447_Q1
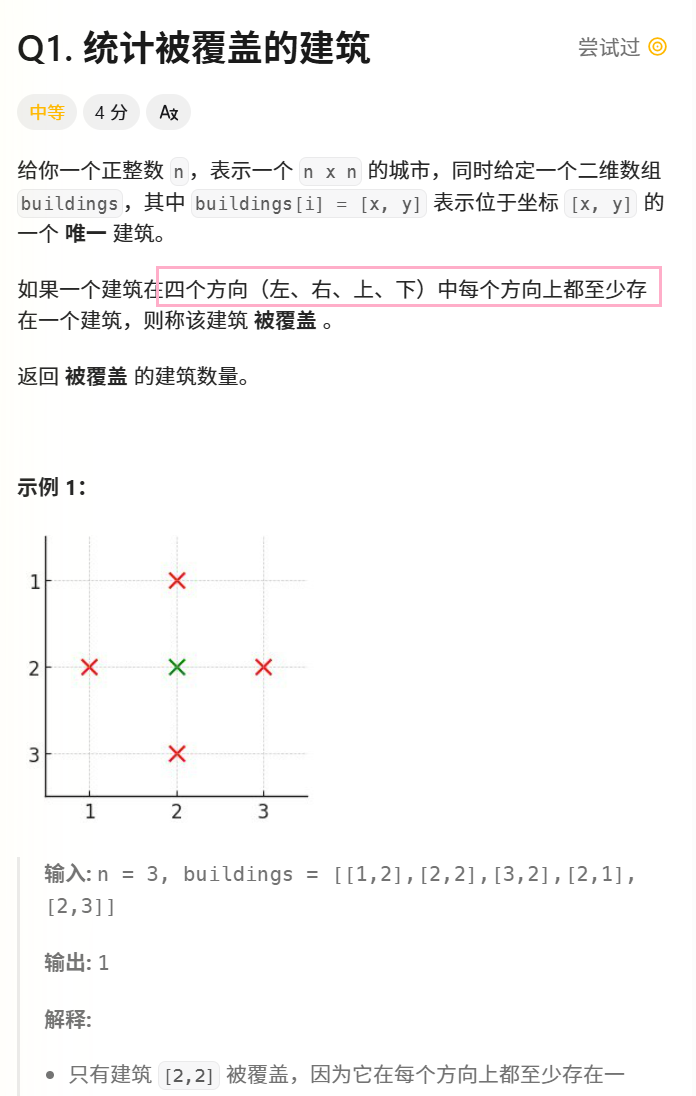
题解
class Solution {
typedef pair<int,int> PII;
// 自定义哈希函数
struct HashPII {
size_t operator()(const PII& p) const {
return hash<int>()(p.first) ^ (hash<int>()(p.second) << 1);
}
};
public:
int countCoveredBuildings(int n, vector<vector<int>>& buildings)
{
//不管有多远 至少存在一个建筑
//bfs变形
//哦不对,hash 快速查找同行同列,不就可以判断了吗
//添加自定义哈希函数HashPII,使用unordered_map<PII, bool, HashPII>解决pair作为键的问题
unordered_map<PII,bool,HashPII> hash;
int ans=0;
for(auto& b:buildings)
{
PII p={b[0],b[1]}; //pair构造和调用 要用{}
hash[p]=true;
}
for(auto& b:buildings)
{
int cnt=0;
//四个 方向的查找
//上
for(int i=b[1]+1;i<=n;i++)
{
if(hash.count({b[0],i}))
{
cnt++;
break;
}
}
for(int i=b[1]-1;i>=1;i--)
{
if(hash.count({b[0],i}))
{
cnt++;
break;
}
}
for(int i=b[0]-1;i>=1;i--)
{
if(hash.count({i,b[1]}))
{
cnt++;
break;
}
}
for(int i=b[0]+1;i<=n;i++)
{
if(hash.count({i,b[1]}))
{
cnt++;
break;
}
}
if(cnt==4) ans++;
}
return ans;
}
};©leetcode
- pair 构造和调用 要用 {}
- !!!!!!!!!!!!!!花括号
超时了
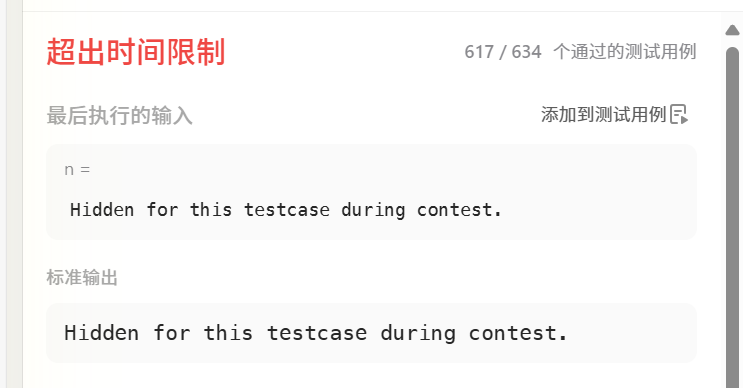
优化
-
采取 行列极值法
class Solution {
public:
int countCoveredBuildings(int n, vector<vector<int>>& buildings) {
