示例:
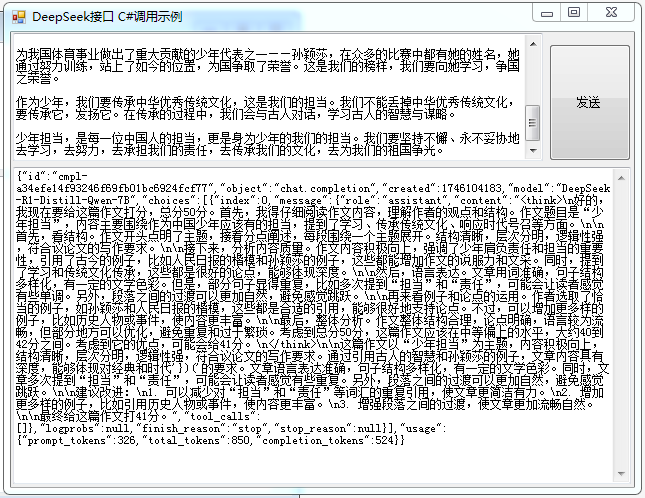
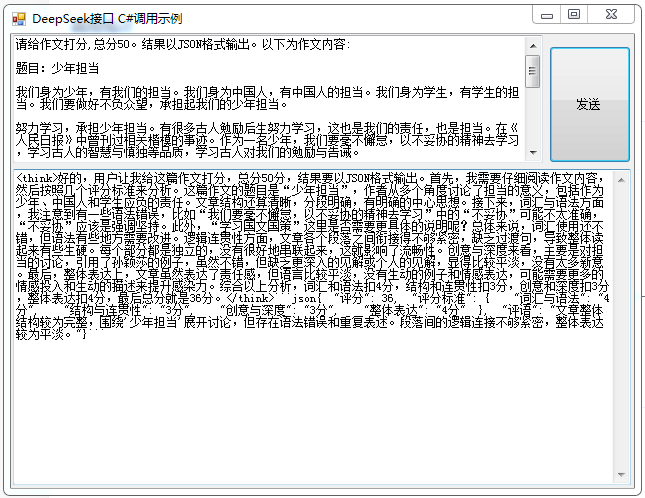
DeepSeek官方接口说明文档:对话补全 | DeepSeek API Docs
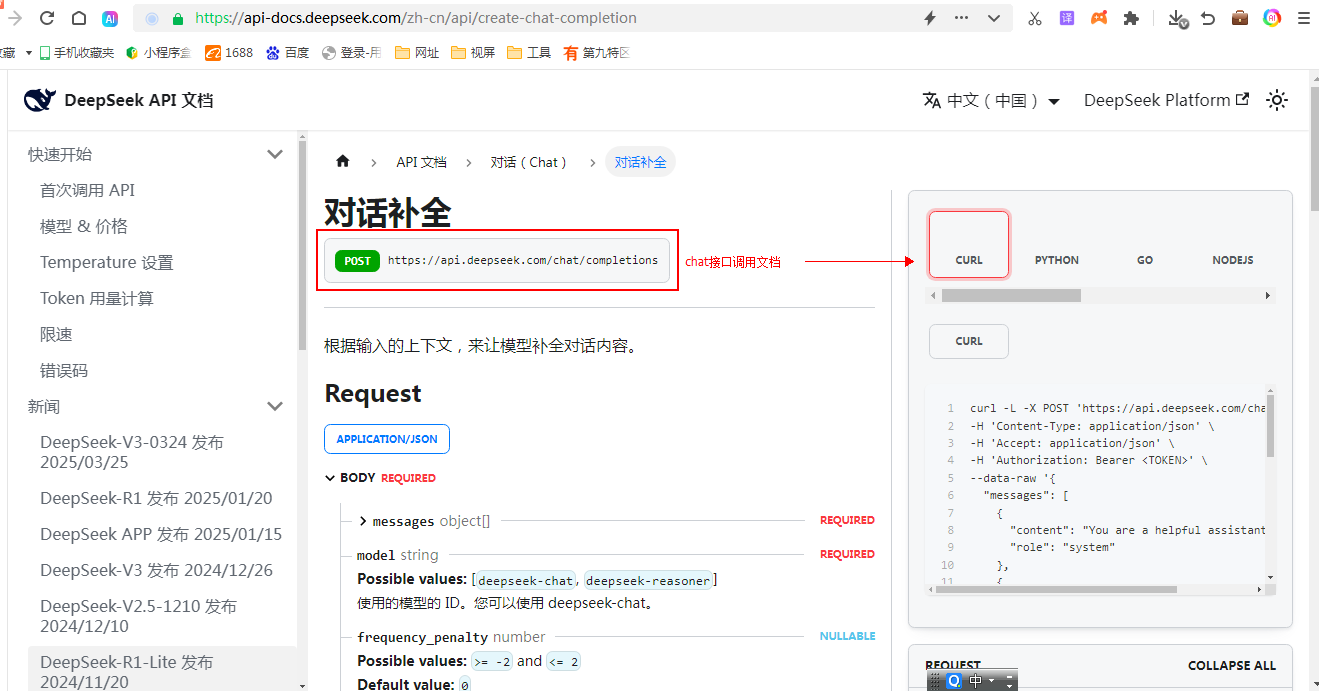
官网暂未提供C#代码实现:(以下为根据CURL接口C#代码调用)
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net.Http;
using Newtonsoft.Json;
using System.Net;
using System.IO;
namespace DeepSeekDemo
{
// DeepSeek API接口(无免费试用,接口需付费开通)
// https://api-docs.deepseek.com/zh-cn/api/create-chat-completion
public class DeepSeekChatService
{
private const string API_KEY = "sk-b2eda36fc94032a966427d01a4e0**"; // 修改为自己申请的KEY值: https://platform.deepseek.com/api_keys
private const string API_ENDPOINT = "https://api.deepseek.com/chat/completions";
private const string CHAT_MODEL = "deepseek-chat"; // 根据实际模型名称修改
private static readonly HttpClient _httpClient = new HttpClient();
public async Task<string> ChatAsync(string userMessage)
{
try
{
// 构造对话请求体
var requestData = new
{
model = CHAT_MODEL,
messages = new[]
{
new { role = "user", content = userMessage }
},
temperature = 1,
max_tokens = 500
};
System.Net.ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12;
string content = JsonConvert.SerializeObject(requestData);
//string response0 = Request(API_ENDPOINT, API_KEY, content);
//return response0;
// 设置认证头
_httpClient.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Bearer", API_KEY);
// 发送请求
var response = await _httpClient.PostAsync(
API_ENDPOINT,
new StringContent(content, Encoding.UTF8, "application/json")
);
// 处理响应
if (response.IsSuccessStatusCode)
{
var jsonResponse = await response.Content.ReadAsStringAsync();
try
{
var result = JsonConvert.DeserializeObject<ChatResponse>(jsonResponse);
return result.choices[0].message.content;
}
catch { return jsonResponse; }
}
else
{
return "API错误: " + response.StatusCode + "\r\n" + response.ToString();
}
}
catch (Exception ex)
{
return "请求异常: " + ex.Message + "\r\n" + ex.ToString();
}
}
// 响应模型
public class ChatResponse
{
public Choice[] choices { get; set; }
public class Choice
{
public Message message { get; set; }
}
public class Message
{
public string role { get; set; }
public string content { get; set; }
}
}
//POST / HTTP/1.1
//Content-Type: application/json;charset=UTF-8
//Authorization: Bearer sk-b02eda3a6fc94032a9626427d0c1a4e0
//Host: 127.0.0.1:30001
//Content-Length: 120
//Expect: 100-continue
//{"model":"deepseek-chat","messages":[{"role":"user","content":"测试"}],"temperature":0.7,"max_tokens":500,"stream":false}
public string ChatAsync2(string userMessage)
{
try
{
// 构造对话请求体
var requestData = new
{
model = CHAT_MODEL,
messages = new[]
{
