面向对象编程(OOP)是现代软件开发的核心范式之一,而C++作为一门支持多范式的编程语言,其对面向对象的支持尤为强大。本文将全面探讨C++中类与对象的概念,重点分析成员变量、成员方法以及访问控制机制,帮助读者构建扎实的面向对象编程基础。
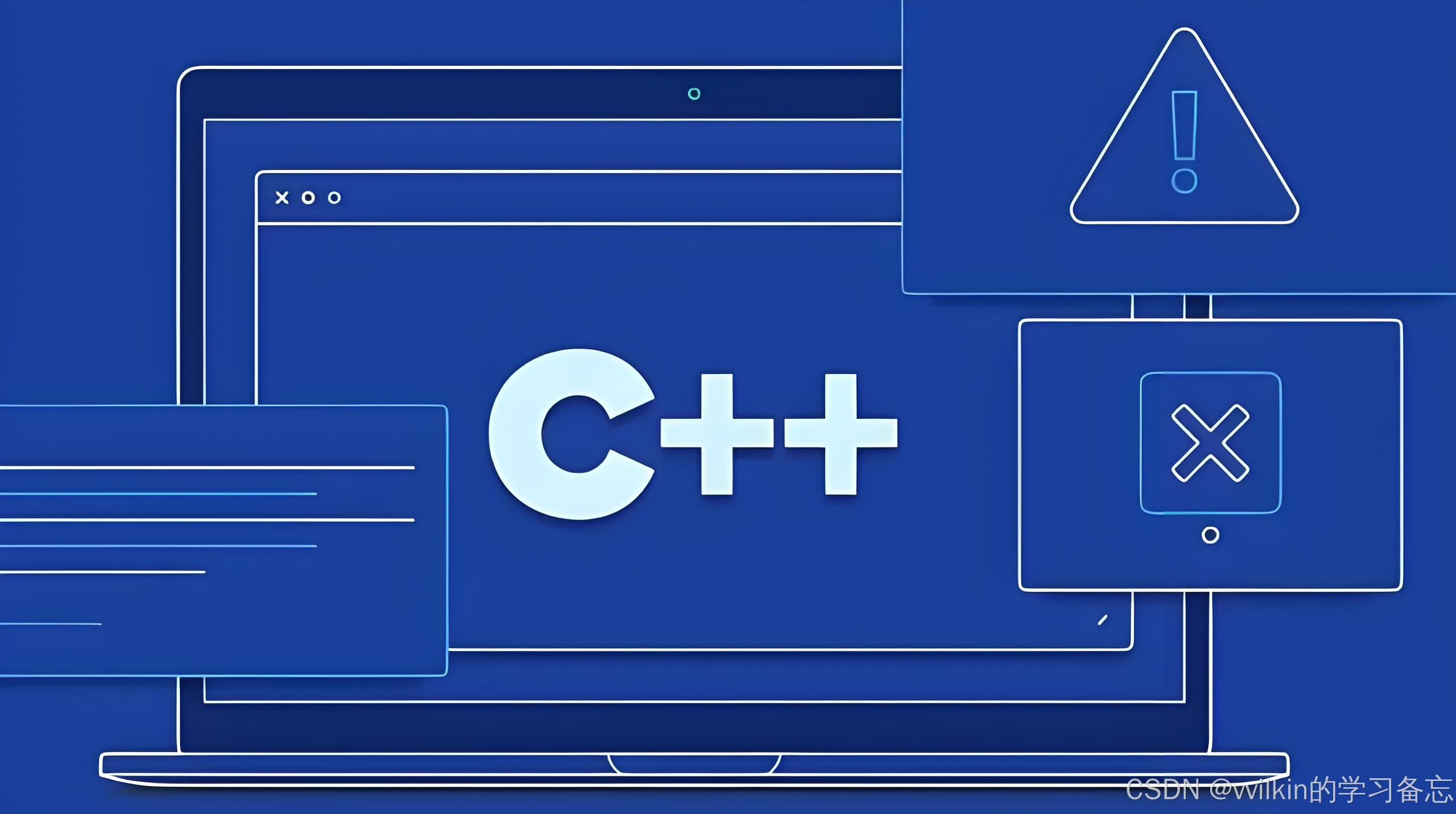
一、类与对象的基本概念
1.1 什么是类
在C++中,**类(class)**是用户自定义的数据类型,它封装了数据(成员变量)和操作这些数据的函数(成员方法)。类可以看作是创建对象的蓝图或模板,定义了对象的结构和行为。
class Car {
private:
std::string brand;
std::string model;
int year;
public:
void accelerate();
void brake();
void displayInfo();
};
1.2 什么是对象
**对象(object)**是类的实例,是根据类的定义创建的具体实体。一个类可以创建多个对象,每个对象都有自己的成员变量副本,但共享相同的成员方法。
Car myCar; // 创建一个Car对象
Car yourCar; // 创建另一个Car对象
二、成员变量详解
2.1 成员变量的定义
成员变量(也称为属性或数据成员)是类中声明的变量,用于描述对象的状态或特征。每个对象都有自己独立的成员变量副本。
class BankAccount {
private:
std::string accountNumber; // 字符串类型成员变量
double balance; // 双精度浮点型成员变量
bool isActive; // 布尔型成员变量
};
2.2 成员变量的初始化
成员变量可以通过多种方式初始化:
-
构造函数初始化列表(推荐方式)
BankAccount::BankAccount(const std::string &num, double bal) : accountNumber(num), balance(bal), isActive(true) {}
-
构造函数体内赋值
BankAccount::BankAccount(const std::string &num, double bal) { accountNumber = num; balance = bal; isActive = true; }
-
类内初始化(C++11起支持)
class BankAccount { private: std::string accountNumber = "00000000"; double balance = 0.0; bool isActive = false; };
2.3 成员变量的生命周期
成员变量的生命周期与其所属对象的生命周期一致:
-
对象创建时,成员变量被创建
-
对象销毁时,成员变量被销毁
-
对于动态分配的对象,成员变量的生命周期由程序员控制
三、成员方法详解
3.1 成员方法的定义
成员方法(也称为成员函数)是类中定义的函数,用于操作成员变量或提供类的功能。
class Rectangle {
private:
double width;
double height;
public:
// 成员方法声明
void setDimensions(double w, double h);
double calculateArea() const;
void display() const;
};
// 成员方法定义
void Rectangle::setDimensions(double w, double h) {
width = w;
height = h;
}
double Rectangle::calculateArea() const {
return width * height;
}
void Rectangle::display() const {
std::cout << "Width: " << width
<< ", Height: " << height
<< ", Area: " << calculateArea() << std::endl;
}
3.2 特殊的成员方法
3.2.1 构造函数
构造函数在对象创建时自动调用,用于初始化对象:
class Student {
private:
std::string name;
int id;
double gpa;
public:
// 默认构造函数
Student() : name(""), id(0), gpa(0.0) {}
// 参数化构造函数