// 存 行位置极值和列位置极值
unordered_map<int, int> row_min, row_max;
unordered_map<int, int> col_min, col_max;for (auto& b : buildings) { int x = b[0], y = b[1]; // 预处理行极值 if (!row_min.count(x) || y < row_min[x]) row_min[x] = y; if (!row_max.count(x) || y > row_max[x]) row_max[x] = y; // 预处理列极值 if (!col_min.count(y) || x < col_min[y]) col_min[y] = x; if (!col_max.count(y) || x > col_max[y]) col_max[y] = x; } int ans = 0; for (auto& b : buildings) { int x = b[0], y = b[1]; bool up = (y < row_max[x]); bool down = (y > row_min[x]); bool left = (x > col_min[y]); bool right = (x < col_max[y]); if (up && down && left && right) ans++; } return ans; }
};©leetcode
155_Q1
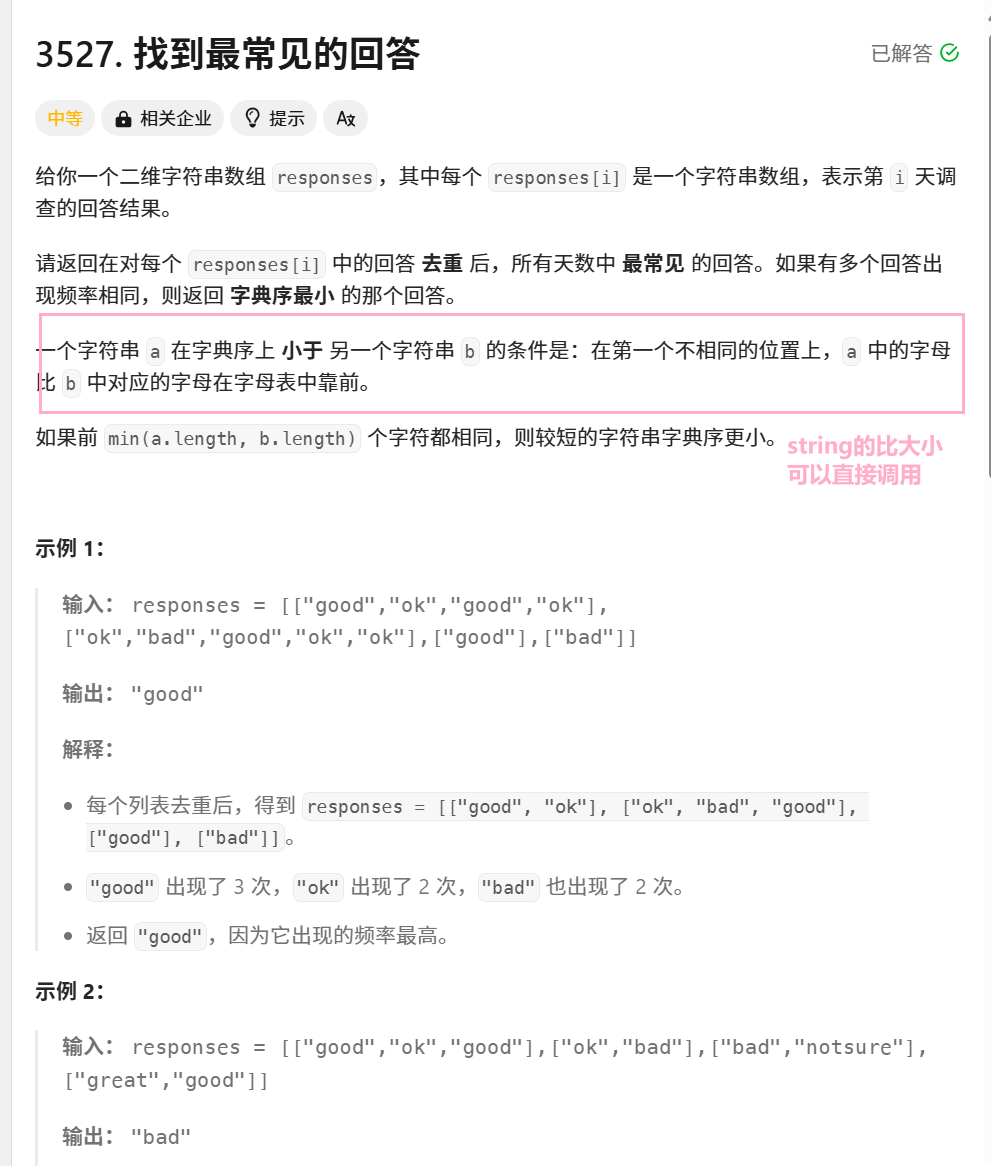
class Solution {
public:
string findCommonResponse(vector<vector<string>>& responses)
{
unordered_map<string,int> hash;
for(auto& re:responses)
{
unordered_map<string,bool> check;
for(auto& str:re)
{
if(!check[str])
{
check[str]=true;
hash[str]++; //确保 每一个 只加一次
}
}
}
pair<string,int> ret(responses[0][0],0);
for(auto& [a,b]:hash)
{
string& f=ret.first;
int& g=ret.second;
if(b==g)
{
if(a<f)
f=a;
}
if(b>g)
{
f=a;
g=b;
}
}
return ret.first;
}
};