new { role = "user", content = userMessage }
},
temperature = 1,
max_tokens = 500
};
System.Net.ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12;
string content = JsonConvert.SerializeObject(requestData);
string response0 = Request(API_ENDPOINT, API_KEY, content);
return response0;
}
catch (Exception ex)
{
return "请求异常: " + ex.Message + "\r\n" + ex.ToString();
}
}
/// <summary>
/// 执行http请求
/// </summary>
/// <param name="url">请求网址</param>
/// <param name="postData">需要通过post提交的数据</param>
/// <returns>返回服务器端响应结果</returns>
public static string Request(string url, string API_KEY, string postData = null)
{
string result = "";
if (string.IsNullOrEmpty(url)) return "";
try
{
// 创建Request请求
var request = (HttpWebRequest)WebRequest.Create(url);
// 提交POStT数据
if (!string.IsNullOrEmpty(postData))
{
request.Method = "POST";
request.ContentType = "application/json;charset=UTF-8";
request.Headers.Add("Authorization: Bearer " + API_KEY);
byte[] bytes = Encoding.UTF8.GetBytes(postData);
request.ContentLength = bytes.Length;
Stream stream = request.GetRequestStream();
stream.Write(bytes, 0, bytes.Length);
stream.Close();
}
// 获取返回的响应结果
try
{
var response = (HttpWebResponse)request.GetResponse();
if (response != null)
{
Stream responseStream = response.GetResponseStream();
StreamReader reader = new StreamReader(responseStream, Encoding.UTF8);
result = reader.ReadToEnd();
reader.Close();
responseStream.Close();
}
}
catch (Exception)
{
result = "4004, url对应的服务器,未返回响应信息!";
}
}
catch (Exception ex)
{
result = ex.ToString();
//result = "4003, url对应的服务器,不存在!";
}
return result;
}
}
}
因为deepseek官方API已经开始收费了,测试都要收费。所以又搜到了国家超算中心,支持暂时试用。就试了下。可以用。同样的接口实现。不同的参数。
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net.Http;
using Newtonsoft.Json;
using System.Net;
using System.IO;
namespace DeepSeekDemo
{
// 国家互联网超算中心DeepSeek API接口(可免费试用,但模型非最新)
//https://www.scnet.cn/ac/openapi/doc/2.0/moduleapi/api/chat.html
public class DeepSeekChatService2
{
private const string API_KEY = "sk-Mjg5LTExNDEwNzMzMDg0LTE3NDYwOTkyNzgyOTc="; // 这个是我的,暂时还可以试用,后面试用量结束不续费会自动关闭。你修改为修改为自己申请的KEY值: https://www.scnet.cn/ui/llm/apikeys
private const string API_ENDPOINT = "https://api.scnet.cn/api/llm/v1/chat/completions";
//private const string CHAT_MODEL = "DeepSeek-R1-Distill-Qwen-7B"; // 根据实际模型名称修改 https://www.scnet.cn/ac/openapi/doc/2.0/moduleapi/tutorial/modulefee.html
private const string CHAT_MODEL = "DeepSeek-R1-Distill-Qwen-32B";
private static readonly HttpClient _httpClient = new HttpClient();
public async Task<string> ChatAsync(string userMessage)
{
try
{
// 构造对话请求体
var requestData = new
