1.配置文件设置
打开配置文件redis.windows.conf,配置以下内容:
1.bind 0.0.0.0(设置所有IP可访问)
2.requirepass 1234.com(密码设置)
3.protected-mode no(远程可访问)
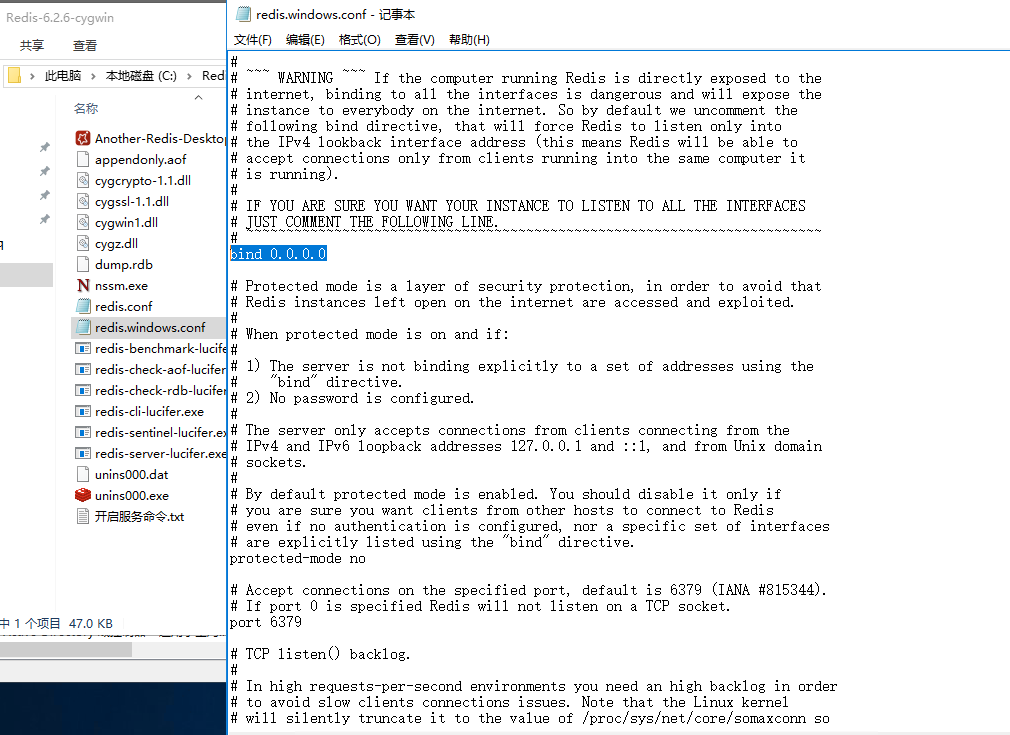
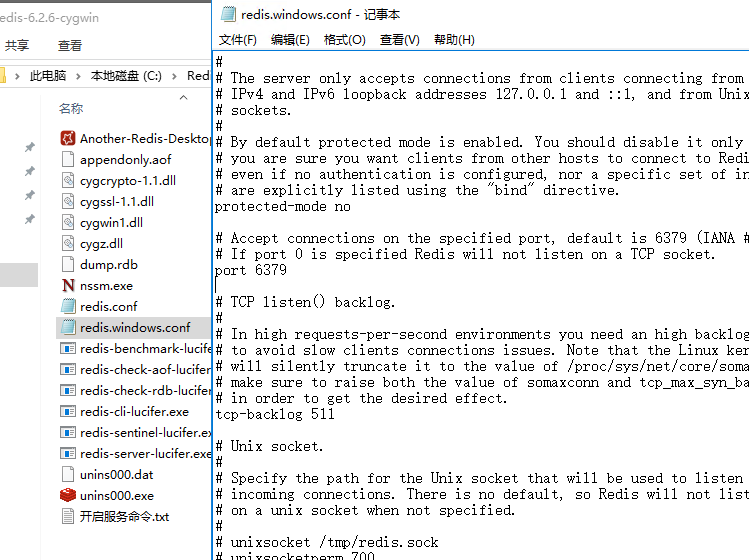
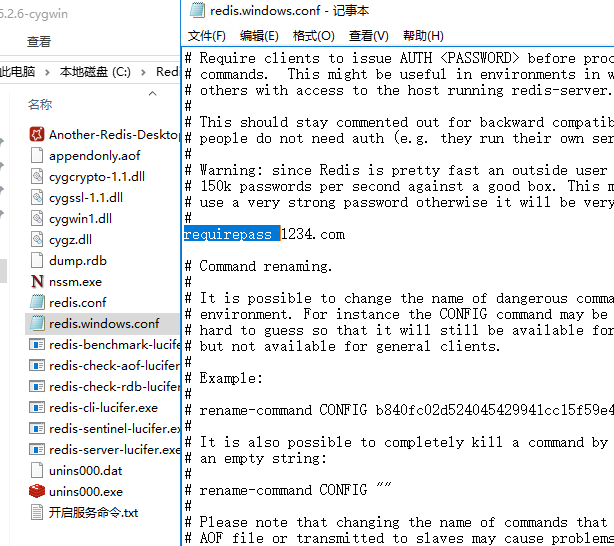
2.防火墙配置入站规则,端口号6379
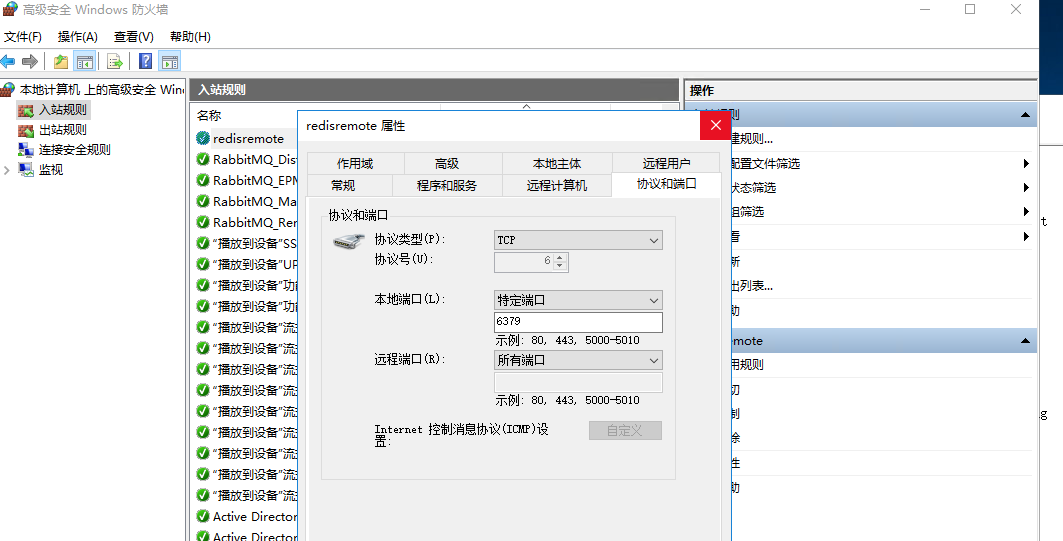
3.安全组配置,开放6379

4.C#代码案例-字符串读写
public static void WriteandReadString()
{
// 连接字符串,根据实际Redis地址和端口调整
string connectionString = "41.162.118.219:6379,password=1234.com";
ConnectionMultiplexer connection = ConnectionMultiplexer.Connect(connectionString);
IDatabase redis = connection.GetDatabase();
// 设置字符串键值对
redis.StringSet("myStringKey", "Hello, Redis!");
// 获取字符串值
string value = redis.StringGet("myStringKey");
Console.WriteLine($"获取的值为: {value}");
connection.Close();
}
5.C#代码案例-哈希读写
public static void WriteandReadHash()
{
// 连接字符串,根据实际Redis地址和端口调整
string connectionString = "41.162.118.219:6379,password=1234.com";
ConnectionMultiplexer connection = ConnectionMultiplexer.Connect(connectionString);
IDatabase redis = connection.GetDatabase();
// 设置哈希字段值
redis.HashSet("myHashKey", new HashEntry[] {
new HashEntry("field1", "value3"),
new HashEntry("field2", "value2")
});
// 获取单个哈希字段值
string field1Value = redis.HashGet("myHashKey", "field1");
Console.WriteLine($"哈希字段field1的值为: {field1Value}");
// 获取所有哈希字段和值