Student(const std::string &n, int i, double g)
: name(n), id(i), gpa(g) {}
// 委托构造函数(C++11)
Student(const std::string &n) : Student(n, 0, 0.0) {}
};
3.2.2 析构函数
析构函数在对象销毁时自动调用,用于清理资源:
class FileHandler {
private:
FILE* filePtr;
public:
FileHandler(const char* filename) {
filePtr = fopen(filename, "r");
}
~FileHandler() {
if(filePtr) {
fclose(filePtr);
std::cout << "File closed successfully." << std::endl;
}
}
};
3.2.3 拷贝控制成员
class String {
private:
char* str;
size_t length;
public:
// 拷贝构造函数
String(const String &other) {
length = other.length;
str = new char[length + 1];
strcpy(str, other.str);
}
// 拷贝赋值运算符
String& operator=(const String &other) {
if(this != &other) {
delete[] str;
length = other.length;
str = new char[length + 1];
strcpy(str, other.str);
}
return *this;
}
// 移动构造函数(C++11)
String(String &&other) noexcept
: str(other.str), length(other.length) {
other.str = nullptr;
other.length = 0;
}
// 移动赋值运算符(C++11)
String& operator=(String &&other) noexcept {
if(this != &other) {
delete[] str;
str = other.str;
length = other.length;
other.str = nullptr;
other.length = 0;
}
return *this;
}
};
3.3 const成员方法
const成员方法承诺不修改对象状态:
class Complex {
private:
double real;
double imag;
public:
// const成员方法
double getReal() const { return real; }
double getImag() const { return imag; }
// 非const成员方法
void setReal(double r) { real = r; }
void setImag(double i) { imag = i; }
};
四、访问控制机制
4.1 三种访问修饰符
-
public:在任何地方都可以访问
-
private:只能在类内部访问(默认访问级别)
-
protected:在类内部和派生类中可以访问
class AccessExample {
public:
int publicVar; // 公有成员void publicMethod() { privateVar = 10; // 可以访问private成员 protectedVar = 20; // 可以访问protected成员 }
protected:
int protectedVar; // 受保护成员void protectedMethod() { privateVar = 30; // 可以访问private成员 }
private:
int privateVar; // 私有成员void privateMethod() { protectedVar = 40; // 可以访问protected成员 }
};
4.2 访问控制的最佳实践
-
数据隐藏原则:成员变量通常设为private,通过public方法访问
-
最小权限原则:只暴露必要的方法和属性
-
接口与实现分离:public方法构成类的接口,private方法处理实现细节
class Temperature {
private:
double celsius; // 内部以摄氏度存储public:
// 接口方法