{
model = CHAT_MODEL,
messages = new[]
{
new { role = "user", content = userMessage }
},
temperature = 1,
max_tokens = 4096
};
System.Net.ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12;
string content = JsonConvert.SerializeObject(requestData);
//string response0 = Request(API_ENDPOINT, API_KEY, content);
//return response0;
// 设置认证头
_httpClient.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Bearer", API_KEY);
// 发送请求
var response = await _httpClient.PostAsync(
API_ENDPOINT,
new StringContent(content, Encoding.UTF8, "application/json")
);
// 处理响应
if (response.IsSuccessStatusCode)
{
var jsonResponse = await response.Content.ReadAsStringAsync();
try
{
var result = JsonConvert.DeserializeObject<ChatResponse>(jsonResponse);
return result.choices[0].message.content;
}
catch { return jsonResponse; }
}
else
{
return "API错误: " + response.StatusCode + "\r\n" + response.ToString();
}
}
catch (Exception ex)
{
return "请求异常: " + ex.Message + "\r\n" + ex.ToString();
}
}
// 响应模型
public class ChatResponse
{
public Choice[] choices { get; set; }
public class Choice
{
public Message message { get; set; }
}
public class Message
{
public string role { get; set; }
public string content { get; set; }
}
}
//POST / HTTP/1.1
//Content-Type: application/json;charset=UTF-8
//Authorization: Bearer sk-b02eda3a6fc94032a9626427d0c1a4e0
//Host: 127.0.0.1:30001
//Content-Length: 120
//Expect: 100-continue
//{"model":"deepseek-chat","messages":[{"role":"user","content":"测试"}],"temperature":0.7,"max_tokens":500,"stream":false}
public string ChatAsync2(string userMessage)
{
try
{
// 构造对话请求体
var requestData = new
{
model = CHAT_MODEL,
messages = new[]
{
new { role = "user", content = userMessage }
},
temperature = 1,
max_tokens = 500
};
System.Net.ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12;
string content = JsonConvert.SerializeObject(requestData);
string response0 = Request(API_ENDPOINT, API_KEY, content);
return response0;
}
catch (Exception ex)
{
return "请求异常: " + ex.Message + "\r\n" + ex.ToString();
}
}
/// <summary>
/// 执行http请求
/// </summary>
/// <param name="url">请求网址</param>
/// <param name="postData">需要通过post提交的数据</param>
/// <returns>返回服务器端响应结果</returns>
public static string Request(string url, string API_KEY, string postData = null)
{
string result = "";
if (string.IsNullOrEmpty(url)) return "";
try
{
// 创建Request请求
var request = (HttpWebRequest)WebRequest.Create(url);
// 提交POStT数据
if (!string.IsNullOrEmpty(postData))
{
request.Method = "POST";
request.ContentType = "application/json;charset=UTF-8";
