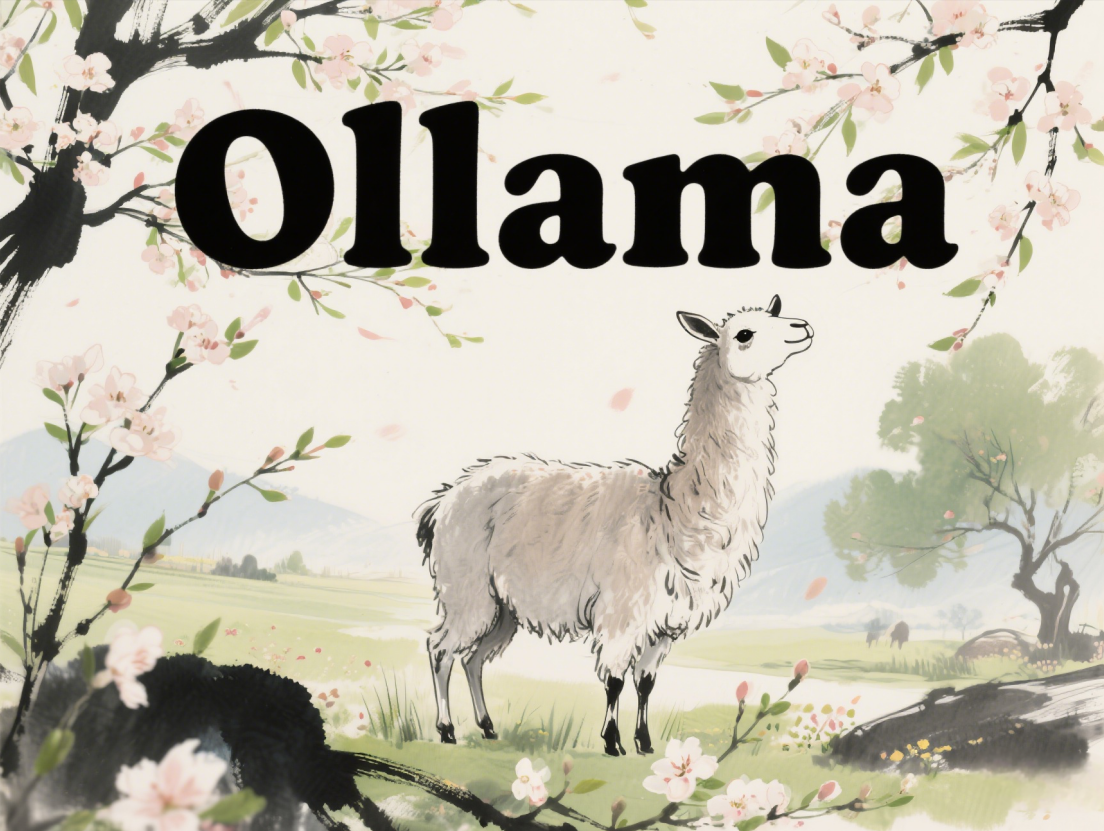
场景:电商客服自动处理退货请求
业务需求:用户通过聊天界面申请退货,模型需调用外部工具验证订单状态、触发退货流程,并返回处理结果。
1. 定义工具列表
在请求中声明模型可调用的工具(函数)及其参数格式(JSON Schema):
python
tools = [
{
"type": "function",
"function": {
"name": "check_order_status", # 工具名称
"description": "根据订单号查询订单状态,判断是否符合退货条件",
"parameters": {
"type": "object",
"properties": {
"order_id": {"type": "string", "description": "用户提供的订单号"}
},
"required": ["order_id"]
}
}
},
{
"type": "function",
"function": {
"name": "initiate_refund", # 工具名称
"description": "触发退货流程,通知物流和财务系统",
"parameters": {
"type": "object",
"properties": {
"order_id": {"type": "string"},
"reason": {"type": "string", "description": "用户填写的退货原因"}
},
"required": ["order_id", "reason"]
}
}
}
]
2. 用户发起对话请求
用户输入退货申请:
python
messages = [
{"role": "user", "content": "我要退货,订单号是ORD123456。退货原因是商品尺寸不符。"}
]
向模型发送请求(启用工具调用):
python
import requests
response = requests.post(
"http://localhost:11434/api/chat",
json={
"model": "llama3:8b-instruct",
"messages": messages,
"tools": tools, # 传递工具定义
"tool_choice": "auto" # 由模型决定是否调用工具
}
)
result = response.json()
3. 解析模型的工具调用请求
模型分析用户输入后,返回需调用的工具及参数:
json
{
"message": {
"role": "assistant",
"content": null,
"tool_calls": [
{
"id": "call_001",
"type": "function",
"function": {
"name": "check_order_status", // 要求调用订单状态查询工具
"arguments": "{\"order_id\": \"ORD123456\"}"
}
}
]
}
}
4. 执行工具函数并返回结果
客户端根据工具名称执行对应的业务逻辑:
python
# 模拟订单状态查询(实际可能调用数据库或内部API)
def check_order_status(order_id):
# 假设订单状态有效且可退货
return {
"status": "success",
"data": {
"order_id": order_id,
