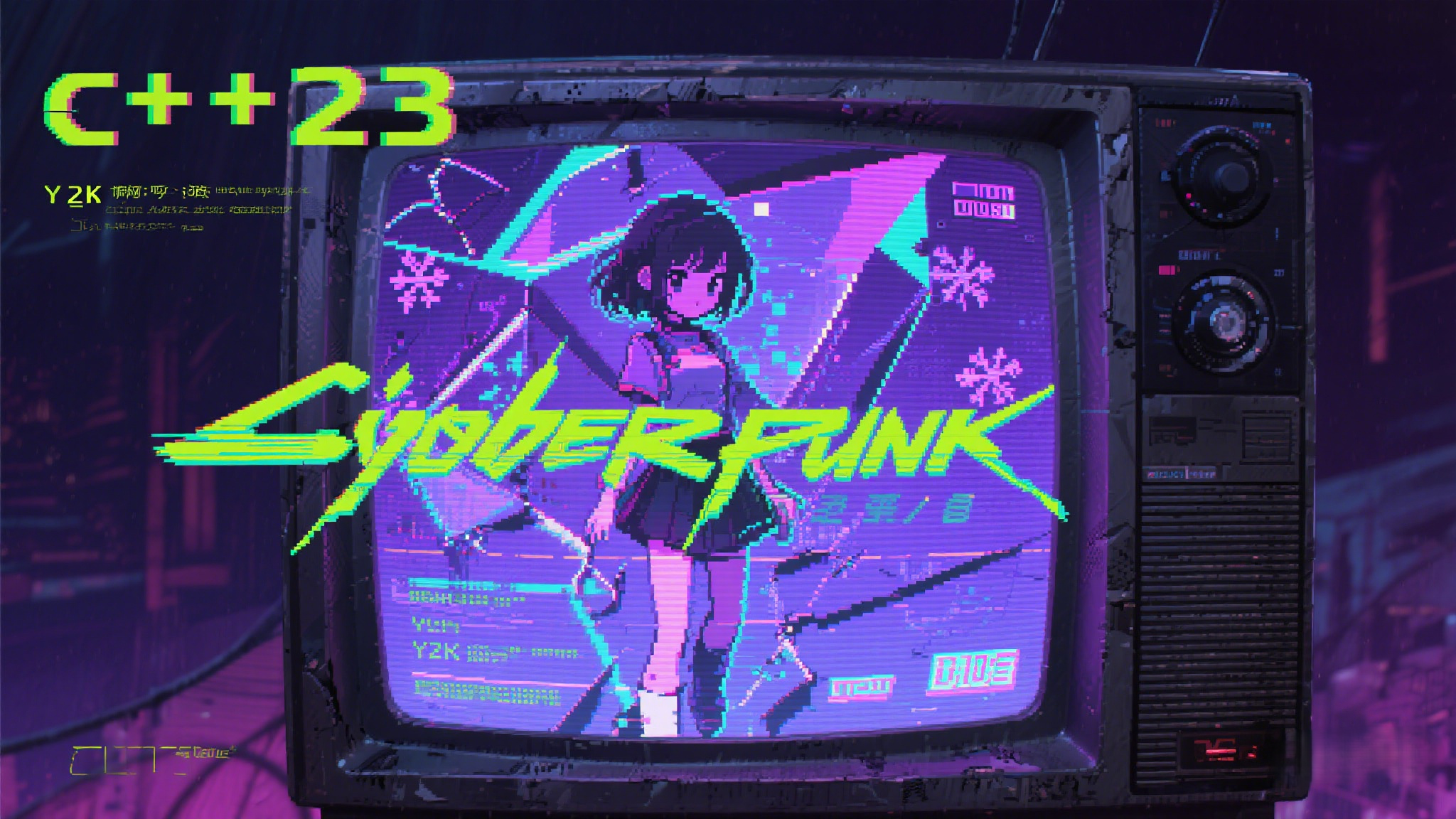
文章目录
引言
在C++的发展历程中,编译时计算一直是一个重要的特性,它可以提高程序的性能和安全性。C++20引入了 consteval
和 std::is_constant_evaluated()
等特性,前者用于声明必须在编译期间完成调用的立即函数,后者用于检查当前是否处于常量求值上下文。而在C++23中,进一步引入了 if consteval
和 if not consteval
语法,使得在代码中区分编译时和运行时行为变得更加方便和灵活。本文将详细介绍 if consteval
和 if not consteval
的相关内容,包括语法规则、设计目的、实际应用场景等。
基本概念
consteval
回顾
在介绍 if consteval
和 if not consteval
之前,我们先回顾一下 consteval
的基本概念。consteval
用于声明立即函数,这类函数的调用必须在编译期间完成。如果尝试在运行时调用 consteval
函数,会导致编译错误。它的目标是确保某些逻辑完全在编译时执行,适用于需要强制编译期计算的场景。例如:
cpp
consteval int square(int x) {
return x * x;
}
constexpr int a = square(5); // 编译时调用
// int b = square(rand()); // 错误:运行时调用
if consteval
和 if not consteval
的定义
if consteval
是C++23引入的编译时条件构造,它允许开发者编写仅在常量求值上下文中执行的代码。而 if not consteval
则是其否定形式,用于编写在非常量求值上下文中执行的代码。
语法规则
if consteval
和 if not consteval
的语法如下:
语法形式 | 描述 |
---|---|
if consteval compound-statement |
如果当前处于常量求值上下文,则执行 compound-statement |
if consteval compound-statement else statement |
如果当前处于常量求值上下文,则执行 compound-statement ;否则执行 statement |
if not consteval compound-statement |
如果当前处于非常量求值上下文,则执行 compound-statement |
if not consteval compound-statement else statement |
如果当前处于非常量求值上下文,则执行 compound-statement ;否则执行 statement |
其中,compound-statement
是复合语句,通常用花括号 {}
括起来;statement
可以是单个语句或复合语句。
设计目的
解决现有问题
在C++20中,虽然有 std::is_constant_evaluated()
可以用于检查当前是否处于常量求值上下文,但使用起来存在一些问题。例如,开发者可能会在运行时进行判断,导致出现"对即时函数的调用不是常量表达式"的错误。而 if consteval
和 if not consteval
则提供了一种更直接、更安全的方式来区分编译时和运行时行为。
增强代码的可读性和可维护性
使用 if consteval
和 if not consteval
可以使代码更加清晰地表达哪些代码是在编译时执行,哪些是在运行时执行,从而提高代码的可读性和可维护性。
提高代码的性能和安全性
通过在编译时执行一些逻辑,可以避免运行时的开销,提高程序的性能。同时,强制某些操作在编译时完成,也可以增强代码的类型安全性。
使用示例
简单示例
cpp
#include <iostream>
consteval int compile_time_abs(int x) {
return x < 0 ? -x : x;
}
constexpr int abs(int x) {
if consteval { // 仅在编译时求值时执行此分支
return compile_time_abs(x);
} else { // 运行时调用
return x < 0 ? -x : x;
}
}
int main() {
constexpr int a = abs(-5); // 编译时调用,使用 if consteval 分支
int b = abs(-10); // 运行时调用,使用 else 分支
std::cout << "a: " << a << ", b: " << b << std::endl;
return 0;
}
在这个示例中,abs
函数根据当前是否处于常量求值上下文,选择不同的执行路径。如果是编译时调用,则使用 compile_time_abs
函数;如果是运行时调用,则直接计算绝对值。
复杂示例
cpp
#include <cmath>
#include <cstdint>
#include <iostream>
constexpr bool is_constant_evaluated() noexcept {
if consteval { return true; } else { return false; }
}
constexpr bool is_runtime_evaluated() noexcept {
