快速排序
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivotIndex = partition(arr, low, high);
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
private static int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
// 交换元素
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
// 交换基准元素到正确位置
int temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return i + 1;
}
- 避免不必要的计算
- 在循环中,尽量避免重复计算或可以提前计算的表达式。将这些计算移到循环外部,以减少计算次数。
- 示例代码:
- 演示在循环中重复计算一个复杂的表达式,以及将表达式计算移到循环外部的情况。
java
// 不优化的代码
double result = 0.0;
for (int i = 0; i < 1000; i++) {
result += Math.sqrt(i) * Math.PI;
}
// 优化后的代码
double sqrtPi = Math.sqrt(Math.PI);
for (int i = 0; i < 1000; i++) {
result += Math.sqrt(i) * sqrtPi;
}
(二)空间复杂度优化
- 使用合适的数据结构
- 根据实际需求选择合适的数据结构,以减少内存的使用。例如,如果只需要存储一组不重复的元素,可以使用 HashSet 而不是 ArrayList。
- 示例代码:
- 比较使用 ArrayList 和 HashSet 存储数据时的内存占用情况。
java
// 使用 ArrayList
List<String> arrayList = new ArrayList<>();
for (int i = 0; i < 1000; i++) {
arrayList.add("Element " + i);
}
// 使用 HashSet
Set<String> hashSet = new HashSet<>();
for (int i = 0; i < 1000; i++) {
hashSet.add("Element " + i);
}
- 原地算法
- 对于一些可以原地处理的数据,尽量使用原地算法,避免额外的内存分配。原地算法是指在不使用额外内存或只使用少量额外内存的情况下,对数据进行处理的算法。
- 示例代码:
- 演示原地反转数组的算法,不需要额外的数组来存储反转后的结果。
java
public static void reverseArray(int[] arr) {
int left = 0;
int right = arr.length - 1;
while (left < right) {
// 交换元素
int temp = arr[left];
arr[left] = arr[right];
arr[right] = temp;
left++;
right--;
}
}
三、并发优化
(一)线程管理优化
- 线程池的应用
- 使用线程池可以有效地控制线程的数量,避免线程过多导致系统资源耗尽。合理配置线程池的参数,如核心线程数、最大线程数、队列大小等,以适应不同的应用场景。
- 示例代码:
- 创建一个固定大小的线程池,提交多个任务到线程池中执行。
java
ExecutorService executor = Executors.newFixedThreadPool(4);
for (int i = 0; i < 10; i++) {
executor.submit(() -> {
System.out.println("任务 " + Thread.currentThread().getId() + " 正在运行");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
});
}
executor.shutdown();
- 避免线程死锁
- 在多线程环境下,要小心线程死锁的问题。死锁是指两个或多个线程相互等待对方持有的锁,导致线程无法继续执行。可以通过合理的锁获取顺序、使用 tryLock 方法等来避免死锁。
- 示例代码:
- 演示一个可能导致死锁的场景,以及如何使用 tryLock 方法来避免死锁。
java
// 可能导致死锁的代码
Object lock1 = new Object();
Object lock2 = new Object();
Thread thread1 = new Thread(() -> {
synchronized (lock1) {
System.out.println("线程 1 获取了锁 1");
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
synchronized (lock2) {
System.out.println("线程 1 获取了锁 2");
}
}
});
Thread thread2 = new Thread(() -> {
synchronized (lock2) {
System.out.println("线程 2 获取了锁 2");
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
synchronized (lock1) {
System.out.println("线程 2 获取了锁 1");
}
}
});
thread1.start();
thread2.start();
// 使用 tryLock 方法避免死锁
ReentrantLock lockA = new ReentrantLock();
ReentrantLock lockB = new ReentrantLock();
Thread thread3 = new Thread(() -> {
while (true) {
boolean gotLockA = false;