request.Headers.Add("Authorization: Bearer " + API_KEY);
byte[] bytes = Encoding.UTF8.GetBytes(postData);
request.ContentLength = bytes.Length;
Stream stream = request.GetRequestStream();
stream.Write(bytes, 0, bytes.Length);
stream.Close();
}
// 获取返回的响应结果
try
{
var response = (HttpWebResponse)request.GetResponse();
if (response != null)
{
Stream responseStream = response.GetResponseStream();
StreamReader reader = new StreamReader(responseStream, Encoding.UTF8);
result = reader.ReadToEnd();
reader.Close();
responseStream.Close();
}
}
catch (Exception)
{
result = "4004, url对应的服务器,未返回响应信息!";
}
}
catch (Exception ex)
{
result = ex.ToString();
//result = "4003, url对应的服务器,不存在!";
}
return result;
}
}
}
C#调用接口示例:
cs
private void button1_Click(object sender, EventArgs e)
{
button1.Enabled = false;
button1.Text = "已发送";
string Title = this.Text;
this.Text += " - DeepSeek处理中,请稍后...";
string chatContent = "有些人在想着怎么干活更省力,有些人在想着怎么捅刀更隐蔽,什么原因?";
Chat(chatContent, respone =>
{
textOutPut.Text = respone.Replace("\n", "\r\n");
button1.Enabled = true;
button1.Text = "发送";
this.Text = Title;
});
}
DeepSeekChatService2 chatService = new DeepSeekChatService2();
public async void Chat(string input, Action<string> callBack)
{
// 使用示例
var response = await chatService.ChatAsync(input);
//Console.WriteLine(response);
/* 预期响应格式示例:
{
"choices": [
{
"message": {
"role": "assistant",
"content": "<think>
嗯,这个问题挺有意思的,为什么会有人想着怎么干活省力,而另一些人却想着怎么捅刀更隐蔽呢?首先,我需要理解这两类人的想法和他们背后的动机是什么。
先说第一种人,想着怎么干活更省力。这部分人可能是出于以下几个原因。首先,效率。他们可能觉得如果能找到更高效的方法完成工作,可以节省时间和精力,从而提高生产力。比如说,使用工具、优化流程或者学习新的技能,这些都是为了减少重复劳动,提升工作效率。其次,可能是因为他们担心过度劳累或者受伤,所以想找更安全、更健康的工作方式。或者是他们对工作的兴趣不高,想要尽快完成任务,腾出时间去做其他事情。
然后是第二种人,想着怎么捅刀更隐蔽,这部分人的动机可能比较复杂。首先,可能是因为他们对某种权威、体系或者权力结构感到不满。他们可能觉得现有的规则或者管理方式对他们不利,所以想通过"捅刀"的方式来表达不满或者破坏现状。另外,也有可能是出于自私的目的,想要获取更多的资源或者权力,通过破坏他人来达到自己的目的。"隐蔽"则可能是因为他们害怕被发现,所以选择偷偷摸摸的方式进行,避免引起注意或者受到惩罚。
为什么会有人选择这两条完全不同的方向呢?可能是因为他们的价值观、背景、经历以及所处的环境不同。比如说,想着干活更省力的人可能处于一个鼓励创新、提高效率的工作环境中,而想着捅刀的人可能处于一个竞争激烈、勾心斗角的环境中,迫使他们采取更极端的方式。
另外,这可能也与他们的个人性格有关。想着干活更省力的人可能更倾向于合作、解决问题,而想着捅刀的人可能更倾向于对抗、破坏。也有可能是他们对现状的不满程度不同,前者可能只是想优化自己的工作方式,而后者则是对整个体系的不信任或者敌意。
还有一个角度是社会压力和个人目标的不同。想着干活更省力的人可能有明确的目标,希望通过提高效率来实现自己的职业发展或者个人成就。而想着捅刀的人可能感到挫败,认为现有的途径无法实现自己的目标,所以采取破坏性的方式来进行报复或者改变现状。
此外,还可能有文化和教育背景的影响。不同的文化对工作和处事方式有不同的价值观,有些人可能受到鼓励去创新和提高效率,而另一些人可能在某些文化背景下被鼓励对抗和破坏。
总的来说,这两种行为反映了一个人的内心世界、所处环境和对现状的态度。要想改变这种状况,可能需要从教育、社会环境、心理健康等多个方面入手,帮助人们找到建设性的方式来表达不满或者解决问题,而不是选择破坏性的行为。
</think>
有些人想着如何干活更省力,是因为他们可能追求更高的工作效率、减轻工作负担,或者希望在工作中寻求更好的解决方案。而另一些人想着如何捅刀更隐蔽,则可能是出于对权力结构的不满、隐藏的动机,或者为了个人利益而采取的不当手段。这两种行为反映了个人的价值观、所处环境以及对问题的应对方式的不同。"
}
}
]
}
*/
if (callBack != null) callBack(response);
}