if not consteval { return true; } else { return false; }
}
consteval std::uint64_t ipow_ct(std::uint64_t base, std::uint8_t exp) {
if (!base) return base;
std::uint64_t res{1};
while (exp) {
if (exp & 1) res *= base;
exp /= 2;
base *= base;
}
return res;
}
constexpr std::uint64_t ipow(std::uint64_t base, std::uint8_t exp) {
if consteval { // 使用编译时友好的算法
return ipow_ct(base, exp);
} else {
return std::pow(base, exp);
}
}
int main() {
constexpr std::uint64_t c = ipow(2, 10); // 编译时调用
std::uint64_t r = ipow(3, 5); // 运行时调用
std::cout << "c: " << c << ", r: " << r << std::endl;
return 0;
}
在这个示例中,ipow
函数根据当前是否处于常量求值上下文,选择不同的幂运算算法。如果是编译时调用,则使用 ipow_ct
函数;如果是运行时调用,则使用 std::pow
函数。
与其他特性的对比
与 if constexpr
的对比
if constexpr
是C++17引入的特性,用于在编译时进行条件判断。它的条件表达式必须是一个常量表达式,并且会在编译时根据条件的值选择执行哪个分支,未被选择的分支会被丢弃。而 if consteval
则是根据当前是否处于常量求值上下文来选择执行路径,它可以在运行时进行判断。例如:
cpp
template <typename T>
constexpr auto get_value() {
if constexpr (std::is_integral_v<T>) {
return 42;
} else {
return 3.14;
}
}
constexpr int a = get_value<int>(); // 编译时选择分支
与 std::is_constant_evaluated()
的对比
std::is_constant_evaluated()
是C++20引入的库函数,用于检查当前是否处于常量求值上下文。但使用它时需要注意,可能会在运行时进行判断,导致一些意外的错误。而 if consteval
和 if not consteval
则提供了更直接、更安全的方式来区分编译时和运行时行为。例如:
cpp
constexpr int Add(std::span<const int> sp) {
if (std::is_constant_evaluated()) {
// 可能在运行时判断
}
if consteval {
// 仅在编译时判断
}
}
实际应用场景
编译时反射
在编译时反射中,需要生成类型相关的元信息。使用 if consteval
可以确保这些操作在编译时完成,提高程序的性能。例如:
cpp
// 伪代码示例
template <typename T>
consteval auto get_type_info() {
// 编译时生成类型信息
return ...;
}
template <typename T>
constexpr auto print_type_info() {
if consteval {
auto info = get_type_info<T>();
// 处理类型信息
}
}
数学计算
对于需要确保编译时优化的常量计算,使用 if consteval
可以避免运行时的开销。例如:
cpp
consteval int factorial(int n) {
return n <= 1 ? 1 : n * factorial(n - 1);
}
constexpr int a = factorial(5); // 编译时计算
模板元编程
if consteval
可以替代部分模板元编程逻辑,简化代码。例如:
cpp
template <typename T>
constexpr auto process(T value) {
if consteval {
// 编译时处理逻辑
} else {
// 运行时处理逻辑
}
}
类型安全操作
通过强制某些类型转换在编译时完成,可以增强代码的类型安全性。例如:
cpp
template <typename T>
constexpr auto convert(T value) {
if consteval {
// 编译时类型转换
return static_cast<...>(value);
} else {
// 运行时类型转换
return ...;
}
}
总结
C++23中引入的 if consteval
和 if not consteval
语法,为开发者提供了一种更直接、更安全的方式来区分编译时和运行时行为。它可以提高代码的可读性、可维护性、性能和安全性,适用于编译时反射、数学计算、模板元编程、类型安全操作等多种场景。在实际开发中,建议结合最新编译器(如GCC 13+、Clang 16+或MSVC 2022)体验这些特性。