HashEntry[] hashEntries = redis.HashGetAll("myHashKey");
foreach (var entry in hashEntries)
{
Console.WriteLine($"哈希字段: {entry.Name}, 值: {entry.Value}");
}
connection.Close();
}
6.C#代码案例-集合读写
public static void WriteandReadJiHe()
{
// 连接字符串,根据实际Redis地址和端口调整
string connectionString = "41.162.118.219:6379,password=1234.com";
ConnectionMultiplexer connection = ConnectionMultiplexer.Connect(connectionString);
IDatabase redis = connection.GetDatabase();
// 向集合添加元素
redis.SetAdd("mySetKey", "member1");
redis.SetAdd("mySetKey", "member2");
// 获取集合所有成员
RedisValue[] members = redis.SetMembers("mySetKey");
foreach (var member in members)
{
Console.WriteLine($"集合成员: {member}");
}
connection.Close();
}
7.有序集合读写
public static void WriteandReadSortedSet()
{
// 连接字符串,根据实际Redis地址和端口调整
string connectionString = "41.162.118.209:6379,password=1234.com";
ConnectionMultiplexer connection = ConnectionMultiplexer.Connect(connectionString);
IDatabase redis = connection.GetDatabase();
// 向有序集合添加元素及分值
redis.SortedSetAdd("mySortedSetKey", "item1", 5);
redis.SortedSetAdd("mySortedSetKey", "item2", 3);
// 获取元素排名
//long rank = 0;
// rank = redis.SortedSetRank("mySortedSetKey", "item2");
//Console.WriteLine($"元素item2的排名为: {rank}");
//connection.Close();
long? rank = 0;
rank = redis.SortedSetRank("mySortedSetKey", "item2");
Console.WriteLine($"元素的排名为: {rank}");
connection.Close();
}