- 操作系统:ubuntu22.04
- OpenCV版本:OpenCV4.9
- IDE:Visual Studio Code
- 编程语言:C++11
算法描述
cv::bioinspired::Retina 是 OpenCV 中用于仿生视觉处理的一个类,它基于生物视觉模型进行图像预处理。该算法特别适用于动态范围调整、边缘增强、颜色恒常性等复杂场景。
主要特点
- 高效性:能够在保持较高检测准确性的同时实现较快的处理速度。
- 适应性强:能够很好地适应光照变化和其他环境变化。
- 参数可调:允许用户通过调整多个参数来优化背景建模过程。
- 基于生物视觉模型:利用了视网膜的 Parvo 和 Magno 通道模型,从而提高了对细节和运动信息的捕捉能力。
构造函数
与大多数现代 OpenCV 视觉处理工具一样,不直接使用构造函数创建 Retina 实例,而是通过工厂方法 cv::bioinspired::Retina::create() 来创建实例。
cpp
Ptr<Retina> cv::bioinspired::Retina::create
(
cv::Size(width, height), // 图像尺寸
bool colorMode = true, // 是否启用颜色处理
bool useRetinaLogSampling = false, // 是否使用对数采样(模拟人眼非均匀采样)
int reductionFactor = 1, // 对数采样时的降维因子
float samplingStrength = 0.5f // 采样强度
);
参数:
- width, height: 输入图像的宽度和高度。
- colorMode: 是否启用颜色处理,默认为 true。
- useRetinaLogSampling: 是否使用对数采样,默认为 false。
- reductionFactor: 对数采样时的降维因子,默认为 1。
- samplingStrength: 采样强度,默认为 0.5f。
主要方法
apply函数
函数原型
cpp
void apply
(
InputArray inputImage
);
参数
- inputImage: 输入原始图像。
getParvo 函数
函数原型
cpp
void getParvo
(
OutputArray retinaParvoOutput
);
参数
- retinaParvoOutput: 输出 Parvo 通道结果(颜色和细节信息)。
getMagno函数
函数原型
cpp
void getMagno
(
OutputArray retinaMagnoOutput
);
参数
- retinaMagnoOutput: 输出 Magno 通道结果(运动和瞬态响应)。
setupOPLandIPLParvoChannel函数
函数原型
cpp
void setupOPLandIPLParvoChannel
(
const std::string retinaParameterFile = "",
bool applyDefaultSetupOnFailure = true
);
参数
- retinaParameterFile: 可选配置文件路径。
