介绍
五子棋,作为一种经典的棋类游戏,不仅考验玩家的策略与判断力,还能在繁忙的生活中带来一丝轻松。今天,我们将用 HTML 、CSS 和 JavaScript 来开发一个简易的五子棋游戏,玩家可以在浏览器中与朋友展开一场刺激的对决。
这篇文章将带你走进游戏开发的基本流程,从零开始创建一个功能完整的五子棋游戏,展示如何通过简单的网页技术打造一个互动性强的游戏。
技术栈
- • HTML:构建页面的结构和棋盘的布局。
- • CSS:使用网格布局(CSS Grid)和样式调整,设计游戏界面。
- • JavaScript:处理用户点击事件、棋盘状态、胜负判定和游戏重置。
项目功能
- • 双人对战:游戏支持两位玩家轮流下棋。
- • 胜利判定:通过检查五子连珠来判定是否有玩家获胜。
- • 游戏重置:点击"重新开始"按钮可以清空棋盘,重新开始一局。
完整代码
以下是实现五子棋游戏的完整代码,包含 HTML、CSS 和 JavaScript:
ini
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<title>五子棋</title>
<style>
body {
font-family: sans-serif;
text-align: center;
background-color: #f5f5f5;
}
h1 {
margin: 20px;
}
#board {
display: grid;
grid-template-columns: repeat(15, 40px);
grid-template-rows: repeat(15, 40px);
margin: 0 auto;
width: 600px;
height: 600px;
background-color: #deb887;
border: 2px solid #333;
}
.cell {
border: 1px solid #999;
position: relative;
}
.piece {
width: 80%;
height: 80%;
border-radius: 50%;
position: absolute;
top: 10%;
left: 10%;
}
.black {
background-color: black;
}
.white {
background-color: white;
border: 1px solid #999;
}
#status {
margin-top: 20px;
font-size: 20px;
}
button {
margin-top: 10px;
padding: 8px 20px;
font-size: 16px;
}
</style>
</head>
<body>
<h1>五子棋(双人对战)</h1>
<div id="board"></div>
<div id="status">当前轮到:黑子</div>
<button onclick="resetGame()">重新开始</button>
<script>
const boardSize = 15;
const board = [];
let currentPlayer = 'black';
let gameOver = false;
const boardElement = document.getElementById('board');
const statusElement = document.getElementById('status');
function createBoard() {
boardElement.innerHTML = '';
for (let i = 0; i < boardSize; i++) {
board[i] = [];
for (let j = 0; j < boardSize; j++) {
board[i][j] = null;
const cell = document.createElement('div');
cell.classList.add('cell');
cell.dataset.x = i;
cell.dataset.y = j;
cell.addEventListener('click', handleClick);
boardElement.appendChild(cell);
}
}
}
function handleClick(e) {
if (gameOver) return;