boolean gotLockB = false;
try {
gotLockA = lockA.tryLock();
Thread.sleep(100);
if (gotLockA) {
System.out.println("线程 3 获取了锁 A");
gotLockB = lockB.tryLock();
if (gotLockB) {
System.out.println("线程 3 获取了锁 B");
// 执行任务
}
}
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
if (gotLockA) {
lockA.unlock();
}
if (gotLockB) {
lockB.unlock();
}
}
}
});
Thread thread4 = new Thread(() -> {
while (true) {
boolean gotLockB = false;
boolean gotLockA = false;
try {
gotLockB = lockB.tryLock();
Thread.sleep(100);
if (gotLockB) {
System.out.println("线程 4 获取了锁 B");
gotLockA = lockA.tryLock();
if (gotLockA) {
System.out.println("线程 4 获取了锁 A");
// 执行任务
}
}
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
if (gotLockB) {
lockB.unlock();
}
if (gotLockA) {
lockA.unlock();
}
}
}
});
thread3.start();
thread4.start();
(二)并发数据结构
- 使用线程安全的集合
- Java 提供了许多线程安全的集合类,如 ConcurrentHashMap、CopyOnWriteArrayList 等。在多线程环境下,使用这些线程安全的集合可以避免数据不一致的问题。
- 示例代码:
- 演示使用 ConcurrentHashMap 存储和访问数据,与使用普通 HashMap 的区别。
java
// 使用 ConcurrentHashMap
ConcurrentHashMap<String, String> concurrentMap = new ConcurrentHashMap<>();
for (int i = 0; i < 10; i++) {
concurrentMap.put("Key " + i, "Value " + i);
}
String value = concurrentMap.get("Key 5");
// 使用 HashMap(不安全的示例,仅用于演示)
Map<String, String> hashMap = new HashMap<>();
for (int i = 0; i < 10; i++) {
hashMap.put("Key " + i, "Value " + i);
}
// 在多线程环境下,这可能会导致问题
String valueHashMap = hashMap.get("Key 5");
- 原子变量类的使用
- Java 的原子变量类(如 AtomicInteger、 AtomicLong 等)提供了原子性的读写操作,可以在不使用锁的情况下实现高效的并发操作。
- 示例代码:
- 演示使用 AtomicInteger 进行原子性的计数操作,与使用普通 int 变量配合 synchronized 关键字的对比。
java
// 使用 AtomicInteger
AtomicInteger atomicCount = new AtomicInteger(0);
for (int i = 0; i < 1000; i++) {
new Thread(() -> {
atomicCount.incrementAndGet();
}).start();
}
System.out.println("AtomicInteger 最终值:" + atomicCount.get());
// 使用普通 int 变量配合 synchronized
int normalCount = 0;
Object lock = new Object();
for (int i = 0; i < 1000; i++) {
new Thread(() -> {
synchronized (lock) {
normalCount++;
}
}).start();
}
System.out.println("普通 int 变量最终值:" + normalCount);
四、I/O 操作优化
(一)缓冲 I/O
- 使用缓冲流
- 缓冲流(如 BufferedInputStream、BufferedOutputStream、BufferedReader、BufferedWriter 等)可以减少底层 I/O 操作的次数,提高 I/O 操作的效率。通过内部维护一个缓冲区,将多次小的读写操作合并为一次大的读写操作。
- 示例代码:
- 演示使用 FileReader 和 BufferedReader 读取文件的区别,比较读取速度。
java
// 使用 FileReader(无缓冲)
FileReader fileReader = new FileReader("example.txt");
int c;
long startTime = System.currentTimeMillis();
while ((c = fileReader.read()) != -1) {
// 读取字符
}
long endTime = System.currentTimeMillis();
System.out.println("FileReader 读取时间:" + (endTime - startTime) + "ms");
fileReader.close();
// 使用 BufferedReader(带缓冲)
BufferedReader bufferedReader = new BufferedReader(new FileReader("example.txt"));