- applyDefaultSetupOnFailure: 如果加载配置失败是否应用默认设置。
setupIPLMagnoChannel函数
函数原型
cpp
void setupIPLMagnoChannel
(
const std::string retinaParameterFile = "",
bool applyDefaultSetupOnFailure = true
);
参数
- retinaParameterFile: 可选配置文件路径。
- applyDefaultSetupOnFailure: 如果加载配置失败是否应用默认设置。
write函数
函数原型
cpp
void write
(
const String& filename
) const;
参数
- filename: 将当前参数保存到 XML/YAML 文件。
read函数
函数原型
cpp
void read
(
const String& filename
);
参数
- filename: 从 XML/YAML 文件中读取参数并应用。
代码示例
cpp
#include <opencv2/bioinspired.hpp>
#include <opencv2/opencv.hpp>
int main()
{
// 加载输入图像
cv::Mat inputImage = cv::imread( "/media/dingxin/data/study/OpenCV/sources/images/Lenna.png" );
if ( inputImage.empty() )
{
std::cerr << "无法加载图像!" << std::endl;
return -1;
}
// 创建 Retina 实例
cv::Ptr< cv::bioinspired::Retina > retina = cv::bioinspired::Retina::create( inputImage.size(), true );
// 设置 Parvo 和 Magno 通道参数(使用默认配置)
retina->setupOPLandIPLParvoChannel(); // Parvo 通道设置
retina->setupIPLMagnoChannel(); // Magno 通道设置
// 运行 Retina 处理
retina->run( inputImage );
// 获取输出结果
cv::Mat parvoOutput, magnoOutput;
retina->getParvo( parvoOutput );
retina->getMagno( magnoOutput );
// 显示结果
cv::imshow( "Input Image", inputImage );
cv::imshow( "Retina Parvo Output", parvoOutput );
cv::imshow( "Retina Magno Output", magnoOutput );
cv::waitKey( 0 ); // 等待按键退出
return 0;
}
运行结果
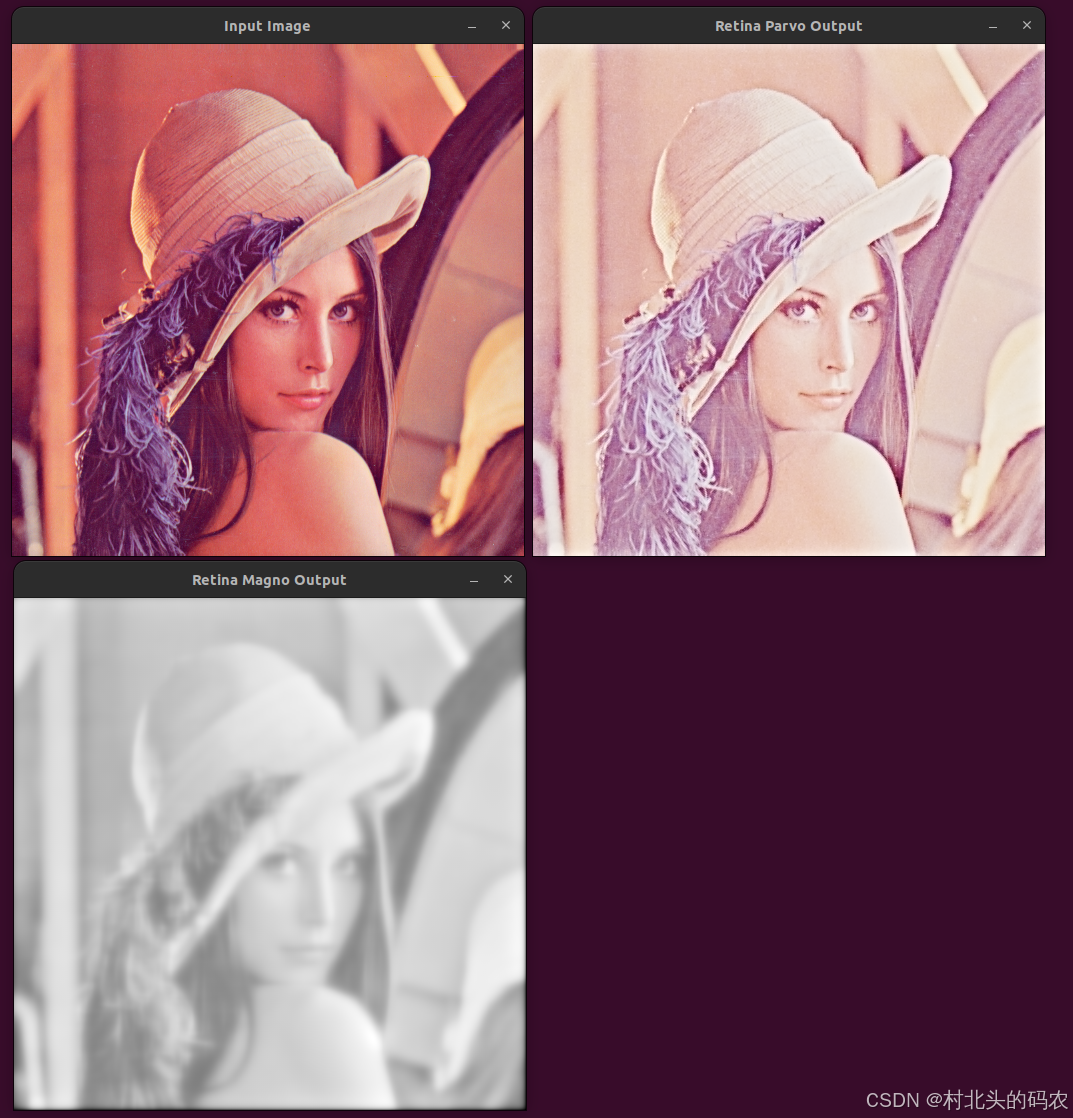