const x = parseInt(e.target.dataset.x);
const y = parseInt(e.target.dataset.y);
if (board[x][y]) return;
const piece = document.createElement('div');
piece.classList.add('piece', currentPlayer);
e.target.appendChild(piece);
board[x][y] = currentPlayer;
if (checkWin(x, y, currentPlayer)) {
statusElement.textContent = `🎉 玩家 ${currentPlayer === 'black' ? '黑子' : '白子'} 获胜!`;
gameOver = true;
return;
}
currentPlayer = currentPlayer === 'black' ? 'white' : 'black';
statusElement.textContent = `当前轮到:${currentPlayer === 'black' ? '黑子' : '白子'}`;
}
function checkWin(x, y, player) {
const directions = [
[1, 0], [0, 1], [1, 1], [1, -1]
];
for (const [dx, dy] of directions) {
let count = 1;
for (let i = 1; i < 5; i++) {
const nx = x + dx * i;
const ny = y + dy * i;
if (nx >= 0 && nx < boardSize && ny >= 0 && ny < boardSize && board[nx][ny] === player) {
count++;
} else break;
}
for (let i = 1; i < 5; i++) {
const nx = x - dx * i;
const ny = y - dy * i;
if (nx >= 0 && nx < boardSize && ny >= 0 && ny < boardSize && board[nx][ny] === player) {
count++;
} else break;
}
if (count >= 5) return true;
}
return false;
}
function resetGame() {
gameOver = false;
currentPlayer = 'black';
statusElement.textContent = '当前轮到:黑子';
createBoard();
}
createBoard();
</script>
</body>
</html>
效果图
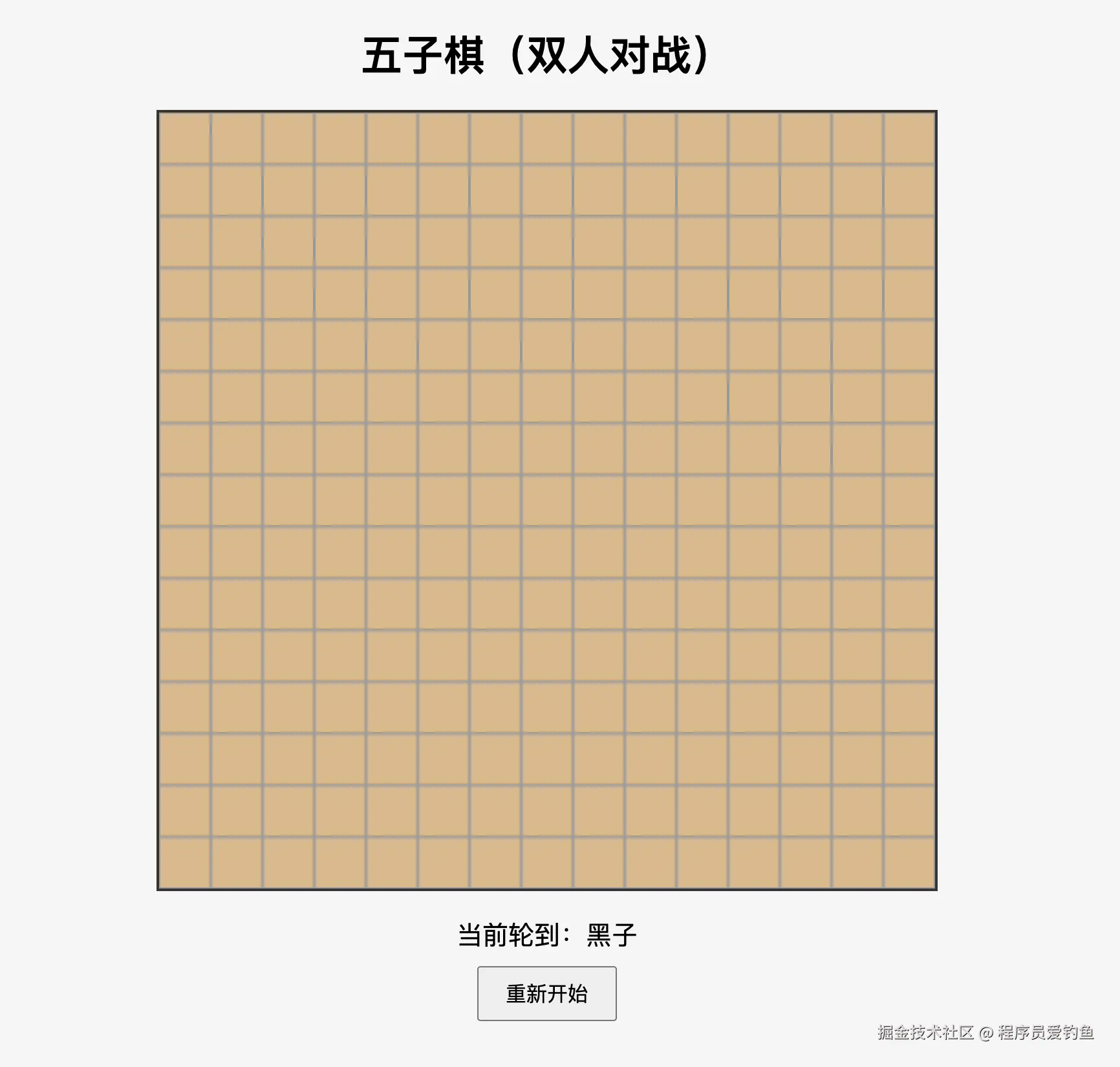
代码讲解
1. HTML 部分:
- •
#board
:这是棋盘的容器,我们使用 CSS Grid 来创建一个 15x15 的网格,每个小格子代表一个棋盘位置。 - •
#status
:显示当前玩家是谁(黑子或白子),以及游戏是否结束的提示。 - •
<button>
:点击此按钮可以重置游戏。
2. CSS 部分:
- • 使用
grid-template-columns
和grid-template-rows
创建一个 15x15 的棋盘网格。 - • 使用
.cell
类来定义每个小格子的边框和大小。 - •
.piece
类控制棋子的样式,给棋子设置圆形外观。
3. JavaScript 部分:
- •
createBoard()
:生成棋盘并为每个格子绑定点击事件。 - •
handleClick()
:处理玩家点击的事件,放置棋子并检查是否有玩家获胜。 - •
checkWin()
:检查当前玩家是否已经完成五子连珠。 - •
resetGame()
:重置游戏状态和棋盘。
小结
通过本篇文章,你已经学会了如何使用 HTML、CSS 和 JavaScript 开发一个基本的五子棋游戏。你可以根据自己的需求,进一步拓展功能,例如增加AI对战、计时器、游戏历史等。
这个项目非常适合初学者,通过简单的实践掌握前端开发中的一些基本概念,并能够为进一步的学习和项目开发打下坚实的基础。
快试试吧,和朋友一起体验这款简单而经典的五子棋游戏!