String line;
long startTimeBuffered = System.currentTimeMillis();
while ((line = bufferedReader.readLine()) != null) {
// 读取行
}
long endTimeBuffered = System.currentTimeMillis();
System.out.println("BufferedReader 读取时间:" + (endTimeBuffered - startTimeBuffered) + "ms");
bufferedReader.close();
- 调整缓冲区大小
- 根据实际应用场景,可以调整缓冲流的缓冲区大小,以达到最佳的性能。较大的缓冲区可以减少 I/O 操作的次数,但会占用更多的内存。
- 示例代码:
- 创建自定义缓冲区大小的缓冲流,演示如何设置缓冲区大小。
java
// 自定义缓冲区大小的缓冲流
FileInputStream fis = new FileInputStream("example.txt");
BufferedInputStream bis = new BufferedInputStream(fis, 8192); // 设置缓冲区大小为 8KB
int c;
while ((c = bis.read()) != -1) {
// 读取字符
}
bis.close();
fis.close();
(二)文件操作优化
- 批量读写操作
- 对于文件的读写操作,尽量采用批量读写的方式,而不是逐个字节或逐行读写。批量读写可以减少系统调用的次数,提高效率。
- 示例代码:
- 演示批量写入文件和逐行写入文件的性能差异。
java
// 逐行写入文件
FileWriter fileWriter = new FileWriter("example.txt");
long startTime = System.currentTimeMillis();
for (int i = 0; i < 10000; i++) {
fileWriter.write("Line " + i + "\n");
}
long endTime = System.currentTimeMillis();
System.out.println("逐行写入时间:" + (endTime - startTime) + "ms");
fileWriter.close();
// 批量写入文件
FileWriter fileWriterBatch = new FileWriter("example_batch.txt");
StringBuffer stringBuffer = new StringBuffer();
long startTimeBatch = System.currentTimeMillis();
for (int i = 0; i < 10000; i++) {
stringBuffer.append("Line ").append(i).append("\n");
}
fileWriterBatch.write(stringBuffer.toString());
long endTimeBatch = System.currentTimeMillis();
System.out.println("批量写入时间:" + (endTimeBatch - startTimeBatch) + "ms");
fileWriterBatch.close();
- 文件压缩与解压缩
- 对于大数据量的文件传输或存储,可以考虑采用文件压缩技术。压缩文件可以减少文件的大小,从而减少传输时间或存储空间的占用。
- 示例代码:
- 演示使用 GZIP 压缩和解压缩文件。
java
// 压缩文件
FileInputStream fis = new FileInputStream("example.txt");
GZIPOutputStream gzos = new GZIPOutputStream(new FileOutputStream("example.txt.gz"));
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
gzos.write(buffer, 0, len);
}
gzos.close();
fis.close();
// 解压缩文件
GZIPInputStream gzis = new GZIPInputStream(new FileInputStream("example.txt.gz"));
FileOutputStream fos = new FileOutputStream("example_decompressed.txt");
byte[] bufferDecompress = new byte[1024];
int lenDecompress;
while ((lenDecompress = gzis.read(bufferDecompress)) != -1) {
fos.write(bufferDecompress, 0, lenDecompress);
}
fos.close();
gzis.close();
五、总结
在资源受限的环境下,Java 性能优化是一个综合性的任务,涉及内存管理、算法优化、并发优化和 I/O 操作优化等多个方面。通过合理地应用上述优化策略和技巧,可以显著提高 Java 程序的性能和效率。在实际开发过程中,需要根据具体的业务场景和性能瓶颈,有针对性地进行优化。
例如,在一个内存受限的系统中,可以重点关注内存管理优化,减少不必要的对象创建,使用对象池技术,及时释放资源,避免内存泄漏。在处理大数据量的计算时,可以选择高效的算法,优化时间复杂度和空间复杂度。在多线程环境下,合理使用线程池,避免线程死锁,使用并发数据结构和原子变量类来提高并发性能。在进行文件读写操作时,采用缓冲 I/O,批量读写操作,以及文件压缩与解压缩技术来优化 I/O 性能。
总之,性能优化是一个持续的过程,需要不断地分析、测试和调整。通过不断地实践和积累经验,我们可以更好地应对各种性能挑战,让 Java 程序在资源受限的环境中也能高效运行。
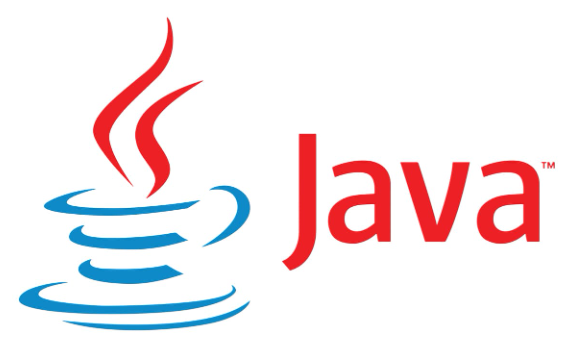