void setCelsius(double c) { celsius = c; }
void setFahrenheit(double f) { celsius = (f - 32) * 5 / 9; }double getCelsius() const { return celsius; } double getFahrenheit() const { return celsius * 9 / 5 + 32; }
};
五、this指针
每个成员函数都有一个隐式的this
指针,指向调用该函数的对象:
class Point {
private:
int x;
int y;
public:
void setX(int x) {
this->x = x; // 使用this区分成员变量和参数
}
void setY(int y) {
this->y = y;
}
Point& move(int dx, int dy) {
x += dx;
y += dy;
return *this; // 返回当前对象的引用,支持链式调用
}
};
六、静态成员
静态成员属于类本身而不是特定对象:
6.1 静态成员变量
class Employee {
private:
static int count; // 静态成员变量声明
int id;
std::string name;
public:
Employee(const std::string &n) : name(n) {
id = ++count; // 每次创建对象时递增计数器
}
static int getCount() { return count; }
};
int Employee::count = 0; // 静态成员变量定义和初始化
6.2 静态成员方法
静态成员方法只能访问静态成员,没有this指针:
class MathUtils {
public:
static double pi() { return 3.141592653589793; }
static int max(int a, int b) {
return (a > b) ? a : b;
}
};
// 使用静态方法
double circleArea = MathUtils::pi() * radius * radius;
int larger = MathUtils::max(x, y);
七、类的高级特性
7.1 友元(friend)
友元可以突破访问限制,谨慎使用:
class Box {
private:
double width;
public:
friend void printWidth(Box box); // 友元函数
friend class BoxPrinter; // 友元类
};
void printWidth(Box box) {
std::cout << "Width: " << box.width << std::endl;
}
class BoxPrinter {
public:
void print(const Box &box) {
std::cout << "Box width: " << box.width << std::endl;
}
};
7.2 嵌套类
类中可以定义其他类:
class University {
private:
class Department { // 嵌套类
private:
std::string name;
int numStudents;
public:
Department(const std::string &n) : name(n), numStudents(0) {}
void enrollStudent() { numStudents++; }
};
Department cs{"Computer Science"};
Department math{"Mathematics"};
public:
void enrollCSStudent() { cs.enrollStudent(); }
void enrollMathStudent() { math.enrollStudent(); }
};
总结
C++的类与对象机制为面向对象编程提供了强大的支持。通过合理使用成员变量、成员方法和访问控制,我们可以:
-
实现数据的封装与隐藏
-
构建清晰的类接口
-
确保数据的完整性和安全性
-
创建可重用、可维护的代码结构
掌握这些基础知识是成为高效C++程序员的关键一步,也是理解更高级面向对象概念(如继承、多态等)的必要前提。在实际开发中,应当根据具体需求合理设计类的结构,遵循面向对象的设计原则,编写出高质量的C++代码。