"is_refundable": True,
"product": "男士运动鞋",
"purchase_date": "2024-05-01"
}
}
# 获取工具调用参数
tool_call = result["message"]["tool_calls"][0]
args = json.loads(tool_call["function"]["arguments"])
# 执行工具
tool_response = check_order_status(args["order_id"])
# 将结果附加到对话历史
messages.append({
"role": "tool",
"content": json.dumps(tool_response),
"tool_call_id": tool_call["id"] # 关联工具调用ID
})
5. 模型生成最终回复
继续请求模型,传递工具执行结果:
python
response = requests.post(
"http://localhost:11434/api/chat",
json={
"model": "llama3:8b-instruct",
"messages": messages
}
)
final_reply = response.json()["message"]["content"]
print(final_reply)
模型返回:
订单ORD123456(男士运动鞋,购买日期2024-05-01)符合退货条件。已为您启动退货流程,请将商品寄回至仓库地址。物流单号将通过短信发送。
其他业务场景示例
场景1:天气查询助手
- 工具定义:调用天气API获取实时数据。
- 用户输入:"上海今天会下雨吗?"
- 模型行为 :调用
get_weather
工具,参数{"location": "上海"}
。 - 工具响应:返回温度、降水概率。
- 最终回复:"上海今日多云转小雨,气温22-28°C,降水概率60%。"
场景2:数据分析报告生成
- 工具定义 :调用
generate_chart
工具,根据数据生成图表。 - 用户输入:"帮我用柱状图展示2023年季度销售额。"
- 模型行为 :调用工具,参数
{"data_type": "sales", "chart_type": "bar"}
。 - 工具响应:返回图表URL或Base64图片。
- 最终回复:Markdown格式嵌入图表,并总结趋势。
场景3:智能家居控制
- 工具定义 :调用
control_device
工具,操作IoT设备。 - 用户输入:"把客厅的灯调暗一点。"
- 模型行为 :调用工具,参数
{"device": "客厅灯", "action": "set_brightness", "value": 50}
。 - 工具响应:返回操作状态。
- 最终回复:"已将客厅灯亮度调整为50%。"
关键优势
- 自动化业务流程:将模型与内部系统(订单、库存、CRM)无缝连接。
- 动态决策能力:模型根据对话上下文自主选择调用工具。
- 减少幻觉:依赖真实数据生成回答,提升准确性。
- 多模态扩展:结合图像生成、语音合成等工具。
注意事项
- 权限控制:限制工具调用的范围,避免未授权操作。
- 错误处理:捕获工具执行异常,返回友好提示(如"暂时无法查询订单,请稍后再试")。
- 输入验证:检查工具参数合法性,防止注入攻击。
- 上下文管理 :确保
tool_call_id
与对话历史关联,避免混淆。
通过工具调用,Ollama 可深度融入企业工作流,实现从"对话"到"行动"的智能化升级。